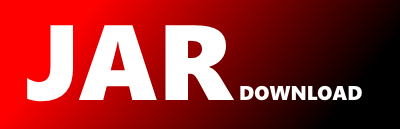
org.microbean.helm.chart.AbstractChartLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of microbean-helm Show documentation
Show all versions of microbean-helm Show documentation
Java bindings for the Helm API
The newest version!
/* -*- mode: Java; c-basic-offset: 2; indent-tabs-mode: nil; coding: utf-8-unix -*-
*
* Copyright © 2017 MicroBean.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package org.microbean.helm.chart;
import java.io.Closeable;
import java.io.IOException;
import hapi.chart.ChartOuterClass.Chart; // for javadoc only
import hapi.chart.ChartOuterClass.Chart.Builder;
/**
* An abstract class whose implementations are capable of reading in
* the raw materials for a {@linkplain Chart Helm chart} from some
* kind of source and creating new {@link Chart} instances
* from such raw materials.
*
* Implementations should pay close attention to any
* potential resource leaks and control them in their implementation
* of the {@link Closeable#close()} method.
*
* @param the type of source from which {@link Chart}s may be
* loaded
*
* @author Laird Nelson
*
* @see #load(Object)
*
* @see #load(hapi.chart.ChartOuterClass.Chart.Builder, Object)
*
* @see Chart
*/
public abstract class AbstractChartLoader implements Closeable {
/*
* Constructors.
*/
/**
* Creates a new {@link AbstractChartLoader}.
*/
protected AbstractChartLoader() {
super();
}
/*
* Instance methods.
*/
/**
* Creates a new "top-level" {@link Builder} from the supplied
* {@code source} and returns it.
*
* Implementations of this method must not return {@code null}.
*
* @param source the source from which a new {@link Builder} should be
* created; must not be {@code null}
*
* @return a new {@link Builder}; never {@code null}
*
* @exception NullPointerException if {@code source} is {@code null}
*
* @exception IOException if reading the supplied {@code source}
* could not complete normally
*
* @see #load(ChartOuterClass.Chart.Builder, Object)
*/
public final Builder load(final T source) throws IOException {
return this.load(null, source);
}
/**
* Creates a new {@link Builder} from the supplied {@code source}
* and returns it.
*
* Implementations of this method must not return {@code null}
* and must not return {@code parent}.
*
* @param parent the {@link Builder} that will serve as the parent
* of the {@link Builder} that will be returned; may be (and often
* is) {@code null}, indicating that the {@link Builder} being
* returned does not represent a subchart; must not be what is
* returned
*
* @param source the source from which a new {@link Builder} should
* be created; must not be {@code null}
*
* @return a new {@link Builder}; never {@code null}; never {@code
* parent}
*
* @exception NullPointerException if {@code source} is {@code null}
*
* @exception IOException if reading the supplied {@code source}
* could not complete normally
*/
public abstract Builder load(final Builder parent, final T source) throws IOException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy