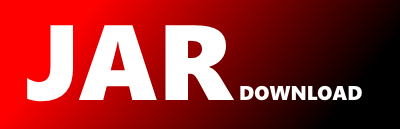
org.minimalj.frontend.Frontend Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of minimalj Show documentation
Show all versions of minimalj Show documentation
A java framework aiming for a minimal programming style. Includes GUI and persistence layer.
package org.minimalj.frontend;
import java.util.Collections;
import java.util.List;
import java.util.function.Function;
import org.minimalj.frontend.action.Action;
import org.minimalj.frontend.page.IDialog;
import org.minimalj.frontend.page.Page;
import org.minimalj.frontend.page.PageManager;
import org.minimalj.model.Rendering;
import org.minimalj.security.Subject;
/**
* To provide a new kind (Xy) of client you have to implement two things:
*
* - This class, like XyFrontend
* - Some kind of XyApplication with a main. The XyApplication should take an instance of Application and
* start the client. Take a look at the existing SwingFrontend, JsonFrontend or LanternaFrontend. The trickiest part will be to implement
* the PageManager.
*
*
*/
public abstract class Frontend {
private static Frontend instance;
public static Frontend getInstance() {
if (instance == null) {
throw new IllegalStateException("Frontend has to be initialized");
}
return instance;
}
public static synchronized void setInstance(Frontend frontend) {
if (Frontend.instance != null) {
throw new IllegalStateException("Frontend cannot be changed");
}
if (frontend == null) {
throw new IllegalArgumentException("Frontend cannot be null");
}
Frontend.instance = frontend;
}
public static boolean isAvailable() {
return Frontend.instance != null;
}
/**
* Components are the smallest part of the gui. Things like textfields
* and comboboxes. A form is filled with components.
*/
public interface IComponent {
}
public interface Input extends IComponent {
public void setValue(T value);
public T getValue();
public void setEditable(boolean editable);
}
public interface PasswordField extends Input {
}
// http://www.w3schools.com/html/html_form_input_types.asp
public enum InputType { FREE, EMAIL, URL, TEL, NUMBER; }
public abstract IComponent createText(String string);
public abstract IComponent createText(Action action);
public abstract IComponent createText(Rendering rendering);
public abstract IComponent createTitle(String string);
public abstract Input createReadOnlyTextField();
public abstract Input createTextField(int maxLength, String allowedCharacters, InputType inputType, Search suggestionSearch, InputComponentListener changeListener);
public abstract Input createAreaField(int maxLength, String allowedCharacters, InputComponentListener changeListener);
public abstract PasswordField createPasswordField(InputComponentListener changeListener, int maxLength);
public abstract IList createList(Action... actions);
public abstract Input createComboBox(List object, InputComponentListener changeListener);
public abstract Input createCheckBox(InputComponentListener changeListener, String text);
public enum Size { SMALL, MEDIUM, LARGE };
public abstract Input createImage(Size size, InputComponentListener changeListener);
public interface IList extends IComponent {
/**
* @param enabled if false no content should be shown (or
* only in gray) and all actions must get disabled
*/
public void setEnabled(boolean enabled);
public void clear();
public void add(IComponent component, Action... actions);
}
public interface InputComponentListener {
void changed(IComponent source);
}
public interface Search {
public List search(String query);
}
public abstract Input createLookup(InputComponentListener changeListener, Search index, Object[] keys);
public abstract IComponent createComponentGroup(IComponent... components);
/**
* Content means the content of a dialog or of a page
*/
public interface IContent {
}
public interface FormContent extends IContent {
public void add(IComponent component);
public void add(String caption, IComponent component, int span);
public void setValidationMessages(IComponent component, List validationMessages);
}
public abstract FormContent createFormContent(int columns, int columnWidth);
public interface SwitchContent extends IContent {
public void show(IContent content);
}
public abstract SwitchContent createSwitchContent();
public interface ITable extends IContent {
public void setObjects(List objects);
}
public static interface TableActionListener {
public default void selectionChanged(U selectedObject, List selectedObjects) {
}
public default void action(U selectedObject) {
}
public default List getActions(U selectedObject, List selectedObjects) {
return Collections.emptyList();
}
}
public abstract ITable createTable(Object[] keys, TableActionListener listener);
public abstract IContent createHtmlContent(String htmlOrUrl);
//
public Subject getSubject() {
return getPageManager() != null ? getPageManager().getSubject() : null;
}
public void setSubject(Subject subject) {
getPageManager().setSubject(subject);
}
//
public abstract PageManager getPageManager();
//
public RESULT executeSync(Function function, INPUT input) {
return function.apply(input);
}
// delegating shortcuts
public static void show(Page page) {
getInstance().getPageManager().show(page);
}
public static void showDetail(Page mainPage, Page detail) {
getInstance().getPageManager().showDetail(mainPage, detail);
}
public static void hideDetail(Page page) {
getInstance().getPageManager().hideDetail(page);
}
public static boolean isDetailShown(Page page) {
return getInstance().getPageManager().isDetailShown(page);
}
public static IDialog showDialog(String title, IContent content, Action saveAction, Action closeAction, Action... actions) {
return getInstance().getPageManager().showDialog(title, content, saveAction, closeAction, actions);
}
public static IDialog showSearchDialog(Search index, Object[] keys, TableActionListener listener) {
return getInstance().getPageManager().showSearchDialog(index, keys, listener);
}
public static void showMessage(String text) {
getInstance().getPageManager().showMessage(text);
}
public static void showError(String text) {
getInstance().getPageManager().showError(text);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy