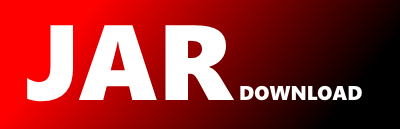
org.micromanager.acqj.util.AcqEventModules Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AcqEngJ Show documentation
Show all versions of AcqEngJ Show documentation
Java-based Acquisition engine for Micro-Manager
package org.micromanager.acqj.util;
import java.util.Iterator;
import java.util.List;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import org.micromanager.acqj.main.AcqEngMetadata;
import org.micromanager.acqj.main.AcquisitionEvent;
import org.micromanager.acqj.util.xytiling.XYStagePosition;
import org.micromanager.acqj.internal.Engine;
/**
* A utility class with multiple "modules" functions for creating common
* acquisition functions that can be combined to encode complex behaviors
*
* @author henrypinkard
*/
public class AcqEventModules {
public static Function> zStack(int startSliceIndex, int stopSliceIndex, double zStep, double zOrigin) {
return (AcquisitionEvent event) -> {
return new Iterator() {
private int zIndex_ = startSliceIndex;
@Override
public boolean hasNext() {
return zIndex_ < stopSliceIndex;
}
@Override
public AcquisitionEvent next() {
double zPos = zIndex_ * zStep + zOrigin;
AcquisitionEvent sliceEvent = event.copy();
//Do plus equals here in case z positions have been modified by another function (e.g. channel specific focal offsets)
sliceEvent.setZ(zIndex_,
(sliceEvent.getZPosition() == null ? 0.0 : sliceEvent.getZPosition()) + zPos);
zIndex_++;
return sliceEvent;
}
};
};
}
public static Function> timelapse(int numTimePoints, double interval_ms) {
return (AcquisitionEvent event) -> {
return new Iterator() {
int frameIndex_ = 0;
@Override
public boolean hasNext() {
if (frameIndex_ == 0) {
return true;
}
if (frameIndex_ < numTimePoints) {
return true;
}
return false;
}
@Override
public AcquisitionEvent next() {
AcquisitionEvent timePointEvent = event.copy();
timePointEvent.setMinimumStartTime((long) (interval_ms * frameIndex_));
timePointEvent.setTimeIndex(frameIndex_);
frameIndex_++;
return timePointEvent;
}
};
};
}
/**
* Make an iterator for events for each active channel
*
* @param channelList
* @return
*/
public static Function> channels(List channelList) {
return (AcquisitionEvent event) -> {
return new Iterator() {
int index = 0;
@Override
public boolean hasNext() {
return index < channelList.size();
}
@Override
public AcquisitionEvent next() {
AcquisitionEvent channelEvent = event.copy();
channelEvent.setConfigGroup(channelList.get(index).group_);
channelEvent.setConfigPreset(channelList.get(index).config_);
channelEvent.setChannelName(channelList.get(index).config_);
boolean hasZOffsets = channelList.stream().map(t -> t.offset_).
filter(t -> t != 0).collect(Collectors.toList()).size() > 0;
Double zPos;
if (channelEvent.getZPosition() == null) {
if (hasZOffsets) {
try {
zPos = Engine.getCore().getPosition() + channelList.get(index).offset_;
} catch (Exception e) {
throw new RuntimeException(e);
}
} else {
zPos = null;
}
} else {
zPos = channelEvent.getZPosition() + channelList.get(index).offset_;
}
channelEvent.setZ(channelEvent.getZIndex(), zPos);
// try {
// channelEvent.setZ(channelEvent.getZIndex(),
// channelEvent.getZPosition() == null ?
// hasZOffsets ?
// Engine.getCore().getPosition() + channelList.get(index).offset_ : null
// : channelEvent.getZPosition() + channelList.get(index).offset_
// );
// } catch (Exception e) {
// throw new RuntimeException(e);
// }
channelEvent.setExposure(channelList.get(index).exposure_);
index++;
return channelEvent;
}
};
};
}
/**
* Iterate over an arbitrary list of positions. Adds in postition indices to
* the axes that assumer the order in the list provided correspond to the
* desired indices
*
* @param positions
* @return
*/
public static Function> positions(List positions) {
return (AcquisitionEvent event) -> {
Stream.Builder builder = Stream.builder();
if (positions == null) {
builder.accept(event);
} else {
for (int index = 0; index < positions.size(); index++) {
AcquisitionEvent posEvent = event.copy();
posEvent.setX(positions.get(index).getCenter().x);
posEvent.setY(positions.get(index).getCenter().y);
posEvent.setGridRow(positions.get(index).getGridRow());
posEvent.setGridCol(positions.get(index).getGridCol());
posEvent.setAxisPosition(AcqEngMetadata.AXES_GRID_ROW, positions.get(index).getGridRow());
posEvent.setAxisPosition(AcqEngMetadata.AXES_GRID_COL, positions.get(index).getGridCol());
// posEvent.setAxisPosition(AcqEngMetadata.POSITION_AXIS, index);
builder.accept(posEvent);
}
}
return builder.build().iterator();
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy