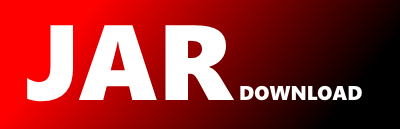
org.mockserver.collections.SubSets Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockserver-core Show documentation
Show all versions of mockserver-core Show documentation
Functionality used by all MockServer modules for matching and expectations
package org.mockserver.collections;
import com.google.common.collect.Multimap;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Deque;
import java.util.List;
public class SubSets {
public static Multimap> distinctSubSetsMap(List input, Multimap> allSubSets, int i) {
return distinctSubSetsMap(input, new ArrayDeque<>(), allSubSets, i);
}
private static Multimap> distinctSubSetsMap(List input, Deque subSet, Multimap> allSubSets, int i) {
// if all elements are processed, print the current subset
if (i < 0) {
allSubSets.put(subSet.size(), new ArrayList<>(subSet));
return allSubSets;
}
// include the current element in the current subset and recur
subSet.addLast(input.get(i));
distinctSubSetsMap(input, subSet, allSubSets, i - 1);
// backtrack: exclude the current element in the current subset
subSet.pollLast();
// remove adjacent duplicate elements
while (i > 0 && input.get(i) == input.get(i - 1)) {
i--;
}
// exclude the current element in the current subset and recurse
distinctSubSetsMap(input, subSet, allSubSets, i - 1);
return allSubSets;
}
public static List> distinctSubSetsList(List input, List> allSubSets, int i) {
return distinctSubSetsList(input, new ArrayDeque<>(), allSubSets, i);
}
private static List> distinctSubSetsList(List input, Deque subSet, List> allSubSets, int i) {
// if all elements are processed, print the current subset
if (i < 0) {
allSubSets.add(new ArrayList<>(subSet));
return allSubSets;
}
// include the current element in the current subset and recur
subSet.addLast(input.get(i));
distinctSubSetsList(input, subSet, allSubSets, i - 1);
// backtrack: exclude the current element in the current subset
subSet.pollLast();
// remove adjacent duplicate elements
while (i > 0 && input.get(i) == input.get(i - 1)) {
i--;
}
// exclude the current element in the current subset and recurse
distinctSubSetsList(input, subSet, allSubSets, i - 1);
return allSubSets;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy