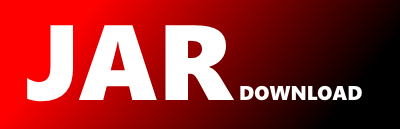
org.meeuw.i18n.languages.ISO_639 Maven / Gradle / Ivy
package org.meeuw.i18n.languages;
import java.util.*;
import java.util.logging.Level;
import java.util.stream.Stream;
import jakarta.validation.constraints.Size;
import org.checkerframework.checker.nullness.qual.PolyNull;
import com.fasterxml.jackson.annotation.JsonCreator;
import static org.meeuw.i18n.languages.ISO_639_3_Code.KNOWN;
/**
* A utility class for working with ISO 639 language codes.
*
* @since 3.1
*/
public class ISO_639 {
static ThreadLocal ignoreNotFound = ThreadLocal.withInitial(() -> Boolean.FALSE);
public static void setIgnoreNotFound() {
ignoreNotFound.set(Boolean.TRUE);
}
public static void removeIgnoreNotFound() {
ignoreNotFound.remove();
}
private ISO_639() {
}
/**
* Retrieves a {@link ISO_639_3_Code} by its Part1 code {@link ISO_639_3_Code#part1()}
*
* @param code A 2 letter language code
* @return An optional containing the {@link ISO_639_3_Code} if found.
*/
public static Optional getByPart1(String code) {
return ISO_639_3_Code
.getByPart1(code)
.map(LanguageCode::updateToEnum);
}
/**
* Retrieves a {@link LanguageCode} by its Part2B ('bibliographic') code {@link ISO_639_3_Code#part2B()}
*
* @param code A 2 or 3 letter language code
* @return An optional containing the {@link ISO_639_3_Code} if found.
*/
public static Optional getByPart2B(String code) {
if (code == null) {
return Optional.empty();
}
final String lowerCode = code.toLowerCase();
return ISO_639_3_Code.stream()
.filter(i -> lowerCode.equals(i.part2B()))
.map(LanguageCode::updateToEnum)
.findFirst();
}
/**
* Retrieves a {@link ISO_639_3_Code} by its Part2T ('terminology') code {@link ISO_639_3_Code#part2T()}
*
* @param code A 2 or 3 letter language code
* @return An optional containing the {@link ISO_639_3_Code} if found.
*/
public static Optional getByPart2T(String code) {
if (code == null) {
return Optional.empty();
}
final String lowerCode = code.toLowerCase();
return KNOWN.values().stream()
.filter(i -> lowerCode.equals(i.part2T()))
.map(LanguageCode::updateToEnum)
.findFirst();
}
/**
* Retrieves a {@link ISO_639_3_Code} by {@link ISO_639_3_Code#part3() its three-letter identifier}
*
* If the given code is a {@link RetiredLanguageCode retired code}, the replacement code is returned if possible. If a retired code is matched, but no single replacement is found, an empty optional is returned, and a warning is logged (using {@link java.util.logging JUL})
*
* @param code A 2 or 3 letter language code
* @return An optional containing the {@link ISO_639_3_Code} if found.
* @since 2.2
*/
public static Optional getByPart3(@Size(min = 3, max = 3) String code, boolean matchRetired) {
return ISO_639_3_Code.getByPart3(code, matchRetired, Level.WARNING)
.map(LanguageCode::updateToEnum)
;
}
/**
* Defaulting version of {@link #getByPart3(String, boolean)}, matching retired codes too.
*/
public static Optional getByPart3(@Size(min = 3, max = 3) String code) {
return getByPart3(code, true);
}
/**
* Retrieves a language family code by its 3 letter code.
*
* @see LanguageFamilyCode#get(String)
*/
public static Optional getByPart5(@Size(min = 3, max = 3) String code) {
return LanguageFamilyCode.get(code);
}
/**
* A stream with all known {@link ISO_639_Code language (or language family) codes} .
*
*
* @return a stream of all known language codes.
*/
public static Stream stream() {
return Stream.concat(
LanguageCode.stream(),
Arrays.stream(LanguageFamilyCode.values())
);
}
public static Stream> streamByNames() {
return Stream.concat(
LanguageCode.streamByNames(),
Arrays.stream(LanguageFamilyCode.values())
.map(l -> new AbstractMap.SimpleEntry<>(l.refName(), l))
);
}
private static final ThreadLocal