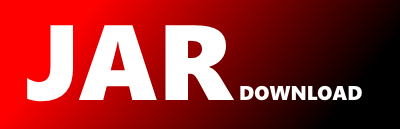
org.microprofileext.config.source.yaml.YamlConfigSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configsource-yaml Show documentation
Show all versions of configsource-yaml Show documentation
A config that get the values from a yaml file
The newest version!
package org.microprofileext.config.source.yaml;
import java.io.InputStream;
import java.util.*;
import org.microprofileext.config.source.base.file.AbstractUrlBasedSource;
import org.yaml.snakeyaml.Yaml;
/**
* Yaml config source
* @author Phillip Kruger
*/
public class YamlConfigSource extends AbstractUrlBasedSource {
@Override
protected String getFileExtension() {
return "yaml";
}
@Override
@SuppressWarnings("unchecked")
protected Map toMap(InputStream inputStream) {
final Map properties = new TreeMap<>();
Yaml yaml = new Yaml();
Map yamlInput = yaml.loadAs(inputStream, TreeMap.class);
if(Objects.nonNull(yamlInput)) {
for (String key : yamlInput.keySet()) {
populateMap(properties,key, yamlInput.get(key));
}
}
return properties;
}
@SuppressWarnings("unchecked")
private void populateMap(Map properties, String key, Object o) {
if (o instanceof Map) {
Map map = (Map)o;
for (Object mapKey : map.keySet()) {
populateEntry(properties, key,mapKey.toString(),map);
}
} else if (o instanceof List) {
List l = toStringList((List)o);
properties.put(key,String.join(COMMA, l));
} else{
if(o!=null)properties.put(key,o.toString());
}
}
@SuppressWarnings("unchecked")
private void populateEntry(Map properties, String key, String mapKey, Map map){
String format = "%s" + super.getKeySeparator() + "%s";
if (map.get(mapKey) instanceof Map) {
populateMap(properties, String.format(format, key, mapKey), (Map) map.get(mapKey));
} else if (map.get(mapKey) instanceof List) {
List l = toStringList((List)map.get(mapKey));
properties.put(String.format(format, key, mapKey),String.join(COMMA, l));
} else {
properties.put(String.format(format, key, mapKey), map.get(mapKey).toString());
}
}
private List toStringList(List l){
List nl = new ArrayList<>();
for(Object o:l){
String s = String.valueOf(o);
if(s.contains(COMMA))s = s.replaceAll(COMMA, "\\\\,"); // Escape comma
nl.add(s);
}
return nl;
}
private static final String COMMA = ",";
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy