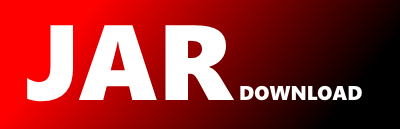
net.fortuna.ical4j.model.ContentCollection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ical4j Show documentation
Show all versions of ical4j Show documentation
A Java library for reading and writing iCalendar (*.ics) files
package net.fortuna.ical4j.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.stream.Collectors;
/**
* Implementors of this interface support the immutable collection contract specified by this interface.
*
* The contract states that any mutation function will not modify the underlying collection, but rather
* will return a copy of the collection with the applied mutation.
*
* @param
*/
public interface ContentCollection extends Serializable {
ContentCollection add(T content);
ContentCollection addAll(Collection content);
ContentCollection remove(T content);
ContentCollection removeAll(String... name);
ContentCollection removeIf(Predicate filter);
ContentCollection replace(T content);
List getAll();
/**
* Return a list of elements filtered by name. If no names are specified return all elements.
* @param names a list of zero or more names to match
* @param content type
* @return a list of elements less than or equal to the elements in this collection
*/
@SuppressWarnings("unchecked")
default List get(String... names) {
if (names.length > 0) {
List filter = Arrays.stream(names).map(String::toUpperCase).collect(Collectors.toList());
return getAll().stream().filter(c -> filter.contains(c.getName())).map(c -> (R) c).collect(Collectors.toList());
} else {
return (List) getAll();
}
}
@SuppressWarnings("unchecked")
default Optional getFirst(String name) {
return (Optional) getAll().stream().filter(c -> c.getName().equalsIgnoreCase(name)).findFirst();
}
@SuppressWarnings("unchecked")
default R getRequired(String name) throws ConstraintViolationException {
return (R) getFirst(name).orElseThrow(() -> new ConstraintViolationException(
String.format("Missing required %s", name)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy