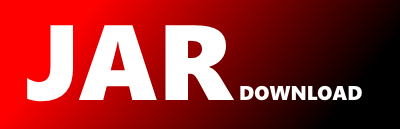
org.oma.protocols.mlp.svc_init.Msids Maven / Gradle / Ivy
package org.oma.protocols.mlp.svc_init;
import java.util.ArrayList;
import java.util.List;
/**
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="MLP_SVC_INIT_310.dtd" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="msids">
* <xs:complexType>
* <xs:sequence>
* <xs:choice maxOccurs="unbounded">
* <!-- Reference to inner class Choice -->
* </xs:choice>
* </xs:sequence>
* </xs:complexType>
* </xs:element>
*
*/
public class Msids
{
private List choiceList = new ArrayList();
/**
* Get the list of 'msids' element items.
*
* @return list
*/
public List getChoiceList() {
return choiceList;
}
/**
* Set the list of 'msids' element items.
*
* @param list
*/
public void setChoiceList(List list) {
choiceList = list;
}
/**
* Schema fragment(s) for this class:
*
* <xs:choice xmlns:ns="MLP_SVC_INIT_310.dtd" xmlns:xs="http://www.w3.org/2001/XMLSchema" maxOccurs="unbounded">
* <xs:sequence>
* <xs:element ref="ns:msid"/>
* <xs:element ref="ns:codeword" minOccurs="0"/>
* <xs:element ref="ns:session" minOccurs="0"/>
* </xs:sequence>
* <xs:sequence>
* <xs:element ref="ns:msid_range"/>
* <xs:element ref="ns:codeword" minOccurs="0" maxOccurs="unbounded"/>
* </xs:sequence>
* </xs:choice>
*
*/
public static class Choice
{
private int choiceListSelect = -1;
private static final int MSID_CHOICE = 0;
private static final int MSID_RANGE_CHOICE = 1;
private Msid msid;
private Codeword codeword;
private Session session;
private MsidRange msidRange;
private List codewordList = new ArrayList();
private void setChoiceListSelect(int choice) {
if (choiceListSelect == -1) {
choiceListSelect = choice;
} else if (choiceListSelect != choice) {
throw new IllegalStateException(
"Need to call clearChoiceListSelect() before changing existing choice");
}
}
/**
* Clear the choice selection.
*/
public void clearChoiceListSelect() {
choiceListSelect = -1;
}
/**
* Check if Msid is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifMsid() {
return choiceListSelect == MSID_CHOICE;
}
/**
* Get the 'msid' element value.
*
* @return value
*/
public Msid getMsid() {
return msid;
}
/**
* Set the 'msid' element value.
*
* @param msid
*/
public void setMsid(Msid msid) {
setChoiceListSelect(MSID_CHOICE);
this.msid = msid;
}
/**
* Get the 'codeword' element value.
*
* @return value
*/
public Codeword getCodeword() {
return codeword;
}
/**
* Set the 'codeword' element value.
*
* @param codeword
*/
public void setCodeword(Codeword codeword) {
setChoiceListSelect(MSID_CHOICE);
this.codeword = codeword;
}
/**
* Get the 'session' element value.
*
* @return value
*/
public Session getSession() {
return session;
}
/**
* Set the 'session' element value.
*
* @param session
*/
public void setSession(Session session) {
setChoiceListSelect(MSID_CHOICE);
this.session = session;
}
/**
* Check if MsidRange is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifMsidRange() {
return choiceListSelect == MSID_RANGE_CHOICE;
}
/**
* Get the 'msid_range' element value.
*
* @return value
*/
public MsidRange getMsidRange() {
return msidRange;
}
/**
* Set the 'msid_range' element value.
*
* @param msidRange
*/
public void setMsidRange(MsidRange msidRange) {
setChoiceListSelect(MSID_RANGE_CHOICE);
this.msidRange = msidRange;
}
/**
* Get the list of 'codeword' element items.
*
* @return list
*/
public List getCodewordList() {
return codewordList;
}
/**
* Set the list of 'codeword' element items.
*
* @param list
*/
public void setCodewordList(List list) {
setChoiceListSelect(MSID_RANGE_CHOICE);
codewordList = list;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy