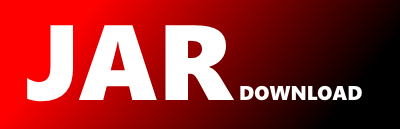
javax.obex.Authenticator Maven / Gradle / Ivy
Show all versions of microemu-jsr-82 Show documentation
/**
* Java docs licensed under the Apache License, Version 2.0
* http://www.apache.org/licenses/LICENSE-2.0
* (c) Copyright 2001, 2002 Motorola, Inc. ALL RIGHTS RESERVED.
*
*
* @version $Id: Authenticator.java 1379 2007-10-13 02:00:43Z vlads $
*/
package javax.obex;
/**
* This interface provides a way to respond to authentication challenge and
* authentication response headers. When a client or server receives an
* authentication challenge or authentication response header, the
* onAuthenticationChallenge()
or
* onAuthenticationResponse()
will be called, respectively, by
* the implementation.
*
* For more information on how the authentication procedure works in OBEX,
* please review the IrOBEX specification at http://www.irda.org.
*
* Authentication Challenges
*
* When a client or server receives an authentication challenge header, the
* onAuthenticationChallenge()
method will be invoked by the OBEX
* API implementation. The application will then return the user name (if
* needed) and password via a PasswordAuthentication
object. The
* password in this object is not sent in the authentication response. Instead,
* the 16-byte challenge received in the authentication challenge is combined
* with the password returned from the onAuthenticationChallenge()
* method and passed through the MD5 hash algorithm. The resulting value is sent
* in the authentication response along with the user name if it was provided.
*
* Authentication Responses
*
* When a client or server receives an authentication response header, the
* onAuthenticationResponse()
method is invoked by the API
* implementation with the user name received in the authentication response
* header. (The user name will be null
if no user name was
* provided in the authentication response header.) The application must
* determine the correct password. This value should be returned from the
* onAuthenticationResponse()
method. If the authentication
* request should fail without the implementation checking the password,
* null
should be returned by the application. (This is needed
* for reasons like not recognizing the user name, etc.) If the returned value
* is not null
, the OBEX API implementation will combine the
* password returned from the onAuthenticationResponse()
method
* and challenge sent via the authentication challenge, apply the MD5 hash
* algorithm, and compare the result to the response hash received in the
* authentication response header. If the values are not equal, an
* IOException
will be thrown if the client requested
* authentication. If the server requested authentication, the
* onAuthenticationFailure()
method will be called on the
* ServerRequestHandler
that failed authentication. The
* connection is not closed if authentication failed.
*
* @version 1.0 February 11, 2002
*/
public interface Authenticator {
/**
* Called when a client or a server receives an authentication challenge
* header. It should respond to the challenge with a
* PasswordAuthentication
that contains the correct user name
* and password for the challenge.
*
* @param description
* the description of which user name and password should be
* used; if no description is provided in the authentication
* challenge or the description is encoded in an encoding scheme
* that is not supported, an empty string will be provided
*
* @param isUserIdRequired
* true
if the user ID is required;
* false
if the user ID is not required
*
* @param isFullAccess
* true
if full access to the server will be
* granted; false
if read only access will be
* granted
*
* @return a PasswordAuthentication
object containing the
* user name and password used for authentication
*/
public PasswordAuthentication onAuthenticationChallenge(String description, boolean isUserIdRequired,
boolean isFullAccess);
/**
* Called when a client or server receives an authentication response
* header. This method will provide the user name and expect the correct
* password to be returned.
*
* @param userName
* the user name provided in the authentication response; may be
* null
*
* @return the correct password for the user name provided; if
* null
is returned then the authentication request
* failed
*/
public byte[] onAuthenticationResponse(byte[] userName);
}