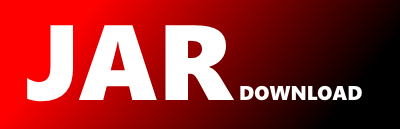
org.mazarineblue.runner.swing.TaskPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of MazarineBlue-Runner-Standalone Show documentation
Show all versions of MazarineBlue-Runner-Standalone Show documentation
The Runner module contains the user interface. Currently only a
standalone is provided.
The newest version!
/*
* Copyright (c) 2012-2014 Alex de Kruijff
* Copyright (c) 2014-2015 Specialisterren
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU Affero General Public License
* as published by the Free Software Foundation; either version 3
* of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*/
package org.mazarineblue.runner.swing;
import java.awt.Color;
import java.io.File;
import java.util.Date;
import java.util.concurrent.ExecutionException;
import javax.swing.Action;
import javax.swing.JFrame;
import javax.swing.SwingWorker;
import org.mazarineblue.runner.tasks.Task;
/**
*
* @author Alex de Kruijff {@literal }
*/
public class TaskPanel
extends javax.swing.JPanel
implements Comparable {
private final Task task;
private static final Color black = new Color(0, 0, 0);
private final Color unclickedColor;
private final Color clickedColor;
// Variables declaration - do not modify//GEN-BEGIN:variables
private org.jdesktop.swingx.JXBusyLabel busyLabel;
private javax.swing.JLabel delayLabel;
private javax.swing.JLabel delayTimeLabel;
private javax.swing.JLabel fileLabel;
private javax.swing.JLabel fileLocation;
private org.jdesktop.swingx.JXHyperlink log;
private org.jdesktop.swingx.JXHyperlink report;
private javax.swing.JLabel scheduledDate;
private javax.swing.JLabel schedulesLabel;
private javax.swing.JCheckBox selectCheckBox;
private javax.swing.JLabel sheetLabel;
private javax.swing.JLabel sheetName;
private org.jdesktop.swingx.JXHyperlink status;
// End of variables declaration//GEN-END:variables
/**
* Creates new form TaskPanel
*/
public TaskPanel(Task task) {
this.task = task;
Date scheduled = task.getScheduledDate();
initComponents();
scheduledDate.setText(scheduled == null ? "now" : scheduled.toString());
setFileLocation(task);
sheetName.setText(task.getSheetName());
delayTimeLabel.setText("" + task.getDelay() + " ms");
unclickedColor = status.getUnclickedColor();
clickedColor = status.getClickedColor();
status.setUnclickedColor(black);
status.setClickedColor(black);
status.setRolloverEnabled(false);
}
private void setFileLocation(Task task) {
String location = task.getSourceLocation();
fileLocation.setText(location);
fileLocation.setToolTipText(location);
int width = fileLocation.getMaximumSize().width;
if (fileLocation.getPreferredSize().width <= width)
return;
int pos = location.length() / 2;
String first = location.substring(0, pos);
String last = location.substring(pos);
while (fileLocation.getPreferredSize().width > width) {
fileLocation.setText(first + "....." + last);
first = location.substring(0, --pos);
last = last.substring(1);
}
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
busyLabel = new org.jdesktop.swingx.JXBusyLabel();
selectCheckBox = new javax.swing.JCheckBox();
schedulesLabel = new javax.swing.JLabel();
scheduledDate = new javax.swing.JLabel();
report = new org.jdesktop.swingx.JXHyperlink();
log = new org.jdesktop.swingx.JXHyperlink();
status = new org.jdesktop.swingx.JXHyperlink();
fileLabel = new javax.swing.JLabel();
fileLocation = new javax.swing.JLabel();
sheetLabel = new javax.swing.JLabel();
sheetName = new javax.swing.JLabel();
delayLabel = new javax.swing.JLabel();
delayTimeLabel = new javax.swing.JLabel();
setBorder(javax.swing.BorderFactory.createEtchedBorder());
schedulesLabel.setText("Scheduled");
scheduledDate.setText("Mon Sep 22 11:55:00 CEST 2014");
report.setText("Report");
report.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
reportActionPerformed(evt);
}
});
log.setText("Logfile");
log.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
logActionPerformed(evt);
}
});
status.setText("WAITING");
status.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
statusActionPerformed(evt);
}
});
fileLabel.setText("File");
fileLocation.setText("W:\\WebDriver\\alexk\\Mazarine\\Cookbook.xlsx");
fileLocation.setMaximumSize(new java.awt.Dimension(350, 14));
sheetLabel.setText("Sheet");
sheetName.setText("Main");
delayLabel.setText("Delay");
delayTimeLabel.setText("0 ms");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(busyLabel, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(selectCheckBox))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(schedulesLabel)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(scheduledDate)
.addGap(18, 18, Short.MAX_VALUE)
.addComponent(report, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(log, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(status, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(sheetLabel)
.addComponent(fileLabel)
.addComponent(delayLabel))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(delayTimeLabel)
.addComponent(fileLocation, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(sheetName))))
.addContainerGap())
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(layout.createSequentialGroup()
.addComponent(busyLabel, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(selectCheckBox))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(schedulesLabel)
.addComponent(scheduledDate)
.addComponent(report, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(log, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(status, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(fileLabel)
.addComponent(fileLocation, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(sheetLabel)
.addComponent(sheetName))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(delayLabel)
.addComponent(delayTimeLabel))))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
}// //GEN-END:initComponents
private Exception exception;
void setException(ExecutionException ex) {
this.exception = ex;
}
private void statusActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_statusActionPerformed
JFrame frame = new TaskFrame(task, exception);
frame.setLocationByPlatform(true);
frame.setVisible(true);
}//GEN-LAST:event_statusActionPerformed
private void logActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_logActionPerformed
new SwingWorker() {
@Override
protected Object doInBackground()
throws Exception {
task.openLog();
return null;
}
}.execute();
}//GEN-LAST:event_logActionPerformed
private void reportActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_reportActionPerformed
new SwingWorker() {
@Override
protected Object doInBackground()
throws Exception {
task.openReport();
return null;
}
}.execute();
}//GEN-LAST:event_reportActionPerformed
void cancle() {
task.cancle();
}
void pauseOrResume() {
if (task.isPaused())
task.resume();
else
task.pause();
}
boolean isSelected() {
return selectCheckBox.isSelected();
}
public void setBusy(boolean flag) {
busyLabel.setBusy(flag);
}
public void setStatus(String status, boolean enableLink) {
this.status.setText(status);
this.status.setRolloverEnabled(enableLink);
this.status.setUnclickedColor(enableLink ? unclickedColor : black);
this.status.setClickedColor(enableLink ? clickedColor : black);
}
void setLog(File file) {
Action action = new OpenWebBrowserAction(file);
report.setAction(action);
}
boolean after(TaskPanel other) {
return task.after(other.task);
}
boolean before(TaskPanel other) {
return task.before(other.task);
}
@Override
public int compareTo(TaskPanel other) {
return task.compareTo(other.task);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy