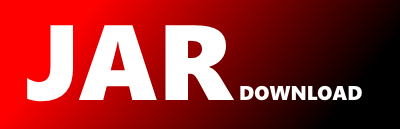
oasis.names.tc.ciq.xpil._3.PersonDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stix Show documentation
Show all versions of stix Show documentation
The Java bindings for STIX v.1.2.0
/**
* Copyright (c) 2015, The MITRE Corporation. All rights reserved.
* See LICENSE for complete terms.
*/
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.5-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2015.06.23 at 10:59:44 AM EDT
//
package oasis.names.tc.ciq.xpil._3;
import java.io.StringReader;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
import javax.xml.namespace.QName;
import javax.xml.transform.stream.StreamSource;
import oasis.names.tc.ciq.ct._3.DataQualityTypeList;
import oasis.names.tc.ciq.xnl._3.PersonNameType;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.HashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBHashCodeStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.mitre.stix.DocumentUtilities;
import org.mitre.stix.STIXSchema;
import org.mitre.stix.ValidationEventHandler;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <extension base="{urn:oasis:names:tc:ciq:xpil:3}PersonDetailsType">
* <anyAttribute namespace='##other'/>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
@XmlRootElement(name = "PersonDetails")
public class PersonDetails extends PersonDetailsType implements Equals,
HashCode, ToString {
/**
* Default no-arg constructor
*
*/
public PersonDetails() {
super();
}
/**
* Fully-initialising value constructor
*
*/
public PersonDetails(final FreeTextLines freeTextLines,
final List personNames, final Addresses addresses,
final Accounts accounts, final ContactNumbers contactNumbers,
final Documents documents,
final ElectronicAddressIdentifiers electronicAddressIdentifiers,
final Events events, final Identifiers identifiers,
final Memberships memberships, final Relationships relationships,
final Revenues revenues, final Stocks stocks,
final Vehicles vehicles, final PersonInfo personInfo,
final BirthInfo birthInfo,
final CountriesOfResidence countriesOfResidence,
final Favourites favourites, final Habits habits,
final Hobbies hobbies, final Languages languages,
final Nationalities nationalities, final Occupations occupations,
final PhysicalInfo physicalInfo, final Preferences preferences,
final Qualifications qualifications, final Visas visas,
final String usage, final String status,
final String personDetailsKey, final String personDetailsKeyRef,
final String languageCode,
final XMLGregorianCalendar dateValidFrom,
final XMLGregorianCalendar dateValidTo,
final DataQualityTypeList dataQualityType,
final XMLGregorianCalendar validFrom,
final XMLGregorianCalendar validTo,
final Map otherAttributes) {
super(freeTextLines, personNames, addresses, accounts, contactNumbers,
documents, electronicAddressIdentifiers, events, identifiers,
memberships, relationships, revenues, stocks, vehicles,
personInfo, birthInfo, countriesOfResidence, favourites,
habits, hobbies, languages, nationalities, occupations,
physicalInfo, preferences, qualifications, visas, usage,
status, personDetailsKey, personDetailsKeyRef, languageCode,
dateValidFrom, dateValidTo, dataQualityType, validFrom,
validTo, otherAttributes);
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator,
Object object, EqualsStrategy strategy) {
if (!(object instanceof PersonDetails)) {
return false;
}
if (this == object) {
return true;
}
if (!super.equals(thisLocator, thatLocator, object, strategy)) {
return false;
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
public int hashCode(ObjectLocator locator, HashCodeStrategy strategy) {
int currentHashCode = super.hashCode(locator, strategy);
return currentHashCode;
}
public int hashCode() {
final HashCodeStrategy strategy = JAXBHashCodeStrategy.INSTANCE;
return this.hashCode(null, strategy);
}
@Override
public PersonDetails withFreeTextLines(FreeTextLines value) {
setFreeTextLines(value);
return this;
}
@Override
public PersonDetails withPersonNames(PersonNameType... values) {
if (values != null) {
for (PersonNameType value : values) {
getPersonNames().add(value);
}
}
return this;
}
@Override
public PersonDetails withPersonNames(Collection values) {
if (values != null) {
getPersonNames().addAll(values);
}
return this;
}
@Override
public PersonDetails withAddresses(Addresses value) {
setAddresses(value);
return this;
}
@Override
public PersonDetails withAccounts(Accounts value) {
setAccounts(value);
return this;
}
@Override
public PersonDetails withContactNumbers(ContactNumbers value) {
setContactNumbers(value);
return this;
}
@Override
public PersonDetails withDocuments(Documents value) {
setDocuments(value);
return this;
}
@Override
public PersonDetails withElectronicAddressIdentifiers(
ElectronicAddressIdentifiers value) {
setElectronicAddressIdentifiers(value);
return this;
}
@Override
public PersonDetails withEvents(Events value) {
setEvents(value);
return this;
}
@Override
public PersonDetails withIdentifiers(Identifiers value) {
setIdentifiers(value);
return this;
}
@Override
public PersonDetails withMemberships(Memberships value) {
setMemberships(value);
return this;
}
@Override
public PersonDetails withRelationships(Relationships value) {
setRelationships(value);
return this;
}
@Override
public PersonDetails withRevenues(Revenues value) {
setRevenues(value);
return this;
}
@Override
public PersonDetails withStocks(Stocks value) {
setStocks(value);
return this;
}
@Override
public PersonDetails withVehicles(Vehicles value) {
setVehicles(value);
return this;
}
@Override
public PersonDetails withPersonInfo(PersonInfo value) {
setPersonInfo(value);
return this;
}
@Override
public PersonDetails withBirthInfo(BirthInfo value) {
setBirthInfo(value);
return this;
}
@Override
public PersonDetails withCountriesOfResidence(CountriesOfResidence value) {
setCountriesOfResidence(value);
return this;
}
@Override
public PersonDetails withFavourites(Favourites value) {
setFavourites(value);
return this;
}
@Override
public PersonDetails withHabits(Habits value) {
setHabits(value);
return this;
}
@Override
public PersonDetails withHobbies(Hobbies value) {
setHobbies(value);
return this;
}
@Override
public PersonDetails withLanguages(Languages value) {
setLanguages(value);
return this;
}
@Override
public PersonDetails withNationalities(Nationalities value) {
setNationalities(value);
return this;
}
@Override
public PersonDetails withOccupations(Occupations value) {
setOccupations(value);
return this;
}
@Override
public PersonDetails withPhysicalInfo(PhysicalInfo value) {
setPhysicalInfo(value);
return this;
}
@Override
public PersonDetails withPreferences(Preferences value) {
setPreferences(value);
return this;
}
@Override
public PersonDetails withQualifications(Qualifications value) {
setQualifications(value);
return this;
}
@Override
public PersonDetails withVisas(Visas value) {
setVisas(value);
return this;
}
@Override
public PersonDetails withUsage(String value) {
setUsage(value);
return this;
}
@Override
public PersonDetails withStatus(String value) {
setStatus(value);
return this;
}
@Override
public PersonDetails withPersonDetailsKey(String value) {
setPersonDetailsKey(value);
return this;
}
@Override
public PersonDetails withPersonDetailsKeyRef(String value) {
setPersonDetailsKeyRef(value);
return this;
}
@Override
public PersonDetails withLanguageCode(String value) {
setLanguageCode(value);
return this;
}
@Override
public PersonDetails withDateValidFrom(XMLGregorianCalendar value) {
setDateValidFrom(value);
return this;
}
@Override
public PersonDetails withDateValidTo(XMLGregorianCalendar value) {
setDateValidTo(value);
return this;
}
@Override
public PersonDetails withDataQualityType(DataQualityTypeList value) {
setDataQualityType(value);
return this;
}
@Override
public PersonDetails withValidFrom(XMLGregorianCalendar value) {
setValidFrom(value);
return this;
}
@Override
public PersonDetails withValidTo(XMLGregorianCalendar value) {
setValidTo(value);
return this;
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer,
ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator,
StringBuilder buffer, ToStringStrategy strategy) {
super.appendFields(locator, buffer, strategy);
return buffer;
}
/**
* Returns A Document representation of this instance that is not formatted.
*
* @return The Document representation for this instance.
*/
public org.w3c.dom.Document toDocument() {
return toDocument(false);
}
/**
* Returns A Document representation for this instance.
*
* @param prettyPrint
* True for pretty print, otherwise false
*
* @return The Document representation for this instance.
*/
public org.w3c.dom.Document toDocument(boolean prettyPrint) {
return DocumentUtilities.toDocument(toJAXBElement(), prettyPrint);
}
/**
* Returns JAXBElement for this instance.
*
* @return The JAXBElement for this instance.
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public JAXBElement> toJAXBElement() {
QName qualifiedName = STIXSchema.getQualifiedName(this);
return new JAXBElement(qualifiedName, PersonDetails.class, this);
}
/**
* Returns String representation of this instance that is not formatted.
*
* @return The String containing the XML mark-up.
*/
public String toXMLString() {
return toXMLString(false);
}
/**
* Returns XML String for JAXB Document Object Model object.
*
* @param prettyPrint
* True for pretty print, otherwise false
*
* @return The String containing the XML mark-up.
*/
public String toXMLString(boolean prettyPrint) {
return DocumentUtilities.toXMLString(toDocument(), prettyPrint);
}
/**
* Creates PersonDetails instance for XML String
*
* @param text
* XML String for the document
* @return The PersonDetails instance for the passed XML String
*/
public static PersonDetails fromXMLString(String text) {
JAXBContext jaxbContext;
try {
jaxbContext = JAXBContext.newInstance(PersonDetails.class
.getPackage().getName());
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
unmarshaller.setSchema(STIXSchema.getInstance().getSchema());
unmarshaller.setEventHandler(new ValidationEventHandler());
StreamSource streamSource = new StreamSource(new StringReader(text));
return (PersonDetails) unmarshaller.unmarshal(streamSource);
} catch (JAXBException e) {
throw new RuntimeException(e);
}
}
/**
* Validates the XML representation of this PersonDetails instance
* Returning true indicating a successful validation, false if not.
*
* @return boolean
*/
public boolean validate() {
return STIXSchema.getInstance().validate(toXMLString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy