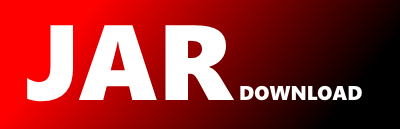
net.fortuna.ical4j.model.ComponentBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ical4j Show documentation
Show all versions of ical4j Show documentation
A Java library for reading and writing iCalendar (*.ics) files
package net.fortuna.ical4j.model;
import net.fortuna.ical4j.model.component.XComponent;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class ComponentBuilder extends AbstractContentBuilder {
private final List> factories;
private String name;
private final List properties = new ArrayList<>();
private final List subComponents = new ArrayList<>();
public ComponentBuilder() {
this(true);
}
public ComponentBuilder(boolean allowIllegalNames) {
this(Collections.emptyList(), allowIllegalNames);
}
public ComponentBuilder(List> factories) {
this(factories, true);
}
public ComponentBuilder(List> factories, boolean allowIllegalNames) {
super(allowIllegalNames);
this.factories = factories;
}
public ComponentBuilder> name(String name) {
// component names are case-insensitive, but convert to upper case to simplify further processing
this.name = name.toUpperCase();
return this;
}
public boolean hasName(String name) {
return name.equals(this.name);
}
public ComponentBuilder> property(Property property) {
properties.add(property);
return this;
}
public ComponentBuilder> subComponent(Component subComponent) {
subComponents.add(subComponent);
return this;
}
@SuppressWarnings("unchecked")
public T build() {
Component component = null;
for (ComponentFactory> factory : factories) {
if (factory.supports(name)) {
if (!subComponents.isEmpty()) {
component = factory.createComponent(new PropertyList(properties),
new ComponentList<>(subComponents));
} else {
component = factory.createComponent(new PropertyList(properties));
}
}
}
if (component == null) {
if (isExperimentalName(name)) {
component = new XComponent(name, new PropertyList(properties));
} else if (allowIllegalNames()) {
component = new XComponent(name, new PropertyList(properties));
} else {
throw new IllegalArgumentException("Unsupported component [" + name + "]");
}
}
return (T) component;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy