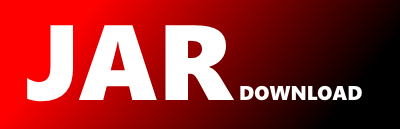
org.mockito.matchers.AnyMatchers.scala Maven / Gradle / Ivy
The newest version!
package org.mockito.matchers
import org.mockito.{ ArgumentMatchers => JavaMatchers }
private[mockito] trait AnyMatchers {
/**
* List matcher that use Scala List to avoid compile errors like Error:(40, 60) type mismatch; found : List[String] (in java.util) required: List[?] (in
* scala.collection.immutable)
*
* when trying to do something like ArgumentMatchers.anyList[String]()
*/
def anyList[T]: List[T] = JavaMatchers.any[List[T]]()
/**
* Seq matcher that use Scala Seq to avoid compile errors like Error:(40, 60) type mismatch; found : List[String] (in java.util) required: Seq[?] (in scala.collection.immutable)
*
* when trying to do something like ArgumentMatchers.anyList[String]()
*/
def anySeq[T]: Seq[T] = JavaMatchers.any[Seq[T]]()
/**
* Iterable matcher that use Scala Iterable to avoid compile errors like Error:(40, 60) type mismatch; found : Iterable[String] (in java.util) required: Iterable[?] (in
* scala.collection.immutable)
*
* when trying to do something like ArgumentMatchers.anyIterable[String]()
*/
def anyIterable[T]: Iterable[T] = JavaMatchers.any[Iterable[T]]()
/**
* Set matcher that use Scala Set to avoid compile errors like Error:(40, 60) type mismatch; found : Set[String] (in java.util) required: Set[?] (in scala.collection.immutable)
*
* when trying to do something like ArgumentMatchers.anySet[String]()
*/
def anySet[T]: Set[T] = JavaMatchers.any[Set[T]]()
/**
* Map matcher that use Scala Map to avoid compile errors like Error:(40, 60) type mismatch; found : Map[String, String] (in java.util) required: Map[?] (in
* scala.collection.immutable)
*
* when trying to do something like ArgumentMatchers.anyMap[String, String]()
*/
def anyMap[K, V]: Map[K, V] = JavaMatchers.any[Map[K, V]]()
/**
* Delegates to ArgumentMatchers.anyByte()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyByte: Byte = JavaMatchers.anyByte
/**
* Delegates to ArgumentMatchers.anyBoolean()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyBoolean: Boolean = JavaMatchers.anyBoolean
/**
* Delegates to ArgumentMatchers.anyChar()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyChar: Char = JavaMatchers.anyChar
/**
* Delegates to ArgumentMatchers.anyDouble()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyDouble: Double = JavaMatchers.anyDouble
/**
* Delegates to ArgumentMatchers.anyInt()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyInt: Int = JavaMatchers.anyInt
/**
* Delegates to ArgumentMatchers.anyFloat()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyFloat: Float = JavaMatchers.anyFloat
/**
* Delegates to ArgumentMatchers.anyShort()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyShort: Short = JavaMatchers.anyShort
/**
* Delegates to ArgumentMatchers.anyLong()
, it's only here so we expose all the `ArgumentMatchers` on a single place as any[T] would do the job just fine
*/
def anyLong: Long = JavaMatchers.anyLong
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy