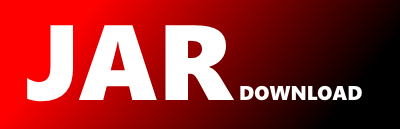
org.mockserver.collections.CaseInsensitiveRegexHashMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockserver-core Show documentation
Show all versions of mockserver-core Show documentation
Functionality used by all MockServer modules for matching and expectations
package org.mockserver.collections;
import org.mockserver.matchers.RegexStringMatcher;
import java.util.*;
/**
* Map that uses case insensitive regex expression matching for keys
*
* @author jamesdbloom
*/
class CaseInsensitiveRegexHashMap extends LinkedHashMap implements Map {
static final long serialVersionUID = 1530623482381786485L;
@Override
public synchronized boolean containsKey(Object key) {
if (key instanceof String) {
if (super.containsKey(key)) {
return true;
} else {
for (String keyToCompare : keySet()) {
if (RegexStringMatcher.matches((String) key, keyToCompare, true)) {
return true;
}
}
}
}
return false;
}
@Override
public synchronized V get(Object key) {
if (key instanceof String) {
if (super.get(key) != null) {
return super.get(key);
} else {
for (String keyToCompare : keySet()) {
if (RegexStringMatcher.matches((String) key, keyToCompare, true)) {
return super.get(keyToCompare);
}
}
}
}
return null;
}
public synchronized Collection getAll(Object key) {
List values = new ArrayList();
if (key instanceof String) {
for (String keyToCompare : keySet()) {
if (RegexStringMatcher.matches((String) key, keyToCompare, true)) {
values.add(super.get(keyToCompare));
}
}
}
return values;
}
@Override
public synchronized V remove(Object key) {
if (key instanceof String) {
if (super.get(key) != null) {
return super.remove(key);
} else {
for (String keyToCompare : keySet()) {
if (RegexStringMatcher.matches((String) key, keyToCompare, true)) {
return super.remove(keyToCompare);
}
}
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy