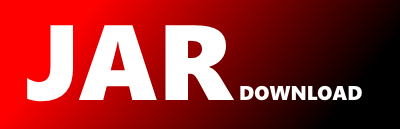
org.mixer2.xhtml.util.InsertByIdUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mixer2 Show documentation
Show all versions of mixer2 Show documentation
Mixer2 is template engine for java.
package org.mixer2.xhtml.util;
import java.util.List;
import java.util.ListIterator;
import org.mixer2.jaxb.xhtml.*;
import org.mixer2.xhtml.AbstractJaxb;
import org.mixer2.xhtml.TagEnum;
import org.mixer2.xhtml.exception.TagTypeUnmatchException;
/**
*
*
* @author watanabe
*
*/
public class InsertByIdUtil {
/**
* 指定したid属性を持つ要素の次の要素としてinsObjectを挿入する なお、実際に挿入されるのはinsObjectのディープコピーです。
*
* @param
* @param id
* @param insObject
* @param target
* @return
* @throws TagTypeUnmatchException
*/
public static boolean insertAfterId(String id,
T insObject, T target) throws TagTypeUnmatchException {
return execute(OperationEnum.AFTER, id,
insObject.copy(insObject.getClass()), target);
}
public static boolean insertAfterId(String id,
String insString, T target) throws TagTypeUnmatchException {
return execute(OperationEnum.AFTER, id, insString, target);
}
public static boolean insertBeforeId(String id,
T insObject, T target) throws TagTypeUnmatchException {
return execute(OperationEnum.BEFORE, id,
insObject.copy(insObject.getClass()), target);
}
public static boolean insertBeforeId(String id,
String insString, T target) throws TagTypeUnmatchException {
execute(OperationEnum.BEFORE, id, insString, target);
return false;
}
private enum OperationEnum {
AFTER, BEFORE;
}
@SuppressWarnings("unchecked")
private static boolean executeWithinObjectList(
OperationEnum opEnum, String id, java.lang.Object val,
List list) throws TagTypeUnmatchException {
for (ListIterator j = list.listIterator(); j
.hasNext();) {
java.lang.Object obj = j.next();
if (obj instanceof AbstractJaxb) {
if (id.equals(((AbstractJaxb) obj).getId())) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
if (ii <= 0) {
list.add(0, val);
} else {
list.add(ii, val);
}
break;
case AFTER:
j.add(val);
break;
}
return true;
} else {
if (execute(opEnum, id, val, (T) obj)) {
return true;
}
}
}
}
return false;
}
@SuppressWarnings("unchecked")
private static boolean execute(
OperationEnum opEnum, String id, java.lang.Object val, T target)
throws TagTypeUnmatchException {
TagEnum tagEnum = TagEnum.valueOf(target.getClass().getSimpleName()
.toUpperCase());
TagEnum valTagEnum = null;
if (val instanceof AbstractJaxb) {
valTagEnum = TagEnum.valueOf(val.getClass().getSimpleName()
.toUpperCase());
}
int size = 0;
switch (tagEnum) {
case A:
A a = (A) target;
if (a.isSetContent()
&& executeWithinObjectList(opEnum, id, val, a.getContent())) {
return true;
}
break;
case ABBR:
Abbr abbr = (Abbr) target;
if (abbr.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
abbr.getContent())) {
return true;
}
break;
case ACRONYM:
Acronym acronym = (Acronym) target;
if (acronym.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
acronym.getContent())) {
return true;
}
break;
case ADDRESS:
Address address = (Address) target;
if (address.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
address.getContent())) {
return true;
}
break;
case APPLET:
Applet applet = (Applet) target;
if (applet.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
applet.getContent())) {
return true;
}
break;
case AREA:
// empty element.
break;
case B:
B b = (B) target;
if (b.isSetContent()
&& executeWithinObjectList(opEnum, id, val, b.getContent())) {
return true;
}
break;
case BASE:
// empty element.
break;
case BASEFONT:
// empty element.
break;
case BDO:
Bdo bdo = (Bdo) target;
if (bdo.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
bdo.getContent())) {
return true;
}
break;
case BIG:
Big big = (Big) target;
if (big.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
big.getContent())) {
return true;
}
break;
case BLOCKQUOTE:
Blockquote blockquote = (Blockquote) target;
if (blockquote.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
blockquote.getContent())) {
return true;
}
break;
case BODY:
Body body = (Body) target;
if (body.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
body.getContent())) {
return true;
}
break;
case BR:
// empty element
break;
case BUTTON:
Button button = (Button) target;
if (button.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
button.getContent())) {
return true;
}
break;
case CAPTION:
Caption caption = (Caption) target;
if (caption.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
caption.getContent())) {
return true;
}
break;
case CENTER:
Center center = (Center) target;
if (center.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
center.getContent())) {
return true;
}
break;
case CITE:
Cite cite = (Cite) target;
if (cite.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
cite.getContent())) {
return true;
}
break;
case CODE:
Code code = (Code) target;
if (code.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
code.getContent())) {
return true;
}
break;
case COL:
// empty element.
break;
case COLGROUP:
Colgroup colgroup = (Colgroup) target;
if (colgroup.isSetCol()) {
for (ListIterator j = colgroup.getCol().listIterator(); j
.hasNext();) {
Col col = j.next();
if (id.equals(col.getId())) {
if (val.getClass().equals(Col.class)) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
colgroup.getCol().add(ii, (Col) val);
break;
case AFTER:
j.add((Col) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(Col.class,
val.getClass());
}
}
}
}
break;
case DD:
Dd dd = (Dd) target;
if (dd.isSetContent()
&& executeWithinObjectList(opEnum, id, val, dd.getContent())) {
return true;
}
break;
case DEL:
Del del = (Del) target;
if (del.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
del.getContent())) {
return true;
}
break;
case DFN:
Dfn dfn = (Dfn) target;
if (dfn.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
dfn.getContent())) {
return true;
}
break;
case DIR:
Dir dir = (Dir) target;
if (dir.isSetLi()) {
for (ListIterator j = dir.getLi().listIterator(); j
.hasNext();) {
Li li = j.next();
if (id.equals(li.getId())) {
if (val.getClass().equals(Li.class)) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
dir.getLi().add(ii, (Li) val);
break;
case AFTER:
j.add((Li) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(Li.class,
val.getClass());
}
} else {
if (execute(opEnum, id, val, li)) {
return true;
}
}
}
}
break;
case DIV:
Div div = (Div) target;
if (div.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
div.getContent())) {
return true;
}
break;
case DL:
Dl dl = (Dl) target;
if (dl.isSetDtOrDd()) {
for (ListIterator j = dl.getDtOrDd()
.listIterator(); j.hasNext();) {
AbstractJaxb aj = j.next();
if (id.equals(aj.getId())) {
if (val instanceof AbstractJaxb) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
dl.getDtOrDd().add(ii, (T) val);
break;
case AFTER:
j.add((Li) val);
break;
}
return true;
} else {
}
} else {
if (execute(opEnum, id, val, aj)) {
return true;
}
}
}
}
break;
case DT:
Dt dt = (Dt) target;
if (dt.isSetContent()
&& executeWithinObjectList(opEnum, id, val, dt.getContent())) {
return true;
}
break;
case EM:
Em em = (Em) target;
if (em.isSetContent()
&& executeWithinObjectList(opEnum, id, val, em.getContent())) {
return true;
}
break;
case FIELDSET:
Fieldset fieldset = (Fieldset) target;
if (fieldset.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
fieldset.getContent())) {
return true;
}
break;
case FONT:
Font font = (Font) target;
if (font.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
font.getContent())) {
return true;
}
break;
case FORM:
Form form = (Form) target;
if (form.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
form.getContent())) {
return true;
}
break;
case H1:
H1 h1 = (H1) target;
if (h1.isSetContent()
&& executeWithinObjectList(opEnum, id, val, h1.getContent())) {
return true;
}
break;
case H2:
H2 h2 = (H2) target;
if (h2.isSetContent()
&& executeWithinObjectList(opEnum, id, val, h2.getContent())) {
return true;
}
break;
case H3:
H3 h3 = (H3) target;
if (h3.isSetContent()
&& executeWithinObjectList(opEnum, id, val, h3.getContent())) {
return true;
}
break;
case H4:
H4 h4 = (H4) target;
if (h4.isSetContent()
&& executeWithinObjectList(opEnum, id, val, h4.getContent())) {
return true;
}
break;
case H5:
H5 h5 = (H5) target;
if (h5.isSetContent()
&& executeWithinObjectList(opEnum, id, val, h5.getContent())) {
return true;
}
break;
case H6:
H6 h6 = (H6) target;
if (h6.isSetContent()
&& executeWithinObjectList(opEnum, id, val, h6.getContent())) {
return true;
}
break;
case HGROUP:
Hgroup hgroup = (Hgroup) target;
if (hgroup.isSetH1OrH2OrH3()) {
for (ListIterator j = hgroup.getH1OrH2OrH3()
.listIterator(); j.hasNext();) {
Inline inline = j.next();
if (id.equals(inline.getId())) {
if (val instanceof H1 || val instanceof H2
|| val instanceof H3 || val instanceof H4
|| val instanceof H5 || val instanceof H6) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
hgroup.getH1OrH2OrH3().add(ii, (Inline) val);
break;
case AFTER:
j.add((Inline) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(
"h1-h6以外のタグをhgroupにInsertしようとしました");
}
} else {
if (execute(opEnum, id, val, inline)) {
return true;
}
}
}
}
break;
case HEAD:
Head head = (Head) target;
for (ListIterator j = head.getContent()
.listIterator(); j.hasNext();) {
AbstractJaxb aj = j.next();
if (id.equals(aj.getId())) {
if (val instanceof AbstractJaxb) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
head.getContent().add(ii, (AbstractJaxb) val);
break;
case AFTER:
j.add((AbstractJaxb) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(AbstractJaxb.class,
aj.getClass());
}
} else {
if (execute(opEnum, id, val, aj)) {
return true;
}
}
}
break;
case HR:
// empty element
break;
case HTML:
Html html = (Html) target;
if (html.isSetHead()) {
if (execute(opEnum, id, val, html.getHead())) {
return true;
}
}
if (html.isSetBody()) {
if (execute(opEnum, id, val, html.getBody())) {
return true;
}
}
break;
case I:
I i = (I) target;
if (i.isSetContent()
&& executeWithinObjectList(opEnum, id, val, i.getContent())) {
return true;
}
break;
case IFRAME:
Iframe iframe = (Iframe) target;
if (iframe.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
iframe.getContent())) {
return true;
}
break;
case IMG:
// empty element
break;
case INPUT:
// empty element
break;
case INS:
Ins ins = (Ins) target;
if (ins.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
ins.getContent())) {
return true;
}
break;
case ISINDEX:
// empty element.
break;
case KBD:
Kbd kbd = (Kbd) target;
if (kbd.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
kbd.getContent())) {
return true;
}
break;
case LABEL:
Label label = (Label) target;
if (label.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
label.getContent())) {
return true;
}
break;
case LEGEND:
Legend legend = (Legend) target;
if (legend.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
legend.getContent())) {
return true;
}
break;
case LI:
Li li = (Li) target;
if (li.isSetContent()
&& executeWithinObjectList(opEnum, id, val, li.getContent())) {
return true;
}
break;
case LINK:
// empty element
break;
case MAP:
Map map = (Map) target;
if (map.isSetArea()) {
for (ListIterator j = map.getArea().listIterator(); j
.hasNext();) {
Area area = j.next();
if (id.equals(area.getId())) {
if (val instanceof Area) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
map.getArea().add(ii, (Area) val);
break;
case AFTER:
j.add((Area) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(Area.class,
val.getClass());
}
}
}
}
if (map.isSetPOrH1OrH2()) {
for (ListIterator j = map.getPOrH1OrH2()
.listIterator(); j.hasNext();) {
AbstractJaxb aj = j.next();
if (id.equals(aj.getId())) {
if (val instanceof AbstractJaxb) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
map.getPOrH1OrH2().add(ii, (AbstractJaxb) val);
break;
case AFTER:
j.add((AbstractJaxb) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(
AbstractJaxb.class, val.getClass());
}
} else {
if (execute(opEnum, id, val, aj)) {
return true;
}
}
}
}
break;
case MENU:
Menu menu = (Menu) target;
if (menu.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
menu.getContent())) {
return true;
}
break;
case META:
// empty element
break;
case NOFRAMES:
Noframes noframes = (Noframes) target;
if (noframes.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
noframes.getContent())) {
return true;
}
break;
case NOSCRIPT:
Noscript noscript = (Noscript) target;
if (noscript.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
noscript.getContent())) {
return true;
}
break;
case OBJECT:
org.mixer2.jaxb.xhtml.Object object = (org.mixer2.jaxb.xhtml.Object) target;
if (object.isSetContent()
&& executeWithinObjectList(opEnum, id, val,
object.getContent())) {
return true;
}
break;
case OL:
Ol ol = (Ol) target;
if (ol.isSetLi()) {
for (ListIterator j = ol.getLi().listIterator(); j
.hasNext();) {
Li tmpli = j.next();
if (id.equals(tmpli.getId())) {
if (val instanceof Li) {
switch (opEnum) {
case BEFORE:
int ii = j.previousIndex();
ol.getLi().add(ii, (Li) val);
break;
case AFTER:
j.add((Li) val);
break;
}
return true;
} else {
throw new TagTypeUnmatchException(Li.class,
val.getClass());
}
} else {
if (execute(opEnum, id, val, tmpli)) {
return true;
}
}
}
}
break;
case OPTGROUP:
Optgroup optgroup = (Optgroup) target;
if (optgroup.isSetOption()) {
for (ListIterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy