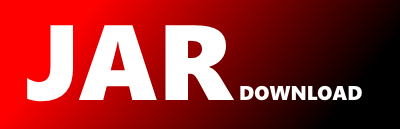
org.metafacture.flowcontrol.ObjectPipeDecoupler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of metafacture-flowcontrol Show documentation
Show all versions of metafacture-flowcontrol Show documentation
Modules for controlling the data flow in a Metafacture pipeline
/*
* Copyright 2013-2019 Deutsche Nationalbibliothek and others
*
* Licensed under the Apache License, Version 2.0 the "License";
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.metafacture.flowcontrol;
import org.metafacture.framework.FluxCommand;
import org.metafacture.framework.ObjectPipe;
import org.metafacture.framework.ObjectReceiver;
import org.metafacture.framework.annotations.Description;
import org.metafacture.framework.annotations.In;
import org.metafacture.framework.annotations.Out;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
/**
* Creates a new thread in which subsequent flow elements run.
*
* @param Object type
*
* @author Markus Micheal Geipel
* @author Pascal Christoph (dr0i)
*/
@In(Object.class)
@Out(Object.class)
@Description("creates a new thread in which subsequent flow elements run.")
@FluxCommand("decouple")
public final class ObjectPipeDecoupler implements ObjectPipe> {
public static final int DEFAULT_CAPACITY = 10000;
private static final Logger LOG = LoggerFactory.getLogger(ObjectPipeDecoupler.class);
private final BlockingQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy