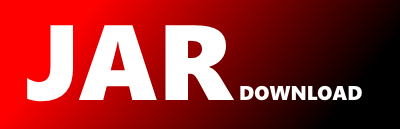
kdux.tools.BufferEnhancer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Kdux Show documentation
Show all versions of Kdux Show documentation
Kdux is a Kotlin-based, platform-agnostic state management library that implements the Redux pattern,
providing structured concurrency with built-in coroutine support. It is designed to integrate seamlessly
with any Kotlin project, particularly excelling in Android applications using MVI architecture.
package kdux.tools
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.sync.Mutex
import kotlinx.coroutines.sync.withLock
import org.mattshoe.shoebox.kdux.Enhancer
import org.mattshoe.shoebox.kdux.Store
/**
* An enhancer that buffers dispatched actions until a specified buffer size is reached.
* Once the buffer is full, all buffered actions are dispatched to the store at once.
*
* This enhancer is useful in scenarios where you want to delay processing of actions
* and batch them together to minimize state updates or improve performance.
*
* When buffer is flushed, actions are dispatched in the order they entered the buffer.
*
* @param bufferSize The size of the buffer that determines when the buffered actions
* should be dispatched. Must be greater than zero.
*
* @throws IllegalArgumentException if `bufferSize` is less than or equal to zero.
*/
class BufferEnhancer(
private val bufferSize: Int
): Enhancer {
init {
require(bufferSize > 0) {
"Buffer size must be greater than zero."
}
}
override fun enhance(store: Store): Store {
return object : Store {
private val bufferMutex = Mutex()
private val buffer = mutableListOf()
override val state: Flow
get() = store.state
override val currentState: State
get() = store.currentState
override suspend fun dispatch(action: Action) {
val actionsToDispatch = mutableListOf()
bufferMutex.withLock {
buffer.add(action)
if (buffer.size >= bufferSize) {
actionsToDispatch.addAll(buffer)
buffer.clear()
}
}
actionsToDispatch.forEach {
store.dispatch(it)
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy