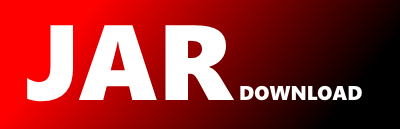
org.minimalj.backend.sql.Table Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of minimalj Show documentation
Show all versions of minimalj Show documentation
A java framework aiming for a minimal programming style. Includes GUI and persistence layer.
package org.minimalj.backend.sql;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import org.minimalj.model.Code;
import org.minimalj.model.Keys;
import org.minimalj.model.annotation.Searched;
import org.minimalj.model.properties.FlatProperties;
import org.minimalj.model.properties.PropertyInterface;
import org.minimalj.transaction.criteria.Criteria;
import org.minimalj.transaction.criteria.Criteria.AndCriteria;
import org.minimalj.transaction.criteria.Criteria.OrCriteria;
import org.minimalj.transaction.criteria.FieldCriteria;
import org.minimalj.transaction.criteria.SearchCriteria;
import org.minimalj.util.GenericUtils;
import org.minimalj.util.IdUtils;
import org.minimalj.util.LoggingRuntimeException;
@SuppressWarnings("rawtypes")
public class Table extends AbstractTable {
protected final PropertyInterface idProperty;
protected final String selectAllQuery;
protected final HashMap lists;
public Table(SqlPersistence sqlPersistence, Class clazz) {
this(sqlPersistence, null, clazz);
}
public Table(SqlPersistence sqlPersistence, String name, Class clazz) {
super(sqlPersistence, name, clazz);
this.idProperty = FlatProperties.getProperty(clazz, "id", true);
Objects.nonNull(idProperty);
List lists = FlatProperties.getListProperties(clazz);
this.lists = createListTables(lists);
this.selectAllQuery = selectAllQuery();
}
@Override
public void createTable(SqlSyntax syntax) {
super.createTable(syntax);
for (Object object : lists.values()) {
AbstractTable subTable = (AbstractTable) object;
subTable.createTable(syntax);
}
}
@Override
public void createIndexes(SqlSyntax syntax) {
super.createIndexes(syntax);
for (Object object : lists.values()) {
AbstractTable subTable = (AbstractTable) object;
subTable.createIndexes(syntax);
}
}
@Override
public void createConstraints(SqlSyntax syntax) {
super.createConstraints(syntax);
for (Object object : lists.values()) {
AbstractTable subTable = (AbstractTable) object;
subTable.createConstraints(syntax);
}
}
public Object insert(T object) {
try (PreparedStatement insertStatement = createStatement(sqlPersistence.getConnection(), insertQuery, true)) {
Object id;
if (IdUtils.hasId(object.getClass())) {
id = IdUtils.getId(object);
if (id == null) {
id = IdUtils.createId();
IdUtils.setId(object, id);
}
} else {
id = IdUtils.createId();
}
setParameters(insertStatement, object, false, ParameterMode.INSERT, id);
insertStatement.execute();
insertLists(object);
if (object instanceof Code) {
sqlPersistence.invalidateCodeCache(object.getClass());
}
return id;
} catch (SQLException x) {
throw new LoggingRuntimeException(x, sqlLogger, "Couldn't insert in " + getTableName() + " with " + object);
}
}
protected void insertLists(T object) {
for (Entry listEntry : lists.entrySet()) {
List list = (List) listEntry.getKey().getValue(object);
if (list != null && !list.isEmpty()) {
listEntry.getValue().addList(object, list);
}
}
}
public void delete(Object id) {
try (PreparedStatement updateStatement = createStatement(sqlPersistence.getConnection(), deleteQuery, false)) {
updateStatement.setObject(1, id);
updateStatement.execute();
} catch (SQLException x) {
throw new LoggingRuntimeException(x, sqlLogger, "Couldn't delete " + getTableName() + " with ID " + id);
}
}
private LinkedHashMap createListTables(List listProperties) {
LinkedHashMap lists = new LinkedHashMap<>();
for (PropertyInterface listProperty : listProperties) {
ListTable listTable = createListTable(listProperty);
lists.put(listProperty, listTable);
}
return lists;
}
ListTable createListTable(PropertyInterface property) {
Class> elementClass = GenericUtils.getGenericClass(property.getType());
String subTableName = buildSubTableName(property);
if (IdUtils.hasId(elementClass)) {
return new CrossTable<>(sqlPersistence, subTableName, elementClass, idProperty);
} else {
return new SubTable(sqlPersistence, subTableName, elementClass, idProperty);
}
}
protected String buildSubTableName(PropertyInterface property) {
return getTableName() + "__" + property.getName();
}
public void update(T object) {
updateWithId(object, IdUtils.getId(object));
}
void updateWithId(T object, Object id) {
try (PreparedStatement updateStatement = createStatement(sqlPersistence.getConnection(), updateQuery, false)) {
setParameters(updateStatement, object, false, ParameterMode.UPDATE, id);
updateStatement.execute();
for (Entry listTableEntry : lists.entrySet()) {
List list = (List) listTableEntry.getKey().getValue(object);
listTableEntry.getValue().replaceList(object, list);
}
if (object instanceof Code) {
sqlPersistence.invalidateCodeCache(object.getClass());
}
} catch (SQLException x) {
throw new LoggingRuntimeException(x, sqlLogger, "Couldn't update in " + getTableName() + " with " + object);
}
}
public T read(Object id) {
return read(id, true);
}
public T read(Object id, boolean complete) {
try (PreparedStatement selectByIdStatement = createStatement(sqlPersistence.getConnection(), selectByIdQuery, false)) {
selectByIdStatement.setObject(1, id);
T object = executeSelect(selectByIdStatement);
if (object != null) {
if (complete) {
loadLists(object);
} else {
IdUtils.setId(object, new ReadOnlyId(id));
}
}
return object;
} catch (SQLException x) {
throw new LoggingRuntimeException(x, sqlLogger, "Couldn't read " + getTableName() + " with ID " + id);
}
}
private List getColumns(Object[] keys) {
List result = new ArrayList<>();
PropertyInterface[] properties = Keys.getProperties(keys);
for (Map.Entry entry : columns.entrySet()) {
PropertyInterface property = entry.getValue();
for (PropertyInterface p : properties) {
if (p.getPath().equals(property.getPath())) {
result.add(entry.getKey());
}
}
}
return result;
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy