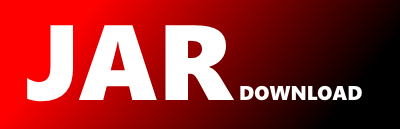
org.mechio.api.animation.Transforms Maven / Gradle / Ivy
/*
* Copyright 2014 the MechIO Project.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.mechio.api.animation;
import java.awt.geom.Point2D;
import java.util.ArrayList;
import java.util.List;
import org.jflux.api.common.rk.utils.Utils;
/**
* Provides functions to transforming Animations, Channels, and Motion Paths.
*
* @author Matthew Stevenson
*/
public class Transforms {
/**
* Moves all the points in a MotionPath by the given x and y amounts.
* @param src the MotionPath defining the starting points. This MotionPath
* is unchanged
* @param dest the MotionPath to set. The Points in dest are set to the
* translated coordinates from src
* @param amtX x amount
* @param amtY y amount
*/
public static void translatePath(MotionPath src, MotionPath dest, double amtX, double amtY){
int min = 0;
for(int i=0; i points = src.getControlPoints();
int len = points.size();
if(scale <= 0 || ref < 0 || len < 2){
return;
}
ref = Utils.bound(ref, points.get(0).getX(), points.get(len-1).getX());
int min = 0;
for(int i=0; i points){
for(int i=0; i scaleTimes(List points, double scale, double ref){
List ret = new ArrayList();
if(scale <= 0 || ref < 0){
return ret;
}
int min = 0;
for(Point2D p : points){
double x = (p.getX()-ref)*scale + ref;
x = Math.max(x,min);
if(x==min){
min++;
}
ret.add(new Point2D.Double(x, p.getY()));
}
return ret;
}
/**
* Scales the y-distance from the reference point by the given amount for
* each point in the list.
*
* @param points the list of points to scale
* @param scale the amount to scale the points
* @param ref the scale reference point
* @return a new list of points with scaled y values
*/
public static List scalePositions(List points, double scale, double ref){
List ret = new ArrayList();
if(scale <= 0 || ref < 0 || ref > 1){
return ret;
}
for(Point2D p : points){
double y = (p.getY()-ref)*scale + ref;
y = Utils.bound(y, 0.0, 1.0);
ret.add(new Point2D.Double(p.getX(), y));
}
return ret;
}
/**
* Moves all the points in the list be the given x and y amounts.
* @param points the points to move
* @param amtX x amount
* @param amtY y amount
* @return a new list of points translated by the given amounts
*/
public static List translatePoints(List points, double amtX, double amtY){
List ret = new ArrayList();
for(Point2D p : points){
ret.add(new Point2D.Double(p.getX()+amtX, p.getY()+amtY));
}
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy