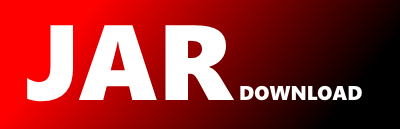
org.oma.protocols.mlp.svc_result.CircularArcArea Maven / Gradle / Ivy
package org.oma.protocols.mlp.svc_result;
/**
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="MLP_SVC_RESULT_310.dtd" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="CircularArcArea">
* <xs:complexType>
* <xs:sequence>
* <xs:element ref="ns:coord"/>
* <xs:element ref="ns:inRadius"/>
* <xs:element ref="ns:outRadius"/>
* <xs:element ref="ns:startAngle"/>
* <xs:element ref="ns:stopAngle"/>
* <xs:element ref="ns:angularUnit" minOccurs="0"/>
* <xs:element ref="ns:distanceUnit" minOccurs="0"/>
* </xs:sequence>
* <xs:attribute type="xs:string" name="gid"/>
* <xs:attribute type="xs:string" name="srsName"/>
* </xs:complexType>
* </xs:element>
*
*/
public class CircularArcArea
{
private Coord coord;
private InRadius inRadius;
private OutRadius outRadius;
private StartAngle startAngle;
private StopAngle stopAngle;
private AngularUnit angularUnit;
private DistanceUnit distanceUnit;
private String gid;
private String srsName;
/**
* Get the 'coord' element value.
*
* @return value
*/
public Coord getCoord() {
return coord;
}
/**
* Set the 'coord' element value.
*
* @param coord
*/
public void setCoord(Coord coord) {
this.coord = coord;
}
/**
* Get the 'inRadius' element value.
*
* @return value
*/
public InRadius getInRadius() {
return inRadius;
}
/**
* Set the 'inRadius' element value.
*
* @param inRadius
*/
public void setInRadius(InRadius inRadius) {
this.inRadius = inRadius;
}
/**
* Get the 'outRadius' element value.
*
* @return value
*/
public OutRadius getOutRadius() {
return outRadius;
}
/**
* Set the 'outRadius' element value.
*
* @param outRadius
*/
public void setOutRadius(OutRadius outRadius) {
this.outRadius = outRadius;
}
/**
* Get the 'startAngle' element value.
*
* @return value
*/
public StartAngle getStartAngle() {
return startAngle;
}
/**
* Set the 'startAngle' element value.
*
* @param startAngle
*/
public void setStartAngle(StartAngle startAngle) {
this.startAngle = startAngle;
}
/**
* Get the 'stopAngle' element value.
*
* @return value
*/
public StopAngle getStopAngle() {
return stopAngle;
}
/**
* Set the 'stopAngle' element value.
*
* @param stopAngle
*/
public void setStopAngle(StopAngle stopAngle) {
this.stopAngle = stopAngle;
}
/**
* Get the 'angularUnit' element value.
*
* @return value
*/
public AngularUnit getAngularUnit() {
return angularUnit;
}
/**
* Set the 'angularUnit' element value.
*
* @param angularUnit
*/
public void setAngularUnit(AngularUnit angularUnit) {
this.angularUnit = angularUnit;
}
/**
* Get the 'distanceUnit' element value.
*
* @return value
*/
public DistanceUnit getDistanceUnit() {
return distanceUnit;
}
/**
* Set the 'distanceUnit' element value.
*
* @param distanceUnit
*/
public void setDistanceUnit(DistanceUnit distanceUnit) {
this.distanceUnit = distanceUnit;
}
/**
* Get the 'gid' attribute value.
*
* @return value
*/
public String getGid() {
return gid;
}
/**
* Set the 'gid' attribute value.
*
* @param gid
*/
public void setGid(String gid) {
this.gid = gid;
}
/**
* Get the 'srsName' attribute value.
*
* @return value
*/
public String getSrsName() {
return srsName;
}
/**
* Set the 'srsName' attribute value.
*
* @param srsName
*/
public void setSrsName(String srsName) {
this.srsName = srsName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy