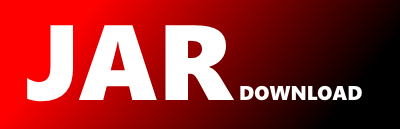
org.mixer2.xhtml.util.RemoveByIdUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mixer2 Show documentation
Show all versions of mixer2 Show documentation
Mixer2 is template engine for java.
package org.mixer2.xhtml.util;
import java.util.List;
import org.mixer2.jaxb.xhtml.*;
import org.mixer2.xhtml.AbstractJaxb;
import org.mixer2.xhtml.TagEnum;
/**
*
* @see org.mixer2.xhtml.AbstractJaxb#removeById(String)
* @author watanabe
*
*/
public class RemoveByIdUtil {
@SuppressWarnings("unchecked")
private static boolean removeByIdWithinObjectList(
String id, List list) {
java.lang.Object tmpobj;
int size = list.size();
for (int i = 0; i < size; i++) {
tmpobj = list.get(i);
if (tmpobj instanceof AbstractJaxb) {
if (id.equals(((AbstractJaxb) tmpobj).getId())) {
list.remove(i);
return true;
} else {
if (removeById(id, (T) tmpobj)) {
return true;
}
}
}
}
return false;
}
public static boolean removeById(String id,
T target) {
TagEnum tagEnum = TagEnum.valueOf(target.getClass().getSimpleName()
.toUpperCase());
if (id.equals(target.getId())) {
return false;
}
switch (tagEnum) {
case A:
A a = (A) target;
if (a.isSetContent()) {
return removeByIdWithinObjectList(id, a.getContent());
}
break;
case ABBR:
Abbr abbr = (Abbr) target;
if (abbr.isSetContent()) {
return removeByIdWithinObjectList(id, abbr.getContent());
}
break;
case ACRONYM:
Acronym acronym = (Acronym) target;
if (acronym.isSetContent()) {
return removeByIdWithinObjectList(id, acronym.getContent());
}
break;
case ADDRESS:
Address address = (Address) target;
if (address.isSetContent()) {
return removeByIdWithinObjectList(id, address.getContent());
}
break;
case APPLET:
Applet applet = (Applet) target;
if (applet.isSetContent()) {
return removeByIdWithinObjectList(id, applet.getContent());
}
break;
case AREA:
// area is empty element.
break;
case B:
B b = (B) target;
if (b.isSetContent()) {
return removeByIdWithinObjectList(id, b.getContent());
}
break;
case BASE:
// empty element.
break;
case BASEFONT:
// empty element.
break;
case BDO:
Bdo bdo = (Bdo) target;
if (bdo.isSetContent()) {
return removeByIdWithinObjectList(id, bdo.getContent());
}
break;
case BIG:
Big big = (Big) target;
if (big.isSetContent()) {
return removeByIdWithinObjectList(id, big.getContent());
}
break;
case BLOCKQUOTE:
Blockquote blockquote = (Blockquote) target;
if (blockquote.isSetContent()) {
return removeByIdWithinObjectList(id, blockquote.getContent());
}
break;
case BODY:
Body body = (Body) target;
if (body.isSetContent()) {
return removeByIdWithinObjectList(id, body.getContent());
}
break;
case BR:
// empty element.
break;
case BUTTON:
Button button = (Button) target;
if (button.isSetContent()) {
return removeByIdWithinObjectList(id, button.getContent());
}
break;
case CAPTION:
Caption caption = (Caption) target;
if (caption.isSetContent()) {
return removeByIdWithinObjectList(id, caption.getContent());
}
break;
case CENTER:
Center center = (Center) target;
if (center.isSetContent()) {
return removeByIdWithinObjectList(id, center.getContent());
}
break;
case CITE:
Cite cite = (Cite) target;
if (cite.isSetContent()) {
return removeByIdWithinObjectList(id, cite.getContent());
}
break;
case CODE:
Code code = (Code) target;
if (code.isSetContent()) {
return removeByIdWithinObjectList(id, code.getContent());
}
break;
case COL:
// empty element.
break;
case COLGROUP:
Colgroup colgroup = (Colgroup) target;
if (colgroup.isSetCol()) {
for (int i = 0; i < colgroup.getCol().size(); i++) {
Col col1 = colgroup.getCol().get(i);
if (col1.isSetId() && col1.getId().equals(col1)) {
colgroup.getCol().remove(i);
return true;
}
}
}
break;
case DD:
Dd dd = (Dd) target;
if (dd.isSetContent()) {
return removeByIdWithinObjectList(id, dd.getContent());
}
break;
case DEL:
Del del = (Del) target;
if (del.isSetContent()) {
return removeByIdWithinObjectList(id, del.getContent());
}
break;
case DFN:
Dfn dfn = (Dfn) target;
if (dfn.isSetContent()) {
return removeByIdWithinObjectList(id, dfn.getContent());
}
break;
case DIR:
Dir dir = (Dir) target;
if (dir.isSetLi()) {
for (int i = 0; i < dir.getLi().size(); i++) {
Li li = dir.getLi().get(i);
if (li.isSetId() && li.getId().equals(id)) {
dir.getLi().remove(i);
return true;
} else {
if (removeById(id, li)) {
return true;
}
}
}
}
break;
case DIV:
Div div = (Div) target;
if (div.isSetContent()) {
return removeByIdWithinObjectList(id, div.getContent());
}
break;
case DL:
Dl dl = (Dl) target;
if (dl.isSetDtOrDd()) {
for (int i = 0; i < dl.getDtOrDd().size(); i++) {
AbstractJaxb obj = dl.getDtOrDd().get(i);
if (id.equals(obj.getId())) {
dl.getDtOrDd().remove(i);
return true;
} else {
if (removeById(id, obj)) {
return true;
}
}
}
}
break;
case DT:
Dt dt = (Dt) target;
if (dt.isSetContent()) {
return removeByIdWithinObjectList(id, dt.getContent());
}
break;
case EM:
Em em = (Em) target;
if (em.isSetId() && em.getId().equals(id)) {
return false;
}
if (em.isSetContent()) {
return removeByIdWithinObjectList(id, em.getContent());
}
break;
case FIELDSET:
Fieldset fieldset = (Fieldset) target;
if (fieldset.isSetContent()) {
return removeByIdWithinObjectList(id, fieldset.getContent());
}
break;
case FONT:
Font font = (Font) target;
if (font.isSetContent()) {
return removeByIdWithinObjectList(id, font.getContent());
}
break;
case FORM:
Form form = (Form) target;
if (form.isSetContent()) {
return removeByIdWithinObjectList(id, form.getContent());
}
break;
case H1:
H1 h1 = (H1) target;
if (h1.isSetContent()) {
return removeByIdWithinObjectList(id, h1.getContent());
}
break;
case H2:
H2 h2 = (H2) target;
if (h2.isSetContent()) {
return removeByIdWithinObjectList(id, h2.getContent());
}
break;
case H3:
H3 h3 = (H3) target;
if (h3.isSetContent()) {
return removeByIdWithinObjectList(id, h3.getContent());
}
break;
case H4:
H4 h4 = (H4) target;
if (h4.isSetContent()) {
return removeByIdWithinObjectList(id, h4.getContent());
}
break;
case H5:
H5 h5 = (H5) target;
if (h5.isSetContent()) {
return removeByIdWithinObjectList(id, h5.getContent());
}
break;
case H6:
H6 h6 = (H6) target;
if (h6.isSetContent()) {
return removeByIdWithinObjectList(id, h6.getContent());
}
break;
case HGROUP:
Hgroup hgroup = (Hgroup)target;
if (hgroup.isSetH1OrH2OrH3()) {
for (AbstractJaxb obj : hgroup.getH1OrH2OrH3()) {
if (removeById(id,obj)) {
return true;
}
}
}
break;
case HEAD:
Head head = (Head) target;
for (int j =0; j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy