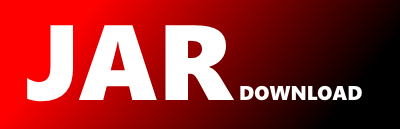
javax.bluetooth.DiscoveryAgent Maven / Gradle / Ivy
Show all versions of microemu-jsr-82 Show documentation
/**
* Java docs licensed under the Apache License, Version 2.0
* http://www.apache.org/licenses/LICENSE-2.0
* (c) Copyright 2001, 2002 Motorola, Inc. ALL RIGHTS RESERVED.
*
*
* @version $Id: DiscoveryAgent.java 1379 2007-10-13 02:00:43Z vlads $
*/
package javax.bluetooth;
/**
* The DiscoveryAgent
class provides methods to perform
* device and service discovery. A local device must have only one
* DiscoveryAgent
object. This object must be retrieved
* by a call to getDiscoveryAgent()
on the
* LocalDevice
object.
*
* Device Discovery
*
* There are two ways to discover devices. First, an application may
* use startInquiry()
to start an inquiry to find devices
* in proximity to the local device. Discovered devices are returned
* via the deviceDiscovered()
method of the interface
* DiscoveryListener
. The second way to
* discover devices is via the retrieveDevices()
method.
* This method will return devices that have been discovered via a
* previous inquiry or devices that are classified as pre-known.
* (Pre-known devices are those devices that are defined in the
* Bluetooth Control Center as devices this device frequently contacts.)
* The retrieveDevices()
method does not perform an
* inquiry, but provides a quick way to get a list of devices that may
* be in the area.
*
* Service Discovery
* The DiscoveryAgent
class also encapsulates the
* functionality provided by the service discovery application profile.
* The class provides an interface for an application to search and
* retrieve attributes for a particular service. There are two ways to
* search for services. To search for a service on a single device,
* the searchServices()
method should be used. On the
* other hand, if you don't care which device a service is on, the
* selectService()
method does a service search on a
* set of remote devices.
*
*
* @version 1.0 February 11, 2002
*
*/
public class DiscoveryAgent {
/**
* Takes the device out of discoverable mode.
*
* The value of NOT_DISCOVERABLE
is 0x00 (0).
*/
public static final int NOT_DISCOVERABLE = 0;
/**
* The inquiry access code for General/Unlimited Inquiry Access Code
* (GIAC). This is used to specify the type of inquiry to complete or
* respond to.
*
* The value of GIAC
is 0x9E8B33 (10390323). This value
* is defined in the Bluetooth Assigned Numbers document.
*/
public static final int GIAC = 0x9E8B33;
/**
* The inquiry access code for Limited Dedicated Inquiry Access Code
* (LIAC). This is used to specify the type of inquiry to complete or
* respond to.
*
* The value of LIAC
is 0x9E8B00 (10390272). This value
* is defined in the Bluetooth Assigned Numbers document.
*/
public static final int LIAC = 0x9E8B00;
/**
* Used with the retrieveDevices()
method to return
* those devices that were found via a previous inquiry. If no
* inquiries have been started, this will cause the method to return
* null
.
*
* The value of CACHED
is 0x00 (0).
*
* @see #retrieveDevices
*/
public static final int CACHED = 0x00;
/**
* Used with the retrieveDevices()
method to return
* those devices that are defined to be pre-known devices. Pre-known
* devices are specified in the BCC. These are devices that are
* specified by the user as devices with which the local device will
* frequently communicate.
*
* The value of PREKNOWN
is 0x01 (1).
*
* @see #retrieveDevices
*/
public static final int PREKNOWN = 0x01;
/**
* Creates a DiscoveryAgent
object.
*/
private DiscoveryAgent() {
}
/**
* Returns an array of Bluetooth devices that have either been found
* by the local device during previous inquiry requests or been
* specified as a pre-known device depending on the argument. The list
* of previously found devices is maintained by the implementation of
* this API. (In other words, maintenance of the list of previously
* found devices is an implementation detail.) A device can be set as
* a pre-known device in the Bluetooth Control Center.
*
* @param option CACHED
if previously found devices
* should be returned; PREKNOWN
if pre-known devices
* should be returned
*
* @return an array containing the Bluetooth devices that were
* previously found if option
is CACHED
;
* an array of devices that are pre-known devices if
* option
is PREKNOWN
; null
* if no devices meet the criteria
*
* @exception IllegalArgumentException if option
is
* not CACHED
or PREKNOWN
*/
public RemoteDevice[] retrieveDevices(int option) {
return null;
}
/**
* Places the device into inquiry mode. The length of the inquiry is
* implementation dependent. This method will search for devices with the
* specified inquiry access code. Devices that responded to the inquiry
* are returned to the application via the method
* deviceDiscovered()
of the interface
* DiscoveryListener
. The cancelInquiry()
* method is called to stop the inquiry.
*
* @see #cancelInquiry
* @see #GIAC
* @see #LIAC
*
* @param accessCode the type of inquiry to complete
*
* @param listener the event listener that will receive device
* discovery events
*
* @return true
if the inquiry was started;
* false
if the inquiry was not started because the
* accessCode
is not supported
*
* @exception IllegalArgumentException if the access code provided
* is not LIAC
, GIAC
, or in the range
* 0x9E8B00 to 0x9E8B3F
*
* @exception NullPointerException if listener
is
* null
*
* @exception BluetoothStateException if the Bluetooth device does
* not allow an inquiry to be started due to other operations that are being
* performed by the device
*/
public boolean startInquiry(int accessCode, DiscoveryListener listener) throws BluetoothStateException {
if (listener == null) {
throw new NullPointerException("DiscoveryListener is null");
}
if ((accessCode != LIAC) && (accessCode != GIAC) && ((accessCode < 0x9E8B00) || (accessCode > 0x9E8B3F))) {
throw new IllegalArgumentException("Invalid accessCode " + accessCode);
}
return false;
}
/**
* Removes the device from inquiry mode.
*
* An inquiryCompleted()
event will occur with a type of
* INQUIRY_TERMINATED
as a result of calling this
* method. After receiving this
* event, no further deviceDiscovered()
events will occur
* as a result of this inquiry.
*
*
*
* This method will only cancel the inquiry if the
* listener
provided is the listener that started
* the inquiry.
*
* @param listener the listener that is receiving inquiry events
*
* @return true
if the inquiry was canceled; otherwise
* false
if the inquiry was not canceled or if the inquiry
* was not started using listener
*
* @exception NullPointerException if listener
is
* null
*/
public boolean cancelInquiry(DiscoveryListener listener) {
if (listener == null) {
throw new NullPointerException("DiscoveryListener is null");
}
return false;
}
/**
* Searches for services on a remote Bluetooth device that have all the
* UUIDs specified in uuidSet
. Once the service is found,
* the attributes specified in attrSet
and the default
* attributes are retrieved. The default attributes are
* ServiceRecordHandle (0x0000), ServiceClassIDList
* (0x0001), ServiceRecordState (0x0002), ServiceID (0x0003), and
* ProtocolDescriptorList (0x0004).If attrSet
is
* null
then only the default attributes will be retrieved.
* attrSet
does not have to be sorted in increasing order,
* but must only contain values in the range [0 - (216-1)].
*
* @see DiscoveryListener
*
* @param attrSet indicates the attributes whose values will be
* retrieved on services which have the UUIDs specified in
* uuidSet
*
* @param uuidSet the set of UUIDs that are being searched for; all
* services returned will contain all the UUIDs specified here
*
* @param btDev the remote Bluetooth device to search for services on
*
* @param discListener the object that will receive events when
* services are discovered
*
* @return the transaction ID of the service search; this number
* must be positive
*
* @exception BluetoothStateException if the number of concurrent
* service search transactions exceeds the limit specified by the
* bluetooth.sd.trans.max
property obtained from the
* class LocalDevice
or the system is unable to start
* one due to current conditions
*
* @exception IllegalArgumentException if attrSet
has
* an illegal service attribute ID or exceeds the property
* bluetooth.sd.attr.retrievable.max
* defined in the class LocalDevice
; if
* attrSet
* or uuidSet
is of length 0; if attrSet
* or uuidSet
contains duplicates
*
* @exception NullPointerException if uuidSet
,
* btDev
, or discListener
is
* null
; if an element in uuidSet
array is
* null
*
*/
public int searchServices(int[] attrSet, UUID[] uuidSet, RemoteDevice btDev, DiscoveryListener discListener)
throws BluetoothStateException {
if (uuidSet == null) {
throw new NullPointerException("uuidSet is null");
}
if (uuidSet.length == 0) {
// The same as on Motorola, Nokia and SE Phones
throw new IllegalArgumentException("uuidSet is empty");
}
for (int u1 = 0; u1 < uuidSet.length; u1++) {
for (int u2 = u1 + 1; u2 < uuidSet.length; u2++) {
if (uuidSet[u1].equals(uuidSet[u2])) {
throw new IllegalArgumentException("uuidSet has duplicate values " + uuidSet[u1].toString());
}
}
}
if (btDev == null) {
throw new NullPointerException("RemoteDevice is null");
}
if (discListener == null) {
throw new NullPointerException("DiscoveryListener is null");
}
for (int i = 0; attrSet != null && i < attrSet.length; i++) {
if (attrSet[i] < 0x0000 || attrSet[i] > 0xffff) {
throw new IllegalArgumentException("attrSet[" + i + "] not in range");
}
}
return 0;
}
/**
* Cancels the service search transaction that has the specified
* transaction ID. The ID was assigned to the transaction by the
* method searchServices()
. A
* serviceSearchCompleted()
event with a discovery type
* of SERVICE_SEARCH_TERMINATED
will occur when
* this method is called. After receiving this event, no further
* servicesDiscovered()
events will occur as a result
* of this search.
*
* @param transID the ID of the service search transaction to
* cancel; returned by searchServices()
*
* @return true
if the service search transaction is
* terminated, else false
if the transID
* does not represent an active service search transaction
*/
public boolean cancelServiceSearch(int transID) {
return false;
}
/**
* Attempts to locate a service that contains uuid
in
* the ServiceClassIDList of its service record. This
* method will return a string that may be used in
* Connector.open()
to establish a connection to the
* service. How the service is selected if there are multiple services
* with uuid
and which devices to
* search is implementation dependent.
*
* @see ServiceRecord#NOAUTHENTICATE_NOENCRYPT
* @see ServiceRecord#AUTHENTICATE_NOENCRYPT
* @see ServiceRecord#AUTHENTICATE_ENCRYPT
*
* @param uuid the UUID to search for in the ServiceClassIDList
*
* @param security specifies the security requirements for a connection
* to this service; must be one of
* ServiceRecord.NOAUTHENTICATE_NOENCRYPT
,
* ServiceRecord.AUTHENTICATE_NOENCRYPT
, or
* ServiceRecord.AUTHENTICATE_ENCRYPT
*
* @param master determines if this client must be the master of the
* connection; true
if the client must be the master;
* false
if the client can be the master or the slave
*
* @return the connection string used to connect to the service
* with a UUID of uuid
; or null
if no
* service could be found with a UUID of uuid
in the
* ServiceClassIDList
*
* @exception BluetoothStateException if the Bluetooth system cannot
* start the request due to the current state of the Bluetooth system
*
* @exception NullPointerException if uuid
is
* null
*
* @exception IllegalArgumentException if security
is
* not ServiceRecord.NOAUTHENTICATE_NOENCRYPT
,
* ServiceRecord.AUTHENTICATE_NOENCRYPT
, or
* ServiceRecord.AUTHENTICATE_ENCRYPT
*/
public String selectService(UUID uuid, int security, boolean master) throws BluetoothStateException {
return null;
}
}