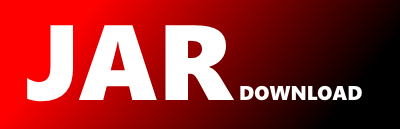
org.mockserver.serialization.VerificationSerializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mockserver-core Show documentation
Show all versions of mockserver-core Show documentation
Functionality used by all MockServer modules for matching and expectations
package org.mockserver.serialization;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.ObjectWriter;
import org.apache.commons.lang3.StringUtils;
import org.mockserver.log.model.LogEntry;
import org.mockserver.logging.MockServerLogger;
import org.mockserver.serialization.model.VerificationDTO;
import org.mockserver.validator.jsonschema.JsonSchemaVerificationValidator;
import org.mockserver.verify.Verification;
import org.slf4j.event.Level;
import static org.apache.commons.lang3.StringUtils.isBlank;
import static org.mockserver.character.Character.NEW_LINE;
import static org.mockserver.formatting.StringFormatter.formatLogMessage;
import static org.mockserver.validator.jsonschema.JsonSchemaValidator.OPEN_API_SPECIFICATION_URL;
import static org.mockserver.validator.jsonschema.JsonSchemaVerificationValidator.jsonSchemaVerificationValidator;
/**
* @author jamesdbloom
*/
@SuppressWarnings("FieldMayBeFinal")
public class VerificationSerializer implements Serializer {
private final MockServerLogger mockServerLogger;
private ObjectWriter objectWriter = ObjectMapperFactory.createObjectMapper(true, false);
private ObjectMapper objectMapper = ObjectMapperFactory.createObjectMapper();
private JsonSchemaVerificationValidator verificationValidator;
public VerificationSerializer(MockServerLogger mockServerLogger) {
this.mockServerLogger = mockServerLogger;
}
private JsonSchemaVerificationValidator getValidator() {
if (verificationValidator == null) {
verificationValidator = jsonSchemaVerificationValidator(mockServerLogger);
}
return verificationValidator;
}
public String serialize(Verification verification) {
try {
return objectWriter.writeValueAsString(new VerificationDTO(verification));
} catch (Exception e) {
mockServerLogger.logEvent(
new LogEntry()
.setLogLevel(Level.ERROR)
.setMessageFormat("exception while serializing verification to JSON with value " + verification)
.setThrowable(e)
);
throw new RuntimeException("Exception while serializing verification to JSON with value " + verification, e);
}
}
public Verification deserialize(String jsonVerification) {
if (isBlank(jsonVerification)) {
throw new IllegalArgumentException(
"1 error:" + NEW_LINE +
" - a verification is required but value was \"" + jsonVerification + "\"" + NEW_LINE +
NEW_LINE +
OPEN_API_SPECIFICATION_URL
);
} else {
String validationErrors = getValidator().isValid(jsonVerification);
if (validationErrors.isEmpty()) {
Verification verification = null;
try {
VerificationDTO verificationDTO = objectMapper.readValue(jsonVerification, VerificationDTO.class);
if (verificationDTO != null) {
verification = verificationDTO.buildObject();
}
} catch (Throwable throwable) {
mockServerLogger.logEvent(
new LogEntry()
.setLogLevel(Level.ERROR)
.setMessageFormat("exception while parsing{}for Verification " + throwable.getMessage())
.setArguments(jsonVerification)
.setThrowable(throwable)
);
throw new IllegalArgumentException("exception while parsing [" + jsonVerification + "] for Verification", throwable);
}
return verification;
} else {
throw new IllegalArgumentException(StringUtils.removeEndIgnoreCase(formatLogMessage("incorrect verification json format for:{}schema validation errors:{}", jsonVerification, validationErrors), "\n"));
}
}
}
@Override
public Class supportsType() {
return Verification.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy