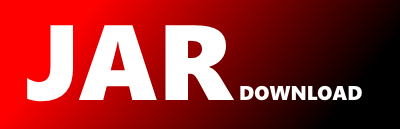
org.mobicents.servlet.sip.address.ParameterableImpl Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright 2011, Red Hat, Inc. and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.mobicents.servlet.sip.address;
import gov.nist.javax.sip.header.ParametersExt;
import java.io.Serializable;
import java.text.ParseException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Map.Entry;
import java.util.concurrent.ConcurrentHashMap;
import javax.servlet.sip.Parameterable;
import javax.sip.header.Header;
import javax.sip.header.Parameters;
import gov.nist.javax.sip.header.ParametersExt;
import org.mobicents.servlet.sip.address.AddressImpl.ModifiableRule;
import org.mobicents.servlet.sip.core.SipContext;
import org.mobicents.servlet.sip.core.session.SipApplicationSessionCreationThreadLocal;
/**
* Implementation of the parameterable interface.
*
* @author mranga
* @author [email protected]
*
*/
public abstract class ParameterableImpl implements Parameterable ,Cloneable, Serializable {
private static final long serialVersionUID = 1L;
// private static Logger logger = Logger.getLogger(ParameterableImpl.class.getCanonicalName());
private static final String PARAM_SEPARATOR = ";";
private static final String PARAM_NAME_VALUE_SEPARATOR = "=";
protected Map parameters = new ConcurrentHashMap();
protected transient Parameters header = null;
protected ModifiableRule isModifiable = ModifiableRule.Modifiable;
protected ParameterableImpl() {
this.parameters = new ConcurrentHashMap();
}
/**
* Create parametrable instance.
* @param value - initial value of parametrable value
* @param parameters - parameter map - it can be null;
*/
public ParameterableImpl(Header header, Map params, ModifiableRule isModifiable) {
this.isModifiable = isModifiable;
if(header instanceof Parameters) {
this.header = (Parameters) header;
}
if(params!=null) {
Iterator> entries=params.entrySet().iterator();
while (entries.hasNext()) {
Map.Entry e=entries.next();
parameters.put(e.getKey(), e.getValue());
}
}
}
/*
* (non-Javadoc)
* @see javax.servlet.sip.Parameterable#getParameter(java.lang.String)
*/
public String getParameter(String name) {
if(name == null) {
throw new NullPointerException("the parameter given in parameter is null !");
}
String value = this.parameters.get(name);
if(value != null) {
return RFC2396UrlDecoder.decode(value);
} else {
if("lr".equals(name)) return "";// special case to pass Addressing spec test from 289 TCK
}
return null;
}
/*
* (non-Javadoc)
* @see javax.servlet.sip.Parameterable#getParameterNames()
*/
public Iterator getParameterNames() {
return this.parameters.keySet().iterator();
}
/*
* (non-Javadoc)
* @see javax.servlet.sip.Parameterable#removeParameter(java.lang.String)
*/
public void removeParameter(String name) {
if(name == null) {
throw new NullPointerException("parameter name is null ! ");
}
if(isModifiable == ModifiableRule.NotModifiable) {
throw new IllegalStateException("it is forbidden to modify the parameters");
}
if(name.equalsIgnoreCase("tag") && (isModifiable == ModifiableRule.From || isModifiable == ModifiableRule.To)) {
throw new IllegalStateException("it is forbidden to remove the tag parameter on To or From Header");
}
if(name.equalsIgnoreCase("branch") && isModifiable == ModifiableRule.Via) {
throw new IllegalStateException("it is forbidden to set the branch parameter on the Via Header");
}
this.parameters.remove(name);
if(header != null) {
header.removeParameter(name);
}
}
/*
* (non-Javadoc)
* @see javax.servlet.sip.Parameterable#setParameter(java.lang.String, java.lang.String)
*/
public void setParameter(String name, String value) {
// Global Fix by alexvinn for Issue 1010 : Unable to set flag parameter to parameterable header
if(name == null) {
throw new NullPointerException("parameter name is null ! ");
}
if(value == null) {
removeParameter(name);
return;
}
if(isModifiable == ModifiableRule.NotModifiable) {
throw new IllegalStateException("it is forbidden to modify the parameters");
}
if(name.equalsIgnoreCase("tag") && (isModifiable == ModifiableRule.From || isModifiable == ModifiableRule.To)) {
throw new IllegalStateException("it is forbidden to set the tag parameter on To or From Header");
}
if(name.equalsIgnoreCase("branch") && isModifiable == ModifiableRule.Via) {
throw new IllegalStateException("it is forbidden to set the branch parameter on the Via Header");
}
//Fix from abondar for Issue 494 and angelo.marletta for Issue 502
this.parameters.put(name.toLowerCase(), value);
if(header != null) {
try {
if (isQuotableParam(name) && (header instanceof ParametersExt)){
((ParametersExt) header).setQuotedParameter(name, value);
}else{
header.setParameter(name, "".equals(value) ? null : value);
}
} catch (ParseException e) {
throw new IllegalArgumentException("Problem setting parameter",e);
}
}
}
/*
* Check if the param name is in the list that its value need to be quoted
*
* @param name - a string specifying the parameter name
*
*/
private boolean isQuotableParam(String name){
boolean isQuotableParameter = false;
SipContext context = SipApplicationSessionCreationThreadLocal.lookupContext();
if (context != null){
List params = (List)context.getServletContext().getAttribute("org.restcomm.servlets.sip.QUOTABLE_PARAMETER");
for (String param : params){
if (param.equalsIgnoreCase(name)){
isQuotableParameter = true;
break;
}
}
}
return isQuotableParameter;
}
/*
* (non-Javadoc)
* @see javax.servlet.sip.Parameterable#getParameters()
*/
public Set> getParameters() {
Map retval = new HashMap ();
for(Entry nameValue : this.parameters.entrySet()) {
retval.put(nameValue.getKey(), (RFC2396UrlDecoder.decode(nameValue.getValue())));
}
return retval.entrySet();
}
/*
* (non-Javadoc)
* @see javax.servlet.sip.Parameterable#getParameters()
*/
public Map getInternalParameters() {
return parameters;
}
/*
* (non-Javadoc)
* @see java.lang.Object#toString()
*/
public String toString() {
StringBuffer retVal = new StringBuffer();
boolean firstTime = true;
for(java.util.Map.Entry entry : parameters.entrySet()) {
if(!firstTime) {
retVal.append(PARAM_SEPARATOR);
}
firstTime = false;
String value = entry.getValue();
if(value != null && value.length() > 0) {
retVal.append(entry.getKey()).append(PARAM_NAME_VALUE_SEPARATOR).append(value);
} else {
retVal.append(entry.getKey());
}
}
return retVal.toString();
}
public void setParameters(Map parameters) {
this.parameters = parameters;
if(header != null) {
for(Entry nameValue : this.parameters.entrySet()) {
try {
header.setParameter(nameValue.getKey(), nameValue.getValue());
} catch (ParseException e) {
throw new IllegalArgumentException("Problem setting parameter",e);
}
}
}
}
public abstract Object clone();
/* (non-Javadoc)
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((header == null) ? 0 : header.hashCode());
result = prime * result
+ ((parameters == null) ? 0 : parameters.hashCode());
return result;
}
/* (non-Javadoc)
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ParameterableImpl other = (ParameterableImpl) obj;
if (header == null) {
if (other.header != null)
return false;
} else if (!header.equals(other.header))
return false;
if (parameters == null) {
if (other.parameters != null)
return false;
} else if (!parameters.equals(other.parameters))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy