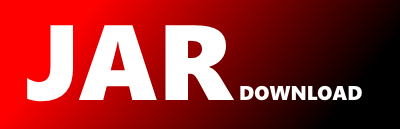
org.oma.protocols.mlp.svc_init.Slir Maven / Gradle / Ivy
package org.oma.protocols.mlp.svc_init;
import java.util.ArrayList;
import java.util.List;
/**
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="MLP_SVC_INIT_310.dtd" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="slir">
* <xs:complexType>
* <xs:sequence>
* <xs:choice>
* <xs:element ref="ns:msids"/>
* <xs:sequence maxOccurs="unbounded">
* <!-- Reference to inner class Sequence -->
* </xs:sequence>
* </xs:choice>
* <xs:element ref="ns:eqop" minOccurs="0"/>
* <xs:element ref="ns:geo_info" minOccurs="0"/>
* <xs:element ref="ns:loc_type" minOccurs="0"/>
* <xs:element ref="ns:prio" minOccurs="0"/>
* <xs:element ref="ns:pushaddr" minOccurs="0"/>
* </xs:sequence>
* <xs:attribute type="xs:string" fixed="3.0.0" name="ver"/>
* <xs:attribute default="SYNC" name="res_type">
* <xs:simpleType>
* <!-- Reference to inner class ResType -->
* </xs:simpleType>
* </xs:attribute>
* </xs:complexType>
* </xs:element>
*
*/
public class Slir
{
private int choiceSelect = -1;
private static final int MSIDS_CHOICE = 0;
private static final int SEQUENCE_LIST_CHOICE = 1;
private Msids msids;
private List sequenceList = new ArrayList();
private Eqop eqop;
private GeoInfo geoInfo;
private LocType locType;
private Prio prio;
private Pushaddr pushaddr;
private String ver;
private ResType resType;
private void setChoiceSelect(int choice) {
if (choiceSelect == -1) {
choiceSelect = choice;
} else if (choiceSelect != choice) {
throw new IllegalStateException(
"Need to call clearChoiceSelect() before changing existing choice");
}
}
/**
* Clear the choice selection.
*/
public void clearChoiceSelect() {
choiceSelect = -1;
}
/**
* Check if Msids is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifMsids() {
return choiceSelect == MSIDS_CHOICE;
}
/**
* Get the 'msids' element value.
*
* @return value
*/
public Msids getMsids() {
return msids;
}
/**
* Set the 'msids' element value.
*
* @param msids
*/
public void setMsids(Msids msids) {
setChoiceSelect(MSIDS_CHOICE);
this.msids = msids;
}
/**
* Check if SequenceList is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifSequenceList() {
return choiceSelect == SEQUENCE_LIST_CHOICE;
}
/**
* Get the list of sequence items.
*
* @return list
*/
public List getSequenceList() {
return sequenceList;
}
/**
* Set the list of sequence items.
*
* @param list
*/
public void setSequenceList(List list) {
setChoiceSelect(SEQUENCE_LIST_CHOICE);
sequenceList = list;
}
/**
* Get the 'eqop' element value.
*
* @return value
*/
public Eqop getEqop() {
return eqop;
}
/**
* Set the 'eqop' element value.
*
* @param eqop
*/
public void setEqop(Eqop eqop) {
this.eqop = eqop;
}
/**
* Get the 'geo_info' element value.
*
* @return value
*/
public GeoInfo getGeoInfo() {
return geoInfo;
}
/**
* Set the 'geo_info' element value.
*
* @param geoInfo
*/
public void setGeoInfo(GeoInfo geoInfo) {
this.geoInfo = geoInfo;
}
/**
* Get the 'loc_type' element value.
*
* @return value
*/
public LocType getLocType() {
return locType;
}
/**
* Set the 'loc_type' element value.
*
* @param locType
*/
public void setLocType(LocType locType) {
this.locType = locType;
}
/**
* Get the 'prio' element value.
*
* @return value
*/
public Prio getPrio() {
return prio;
}
/**
* Set the 'prio' element value.
*
* @param prio
*/
public void setPrio(Prio prio) {
this.prio = prio;
}
/**
* Get the 'pushaddr' element value.
*
* @return value
*/
public Pushaddr getPushaddr() {
return pushaddr;
}
/**
* Set the 'pushaddr' element value.
*
* @param pushaddr
*/
public void setPushaddr(Pushaddr pushaddr) {
this.pushaddr = pushaddr;
}
/**
* Get the 'ver' attribute value.
*
* @return value
*/
public String getVer() {
return ver;
}
/**
* Set the 'ver' attribute value.
*
* @param ver
*/
public void setVer(String ver) {
this.ver = ver;
}
/**
* Get the 'res_type' attribute value.
*
* @return value
*/
public ResType getResType() {
return resType;
}
/**
* Set the 'res_type' attribute value.
*
* @param resType
*/
public void setResType(ResType resType) {
this.resType = resType;
}
/**
* Schema fragment(s) for this class:
*
* <xs:sequence xmlns:ns="MLP_SVC_INIT_310.dtd" xmlns:xs="http://www.w3.org/2001/XMLSchema" maxOccurs="unbounded">
* <xs:element ref="ns:msid"/>
* <xs:element ref="ns:codeword" minOccurs="0"/>
* <xs:element ref="ns:gsm_net_param"/>
* </xs:sequence>
*
*/
public static class Sequence
{
private Msid msid;
private Codeword codeword;
private GsmNetParam gsmNetParam;
/**
* Get the 'msid' element value.
*
* @return value
*/
public Msid getMsid() {
return msid;
}
/**
* Set the 'msid' element value.
*
* @param msid
*/
public void setMsid(Msid msid) {
this.msid = msid;
}
/**
* Get the 'codeword' element value.
*
* @return value
*/
public Codeword getCodeword() {
return codeword;
}
/**
* Set the 'codeword' element value.
*
* @param codeword
*/
public void setCodeword(Codeword codeword) {
this.codeword = codeword;
}
/**
* Get the 'gsm_net_param' element value.
*
* @return value
*/
public GsmNetParam getGsmNetParam() {
return gsmNetParam;
}
/**
* Set the 'gsm_net_param' element value.
*
* @param gsmNetParam
*/
public void setGsmNetParam(GsmNetParam gsmNetParam) {
this.gsmNetParam = gsmNetParam;
}
}
/**
* Schema fragment(s) for this class:
*
* <xs:simpleType xmlns:xs="http://www.w3.org/2001/XMLSchema">
* <xs:restriction base="xs:string">
* <xs:enumeration value="SYNC"/>
* <xs:enumeration value="ASYNC"/>
* </xs:restriction>
* </xs:simpleType>
*
*/
public static enum ResType {
SYNC, ASYNC
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy