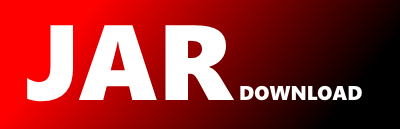
org.mayanjun.mybatisx.dal.handler.SpringAutoDiscoveryProcessor Maven / Gradle / Ivy
/*
* Copyright 2016-2018 mayanjun.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.mayanjun.mybatisx.dal.handler;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.core.OrderComparator;
import org.springframework.util.CollectionUtils;
import java.util.*;
import java.util.concurrent.CopyOnWriteArrayList;
/**
* SpringAutoDiscoveryProcessor
*
* @author mayanjun(9/20/16)
*/
public abstract class SpringAutoDiscoveryProcessor implements AutoDiscoveryProcessor, InitializingBean, ApplicationContextAware {
protected ApplicationContext applicationContext;
private List handlers = new CopyOnWriteArrayList();
private volatile boolean handlersDiscovered;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.applicationContext = applicationContext;
}
@Override
public void afterPropertiesSet() throws Exception {
List hs = discoverHandlers();
if(!CollectionUtils.isEmpty(hs)) {
this.handlers.addAll(hs);
}
}
protected abstract List findHandlers(Class type);
@Override
public List discoverHandlers() {
synchronized (this) {
List handlers = new ArrayList();
if(handlersDiscovered) return handlers;
Class[] types = getAutoDiscoveryTypes();
if(types != null && types.length > 0) {
for(Class type : types) {
List hs = findHandlers(type);
if(!CollectionUtils.isEmpty(hs)) handlers.addAll(hs);
}
}
if(!handlers.isEmpty()) {
Collections.sort(handlers, new OrderComparator());
}
handlersDiscovered = true;
return handlers;
}
}
@Override
public T getHandler(Object source) {
for(T handler : handlers) if(handler.supports(source)) return handler;
return null;
}
@Override
public AutoDiscoveryProcessor setHandlers(T... handlers) {
if(handlers != null && handlers.length > 0) {
this.handlers.addAll(Arrays.asList(handlers));
}
return this;
}
public List getHandlers() {
return handlers;
}
protected Set packageSet() {
String[] packages = getPackages();
Set ps = null;
if(packages != null && packages.length > 0) {
ps = new TreeSet(Arrays.asList(packages));
}
return ps;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy