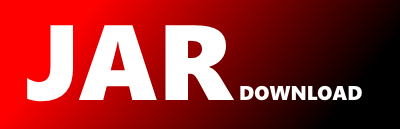
org.meanbean.factories.BasicNewObjectInstanceFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of meanbean Show documentation
Show all versions of meanbean Show documentation
Mean Bean is an open source Java test library that tests equals and hashCode contract compliance, as well as JavaBean/POJO getter and setter methods.
package org.meanbean.factories;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.meanbean.lang.Factory;
import org.meanbean.util.SimpleValidationHelper;
import org.meanbean.util.ValidationHelper;
/**
* Concrete Factory that creates instances of the type of object specified during construction of the Factory. Only
* classes that have a no-argument constructor can be successfully instantiated by this Factory. If the class does not
* have a no-argument constructor, an exception will be thrown when create()
is invoked.
*
* @author Graham Williamson
*/
public class BasicNewObjectInstanceFactory implements Factory
© 2015 - 2025 Weber Informatics LLC | Privacy Policy