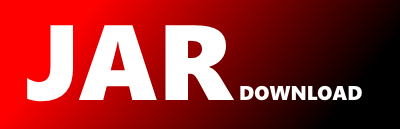
org.mockserver.matchers.JsonPathMatcher Maven / Gradle / Ivy
package org.mockserver.matchers;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.jayway.jsonpath.JsonPath;
import net.minidev.json.JSONArray;
import org.apache.commons.lang3.StringUtils;
import org.mockserver.log.model.LogEntry;
import org.mockserver.logging.MockServerLogger;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
import static org.slf4j.event.Level.DEBUG;
/**
* See https://github.com/json-path/JsonPath
*
* @author jamesdbloom
*/
public class JsonPathMatcher extends BodyMatcher {
private static final String[] EXCLUDED_FIELDS = {"mockServerLogger", "jsonPath"};
private final MockServerLogger mockServerLogger;
private final String matcher;
private JsonPath jsonPath;
JsonPathMatcher(MockServerLogger mockServerLogger, String matcher) {
this.mockServerLogger = mockServerLogger;
this.matcher = matcher;
if (isNotBlank(matcher)) {
try {
jsonPath = JsonPath.compile(matcher);
} catch (Throwable throwable) {
if (MockServerLogger.isEnabled(DEBUG)) {
mockServerLogger.logEvent(
new LogEntry()
.setLogLevel(DEBUG)
.setMessageFormat("error while creating xpath expression for [" + matcher + "] assuming matcher not xpath - " + throwable.getMessage())
.setArguments(throwable)
);
}
}
}
}
public boolean matches(final MatchDifference context, final String matched) {
boolean result = false;
boolean alreadyLoggedMatchFailure = false;
if (jsonPath == null) {
if (context != null) {
context.addDifference(mockServerLogger, "json path match failed expected:{}found:{}failed because:{}", "null", matched, "json path matcher was null");
alreadyLoggedMatchFailure = true;
}
} else if (matcher.equals(matched)) {
result = true;
} else if (matched != null) {
try {
result = !jsonPath.read(matched).isEmpty();
} catch (Throwable throwable) {
if (context != null) {
context.addDifference(mockServerLogger, throwable, "json path match failed expected:{}found:{}failed because:{}", matcher, matched, throwable.getMessage());
alreadyLoggedMatchFailure = true;
}
}
}
if (!result && !alreadyLoggedMatchFailure && context != null) {
context.addDifference(mockServerLogger, "json path match failed expected:{}found:{}failed because:{}", matcher, matched, "json path did not evaluate to truthy");
}
return not != result;
}
public boolean isBlank() {
return StringUtils.isBlank(matcher);
}
@Override
@JsonIgnore
protected String[] fieldsExcludedFromEqualsAndHashCode() {
return EXCLUDED_FIELDS;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy