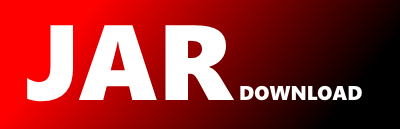
org.meridor.perspective.sql.ParametersParser Maven / Gradle / Ivy
The newest version!
// Generated from org/meridor/perspective/sql/ParametersParser.g4 by ANTLR 4.5.1
package org.meridor.perspective.sql;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class ParametersParser extends Parser {
static { RuntimeMetaData.checkVersion("4.5.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
ID=1, LITERAL=2, COLON=3, POSITIONAL_PLACEHOLDER=4, DELIMITER=5, ANY=6;
public static final int
RULE_queries = 0, RULE_query = 1, RULE_text = 2, RULE_placeholder = 3,
RULE_named_placeholder = 4, RULE_positional_placeholder = 5, RULE_delimiter = 6;
public static final String[] ruleNames = {
"queries", "query", "text", "placeholder", "named_placeholder", "positional_placeholder",
"delimiter"
};
private static final String[] _LITERAL_NAMES = {
null, null, null, "':'", "'?'"
};
private static final String[] _SYMBOLIC_NAMES = {
null, "ID", "LITERAL", "COLON", "POSITIONAL_PLACEHOLDER", "DELIMITER",
"ANY"
};
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "ParametersParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public ParametersParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class QueriesContext extends ParserRuleContext {
public List query() {
return getRuleContexts(QueryContext.class);
}
public QueryContext query(int i) {
return getRuleContext(QueryContext.class,i);
}
public QueriesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_queries; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterQueries(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitQueries(this);
}
}
public final QueriesContext queries() throws RecognitionException {
QueriesContext _localctx = new QueriesContext(_ctx, getState());
enterRule(_localctx, 0, RULE_queries);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(15);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(14);
query();
}
}
setState(17);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==LITERAL || _la==ANY );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QueryContext extends ParserRuleContext {
public List text() {
return getRuleContexts(TextContext.class);
}
public TextContext text(int i) {
return getRuleContext(TextContext.class,i);
}
public DelimiterContext delimiter() {
return getRuleContext(DelimiterContext.class,0);
}
public List placeholder() {
return getRuleContexts(PlaceholderContext.class);
}
public PlaceholderContext placeholder(int i) {
return getRuleContext(PlaceholderContext.class,i);
}
public QueryContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_query; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterQuery(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitQuery(this);
}
}
public final QueryContext query() throws RecognitionException {
QueryContext _localctx = new QueryContext(_ctx, getState());
enterRule(_localctx, 2, RULE_query);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(23);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(19);
text();
setState(21);
_la = _input.LA(1);
if (_la==COLON || _la==POSITIONAL_PLACEHOLDER) {
{
setState(20);
placeholder();
}
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(25);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
setState(28);
_la = _input.LA(1);
if (_la==DELIMITER) {
{
setState(27);
delimiter();
}
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TextContext extends ParserRuleContext {
public TerminalNode ANY() { return getToken(ParametersParser.ANY, 0); }
public TerminalNode LITERAL() { return getToken(ParametersParser.LITERAL, 0); }
public TextContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_text; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterText(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitText(this);
}
}
public final TextContext text() throws RecognitionException {
TextContext _localctx = new TextContext(_ctx, getState());
enterRule(_localctx, 4, RULE_text);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(30);
_la = _input.LA(1);
if ( !(_la==LITERAL || _la==ANY) ) {
_errHandler.recoverInline(this);
} else {
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class PlaceholderContext extends ParserRuleContext {
public Named_placeholderContext named_placeholder() {
return getRuleContext(Named_placeholderContext.class,0);
}
public Positional_placeholderContext positional_placeholder() {
return getRuleContext(Positional_placeholderContext.class,0);
}
public PlaceholderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_placeholder; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterPlaceholder(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitPlaceholder(this);
}
}
public final PlaceholderContext placeholder() throws RecognitionException {
PlaceholderContext _localctx = new PlaceholderContext(_ctx, getState());
enterRule(_localctx, 6, RULE_placeholder);
try {
setState(34);
switch (_input.LA(1)) {
case COLON:
enterOuterAlt(_localctx, 1);
{
setState(32);
named_placeholder();
}
break;
case POSITIONAL_PLACEHOLDER:
enterOuterAlt(_localctx, 2);
{
setState(33);
positional_placeholder();
}
break;
default:
throw new NoViableAltException(this);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Named_placeholderContext extends ParserRuleContext {
public List COLON() { return getTokens(ParametersParser.COLON); }
public TerminalNode COLON(int i) {
return getToken(ParametersParser.COLON, i);
}
public TerminalNode ID() { return getToken(ParametersParser.ID, 0); }
public Named_placeholderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_named_placeholder; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterNamed_placeholder(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitNamed_placeholder(this);
}
}
public final Named_placeholderContext named_placeholder() throws RecognitionException {
Named_placeholderContext _localctx = new Named_placeholderContext(_ctx, getState());
enterRule(_localctx, 8, RULE_named_placeholder);
try {
enterOuterAlt(_localctx, 1);
{
setState(36);
match(COLON);
setState(37);
match(ID);
setState(38);
match(COLON);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class Positional_placeholderContext extends ParserRuleContext {
public TerminalNode POSITIONAL_PLACEHOLDER() { return getToken(ParametersParser.POSITIONAL_PLACEHOLDER, 0); }
public Positional_placeholderContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_positional_placeholder; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterPositional_placeholder(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitPositional_placeholder(this);
}
}
public final Positional_placeholderContext positional_placeholder() throws RecognitionException {
Positional_placeholderContext _localctx = new Positional_placeholderContext(_ctx, getState());
enterRule(_localctx, 10, RULE_positional_placeholder);
try {
enterOuterAlt(_localctx, 1);
{
setState(40);
match(POSITIONAL_PLACEHOLDER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class DelimiterContext extends ParserRuleContext {
public TerminalNode DELIMITER() { return getToken(ParametersParser.DELIMITER, 0); }
public DelimiterContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_delimiter; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).enterDelimiter(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof ParametersParserListener ) ((ParametersParserListener)listener).exitDelimiter(this);
}
}
public final DelimiterContext delimiter() throws RecognitionException {
DelimiterContext _localctx = new DelimiterContext(_ctx, getState());
enterRule(_localctx, 12, RULE_delimiter);
try {
enterOuterAlt(_localctx, 1);
{
setState(42);
match(DELIMITER);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u0430\ud6d1\u8206\uad2d\u4417\uaef1\u8d80\uaadd\3\b/\4\2\t\2\4\3\t"+
"\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\3\2\6\2\22\n\2\r\2\16\2\23"+
"\3\3\3\3\5\3\30\n\3\6\3\32\n\3\r\3\16\3\33\3\3\5\3\37\n\3\3\4\3\4\3\5"+
"\3\5\5\5%\n\5\3\6\3\6\3\6\3\6\3\7\3\7\3\b\3\b\3\b\2\2\t\2\4\6\b\n\f\16"+
"\2\3\4\2\4\4\b\b,\2\21\3\2\2\2\4\31\3\2\2\2\6 \3\2\2\2\b$\3\2\2\2\n&\3"+
"\2\2\2\f*\3\2\2\2\16,\3\2\2\2\20\22\5\4\3\2\21\20\3\2\2\2\22\23\3\2\2"+
"\2\23\21\3\2\2\2\23\24\3\2\2\2\24\3\3\2\2\2\25\27\5\6\4\2\26\30\5\b\5"+
"\2\27\26\3\2\2\2\27\30\3\2\2\2\30\32\3\2\2\2\31\25\3\2\2\2\32\33\3\2\2"+
"\2\33\31\3\2\2\2\33\34\3\2\2\2\34\36\3\2\2\2\35\37\5\16\b\2\36\35\3\2"+
"\2\2\36\37\3\2\2\2\37\5\3\2\2\2 !\t\2\2\2!\7\3\2\2\2\"%\5\n\6\2#%\5\f"+
"\7\2$\"\3\2\2\2$#\3\2\2\2%\t\3\2\2\2&\'\7\5\2\2\'(\7\3\2\2()\7\5\2\2)"+
"\13\3\2\2\2*+\7\6\2\2+\r\3\2\2\2,-\7\7\2\2-\17\3\2\2\2\7\23\27\33\36$";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy