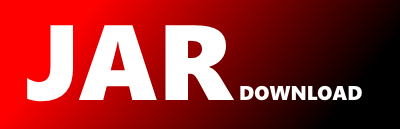
org.minimalj.frontend.impl.lanterna.toolkit.LanternaFrontend Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of minimalj Show documentation
Show all versions of minimalj Show documentation
A java framework aiming for a minimal programming style. Includes GUI and persistence layer.
package org.minimalj.frontend.impl.lanterna.toolkit;
import java.util.List;
import org.minimalj.frontend.Frontend;
import org.minimalj.frontend.action.Action;
import org.minimalj.frontend.impl.lanterna.LanternaGUIScreen;
import org.minimalj.frontend.impl.lanterna.component.LanternaForm;
import org.minimalj.frontend.page.PageManager;
import org.minimalj.model.Rendering;
import com.googlecode.lanterna.gui.component.Button;
public class LanternaFrontend extends Frontend {
private static ThreadLocal screenByThread = new ThreadLocal<>();
public LanternaFrontend() {
}
public static void setGui(LanternaGUIScreen value) {
if (value == null && screenByThread.get() != null) {
screenByThread.get().actionComplete();
}
screenByThread.set(value);
}
@Override
public Input createCheckBox(InputComponentListener changeListener, String text) {
return new LanternaCheckBox(changeListener, text);
}
@Override
public Input createComboBox(List objects, InputComponentListener changeListener) {
return new LanternaComboBox(objects, changeListener);
}
@Override
public IList createList(Action... actions) {
return new LanternaList(actions);
}
@Override
public FormContent createFormContent(int columns, int columnWidth) {
return new LanternaForm(columns);
}
@Override
public IComponent createComponentGroup(IComponent... components) {
return new LanternaHorizontalLayout(components);
}
@Override
public IComponent createText(final Action action) {
LanternaActionAdapter lanternaAction = new LanternaActionAdapter(action);
LanternaActionText button = new LanternaActionText(action.getName(), lanternaAction);
return button;
}
public static class LanternaActionText extends Button implements IComponent {
public LanternaActionText(String name, com.googlecode.lanterna.gui.Action action) {
super(name, action);
}
}
/*
* Cannot be done as inner class because lanterna action has to be provided
* to the button constructor. And the minimal-j action needs the component for
* the action method.
*/
private static class LanternaActionAdapter implements com.googlecode.lanterna.gui.Action {
private final LanternaGUIScreen browser;
private final Action action;
public LanternaActionAdapter(Action action) {
this.action = action;
this.browser = screenByThread.get();
}
@Override
public void doAction() {
screenByThread.set(browser);
action.action();
screenByThread.set(null);
}
}
@Override
public PageManager getPageManager() {
return screenByThread.get();
}
@Override
public IComponent createText(String text) {
return new LanternaText(text);
}
@Override
public IComponent createText(Rendering rendering) {
return new LanternaText(rendering);
}
@Override
public Input createReadOnlyTextField() {
return new LanternaReadOnlyTextField();
}
@Override
public Input createImage(Size size, InputComponentListener changeListener) {
throw new RuntimeException("Image not yet implemented in JsonFrontend");
};
@Override
public SwitchContent createSwitchContent() {
return new LanternaSwitchContent();
}
@Override
public ITable createTable(Object[] keys, TableActionListener listener) {
return new LanternaTable(keys, listener);
}
@Override
public IContent createHtmlContent(String htmlOrUrl) {
// TODO Auto-generated method stub
return null;
}
@Override
public Input createTextField(int maxLength, String allowedCharacters, InputType inputType, Search suggestionSearch,
InputComponentListener changeListener) {
return new LanternaTextField(changeListener);
}
@Override
public PasswordField createPasswordField(InputComponentListener changeListener, int maxLength) {
return new LanternaPasswordField(changeListener);
}
@Override
public Input createAreaField(int maxLength, String allowedCharacters, InputComponentListener changeListener) {
return new LanternaTextField(changeListener);
}
@Override
public IComponent createTitle(String text) {
return new LanternaText(text);
}
@Override
public Input createLookup(InputComponentListener changeListener, Search index, Object[] keys) {
// TODO Auto-generated method stub
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy