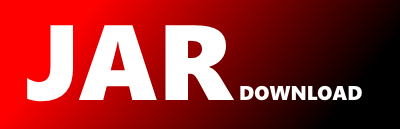
org.mitre.caasd.jlcl.BuildLinearControlComponent Maven / Gradle / Ivy
The newest version!
package org.mitre.caasd.jlcl;
import org.mitre.caasd.jlcl.components.FixedStepEulerIntegration;
import org.mitre.caasd.jlcl.components.FixedStepIntegrationArguments;
import org.mitre.caasd.jlcl.components.NoDifferentiation;
/**
* An example class for showing how to programmatically build a control component. See the main().
*
* @author SBOWMAN
* @see #main(String[])
*/
public class BuildLinearControlComponent {
/**
* Empty constructor.
*/
private BuildLinearControlComponent() {
// nothing here.
}
/**
* @param args
* The args.
*/
public static void main(final String[] args) {
/*
* How to build a PID component:
* 1. Build by hand
* 2. Build via factory methods
* a. P-only component
*/
// 1. Build a fully described PID by hand
// Double kp = 0d, ki = 0d, kd = 0d;
// NoIntegration i = new NoIntegration(Double.class);
// NoDifferentiation d = new NoDifferentiation(Double.class);
// ProportionalOnlyArguments pidArgs = new ProportionalOnlyArguments(Double.class);
// IPID, NoIntegrationArguments, NoDifferentiation, NoDifferentiationArguments> myPOnlyController = new PID, NoIntegrationArguments, NoDifferentiation, NoDifferentiationArguments>(Double.class, kp, i, ki, d, kd);
// 2a. Use the factory to create a simple p-only component
// Double kp = 0d;
// IPID, NoIntegrationArguments, NoDifferentiation, NoDifferentiationArguments> myPOnlyController = ControlComponentFactory.createProportionalOnlyPidComponentAsDouble(kp);
// ProportionalOnlyArguments pidArgs = new ProportionalOnlyArguments(Double.class);
// Use the component
// Double u = 0d;
// pidArgs.updateErrorSignalValue(u);
// Double y = myPOnlyController.evaluate(pidArgs);
// System.out.println("For an input of " + u + ", the PID produced an output of " + y);
}
private static void designProblemsToFix() {
final Double kp = 0.5, ki = 0.2;
final Double timeStep = 1.0;
FixedStepIntegrationArguments fixedStepArgs = new FixedStepIntegrationArguments(Double.class, timeStep); // Set the timestep. It cannot be updated (fixed step).
FixedStepEulerIntegration integrator = new FixedStepEulerIntegration(Double.class, fixedStepArgs);
NoDifferentiation derivator = new NoDifferentiation(Double.class);
/*
* Why do I have to define the integrator args multiple times in the interface declarations?
* SOLVED!!
*/
// PIController, FixedStepIntegrationArguments> testPid = new PIController, FixedStepIntegrationArguments>(Double.class, kp, integrator, ki);
/*
* Can we use the SpecialPID?
* Yup. This works.
*/
// SpecialPID1, FixedStepIntegrationArguments, NoDifferentiation, NoDifferentiationArguments> specialPid = new SpecialPID1, FixedStepIntegrationArguments, NoDifferentiation, NoDifferentiationArguments>(Double.class, kp, ki, integrator, derivator, 0d);
// IPidEvaluationArguments, NoDifferentiationArguments> pidEvalArgs = new ProportionalIntegralArguments>(Double.class);
// pidEvalArgs.updateErrorSignalValue(0d);
// specialPid.evaluate(pidEvalArgs);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy