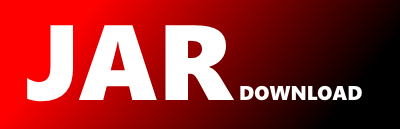
org.meteoinfo.data.TableData Maven / Gradle / Ivy
/* Copyright 2014 Yaqiang Wang,
* [email protected]
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; either version 2.1 of the License, or (at
* your option) any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser
* General Public License for more details.
*/
package org.meteoinfo.data;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.swing.JOptionPane;
import org.meteoinfo.common.MIMath;
import org.meteoinfo.common.util.GlobalUtil;
import org.meteoinfo.data.analysis.Statistics;
import org.meteoinfo.ndarray.DataType;
import org.meteoinfo.table.DataColumn;
import org.meteoinfo.table.DataRow;
import org.meteoinfo.table.DataTable;
/**
*
* @author Yaqiang Wang
*/
public class TableData extends DataTable {
//
//protected DataTable dataTable = new DataTable();
protected double missingValue = -9999.0;
//
//
/**
* Constructor
*/
public TableData() {
super();
}
/**
* Constructor
*
* @param dataTable The data table
*/
public TableData(DataTable dataTable) {
this.columns = dataTable.getColumns();
this.readOnly = dataTable.isReadOnly();
this.rows = dataTable.getRows();
this.tableName = dataTable.getTableName();
this.tag = dataTable.getTag();
}
//
//
// /**
// * Get data table
// *
// * @return Data table
// */
// public DataTable getDataTable() {
// return dataTable;
// }
//
// /**
// * Set data table
// *
// * @param value Data table
// */
// public void setDataTable(DataTable value) {
// dataTable = value;
// }
/**
* Get time column name
*
* @return Time column name
*/
public String getTimeColName() {
return null;
}
/**
* Get missing value
*
* @return Missing value
*/
public double getMissingValue() {
return missingValue;
}
/**
* Set missing value
*
* @param value Missing value
*/
public void setMissingValue(double value) {
missingValue = value;
}
//
//
//
/**
* Add column data
*
* @param colName Column name
* @param dt Data type string
* @param colData The column data
* @throws Exception
*/
@Override
public void addColumnData(String colName, String dt, List
//
/**
* Get years
*
* @param tColName Time column name
* @return Year list
*/
public List getYears(String tColName) {
List years = new ArrayList<>();
LocalDateTime ldt;
int year;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
year = ldt.getYear();
if (!years.contains(year)) {
years.add(year);
}
}
return years;
}
/**
* Get year months
*
* @param tColName Time column name
* @return Year month list
*/
public List getYearMonths(String tColName) {
List yms = new ArrayList<>();
DateTimeFormatter format =DateTimeFormatter.ofPattern("yyyyMM");
String ym;
for (DataRow row : this.getRows()) {
ym = format.format((LocalDateTime) row.getValue(tColName));
if (!yms.contains(ym)) {
yms.add(ym);
}
}
return yms;
}
/**
* Get data row list by year
*
* @param year The year
* @param tColName Time column name
* @return Data row list
*/
public List getDataByYear(int year, String tColName) {
List rows = new ArrayList<>();
LocalDateTime ldt;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
if (ldt.getYear() == year) {
rows.add(row);
}
}
return rows;
}
/**
* Get data row list by year
*
* @param season The season
* @param tColName Time column name
* @return Data row list
*/
public List getDataBySeason(String season, String tColName) {
List months = this.getMonthsBySeason(season);
List rows = new ArrayList<>();
LocalDateTime ldt;
int month;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
month = ldt.getMonthValue();
if (months.contains(month)) {
rows.add(row);
}
}
return rows;
}
private List getMonthsBySeason(String season) {
List months = new ArrayList<>();
if (season.equalsIgnoreCase("spring")) {
months.add(3);
months.add(4);
months.add(5);
} else if (season.equalsIgnoreCase("summer")) {
months.add(6);
months.add(7);
months.add(8);
} else if (season.equalsIgnoreCase("autumn")) {
months.add(9);
months.add(10);
months.add(11);
} else if (season.equalsIgnoreCase("winter")) {
months.add(12);
months.add(1);
months.add(2);
}
return months;
}
/**
* Get data row list by year and month
*
* @param yearMonth The year and month
* @param tColName Time column name
* @return Data row list
*/
public List getDataByYearMonth(String yearMonth, String tColName) {
int year = Integer.parseInt(yearMonth.substring(0, 4));
int month = Integer.parseInt(yearMonth.substring(4));
return this.getDataByYearMonth(year, month, tColName);
}
/**
* Get data row list by year and month
*
* @param year The year
* @param month The month
* @param tColName Time column name
* @return Data row list
*/
public List getDataByYearMonth(int year, int month, String tColName) {
List rows = new ArrayList<>();
LocalDateTime ldt;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
if (ldt.getYear() == year) {
if (ldt.getMonthValue() == month) {
rows.add(row);
}
}
}
return rows;
}
/**
* Get data row list by month
*
* @param month The month
* @param tColName Time column name
* @return Data row list
*/
public List getDataByMonth(int month, String tColName) {
List rows = new ArrayList<>();
LocalDateTime ldt;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
if (ldt.getMonthValue() == month) {
rows.add(row);
}
}
return rows;
}
/**
* Get data row list by day of week
*
* @param dow Day of week
* @param tColName Time column name
* @return Data row list
*/
public List getDataByDayOfWeek(int dow, String tColName) {
dow = dow + 1;
if (dow == 8) {
dow = 1;
}
List rows = new ArrayList<>();
LocalDateTime ldt;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
if (ldt.getDayOfWeek().getValue() == dow) {
rows.add(row);
}
}
return rows;
}
/**
* Get data row list by hour
*
* @param hour The hour
* @param tColName Time column name
* @return Result data row list
*/
public List getDataByHour(int hour, String tColName) {
List rows = new ArrayList<>();
LocalDateTime ldt;
for (DataRow row : this.getRows()) {
ldt = (LocalDateTime) row.getValue(tColName);
if (ldt.getHour() == hour) {
rows.add(row);
}
}
return rows;
}
/**
* Average year by year
*
* @param cols The data columns
* @param tColName The time column name
* @return Result data table
* @throws Exception
*/
public DataTable ave_Year(List cols, String tColName) throws Exception {
DataTable rTable = new DataTable();
rTable.addColumn("Year", DataType.INT);
for (DataColumn col : cols) {
rTable.addColumn(col.getColumnName(), DataType.DOUBLE);
}
List years = this.getYears(tColName);
for (int year : years) {
DataRow nRow = rTable.addRow();
nRow.setValue(0, year);
List rows = this.getDataByYear(year, tColName);
for (DataColumn col : cols) {
List values = this.getValidColumnValues(rows, col);
nRow.setValue(col.getColumnName(), Statistics.mean(values));
}
}
return rTable;
}
/**
* Average month by month
*
* @param cols The data columns
* @param tColName Time column name
* @return Result data table
* @throws Exception
*/
public DataTable ave_Month(List cols, String tColName) throws Exception {
DataTable rTable = new DataTable();
rTable.addColumn("YearMonth", DataType.STRING);
for (DataColumn col : cols) {
rTable.addColumn(col.getColumnName(), DataType.DOUBLE);
}
List yms = this.getYearMonths(tColName);
for (String ym : yms) {
DataRow nRow = rTable.addRow();
nRow.setValue(0, ym);
List rows = this.getDataByYearMonth(ym, tColName);
for (DataColumn col : cols) {
List values = this.getValidColumnValues(rows, col);
nRow.setValue(col.getColumnName(), Statistics.mean(values));
}
}
return rTable;
}
/**
* Average monthly
*
* @param cols The data columns
* @param tColName Time column name
* @return Result data table
* @throws Exception
*/
public DataTable ave_MonthOfYear(List cols, String tColName) throws Exception {
DataTable rTable = new DataTable();
rTable.addColumn("Month", DataType.STRING);
for (DataColumn col : cols) {
rTable.addColumn(col.getColumnName(), DataType.DOUBLE);
}
List monthNames = Arrays.asList(new String[]{"Jan", "Feb", "Mar", "Apr", "May",
"Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"});
List months = new ArrayList<>();
int i;
for (i = 1; i < 13; i++) {
months.add(i);
}
i = 0;
for (int month : months) {
DataRow nRow = rTable.addRow();
nRow.setValue(0, monthNames.get(i));
List rows = this.getDataByMonth(month, tColName);
for (DataColumn col : cols) {
List values = this.getValidColumnValues(rows, col);
nRow.setValue(col.getColumnName(), Statistics.mean(values));
}
i++;
}
return rTable;
}
/**
* Average seasonal
*
* @param cols The data columns
* @param tColName Time column name
* @return Result data table
* @throws Exception
*/
public DataTable ave_SeasonOfYear(List cols, String tColName) throws Exception {
DataTable rTable = new DataTable();
rTable.addColumn("Season", DataType.STRING);
for (DataColumn col : cols) {
rTable.addColumn(col.getColumnName(), DataType.DOUBLE);
}
List seasons = Arrays.asList(new String[]{"Spring", "Summer", "Autumn", "Winter"});
for (String season : seasons) {
DataRow nRow = rTable.addRow();
nRow.setValue(0, season);
List rows = this.getDataBySeason(season, tColName);
for (DataColumn col : cols) {
List values = this.getValidColumnValues(rows, col);
nRow.setValue(col.getColumnName(), Statistics.mean(values));
}
}
return rTable;
}
/**
* Average by day of week
*
* @param cols The data columns
* @param tColName Time column name
* @return Result data table
* @throws Exception
*/
public DataTable ave_DayOfWeek(List cols, String tColName) throws Exception {
DataTable rTable = new DataTable();
rTable.addColumn("Day", DataType.STRING);
for (DataColumn col : cols) {
rTable.addColumn(col.getColumnName(), DataType.DOUBLE);
}
List dowNames = Arrays.asList(new String[]{"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday",
"Saturday"});
List dows = new ArrayList<>();
dows.add(7);
int i;
for (i = 1; i < 7; i++) {
dows.add(i);
}
i = 0;
for (int dow : dows) {
DataRow nRow = rTable.addRow();
nRow.setValue(0, dowNames.get(i));
List rows = this.getDataByDayOfWeek(dow, tColName);
for (DataColumn col : cols) {
List values = this.getValidColumnValues(rows, col);
nRow.setValue(col.getColumnName(), Statistics.mean(values));
}
i++;
}
return rTable;
}
/**
* Average by hour of day
*
* @param cols The data columns
* @param tColName Time column name
* @return Result data table
* @throws Exception
*/
public DataTable ave_HourOfDay(List cols, String tColName) throws Exception {
DataTable rTable = new DataTable();
rTable.addColumn("Hour", DataType.INT);
for (DataColumn col : cols) {
rTable.addColumn(col.getColumnName(), DataType.DOUBLE);
}
List hours = new ArrayList<>();
for (int i = 0; i < 24; i++) {
hours.add(i);
}
for (int hour : hours) {
DataRow nRow = rTable.addRow();
nRow.setValue(0, hour);
List rows = this.getDataByHour(hour, tColName);
for (DataColumn col : cols) {
List values = this.getValidColumnValues(rows, col);
nRow.setValue(col.getColumnName(), Statistics.mean(values));
}
}
return rTable;
}
/**
* SQL select
* @param expression SQL expression
* @return Result TableData
*/
@Override
public TableData sqlSelect(String expression){
TableData r = new TableData((DataTable)super.sqlSelect(expression));
return r;
}
//
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy