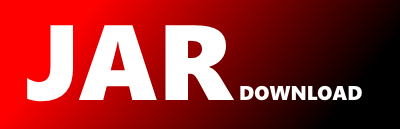
org.mockserver.collections.CircularMultiMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockserver-core Show documentation
Show all versions of mockserver-core Show documentation
Functionality used by all MockServer modules for matching and expectations
package org.mockserver.collections;
import org.mockserver.model.ObjectWithReflectiveEqualsHashCodeToString;
import java.util.*;
/**
* @author jamesdbloom
*/
public class CircularMultiMap implements Map {
private final int maxValuesPerKeySize;
private final CircularHashMap> backingMap;
public CircularMultiMap(int maxNumberOfKeys, int maxNumberOfValuesPerKey) {
this.maxValuesPerKeySize = maxNumberOfValuesPerKey;
backingMap = new CircularHashMap>(maxNumberOfKeys);
}
@Override
public synchronized int size() {
return backingMap.size();
}
@Override
public synchronized boolean isEmpty() {
return backingMap.isEmpty();
}
@Override
public synchronized boolean containsKey(Object key) {
return backingMap.containsKey(key);
}
@Override
public synchronized boolean containsValue(Object value) {
for (Entry> entry : backingMap.entrySet()) {
if (entry.getValue().contains(value)) {
return true;
}
}
return false;
}
@Override
public synchronized V get(Object key) {
List values = backingMap.get(key);
if (values != null && values.size() > 0) {
return values.get(0);
} else {
return null;
}
}
public synchronized List getAll(Object key) {
List values = backingMap.get(key);
if (values == null) {
values = new ArrayList();
}
return values;
}
public synchronized List getKeyListForValues() {
List values = new ArrayList();
for (K key : backingMap.keySet()) {
for (V value : backingMap.get(key)) {
values.add(key);
}
}
return values;
}
@Override
public synchronized V put(K key, V value) {
if (containsKey(key)) {
backingMap.get(key).add(value);
} else {
List list = Collections.synchronizedList(new CircularLinkedList(maxValuesPerKeySize));
list.add(value);
backingMap.put(key, list);
}
return value;
}
@Override
public synchronized V remove(Object key) {
List values = backingMap.get(key);
if (values != null && values.size() > 0) {
return values.remove(0);
} else {
return null;
}
}
public synchronized List removeAll(Object key) {
return backingMap.remove(key);
}
@Override
public synchronized void putAll(Map extends K, ? extends V> map) {
for (Entry extends K, ? extends V> entry : map.entrySet()) {
put(entry.getKey(), entry.getValue());
}
}
@Override
public synchronized void clear() {
backingMap.clear();
}
@Override
public synchronized Set keySet() {
return backingMap.keySet();
}
@Override
public synchronized Collection values() {
Collection values = new ArrayList();
for (List valuesForKey : backingMap.values()) {
values.addAll(valuesForKey);
}
return values;
}
@Override
public synchronized Set> entrySet() {
Set> entrySet = new LinkedHashSet>();
for (Entry> entry : backingMap.entrySet()) {
for (V value : entry.getValue()) {
entrySet.add(new ImmutableEntry(entry.getKey(), value));
}
}
return entrySet;
}
class ImmutableEntry extends ObjectWithReflectiveEqualsHashCodeToString implements Entry {
private final K key;
private final V value;
ImmutableEntry(K key, V value) {
this.key = key;
this.value = value;
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
@Override
public V setValue(V value) {
throw new UnsupportedOperationException("ImmutableEntry is immutable");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy