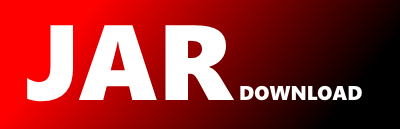
kdux.StoreBuilder.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Kdux Show documentation
Show all versions of Kdux Show documentation
Kdux is a Kotlin-based, platform-agnostic state management library that implements the Redux pattern,
providing structured concurrency with built-in coroutine support. It is designed to integrate seamlessly
with any Kotlin project, particularly excelling in Android applications using MVI architecture.
package org.mattshoe.shoebox.kdux
/**
* A builder class for constructing instances of a [Store] in a Redux-like architecture. The `StoreBuilder`
* allows for the configuration of middleware, enhancers, and a custom store creation function, enabling
* flexible and customizable store initialization.
*
* This class follows the standard builder pattern, allowing for the chaining of methods to add middleware,
* enhancers, and to define a custom store creation process. Once configured, the `build()` function can be
* called to create the fully constructed [Store].
*
* @param State The type of state that the store will manage.
* @param Action The type of actions that the store will handle.
* @param initialState The initial state that the store will start with.
* @param reducer The reducer that will handle state transitions based on dispatched actions.
*/
internal class StoreBuilder(
private val initialState: State,
private val reducer: Reducer
) {
private val middlewares = mutableListOf>()
private val enhancers = mutableListOf>()
private var storeCreatorLambda: (() -> Store)? = null
private var storeCreator: StoreCreator? = null
/**
* Adds a middleware to the builder, allowing it to be included in the store's processing pipeline.
* This function can be chained.
*
* @param middleware The middleware to be added to the builder.
* @return The current [StoreBuilder] instance, allowing for method chaining.
*/
operator fun plus(middleware: Middleware): StoreBuilder {
middlewares.add(middleware)
return this
}
/**
* Adds one or more middleware to the builder. This function can be chained.
*
* @param middlewares Vararg parameter for adding multiple middleware at once.
* @return The current [StoreBuilder] instance, allowing for method chaining.
*/
fun add(vararg middlewares: Middleware): StoreBuilder {
this.middlewares.addAll(middlewares)
return this
}
/**
* Adds an enhancer to the builder, allowing it to modify or extend the behavior of the store.
* This function can be chained.
*
* @param enhancer The enhancer to be added to the builder.
* @return The current [StoreBuilder] instance, allowing for method chaining.
*/
operator fun plus(enhancer: Enhancer): StoreBuilder {
enhancers.add(enhancer)
return this
}
/**
* Adds one or more enhancers to the builder. This function can be chained.
*
* @param enhancers Vararg parameter for adding multiple enhancers at once.
* @return The current [StoreBuilder] instance, allowing for method chaining.
*/
fun add(vararg enhancers: Enhancer): StoreBuilder {
this.enhancers.addAll(enhancers)
return this
}
/**
* Sets a custom store creation function. This allows for creating a custom store instance rather than
* relying on the default store creation logic.
*
* @param creator A lambda function that returns a custom [Store] instance.
* @return The current [StoreBuilder] instance, allowing for method chaining.
*/
fun store(creator: () -> Store): StoreBuilder {
this.storeCreatorLambda = creator
return this
}
/**
* Sets a custom store creation function using a [StoreCreator]. This allows for creating a custom store
* instance using a predefined [StoreCreator] interface implementation, rather than relying on a lambda function.
*
* @param creator A [StoreCreator] instance that defines the custom store creation logic.
* @return The current [StoreBuilder] instance, allowing for method chaining.
*/
fun storeCreator(creator: StoreCreator): StoreBuilder {
this.storeCreator = creator
return this
}
/**
* Builds and returns the fully configured [Store] instance. This method applies all the configured
* middleware and enhancers in the order they were added.
*
* @return A fully constructed and enhanced [Store] instance.
*/
fun build(): Store {
var store = storeCreator?.createStore()
?: this.storeCreatorLambda?.invoke()
?: DefaultStore(
initialState,
reducer,
middlewares
)
// Apply all enhancers in the order they were created.
enhancers.forEach { enhancer ->
store = enhancer.enhance(store)
}
return store
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy