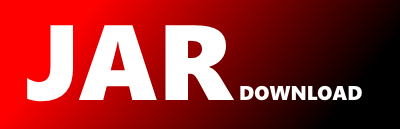
org.oma.protocols.mlp.svc_result.Shape Maven / Gradle / Ivy
package org.oma.protocols.mlp.svc_result;
/**
* Schema fragment(s) for this class:
*
* <xs:element xmlns:ns="MLP_SVC_RESULT_310.dtd" xmlns:xs="http://www.w3.org/2001/XMLSchema" name="shape">
* <xs:complexType>
* <xs:choice>
* <xs:element ref="ns:Point"/>
* <xs:element ref="ns:LineString"/>
* <xs:element ref="ns:Polygon"/>
* <xs:element ref="ns:Box"/>
* <xs:element ref="ns:CircularArea"/>
* <xs:element ref="ns:CircularArcArea"/>
* <xs:element ref="ns:EllipticalArea"/>
* <xs:element ref="ns:MultiLineString"/>
* <xs:element ref="ns:MultiPoint"/>
* <xs:element ref="ns:MultiPolygon"/>
* </xs:choice>
* </xs:complexType>
* </xs:element>
*
*/
public class Shape
{
private int shapeSelect = -1;
private static final int POINT_CHOICE = 0;
private static final int LINE_STRING_CHOICE = 1;
private static final int POLYGON_CHOICE = 2;
private static final int BOX_CHOICE = 3;
private static final int CIRCULAR_AREA_CHOICE = 4;
private static final int CIRCULAR_ARC_AREA_CHOICE = 5;
private static final int ELLIPTICAL_AREA_CHOICE = 6;
private static final int MULTI_LINE_STRING_CHOICE = 7;
private static final int MULTI_POINT_CHOICE = 8;
private static final int MULTI_POLYGON_CHOICE = 9;
private Point point;
private LineString lineString;
private Polygon polygon;
private Box box;
private CircularArea circularArea;
private CircularArcArea circularArcArea;
private EllipticalArea ellipticalArea;
private MultiLineString multiLineString;
private MultiPoint multiPoint;
private MultiPolygon multiPolygon;
private void setShapeSelect(int choice) {
if (shapeSelect == -1) {
shapeSelect = choice;
} else if (shapeSelect != choice) {
throw new IllegalStateException(
"Need to call clearShapeSelect() before changing existing choice");
}
}
/**
* Clear the choice selection.
*/
public void clearShapeSelect() {
shapeSelect = -1;
}
/**
* Check if Point is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifPoint() {
return shapeSelect == POINT_CHOICE;
}
/**
* Get the 'Point' element value.
*
* @return value
*/
public Point getPoint() {
return point;
}
/**
* Set the 'Point' element value.
*
* @param point
*/
public void setPoint(Point point) {
setShapeSelect(POINT_CHOICE);
this.point = point;
}
/**
* Check if LineString is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifLineString() {
return shapeSelect == LINE_STRING_CHOICE;
}
/**
* Get the 'LineString' element value.
*
* @return value
*/
public LineString getLineString() {
return lineString;
}
/**
* Set the 'LineString' element value.
*
* @param lineString
*/
public void setLineString(LineString lineString) {
setShapeSelect(LINE_STRING_CHOICE);
this.lineString = lineString;
}
/**
* Check if Polygon is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifPolygon() {
return shapeSelect == POLYGON_CHOICE;
}
/**
* Get the 'Polygon' element value.
*
* @return value
*/
public Polygon getPolygon() {
return polygon;
}
/**
* Set the 'Polygon' element value.
*
* @param polygon
*/
public void setPolygon(Polygon polygon) {
setShapeSelect(POLYGON_CHOICE);
this.polygon = polygon;
}
/**
* Check if Box is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifBox() {
return shapeSelect == BOX_CHOICE;
}
/**
* Get the 'Box' element value.
*
* @return value
*/
public Box getBox() {
return box;
}
/**
* Set the 'Box' element value.
*
* @param box
*/
public void setBox(Box box) {
setShapeSelect(BOX_CHOICE);
this.box = box;
}
/**
* Check if CircularArea is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifCircularArea() {
return shapeSelect == CIRCULAR_AREA_CHOICE;
}
/**
* Get the 'CircularArea' element value.
*
* @return value
*/
public CircularArea getCircularArea() {
return circularArea;
}
/**
* Set the 'CircularArea' element value.
*
* @param circularArea
*/
public void setCircularArea(CircularArea circularArea) {
setShapeSelect(CIRCULAR_AREA_CHOICE);
this.circularArea = circularArea;
}
/**
* Check if CircularArcArea is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifCircularArcArea() {
return shapeSelect == CIRCULAR_ARC_AREA_CHOICE;
}
/**
* Get the 'CircularArcArea' element value.
*
* @return value
*/
public CircularArcArea getCircularArcArea() {
return circularArcArea;
}
/**
* Set the 'CircularArcArea' element value.
*
* @param circularArcArea
*/
public void setCircularArcArea(CircularArcArea circularArcArea) {
setShapeSelect(CIRCULAR_ARC_AREA_CHOICE);
this.circularArcArea = circularArcArea;
}
/**
* Check if EllipticalArea is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifEllipticalArea() {
return shapeSelect == ELLIPTICAL_AREA_CHOICE;
}
/**
* Get the 'EllipticalArea' element value.
*
* @return value
*/
public EllipticalArea getEllipticalArea() {
return ellipticalArea;
}
/**
* Set the 'EllipticalArea' element value.
*
* @param ellipticalArea
*/
public void setEllipticalArea(EllipticalArea ellipticalArea) {
setShapeSelect(ELLIPTICAL_AREA_CHOICE);
this.ellipticalArea = ellipticalArea;
}
/**
* Check if MultiLineString is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifMultiLineString() {
return shapeSelect == MULTI_LINE_STRING_CHOICE;
}
/**
* Get the 'MultiLineString' element value.
*
* @return value
*/
public MultiLineString getMultiLineString() {
return multiLineString;
}
/**
* Set the 'MultiLineString' element value.
*
* @param multiLineString
*/
public void setMultiLineString(MultiLineString multiLineString) {
setShapeSelect(MULTI_LINE_STRING_CHOICE);
this.multiLineString = multiLineString;
}
/**
* Check if MultiPoint is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifMultiPoint() {
return shapeSelect == MULTI_POINT_CHOICE;
}
/**
* Get the 'MultiPoint' element value.
*
* @return value
*/
public MultiPoint getMultiPoint() {
return multiPoint;
}
/**
* Set the 'MultiPoint' element value.
*
* @param multiPoint
*/
public void setMultiPoint(MultiPoint multiPoint) {
setShapeSelect(MULTI_POINT_CHOICE);
this.multiPoint = multiPoint;
}
/**
* Check if MultiPolygon is current selection for choice.
*
* @return true
if selection, false
if not
*/
public boolean ifMultiPolygon() {
return shapeSelect == MULTI_POLYGON_CHOICE;
}
/**
* Get the 'MultiPolygon' element value.
*
* @return value
*/
public MultiPolygon getMultiPolygon() {
return multiPolygon;
}
/**
* Set the 'MultiPolygon' element value.
*
* @param multiPolygon
*/
public void setMultiPolygon(MultiPolygon multiPolygon) {
setShapeSelect(MULTI_POLYGON_CHOICE);
this.multiPolygon = multiPolygon;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy