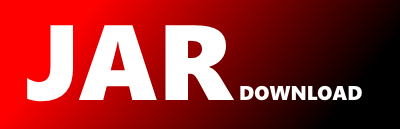
en-US.Section-JDiameter-Source_Overview.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE book PUBLIC "-//OASIS//DTD DocBook XML V4.5//EN" "http://www.oasis-open.org/docbook/xml/4.5/docbookx.dtd" [ <!ENTITY % BOOK_ENTITIES SYSTEM "Diameter_User_Guide.ent"> %BOOK_ENTITIES; ]> <section id="jdiameter-source_overview"> <!-- TODO: Developer: add section per each app session and factory, explain how it is created --> <title>Diameter Stack Source overview</title> <para>Diameter stack is built with the following basic components:</para> <para> <variablelist> <varlistentry> <term>Session Factory</term> <listitem> <para>The Session Factory governs the creation of sessions - raw and specific application sessions.</para> </listitem> </varlistentry> <varlistentry> <term>Raw and Application Sessions</term> <listitem> <para>Sessions govern stateful message routing between peers. Specific application sessions consume different type of messages and act differently based on the data present.</para> </listitem> </varlistentry> <varlistentry> <term>Stack</term> <listitem> <para>The Stack governs all necessary components, which are used to establish connection and communicate with remote peers.</para> </listitem> </varlistentry> </variablelist> <note> <para>For more detailed information, please refer to the Javadoc or the simple examples that can be found here: <ulink url="&THIS.TESTSUITE_TRUNK_SOURCE_CODE_URL;">SVN Testsuite Trunk</ulink>.</para> </note> </para> <section id="jdiameter-source_overview-session_factory"> <title>Session Factory</title> <para><classname>SessionFactory</classname> provides the stack user with access to session objects. It manages registered application session factories in order to allow for the creation of specific application sessions. A Session Factory instance can be obtained from the stack using the <methodname>getSessionFactory()</methodname> method. The base <classname>SessionFactory</classname> interface is defined below:</para> <programlisting role="JAVA" language="Java">package org.jdiameter.api; import org.jdiameter.api.app.AppSession; public interface SessionFactory { RawSession getNewRawSession() throws InternalException; Session getNewSession() throws InternalException; Session getNewSession(String sessionId) throws InternalException; <T extends AppSession> T getNewAppSession(ApplicationId applicationId, Class<? extends AppSession> userSession) throws InternalException; <T extends AppSession> T getNewAppSession(String sessionId, ApplicationId applicationId, Class<? extends AppSession> userSession) throws InternalException; }</programlisting> <para>However, since the stack is extensible, it is safe to cast the <classname>SessionFactory</classname> object to this interface:</para> <programlisting role="JAVA" language="Java">package org.jdiameter.client.api; public interface ISessionFactory extends SessionFactory { <T extends AppSession> T getNewAppSession(String sessionId, ApplicationId applicationId, java.lang.Class<? extends AppSession> aClass, Object... args) throws InternalException; void registerAppFacory(Class<? extends AppSession> sessionClass, IAppSessionFactory factory); void unRegisterAppFacory(Class<? extends AppSession> sessionClass); IConcurrentFactory getConcurrentFactory(); }</programlisting> <variablelist> <varlistentry> <term><methodname>RawSession getNewRawSession() throws InternalException;</methodname></term> <listitem><para>This method creates a <classname>RawSession</classname>. Raw sessions are meant as handles for code performing part of the routing decision on the stack's, such as rely agents for instance.</para></listitem> </varlistentry> <varlistentry> <term><methodname>Session getNewSession() throws InternalException;</methodname></term> <listitem><para>This method creates a session that acts as the endpoint for peer communication (for a given session ID). It declares the method that works with the <classname>Request</classname> and <classname>Answer</classname> objects. A session created with this method has an autogenerated ID. It should be considered as a client session.</para></listitem> </varlistentry> <varlistentry> <term><methodname>Session getNewSession(String sessionId) throws InternalException;</methodname></term> <listitem><para>As above. However, the created session has an ID equal to that passsed as an argument. This created session should be considered a server session.</para></listitem> </varlistentry> <varlistentry> <term><methodname><T extends AppSession> T getNewAppSession(ApplicationId applicationId, Class<? extends AppSession> userSession) throws InternalException;</methodname></term> <listitem><para>This method creates a new specific application session, identified by the application ID and class of the session passed. The session ID is generated by implementation. New application sessions should be considered as client sessions. It is safe to type cast the return value to class passed as an argument. This method delegates the call to a specific application session factory. </para></listitem> </varlistentry> <varlistentry> <term><methodname><T extends AppSession> T getNewAppSession(String sessionId, ApplicationId applicationId, Class<? extends AppSession> userSession) throws InternalException;</methodname></term> <listitem><para>As above. However, the session Id is equal to the argument passed. New sessions should be considered server sessions.</para></listitem> </varlistentry> <varlistentry> <term><methodname><T extends AppSession> T getNewAppSession(String sessionId, ApplicationId applicationId, java.lang.Class<? extends AppSession> aClass, Object... args) throws InternalException;</methodname></term> <listitem><para>As above. However, it allows the stack to pass some additional arguments. Passed values are implementation specifc.</para></listitem> </varlistentry> <varlistentry> <term><methodname>void registerAppFacory(Class<? extends AppSession> sessionClass, IAppSessionFactory factory);</methodname></term> <listitem><para>Registers the <parameter>factory</parameter> for a certain <parameter>sessionClass</parameter>. This factory will receive a delegated call when ever the <methodname>getNewAppSession</methodname> method is called with an application class matching one from the register method.</para></listitem> </varlistentry> <varlistentry> <term><methodname>void unRegisterAppFacory(Class<? extends AppSession> sessionClass);</methodname></term> <listitem><para>Removes the application session factory registered for the <parameter>sessionClass</parameter>. </para></listitem> </varlistentry> </variablelist> <example> <title>SessionFactory use example</title> <programlisting language="Java" role="JAVA">class Test implements EventListener<Request, Answer> { .... public void test(){ Stack stack = new StackImpl(); XMLConfiguration config = new XMLConfiguration(new FileInputStream(new File(configFile)); SessionFactory sessionFactory = stack.init(config); stack.start(); //perferctly legal, both factories are the same. sessionFactor = stack.getSessionFactory(); Session session = sessionFactory.getNewSession(); session.setRequestListener(this); Request r = session.createRequest(308,ApplicationId.createByAuth(100L,10101L), "mobicents.org","aaa://uas.fancyapp.mobicents.org"); //add avps specific for app session.send(r,this); } }</programlisting> </example> <example> <title>SessionFactory use example</title> <programlisting language="Java" role="JAVA">class Test implements EventListener<Request, Answer> { Stack stack = new StackImpl(); XMLConfiguration config = new XMLConfiguration(new FileInputStream(new File(configFile)); ISessionFactory sessionFactory = (ISessionFactory)stack.init(config); stack.start(); //perferctly legal, both factories are the same. sessionFactor = (ISessionFactory)stack.getSessionFactory(); sessionFactory.registerAppFacory(ClientShSession.class, new ShClientSessionFactory(this)); //our implementation of factory does not require any parameters ClientShSession session = (ClientShSession) sessionFactory.getNewAppSession(null, null , ClientShSession.class, null); ... session.sendUserDataRequest(udr); }</programlisting> </example> </section> <section id="jdiameter-source_overview-session"> <title>Sessions</title> <para><classname>RawSessions</classname>, <classname>Sessions</classname> and <classname>ApplicationSessions</classname> provide the means for dispatching and receiving messages. Specific implementation of <classname>ApplicationSession</classname> may provide non standard methods.</para> <para>The <classname>RawSession</classname> and the <classname>Session</classname> life span is controlled entirely by the application. However, the <classname>ApplicationSession</classname> life time depends on the implemented state machine.</para> <para><classname>RawSession</classname> is defined as follows:</para> <programlisting role="JAVA" language="Java">public interface BaseSession extends Wrapper, Serializable { long getCreationTime(); long getLastAccessedTime(); boolean isValid(); Future<Message> send(Message message) throws InternalException, IllegalDiameterStateException, RouteException, OverloadException; Future<Message> send(Message message, long timeOut, TimeUnit timeUnit) throws InternalException, IllegalDiameterStateException, RouteException, OverloadException; void release(); } public interface RawSession extends BaseSession { Message createMessage(int commandCode, ApplicationId applicationId, Avp... avp); Message createMessage(int commandCode, ApplicationId applicationId, long hopByHopIdentifier, long endToEndIdentifier, Avp... avp); Message createMessage(Message message, boolean copyAvps); void send(Message message, EventListener<Message, Message> listener) throws InternalException, IllegalDiameterStateException, RouteException, OverloadException; void send(Message message, EventListener<Message, Message> listener, long timeOut, TimeUnit timeUnit) throws InternalException, IllegalDiameterStateException, RouteException, OverloadException; }</programlisting> <variablelist> <varlistentry> <term><methodname>long getCreationTime();</methodname></term> <listitem><para>Returns the time stamp of this session creation.</para></listitem> </varlistentry> <varlistentry> <term><methodname>long getLastAccessedTime();</methodname></term> <listitem><para>Returns the time stamp indicating the last sent or received operation.</para></listitem> </varlistentry> <varlistentry> <term><methodname>boolean isValid();</methodname></term> <listitem><para>Returns <literal>true</literal> when this session is still valid (ie, <methodname>release()</methodname> has not been called).</para></listitem> </varlistentry> <varlistentry> <term><methodname>void release();</methodname></term> <listitem><para>Application calls this method to inform the user that the session should free any associated resource - it shall not be used anymore.</para></listitem> </varlistentry> <varlistentry> <term><methodname>Future<Message> send(Message message)</methodname></term> <listitem><para>Sends a message in async mode. The <classname>Future</classname> reference provides the means of accessing the answer once it is received</para></listitem> </varlistentry> <varlistentry> <term><methodname>void send(Message message, EventListener<Message, Message> listener, long timeOut, TimeUnit timeUnit)</methodname></term> <listitem><para>As above. Allows to specify the time out value for send operations.</para></listitem> </varlistentry> <varlistentry> <term><methodname>Message createMessage(int commandCode, ApplicationId applicationId, Avp... avp);</methodname></term> <listitem><para>Creates a Diameter message. It should be explicitly set either as a request or answer. Passed parameters are used to build messages.</para></listitem> </varlistentry> <varlistentry> <term><methodname>Message createMessage(int commandCode, ApplicationId applicationId, long hopByHopIdentifier, long endToEndIdentifier, Avp... avp);</methodname></term> <listitem><para>As above. However, it also allows for the Hop-by-Hop and End-to-End Identifiers in the message header to be set. This method should be used to create answers.</para></listitem> </varlistentry> <varlistentry> <term><methodname>Message createMessage(Message message, boolean copyAvps);</methodname></term> <listitem><para>Clones a message and returns the created object. The copyAvps parameter defines whether basic AVPs (Session, Route and Proxy information) should be copied to the new object.</para></listitem> </varlistentry> <varlistentry> <term><methodname>void send(Message message, EventListener<Message, Message> listener)</methodname></term> <listitem><para>Sends a message. The answer will be delivered by the specified listener</para></listitem> </varlistentry> <varlistentry> <term><methodname>void send(Message message, EventListener<Message, Message> listener, long timeOut, TimeUnit timeUnit)</methodname></term> <listitem><para>As above. It also allows for the answer to be passed after timeout.</para></listitem> </varlistentry> </variablelist> <para><classname>Session</classname> defines similar methods, with exactly the same purpose:</para> <programlisting role="JAVA" language="Java">public interface Session extends BaseSession { String getSessionId(); void setRequestListener(NetworkReqListener listener); Request createRequest(int commandCode, ApplicationId appId, String destRealm); Request createRequest(int commandCode, ApplicationId appId, String destRealm, String destHost); Request createRequest(Request prevRequest); void send(Message message, EventListener<Request, Answer> listener) throws InternalException, IllegalDiameterStateException, RouteException, OverloadException; void send(Message message, EventListener<Request, Answer> listener, long timeOut, TimeUnit timeUnit) throws InternalException, IllegalDiameterStateException, RouteException, OverloadException; }</programlisting> </section> <section> <title>Application Session Factories</title> <para>In the table below, you can find session factories provided by current implementation, along with a short description:</para> <table frame="all" pgwide="1" id="table-app_session_factories"> <title>Application Factories</title> <tgroup cols="4" colsep="1"> <colspec colname="c1"/> <colspec colname="c2"/> <colspec colname="c3"/> <colspec colname="c4"/> <thead> <row> <entry>Factory class</entry> <entry>Application type & id</entry> <entry>Application</entry> <entry>Reference</entry> </row> </thead> <tbody> <row> <entry>org.jdiameter.common .impl.app.acc. AccSessionFactoryImpl</entry> <entry>AccountingId[0:3]</entry> <entry>Acc</entry> <entry><ulink url="http://tools.ietf.org/html/rfc3588">RFC3588</ulink> </entry> </row> <row> <entry>org.jdiameter.common .impl.app.auth. AuthSessionFactoryImpl</entry> <entry>Specific</entry> <entry>Auth</entry> <entry><ulink url="http://tools.ietf.org/html/rfc3588">RFC3588</ulink></entry> </row> <row> <entry>org.jdiameter.common .impl.app.cca. CCASessionFactoryImpl</entry> <entry>AuthId[0:4]</entry> <entry>CCA</entry> <entry><ulink url="http://tools.ietf.org/html/rfc4006">RFC4006</ulink></entry> </row> <row> <entry>org.jdiameter.common .impl.app.sh. ShSessionFactoryImpl</entry> <entry>AuthId[10415:16777217]</entry> <entry>Sh</entry> <entry><ulink url="http://www.3gpp.org/ftp/Specs/html-info/29328.htm">TS.29328</ulink>, <ulink url="http://www.3gpp.org/ftp/Specs/html-info/29329.htm">TS.29329</ulink></entry> </row> <row> <entry>org.jdiameter.common .impl.app.cxdx. CxDxSessionFactoryImpl</entry> <entry>AuthId[13019:16777216]</entry> <entry>Cx</entry> <entry><ulink url="http://www.3gpp.org/ftp/Specs/html-info/29228.htm">TS.29228</ulink>, <ulink url="http://www.3gpp.org/ftp/Specs/html-info/29229.htm">TS.29229</ulink></entry> </row> <row> <entry>org.jdiameter.common .impl.app.cxdx. CxDxSessionFactoryImpl</entry> <entry>AuthId[10415:16777216]</entry> <entry>Dx</entry> <entry><ulink url="http://www.3gpp.org/ftp/Specs/html-info/29228.htm">TS.29228</ulink>, <ulink url="http://www.3gpp.org/ftp/Specs/html-info/29229.htm">TS.29229</ulink></entry> </row> <row> <entry>org.jdiameter.common .impl.app.acc. AccSessionFactoryImpl</entry> <entry>AccountingId[10415:3]</entry> <entry>Rf</entry> <entry><ulink url="http://www.3gpp.org/ftp/Specs/html-info/32240.htm">TS.32240</ulink></entry> </row> <row> <entry>org.jdiameter.common .impl.app.cca. CCASessionFactoryImpl</entry> <entry>AuthId[10415:4]</entry> <entry>Ro</entry> <entry><ulink url="http://www.3gpp.org/ftp/Specs/html-info/32240.htm">TS.32240</ulink></entry> </row> </tbody> </tgroup> </table> <note> <para>There is no specific factory for Ro and Rf. Those applications reuse the respective session and session factories.</para> </note> <note> <para>Application IDs contain two numbers - [VendorId:ApplicationId].</para> </note> <important> <para>Spaces have been introduced in the <literal>Factory class</literal> column values to correctly render the table. Please remove them when using copy/paste.</para> </important> </section> </section>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy