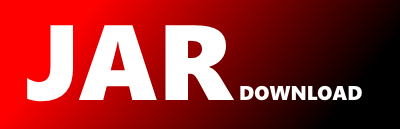
org.microprofileext.config.event.ChangeEventNotifier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of config-events Show documentation
Show all versions of config-events Show documentation
A library to make it easy to add config events
The newest version!
package org.microprofileext.config.event;
import java.lang.annotation.Annotation;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import jakarta.enterprise.context.ApplicationScoped;
import jakarta.enterprise.context.Initialized;
import jakarta.enterprise.event.Event;
import jakarta.enterprise.event.Observes;
import jakarta.inject.Inject;
/**
* Easy way to fire a change event
* @author Phillip Kruger
*
* This gets used from Config sources that is not in the CDI Context. So we can not @Inject a bean.
* For some reason, CDI.current() is only working on Payara, and not on Thorntail and OpenLiberty, so this ugly footwork is to
* get around that.
*/
@ApplicationScoped
public class ChangeEventNotifier {
@Inject
private Event broadcaster;
private static ChangeEventNotifier INSTANCE;
public void init(@Observes @Initialized(ApplicationScoped.class) Object init) {
INSTANCE = this;
}
public static ChangeEventNotifier getInstance(){
//return CDI.current().select(ChangeEventNotifier.class).get();
return INSTANCE;
}
public void detectChangesAndFire(Map before,Map after, String fromSource){
List changes = new ArrayList<>();
if(!before.equals(after)){
Set> beforeEntries = before.entrySet();
for(Map.Entry beforeEntry:beforeEntries){
String key = beforeEntry.getKey();
String oldValue = beforeEntry.getValue();
if(after.containsKey(key)){
String newValue = after.get(key);
if(!newValue.equals(oldValue)){
// Update
changes.add(new ChangeEvent(Type.UPDATE, key, getOptionalOldValue(oldValue), newValue, fromSource));
}
after.remove(key);
}else{
// Removed.
changes.add(new ChangeEvent(Type.REMOVE, key, getOptionalOldValue(oldValue), null, fromSource));
}
}
Set> newEntries = after.entrySet();
for(Map.Entry newEntry:newEntries){
// New
changes.add(new ChangeEvent(Type.NEW, newEntry.getKey(), Optional.empty(), newEntry.getValue(), fromSource));
}
}
if(!changes.isEmpty())fire(changes);
}
public void fire(ChangeEvent changeEvent){
List annotationList = new ArrayList<>();
annotationList.add(new TypeFilter.TypeFilterLiteral(changeEvent.getType()));
annotationList.add(new KeyFilter.KeyFilterLiteral(changeEvent.getKey()));
annotationList.add(new SourceFilter.SourceFilterLiteral(changeEvent.getFromSource()));
broadcaster.select(annotationList.toArray(new Annotation[annotationList.size()])).fire(changeEvent);
}
public void fire(List changeEvents){
for(ChangeEvent changeEvent : changeEvents){
fire(changeEvent);
}
}
public Optional getOptionalOldValue(String oldValue){
if(oldValue==null || oldValue.isEmpty())return Optional.empty();
return Optional.of(oldValue);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy