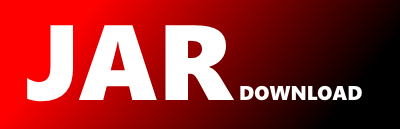
net.fortuna.ical4j.model.PropertyContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ical4j Show documentation
Show all versions of ical4j Show documentation
A Java library for reading and writing iCalendar (*.ics) files
package net.fortuna.ical4j.model;
import org.jetbrains.annotations.NotNull;
import java.util.Collection;
import java.util.function.BiFunction;
import java.util.function.Predicate;
public interface PropertyContainer extends PropertyListAccessor {
BiFunction ADD_IF_NOT_PRESENT = (c, p) -> {
if (!c.getProperty(p.getName()).isPresent()) {
c.add(p);
}
return c;
};
void setPropertyList(PropertyList properties);
/**
* Add a property to the container.
* @param property the property to add
* @return a reference to the container to support method chaining
*/
default T add(@NotNull Property property) {
setPropertyList((PropertyList) getPropertyList().add(property));
return (T) this;
}
/**
* Add multiple properties to the container.
* @param properties a collection of properties to add
* @return a reference to the container to support method chaining
*/
default T addAll(@NotNull Collection properties) {
setPropertyList((PropertyList) getPropertyList().addAll(properties));
return (T) this;
}
/**
* Remove a property from the container.
* @param property the property to remove
* @return a reference to the container to support method chaining
*/
default T remove(Property property) {
setPropertyList((PropertyList) getPropertyList().remove(property));
return (T) this;
}
/**
* Remove all properties with the matching name.
* @param name name of the properties to remove
* @return a reference to the container to support method chaining
*/
default T removeAll(String... name) {
setPropertyList((PropertyList) getPropertyList().removeAll(name));
return (T) this;
}
/**
* Remove all properties matching the specified filter.
* @param filter a filter predicate for matching
* @return a reference to the container to support method chaining
*/
default T removeIf(Predicate filter) {
setPropertyList((PropertyList) getPropertyList().removeIf(filter));
return (T) this;
}
/**
* Add a property to the container whilst removing all other properties with the same property name.
* @param property the property to add
* @return a reference to the container to support method chaining
*/
default T replace(Property property) {
setPropertyList((PropertyList) getPropertyList().replace(property));
return (T) this;
}
/**
* A functional method used to apply a property to a container in an undefined way.
*
* For example, a null check can be introduced as follows:
*
* container.with((c, p) -> if (p != null) c.add(p); return c;)
* @param f
* @param p
* @return
* @param
*/
default T with(BiFunction f, P p) {
return f.apply((T) this, p);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy