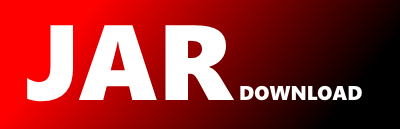
org.mod4j.dsl.presentation.xtext.services.PresentationGrammarAccess Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mod4j-presentation-xtext Show documentation
Show all versions of mod4j-presentation-xtext Show documentation
This Eclipse plug-in project contains the Presentation xtext model.
The newest version!
/*
* generated by Xtext
*/
package org.mod4j.dsl.presentation.xtext.services;
import com.google.inject.Singleton;
import com.google.inject.Inject;
import org.eclipse.xtext.*;
import org.eclipse.xtext.service.GrammarProvider;
import org.eclipse.xtext.common.services.TerminalsGrammarAccess;
@Singleton
public class PresentationGrammarAccess implements IGrammarAccess {
public class PresentationModelElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "PresentationModel");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cPresentationModelKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Assignment cExternalReferencesAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cExternalReferencesExternalReferenceParserRuleCall_3_0 = (RuleCall)cExternalReferencesAssignment_3.eContents().get(0);
private final Assignment cElementsAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final RuleCall cElementsModelElementParserRuleCall_4_0 = (RuleCall)cElementsAssignment_4.eContents().get(0);
//PresentationModel:
// description=STRING? "PresentationModel" name=ID externalReferences+=
// ExternalReference* elements+=ModelElement*;
//
////IMPORTANT: You should change the property 'overwrite.pluginresources=true' in the properties file to 'overwrite.pluginresources=false' AFTER first generation
public ParserRule getRule() { return rule; }
//description=STRING? "PresentationModel" name=ID externalReferences+=
//ExternalReference* elements+=ModelElement*
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"PresentationModel"
public Keyword getPresentationModelKeyword_1() { return cPresentationModelKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//externalReferences+=ExternalReference*
public Assignment getExternalReferencesAssignment_3() { return cExternalReferencesAssignment_3; }
//ExternalReference
public RuleCall getExternalReferencesExternalReferenceParserRuleCall_3_0() { return cExternalReferencesExternalReferenceParserRuleCall_3_0; }
//elements+=ModelElement*
public Assignment getElementsAssignment_4() { return cElementsAssignment_4; }
//ModelElement
public RuleCall getElementsModelElementParserRuleCall_4_0() { return cElementsModelElementParserRuleCall_4_0; }
}
public class ExternalReferenceElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ExternalReference");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cFromKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Assignment cModelNameAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cModelNameIDTerminalRuleCall_1_0 = (RuleCall)cModelNameAssignment_1.eContents().get(0);
private final Keyword cImportKeyword_2 = (Keyword)cGroup.eContents().get(2);
private final Assignment cNameAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cNameIDTerminalRuleCall_3_0 = (RuleCall)cNameAssignment_3.eContents().get(0);
//ExternalReference:
// "from" modelName=ID "import" name=ID;
public ParserRule getRule() { return rule; }
//"from" modelName=ID "import" name=ID
public Group getGroup() { return cGroup; }
//"from"
public Keyword getFromKeyword_0() { return cFromKeyword_0; }
//modelName=ID
public Assignment getModelNameAssignment_1() { return cModelNameAssignment_1; }
//ID
public RuleCall getModelNameIDTerminalRuleCall_1_0() { return cModelNameIDTerminalRuleCall_1_0; }
//"import"
public Keyword getImportKeyword_2() { return cImportKeyword_2; }
//name=ID
public Assignment getNameAssignment_3() { return cNameAssignment_3; }
//ID
public RuleCall getNameIDTerminalRuleCall_3_0() { return cNameIDTerminalRuleCall_3_0; }
}
public class ModelElementElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ModelElement");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final RuleCall cUIModelElementParserRuleCall_0 = (RuleCall)cAlternatives.eContents().get(0);
private final RuleCall cLinkParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
//ModelElement:
// UIModelElement|Link;
public ParserRule getRule() { return rule; }
//UIModelElement|Link
public Alternatives getAlternatives() { return cAlternatives; }
//UIModelElement
public RuleCall getUIModelElementParserRuleCall_0() { return cUIModelElementParserRuleCall_0; }
//Link
public RuleCall getLinkParserRuleCall_1() { return cLinkParserRuleCall_1; }
}
public class UIModelElementElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "UIModelElement");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final RuleCall cDialogueParserRuleCall_0 = (RuleCall)cAlternatives.eContents().get(0);
private final RuleCall cProcessParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
//UIModelElement:
// Dialogue|Process;
public ParserRule getRule() { return rule; }
//Dialogue|Process
public Alternatives getAlternatives() { return cAlternatives; }
//Dialogue
public RuleCall getDialogueParserRuleCall_0() { return cDialogueParserRuleCall_0; }
//Process
public RuleCall getProcessParserRuleCall_1() { return cProcessParserRuleCall_1; }
}
public class DialogueElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Dialogue");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final RuleCall cContentFormParserRuleCall_0 = (RuleCall)cAlternatives.eContents().get(0);
private final RuleCall cCompoundDialogueParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
//Dialogue:
// ContentForm|CompoundDialogue;
public ParserRule getRule() { return rule; }
//ContentForm|CompoundDialogue
public Alternatives getAlternatives() { return cAlternatives; }
//ContentForm
public RuleCall getContentFormParserRuleCall_0() { return cContentFormParserRuleCall_0; }
//CompoundDialogue
public RuleCall getCompoundDialogueParserRuleCall_1() { return cCompoundDialogueParserRuleCall_1; }
}
public class ContentFormElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ContentForm");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cContentFormKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
private final Group cGroup_5 = (Group)cGroup.eContents().get(5);
private final Keyword cReadonlyKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Alternatives cAlternatives_5_1 = (Alternatives)cGroup_5.eContents().get(1);
private final Assignment cReadonlyAssignment_5_1_0 = (Assignment)cAlternatives_5_1.eContents().get(0);
private final Keyword cReadonlyTrueKeyword_5_1_0_0 = (Keyword)cReadonlyAssignment_5_1_0.eContents().get(0);
private final Keyword cFalseKeyword_5_1_1 = (Keyword)cAlternatives_5_1.eContents().get(1);
private final Keyword cSemicolonKeyword_5_2 = (Keyword)cGroup_5.eContents().get(2);
private final Assignment cFormElementsAssignment_6 = (Assignment)cGroup.eContents().get(6);
private final RuleCall cFormElementsFormElementParserRuleCall_6_0 = (RuleCall)cFormElementsAssignment_6.eContents().get(0);
private final Group cGroup_7 = (Group)cGroup.eContents().get(7);
private final Keyword cProcessesKeyword_7_0 = (Keyword)cGroup_7.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_7_1 = (Keyword)cGroup_7.eContents().get(1);
private final Assignment cProcessesAssignment_7_2 = (Assignment)cGroup_7.eContents().get(2);
private final RuleCall cProcessesProcessCallParserRuleCall_7_2_0 = (RuleCall)cProcessesAssignment_7_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_7_3 = (Keyword)cGroup_7.eContents().get(3);
//ContentForm:
// description=STRING? "ContentForm" name=ID "using" contextRef=[ExternalReference] (
// "readonly" (readonly?="true"|"false") ";")? formElements+=FormElement* ("processes"
// "[" processes+=ProcessCall* "]")?;
public ParserRule getRule() { return rule; }
//description=STRING? "ContentForm" name=ID "using" contextRef=[ExternalReference] (
//"readonly" (readonly?="true"|"false") ";")? formElements+=FormElement* ("processes"
//"[" processes+=ProcessCall* "]")?
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"ContentForm"
public Keyword getContentFormKeyword_1() { return cContentFormKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
//("readonly" (readonly?="true"|"false") ";")?
public Group getGroup_5() { return cGroup_5; }
//"readonly"
public Keyword getReadonlyKeyword_5_0() { return cReadonlyKeyword_5_0; }
//readonly?="true"|"false"
public Alternatives getAlternatives_5_1() { return cAlternatives_5_1; }
//readonly?="true"
public Assignment getReadonlyAssignment_5_1_0() { return cReadonlyAssignment_5_1_0; }
//"true"
public Keyword getReadonlyTrueKeyword_5_1_0_0() { return cReadonlyTrueKeyword_5_1_0_0; }
//"false"
public Keyword getFalseKeyword_5_1_1() { return cFalseKeyword_5_1_1; }
//";"
public Keyword getSemicolonKeyword_5_2() { return cSemicolonKeyword_5_2; }
//formElements+=FormElement*
public Assignment getFormElementsAssignment_6() { return cFormElementsAssignment_6; }
//FormElement
public RuleCall getFormElementsFormElementParserRuleCall_6_0() { return cFormElementsFormElementParserRuleCall_6_0; }
//("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup_7() { return cGroup_7; }
//"processes"
public Keyword getProcessesKeyword_7_0() { return cProcessesKeyword_7_0; }
//"["
public Keyword getLeftSquareBracketKeyword_7_1() { return cLeftSquareBracketKeyword_7_1; }
//processes+=ProcessCall*
public Assignment getProcessesAssignment_7_2() { return cProcessesAssignment_7_2; }
//ProcessCall
public RuleCall getProcessesProcessCallParserRuleCall_7_2_0() { return cProcessesProcessCallParserRuleCall_7_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_7_3() { return cRightSquareBracketKeyword_7_3; }
}
public class CompoundDialogueElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "CompoundDialogue");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Assignment cDescriptionAssignment_0_0 = (Assignment)cGroup_0.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0_0 = (RuleCall)cDescriptionAssignment_0_0.eContents().get(0);
private final Keyword cCompoundDialogueKeyword_0_1 = (Keyword)cGroup_0.eContents().get(1);
private final Assignment cNameAssignment_0_2 = (Assignment)cGroup_0.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_0_2_0 = (RuleCall)cNameAssignment_0_2.eContents().get(0);
private final Keyword cUsingKeyword_0_3 = (Keyword)cGroup_0.eContents().get(3);
private final Assignment cContextRefAssignment_0_4 = (Assignment)cGroup_0.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_0_4_0 = (CrossReference)cContextRefAssignment_0_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_0_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_0_4_0.eContents().get(1);
private final Group cGroup_0_5 = (Group)cGroup_0.eContents().get(5);
private final Keyword cReadonlyKeyword_0_5_0 = (Keyword)cGroup_0_5.eContents().get(0);
private final Alternatives cAlternatives_0_5_1 = (Alternatives)cGroup_0_5.eContents().get(1);
private final Assignment cReadonlyAssignment_0_5_1_0 = (Assignment)cAlternatives_0_5_1.eContents().get(0);
private final Keyword cReadonlyTrueKeyword_0_5_1_0_0 = (Keyword)cReadonlyAssignment_0_5_1_0.eContents().get(0);
private final Keyword cFalseKeyword_0_5_1_1 = (Keyword)cAlternatives_0_5_1.eContents().get(1);
private final Keyword cSemicolonKeyword_0_5_2 = (Keyword)cGroup_0_5.eContents().get(2);
private final Group cGroup_0_6 = (Group)cGroup_0.eContents().get(6);
private final Keyword cDialoguesKeyword_0_6_0 = (Keyword)cGroup_0_6.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_0_6_1 = (Keyword)cGroup_0_6.eContents().get(1);
private final Assignment cDialoguesAssignment_0_6_2 = (Assignment)cGroup_0_6.eContents().get(2);
private final RuleCall cDialoguesDialogueCallParserRuleCall_0_6_2_0 = (RuleCall)cDialoguesAssignment_0_6_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_0_6_3 = (Keyword)cGroup_0_6.eContents().get(3);
private final Group cGroup_0_7 = (Group)cGroup_0.eContents().get(7);
private final Keyword cProcessesKeyword_0_7_0 = (Keyword)cGroup_0_7.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_0_7_1 = (Keyword)cGroup_0_7.eContents().get(1);
private final Assignment cProcessesAssignment_0_7_2 = (Assignment)cGroup_0_7.eContents().get(2);
private final RuleCall cProcessesProcessCallParserRuleCall_0_7_2_0 = (RuleCall)cProcessesAssignment_0_7_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_0_7_3 = (Keyword)cGroup_0_7.eContents().get(3);
private final RuleCall cCollectionDialogueParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
private final RuleCall cMasterDetailParserRuleCall_2 = (RuleCall)cAlternatives.eContents().get(2);
//CompoundDialogue:
// description=STRING? "CompoundDialogue" name=ID "using" contextRef=[ExternalReference
// ] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "[" dialogues+=
// DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?|CollectionDialogue
// |MasterDetail;
public ParserRule getRule() { return rule; }
//description=STRING? "CompoundDialogue" name=ID "using" contextRef=[ExternalReference
//] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "[" dialogues+=
//DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?|CollectionDialogue
//|MasterDetail
public Alternatives getAlternatives() { return cAlternatives; }
//description=STRING? "CompoundDialogue" name=ID "using" contextRef=[ExternalReference
//] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "[" dialogues+=
//DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup_0() { return cGroup_0; }
//description=STRING?
public Assignment getDescriptionAssignment_0_0() { return cDescriptionAssignment_0_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0_0; }
//"CompoundDialogue"
public Keyword getCompoundDialogueKeyword_0_1() { return cCompoundDialogueKeyword_0_1; }
//name=ID
public Assignment getNameAssignment_0_2() { return cNameAssignment_0_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_0_2_0() { return cNameIDTerminalRuleCall_0_2_0; }
//"using"
public Keyword getUsingKeyword_0_3() { return cUsingKeyword_0_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_0_4() { return cContextRefAssignment_0_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_0_4_0() { return cContextRefExternalReferenceCrossReference_0_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_0_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_0_4_0_1; }
//("readonly" (readonly?="true"|"false") ";")?
public Group getGroup_0_5() { return cGroup_0_5; }
//"readonly"
public Keyword getReadonlyKeyword_0_5_0() { return cReadonlyKeyword_0_5_0; }
//readonly?="true"|"false"
public Alternatives getAlternatives_0_5_1() { return cAlternatives_0_5_1; }
//readonly?="true"
public Assignment getReadonlyAssignment_0_5_1_0() { return cReadonlyAssignment_0_5_1_0; }
//"true"
public Keyword getReadonlyTrueKeyword_0_5_1_0_0() { return cReadonlyTrueKeyword_0_5_1_0_0; }
//"false"
public Keyword getFalseKeyword_0_5_1_1() { return cFalseKeyword_0_5_1_1; }
//";"
public Keyword getSemicolonKeyword_0_5_2() { return cSemicolonKeyword_0_5_2; }
//("dialogues" "[" dialogues+=DialogueCall* "]")?
public Group getGroup_0_6() { return cGroup_0_6; }
//"dialogues"
public Keyword getDialoguesKeyword_0_6_0() { return cDialoguesKeyword_0_6_0; }
//"["
public Keyword getLeftSquareBracketKeyword_0_6_1() { return cLeftSquareBracketKeyword_0_6_1; }
//dialogues+=DialogueCall*
public Assignment getDialoguesAssignment_0_6_2() { return cDialoguesAssignment_0_6_2; }
//DialogueCall
public RuleCall getDialoguesDialogueCallParserRuleCall_0_6_2_0() { return cDialoguesDialogueCallParserRuleCall_0_6_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_0_6_3() { return cRightSquareBracketKeyword_0_6_3; }
//("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup_0_7() { return cGroup_0_7; }
//"processes"
public Keyword getProcessesKeyword_0_7_0() { return cProcessesKeyword_0_7_0; }
//"["
public Keyword getLeftSquareBracketKeyword_0_7_1() { return cLeftSquareBracketKeyword_0_7_1; }
//processes+=ProcessCall*
public Assignment getProcessesAssignment_0_7_2() { return cProcessesAssignment_0_7_2; }
//ProcessCall
public RuleCall getProcessesProcessCallParserRuleCall_0_7_2_0() { return cProcessesProcessCallParserRuleCall_0_7_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_0_7_3() { return cRightSquareBracketKeyword_0_7_3; }
//CollectionDialogue
public RuleCall getCollectionDialogueParserRuleCall_1() { return cCollectionDialogueParserRuleCall_1; }
//MasterDetail
public RuleCall getMasterDetailParserRuleCall_2() { return cMasterDetailParserRuleCall_2; }
}
public class CollectionDialogueElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "CollectionDialogue");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cCollectionDialogueKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
private final Group cGroup_5 = (Group)cGroup.eContents().get(5);
private final Keyword cReadonlyKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Alternatives cAlternatives_5_1 = (Alternatives)cGroup_5.eContents().get(1);
private final Assignment cReadonlyAssignment_5_1_0 = (Assignment)cAlternatives_5_1.eContents().get(0);
private final Keyword cReadonlyTrueKeyword_5_1_0_0 = (Keyword)cReadonlyAssignment_5_1_0.eContents().get(0);
private final Keyword cFalseKeyword_5_1_1 = (Keyword)cAlternatives_5_1.eContents().get(1);
private final Keyword cSemicolonKeyword_5_2 = (Keyword)cGroup_5.eContents().get(2);
private final Group cGroup_6 = (Group)cGroup.eContents().get(6);
private final Keyword cDialoguesKeyword_6_0 = (Keyword)cGroup_6.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_6_1 = (Keyword)cGroup_6.eContents().get(1);
private final Assignment cDialoguesAssignment_6_2 = (Assignment)cGroup_6.eContents().get(2);
private final RuleCall cDialoguesDialogueCallParserRuleCall_6_2_0 = (RuleCall)cDialoguesAssignment_6_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_6_3 = (Keyword)cGroup_6.eContents().get(3);
private final Group cGroup_7 = (Group)cGroup.eContents().get(7);
private final Keyword cProcessesKeyword_7_0 = (Keyword)cGroup_7.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_7_1 = (Keyword)cGroup_7.eContents().get(1);
private final Assignment cProcessesAssignment_7_2 = (Assignment)cGroup_7.eContents().get(2);
private final RuleCall cProcessesProcessCallParserRuleCall_7_2_0 = (RuleCall)cProcessesAssignment_7_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_7_3 = (Keyword)cGroup_7.eContents().get(3);
//CollectionDialogue:
// description=STRING? "CollectionDialogue" name=ID "using" contextRef=[
// ExternalReference] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "["
// dialogues+=DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?;
public ParserRule getRule() { return rule; }
//description=STRING? "CollectionDialogue" name=ID "using" contextRef=[
//ExternalReference] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "["
//dialogues+=DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"CollectionDialogue"
public Keyword getCollectionDialogueKeyword_1() { return cCollectionDialogueKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
//("readonly" (readonly?="true"|"false") ";")?
public Group getGroup_5() { return cGroup_5; }
//"readonly"
public Keyword getReadonlyKeyword_5_0() { return cReadonlyKeyword_5_0; }
//readonly?="true"|"false"
public Alternatives getAlternatives_5_1() { return cAlternatives_5_1; }
//readonly?="true"
public Assignment getReadonlyAssignment_5_1_0() { return cReadonlyAssignment_5_1_0; }
//"true"
public Keyword getReadonlyTrueKeyword_5_1_0_0() { return cReadonlyTrueKeyword_5_1_0_0; }
//"false"
public Keyword getFalseKeyword_5_1_1() { return cFalseKeyword_5_1_1; }
//";"
public Keyword getSemicolonKeyword_5_2() { return cSemicolonKeyword_5_2; }
//("dialogues" "[" dialogues+=DialogueCall* "]")?
public Group getGroup_6() { return cGroup_6; }
//"dialogues"
public Keyword getDialoguesKeyword_6_0() { return cDialoguesKeyword_6_0; }
//"["
public Keyword getLeftSquareBracketKeyword_6_1() { return cLeftSquareBracketKeyword_6_1; }
//dialogues+=DialogueCall*
public Assignment getDialoguesAssignment_6_2() { return cDialoguesAssignment_6_2; }
//DialogueCall
public RuleCall getDialoguesDialogueCallParserRuleCall_6_2_0() { return cDialoguesDialogueCallParserRuleCall_6_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_6_3() { return cRightSquareBracketKeyword_6_3; }
//("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup_7() { return cGroup_7; }
//"processes"
public Keyword getProcessesKeyword_7_0() { return cProcessesKeyword_7_0; }
//"["
public Keyword getLeftSquareBracketKeyword_7_1() { return cLeftSquareBracketKeyword_7_1; }
//processes+=ProcessCall*
public Assignment getProcessesAssignment_7_2() { return cProcessesAssignment_7_2; }
//ProcessCall
public RuleCall getProcessesProcessCallParserRuleCall_7_2_0() { return cProcessesProcessCallParserRuleCall_7_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_7_3() { return cRightSquareBracketKeyword_7_3; }
}
public class MasterDetailElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "MasterDetail");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cMasterDetailKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
private final Group cGroup_5 = (Group)cGroup.eContents().get(5);
private final Keyword cReadonlyKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Alternatives cAlternatives_5_1 = (Alternatives)cGroup_5.eContents().get(1);
private final Assignment cReadonlyAssignment_5_1_0 = (Assignment)cAlternatives_5_1.eContents().get(0);
private final Keyword cReadonlyTrueKeyword_5_1_0_0 = (Keyword)cReadonlyAssignment_5_1_0.eContents().get(0);
private final Keyword cFalseKeyword_5_1_1 = (Keyword)cAlternatives_5_1.eContents().get(1);
private final Keyword cSemicolonKeyword_5_2 = (Keyword)cGroup_5.eContents().get(2);
private final Keyword cMasterKeyword_6 = (Keyword)cGroup.eContents().get(6);
private final Assignment cMasterAssignment_7 = (Assignment)cGroup.eContents().get(7);
private final RuleCall cMasterDialogueCallParserRuleCall_7_0 = (RuleCall)cMasterAssignment_7.eContents().get(0);
private final Keyword cDetailKeyword_8 = (Keyword)cGroup.eContents().get(8);
private final Assignment cDetailAssignment_9 = (Assignment)cGroup.eContents().get(9);
private final RuleCall cDetailDialogueCallParserRuleCall_9_0 = (RuleCall)cDetailAssignment_9.eContents().get(0);
private final Group cGroup_10 = (Group)cGroup.eContents().get(10);
private final Keyword cProcessesKeyword_10_0 = (Keyword)cGroup_10.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_10_1 = (Keyword)cGroup_10.eContents().get(1);
private final Assignment cProcessesAssignment_10_2 = (Assignment)cGroup_10.eContents().get(2);
private final RuleCall cProcessesProcessCallParserRuleCall_10_2_0 = (RuleCall)cProcessesAssignment_10_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_10_3 = (Keyword)cGroup_10.eContents().get(3);
//MasterDetail:
// description=STRING? "MasterDetail" name=ID "using" contextRef=[ExternalReference] (
// "readonly" (readonly?="true"|"false") ";")? "master" master=DialogueCall "detail"
// detail=DialogueCall ("processes" "[" processes+=ProcessCall* "]")?;
public ParserRule getRule() { return rule; }
//description=STRING? "MasterDetail" name=ID "using" contextRef=[ExternalReference] (
//"readonly" (readonly?="true"|"false") ";")? "master" master=DialogueCall "detail"
//detail=DialogueCall ("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"MasterDetail"
public Keyword getMasterDetailKeyword_1() { return cMasterDetailKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
//("readonly" (readonly?="true"|"false") ";")?
public Group getGroup_5() { return cGroup_5; }
//"readonly"
public Keyword getReadonlyKeyword_5_0() { return cReadonlyKeyword_5_0; }
//readonly?="true"|"false"
public Alternatives getAlternatives_5_1() { return cAlternatives_5_1; }
//readonly?="true"
public Assignment getReadonlyAssignment_5_1_0() { return cReadonlyAssignment_5_1_0; }
//"true"
public Keyword getReadonlyTrueKeyword_5_1_0_0() { return cReadonlyTrueKeyword_5_1_0_0; }
//"false"
public Keyword getFalseKeyword_5_1_1() { return cFalseKeyword_5_1_1; }
//";"
public Keyword getSemicolonKeyword_5_2() { return cSemicolonKeyword_5_2; }
//"master"
public Keyword getMasterKeyword_6() { return cMasterKeyword_6; }
//master=DialogueCall
public Assignment getMasterAssignment_7() { return cMasterAssignment_7; }
//DialogueCall
public RuleCall getMasterDialogueCallParserRuleCall_7_0() { return cMasterDialogueCallParserRuleCall_7_0; }
//"detail"
public Keyword getDetailKeyword_8() { return cDetailKeyword_8; }
//detail=DialogueCall
public Assignment getDetailAssignment_9() { return cDetailAssignment_9; }
//DialogueCall
public RuleCall getDetailDialogueCallParserRuleCall_9_0() { return cDetailDialogueCallParserRuleCall_9_0; }
//("processes" "[" processes+=ProcessCall* "]")?
public Group getGroup_10() { return cGroup_10; }
//"processes"
public Keyword getProcessesKeyword_10_0() { return cProcessesKeyword_10_0; }
//"["
public Keyword getLeftSquareBracketKeyword_10_1() { return cLeftSquareBracketKeyword_10_1; }
//processes+=ProcessCall*
public Assignment getProcessesAssignment_10_2() { return cProcessesAssignment_10_2; }
//ProcessCall
public RuleCall getProcessesProcessCallParserRuleCall_10_2_0() { return cProcessesProcessCallParserRuleCall_10_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_10_3() { return cRightSquareBracketKeyword_10_3; }
}
public class ProcessElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Process");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final RuleCall cAutomatedProcessParserRuleCall_0 = (RuleCall)cAlternatives.eContents().get(0);
private final RuleCall cInteractiveProcessParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
//Process:
// AutomatedProcess|InteractiveProcess;
public ParserRule getRule() { return rule; }
//AutomatedProcess|InteractiveProcess
public Alternatives getAlternatives() { return cAlternatives; }
//AutomatedProcess
public RuleCall getAutomatedProcessParserRuleCall_0() { return cAutomatedProcessParserRuleCall_0; }
//InteractiveProcess
public RuleCall getInteractiveProcessParserRuleCall_1() { return cInteractiveProcessParserRuleCall_1; }
}
public class AutomatedProcessElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "AutomatedProcess");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cAutomatedProcessKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
private final Group cGroup_5 = (Group)cGroup.eContents().get(5);
private final Keyword cStepsKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_5_1 = (Keyword)cGroup_5.eContents().get(1);
private final Assignment cProcessElementsAssignment_5_2 = (Assignment)cGroup_5.eContents().get(2);
private final RuleCall cProcessElementsUICallParserRuleCall_5_2_0 = (RuleCall)cProcessElementsAssignment_5_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_5_3 = (Keyword)cGroup_5.eContents().get(3);
//AutomatedProcess:
// description=STRING? "AutomatedProcess" name=ID "using" contextRef=[ExternalReference
// ] ("steps" "[" processElements+=UICall* "]")?;
public ParserRule getRule() { return rule; }
//description=STRING? "AutomatedProcess" name=ID "using" contextRef=[ExternalReference
//] ("steps" "[" processElements+=UICall* "]")?
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"AutomatedProcess"
public Keyword getAutomatedProcessKeyword_1() { return cAutomatedProcessKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
//("steps" "[" processElements+=UICall* "]")?
public Group getGroup_5() { return cGroup_5; }
//"steps"
public Keyword getStepsKeyword_5_0() { return cStepsKeyword_5_0; }
//"["
public Keyword getLeftSquareBracketKeyword_5_1() { return cLeftSquareBracketKeyword_5_1; }
//processElements+=UICall*
public Assignment getProcessElementsAssignment_5_2() { return cProcessElementsAssignment_5_2; }
//UICall
public RuleCall getProcessElementsUICallParserRuleCall_5_2_0() { return cProcessElementsUICallParserRuleCall_5_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_5_3() { return cRightSquareBracketKeyword_5_3; }
}
public class InteractiveProcessElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "InteractiveProcess");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cInteractiveProcessKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
private final Group cGroup_5 = (Group)cGroup.eContents().get(5);
private final Keyword cStepsKeyword_5_0 = (Keyword)cGroup_5.eContents().get(0);
private final Keyword cLeftSquareBracketKeyword_5_1 = (Keyword)cGroup_5.eContents().get(1);
private final Assignment cProcessElementsAssignment_5_2 = (Assignment)cGroup_5.eContents().get(2);
private final RuleCall cProcessElementsUICallParserRuleCall_5_2_0 = (RuleCall)cProcessElementsAssignment_5_2.eContents().get(0);
private final Keyword cRightSquareBracketKeyword_5_3 = (Keyword)cGroup_5.eContents().get(3);
//InteractiveProcess:
// description=STRING? "InteractiveProcess" name=ID "using" contextRef=[
// ExternalReference] ("steps" "[" processElements+=UICall* "]")?;
public ParserRule getRule() { return rule; }
//description=STRING? "InteractiveProcess" name=ID "using" contextRef=[
//ExternalReference] ("steps" "[" processElements+=UICall* "]")?
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"InteractiveProcess"
public Keyword getInteractiveProcessKeyword_1() { return cInteractiveProcessKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
//("steps" "[" processElements+=UICall* "]")?
public Group getGroup_5() { return cGroup_5; }
//"steps"
public Keyword getStepsKeyword_5_0() { return cStepsKeyword_5_0; }
//"["
public Keyword getLeftSquareBracketKeyword_5_1() { return cLeftSquareBracketKeyword_5_1; }
//processElements+=UICall*
public Assignment getProcessElementsAssignment_5_2() { return cProcessElementsAssignment_5_2; }
//UICall
public RuleCall getProcessElementsUICallParserRuleCall_5_2_0() { return cProcessElementsUICallParserRuleCall_5_2_0; }
//"]"
public Keyword getRightSquareBracketKeyword_5_3() { return cRightSquareBracketKeyword_5_3; }
}
public class FormElementElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "FormElement");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cFormElementKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Assignment cReferencesAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cReferencesDtoPropertyReferenceParserRuleCall_1_0 = (RuleCall)cReferencesAssignment_1.eContents().get(0);
private final Keyword cNamedKeyword_2 = (Keyword)cGroup.eContents().get(2);
private final Assignment cNameAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cNameIDTerminalRuleCall_3_0 = (RuleCall)cNameAssignment_3.eContents().get(0);
private final Group cGroup_4 = (Group)cGroup.eContents().get(4);
private final Keyword cReadonlyKeyword_4_0 = (Keyword)cGroup_4.eContents().get(0);
private final Alternatives cAlternatives_4_1 = (Alternatives)cGroup_4.eContents().get(1);
private final Assignment cReadonlyAssignment_4_1_0 = (Assignment)cAlternatives_4_1.eContents().get(0);
private final Keyword cReadonlyTrueKeyword_4_1_0_0 = (Keyword)cReadonlyAssignment_4_1_0.eContents().get(0);
private final Keyword cFalseKeyword_4_1_1 = (Keyword)cAlternatives_4_1.eContents().get(1);
private final Keyword cSemicolonKeyword_5 = (Keyword)cGroup.eContents().get(5);
//FormElement:
// "formElement" references=DtoPropertyReference "named" name=ID ("readonly" (readonly?=
// "true"|"false"))? ";";
//
//
//
//// ("navigate" LinkRef "to")?
public ParserRule getRule() { return rule; }
//"formElement" references=DtoPropertyReference "named" name=ID ("readonly" (readonly?=
//"true"|"false"))? ";"
//
//// ("navigate" LinkRef "to")?
public Group getGroup() { return cGroup; }
//"formElement"
//
//// ("navigate" LinkRef "to")?
public Keyword getFormElementKeyword_0() { return cFormElementKeyword_0; }
//references=DtoPropertyReference
//// ("navigate" LinkRef "to")?
public Assignment getReferencesAssignment_1() { return cReferencesAssignment_1; }
//DtoPropertyReference
public RuleCall getReferencesDtoPropertyReferenceParserRuleCall_1_0() { return cReferencesDtoPropertyReferenceParserRuleCall_1_0; }
//"named"
public Keyword getNamedKeyword_2() { return cNamedKeyword_2; }
//name=ID
public Assignment getNameAssignment_3() { return cNameAssignment_3; }
//ID
public RuleCall getNameIDTerminalRuleCall_3_0() { return cNameIDTerminalRuleCall_3_0; }
//("readonly" (readonly?="true"|"false"))?
public Group getGroup_4() { return cGroup_4; }
//"readonly"
public Keyword getReadonlyKeyword_4_0() { return cReadonlyKeyword_4_0; }
//readonly?="true"|"false"
public Alternatives getAlternatives_4_1() { return cAlternatives_4_1; }
//readonly?="true"
public Assignment getReadonlyAssignment_4_1_0() { return cReadonlyAssignment_4_1_0; }
//"true"
public Keyword getReadonlyTrueKeyword_4_1_0_0() { return cReadonlyTrueKeyword_4_1_0_0; }
//"false"
public Keyword getFalseKeyword_4_1_1() { return cFalseKeyword_4_1_1; }
//";"
public Keyword getSemicolonKeyword_5() { return cSemicolonKeyword_5; }
}
public class DialogueCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "DialogueCall");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final RuleCall cDirectDialogueCallParserRuleCall_0_0 = (RuleCall)cAlternatives_0.eContents().get(0);
private final RuleCall cLinkedDialogueCallParserRuleCall_0_1 = (RuleCall)cAlternatives_0.eContents().get(1);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cAliasKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cAliasAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cAliasIDTerminalRuleCall_1_1_0 = (RuleCall)cAliasAssignment_1_1.eContents().get(0);
private final Keyword cSemicolonKeyword_2 = (Keyword)cGroup.eContents().get(2);
//DialogueCall:
// (DirectDialogueCall|LinkedDialogueCall) ("alias" alias=ID)? ";";
public ParserRule getRule() { return rule; }
//(DirectDialogueCall|LinkedDialogueCall) ("alias" alias=ID)? ";"
public Group getGroup() { return cGroup; }
//DirectDialogueCall|LinkedDialogueCall
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//DirectDialogueCall
public RuleCall getDirectDialogueCallParserRuleCall_0_0() { return cDirectDialogueCallParserRuleCall_0_0; }
//LinkedDialogueCall
public RuleCall getLinkedDialogueCallParserRuleCall_0_1() { return cLinkedDialogueCallParserRuleCall_0_1; }
//("alias" alias=ID)?
public Group getGroup_1() { return cGroup_1; }
//"alias"
public Keyword getAliasKeyword_1_0() { return cAliasKeyword_1_0; }
//alias=ID
public Assignment getAliasAssignment_1_1() { return cAliasAssignment_1_1; }
//ID
public RuleCall getAliasIDTerminalRuleCall_1_1_0() { return cAliasIDTerminalRuleCall_1_1_0; }
//";"
public Keyword getSemicolonKeyword_2() { return cSemicolonKeyword_2; }
}
public class LinkedDialogueCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "LinkedDialogueCall");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cNavigateKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Assignment cLinkAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cLinkLinkRefParserRuleCall_1_0 = (RuleCall)cLinkAssignment_1.eContents().get(0);
private final Keyword cToKeyword_2 = (Keyword)cGroup.eContents().get(2);
private final Assignment cNameAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cNameIDTerminalRuleCall_3_0 = (RuleCall)cNameAssignment_3.eContents().get(0);
//LinkedDialogueCall:
// "navigate" link=LinkRef "to" name=ID;
public ParserRule getRule() { return rule; }
//"navigate" link=LinkRef "to" name=ID
public Group getGroup() { return cGroup; }
//"navigate"
public Keyword getNavigateKeyword_0() { return cNavigateKeyword_0; }
//link=LinkRef
public Assignment getLinkAssignment_1() { return cLinkAssignment_1; }
//LinkRef
public RuleCall getLinkLinkRefParserRuleCall_1_0() { return cLinkLinkRefParserRuleCall_1_0; }
//"to"
public Keyword getToKeyword_2() { return cToKeyword_2; }
//name=ID
public Assignment getNameAssignment_3() { return cNameAssignment_3; }
//ID
public RuleCall getNameIDTerminalRuleCall_3_0() { return cNameIDTerminalRuleCall_3_0; }
}
public class DirectDialogueCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "DirectDialogueCall");
private final Assignment cNameAssignment = (Assignment)rule.eContents().get(1);
private final RuleCall cNameIDTerminalRuleCall_0 = (RuleCall)cNameAssignment.eContents().get(0);
//DirectDialogueCall:
// name=ID;
public ParserRule getRule() { return rule; }
//name=ID
public Assignment getNameAssignment() { return cNameAssignment; }
//ID
public RuleCall getNameIDTerminalRuleCall_0() { return cNameIDTerminalRuleCall_0; }
}
public class ProcessCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "ProcessCall");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Alternatives cAlternatives_0 = (Alternatives)cGroup.eContents().get(0);
private final RuleCall cDirectProcessCallParserRuleCall_0_0 = (RuleCall)cAlternatives_0.eContents().get(0);
private final RuleCall cLinkedProcessCallParserRuleCall_0_1 = (RuleCall)cAlternatives_0.eContents().get(1);
private final Group cGroup_1 = (Group)cGroup.eContents().get(1);
private final Keyword cAliasKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final Assignment cAliasAssignment_1_1 = (Assignment)cGroup_1.eContents().get(1);
private final RuleCall cAliasIDTerminalRuleCall_1_1_0 = (RuleCall)cAliasAssignment_1_1.eContents().get(0);
private final Keyword cSemicolonKeyword_2 = (Keyword)cGroup.eContents().get(2);
//ProcessCall:
// (DirectProcessCall|LinkedProcessCall) ("alias" alias=ID)? ";";
public ParserRule getRule() { return rule; }
//(DirectProcessCall|LinkedProcessCall) ("alias" alias=ID)? ";"
public Group getGroup() { return cGroup; }
//DirectProcessCall|LinkedProcessCall
public Alternatives getAlternatives_0() { return cAlternatives_0; }
//DirectProcessCall
public RuleCall getDirectProcessCallParserRuleCall_0_0() { return cDirectProcessCallParserRuleCall_0_0; }
//LinkedProcessCall
public RuleCall getLinkedProcessCallParserRuleCall_0_1() { return cLinkedProcessCallParserRuleCall_0_1; }
//("alias" alias=ID)?
public Group getGroup_1() { return cGroup_1; }
//"alias"
public Keyword getAliasKeyword_1_0() { return cAliasKeyword_1_0; }
//alias=ID
public Assignment getAliasAssignment_1_1() { return cAliasAssignment_1_1; }
//ID
public RuleCall getAliasIDTerminalRuleCall_1_1_0() { return cAliasIDTerminalRuleCall_1_1_0; }
//";"
public Keyword getSemicolonKeyword_2() { return cSemicolonKeyword_2; }
}
public class LinkedProcessCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "LinkedProcessCall");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Keyword cNavigateKeyword_0 = (Keyword)cGroup.eContents().get(0);
private final Assignment cLinkAssignment_1 = (Assignment)cGroup.eContents().get(1);
private final RuleCall cLinkLinkRefParserRuleCall_1_0 = (RuleCall)cLinkAssignment_1.eContents().get(0);
private final Keyword cToKeyword_2 = (Keyword)cGroup.eContents().get(2);
private final Assignment cNameAssignment_3 = (Assignment)cGroup.eContents().get(3);
private final RuleCall cNameIDTerminalRuleCall_3_0 = (RuleCall)cNameAssignment_3.eContents().get(0);
//LinkedProcessCall:
// "navigate" link=LinkRef "to" name=ID;
public ParserRule getRule() { return rule; }
//"navigate" link=LinkRef "to" name=ID
public Group getGroup() { return cGroup; }
//"navigate"
public Keyword getNavigateKeyword_0() { return cNavigateKeyword_0; }
//link=LinkRef
public Assignment getLinkAssignment_1() { return cLinkAssignment_1; }
//LinkRef
public RuleCall getLinkLinkRefParserRuleCall_1_0() { return cLinkLinkRefParserRuleCall_1_0; }
//"to"
public Keyword getToKeyword_2() { return cToKeyword_2; }
//name=ID
public Assignment getNameAssignment_3() { return cNameAssignment_3; }
//ID
public RuleCall getNameIDTerminalRuleCall_3_0() { return cNameIDTerminalRuleCall_3_0; }
}
public class DirectProcessCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "DirectProcessCall");
private final Assignment cNameAssignment = (Assignment)rule.eContents().get(1);
private final RuleCall cNameIDTerminalRuleCall_0 = (RuleCall)cNameAssignment.eContents().get(0);
//DirectProcessCall:
// name=ID;
public ParserRule getRule() { return rule; }
//name=ID
public Assignment getNameAssignment() { return cNameAssignment; }
//ID
public RuleCall getNameIDTerminalRuleCall_0() { return cNameIDTerminalRuleCall_0; }
}
public class LinkRefElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "LinkRef");
private final Assignment cNameAssignment = (Assignment)rule.eContents().get(1);
private final RuleCall cNameIDTerminalRuleCall_0 = (RuleCall)cNameAssignment.eContents().get(0);
//LinkRef:
// name=ID;
public ParserRule getRule() { return rule; }
//name=ID
public Assignment getNameAssignment() { return cNameAssignment; }
//ID
public RuleCall getNameIDTerminalRuleCall_0() { return cNameIDTerminalRuleCall_0; }
}
public class LinkElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "Link");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final RuleCall cLinkStepParserRuleCall_0 = (RuleCall)cAlternatives.eContents().get(0);
private final RuleCall cLinkPathParserRuleCall_1 = (RuleCall)cAlternatives.eContents().get(1);
//Link:
// LinkStep|LinkPath;
public ParserRule getRule() { return rule; }
//LinkStep|LinkPath
public Alternatives getAlternatives() { return cAlternatives; }
//LinkStep
public RuleCall getLinkStepParserRuleCall_0() { return cLinkStepParserRuleCall_0; }
//LinkPath
public RuleCall getLinkPathParserRuleCall_1() { return cLinkPathParserRuleCall_1; }
}
public class LinkStepElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "LinkStep");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cLinkStepKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
private final Keyword cNavigateKeyword_5 = (Keyword)cGroup.eContents().get(5);
private final Assignment cReferenceAssignment_6 = (Assignment)cGroup.eContents().get(6);
private final RuleCall cReferenceAssociationRoleReferenceParserRuleCall_6_0 = (RuleCall)cReferenceAssignment_6.eContents().get(0);
//LinkStep:
// description=STRING? "LinkStep" name=ID "using" contextRef=[ExternalReference]
// "navigate" reference=AssociationRoleReference;
public ParserRule getRule() { return rule; }
//description=STRING? "LinkStep" name=ID "using" contextRef=[ExternalReference]
//"navigate" reference=AssociationRoleReference
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"LinkStep"
public Keyword getLinkStepKeyword_1() { return cLinkStepKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
//"navigate"
public Keyword getNavigateKeyword_5() { return cNavigateKeyword_5; }
//reference=AssociationRoleReference
public Assignment getReferenceAssignment_6() { return cReferenceAssignment_6; }
//AssociationRoleReference
public RuleCall getReferenceAssociationRoleReferenceParserRuleCall_6_0() { return cReferenceAssociationRoleReferenceParserRuleCall_6_0; }
}
public class LinkPathElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "LinkPath");
private final Group cGroup = (Group)rule.eContents().get(1);
private final Assignment cDescriptionAssignment_0 = (Assignment)cGroup.eContents().get(0);
private final RuleCall cDescriptionSTRINGTerminalRuleCall_0_0 = (RuleCall)cDescriptionAssignment_0.eContents().get(0);
private final Keyword cLinkPathKeyword_1 = (Keyword)cGroup.eContents().get(1);
private final Assignment cNameAssignment_2 = (Assignment)cGroup.eContents().get(2);
private final RuleCall cNameIDTerminalRuleCall_2_0 = (RuleCall)cNameAssignment_2.eContents().get(0);
private final Keyword cUsingKeyword_3 = (Keyword)cGroup.eContents().get(3);
private final Assignment cContextRefAssignment_4 = (Assignment)cGroup.eContents().get(4);
private final CrossReference cContextRefExternalReferenceCrossReference_4_0 = (CrossReference)cContextRefAssignment_4.eContents().get(0);
private final RuleCall cContextRefExternalReferenceIDTerminalRuleCall_4_0_1 = (RuleCall)cContextRefExternalReferenceCrossReference_4_0.eContents().get(1);
//LinkPath:
// description=STRING? "LinkPath" name=ID "using" contextRef=[ExternalReference];
public ParserRule getRule() { return rule; }
//description=STRING? "LinkPath" name=ID "using" contextRef=[ExternalReference]
public Group getGroup() { return cGroup; }
//description=STRING?
public Assignment getDescriptionAssignment_0() { return cDescriptionAssignment_0; }
//STRING
public RuleCall getDescriptionSTRINGTerminalRuleCall_0_0() { return cDescriptionSTRINGTerminalRuleCall_0_0; }
//"LinkPath"
public Keyword getLinkPathKeyword_1() { return cLinkPathKeyword_1; }
//name=ID
public Assignment getNameAssignment_2() { return cNameAssignment_2; }
//ID
public RuleCall getNameIDTerminalRuleCall_2_0() { return cNameIDTerminalRuleCall_2_0; }
//"using"
public Keyword getUsingKeyword_3() { return cUsingKeyword_3; }
//contextRef=[ExternalReference]
public Assignment getContextRefAssignment_4() { return cContextRefAssignment_4; }
//[ExternalReference]
public CrossReference getContextRefExternalReferenceCrossReference_4_0() { return cContextRefExternalReferenceCrossReference_4_0; }
//ID
public RuleCall getContextRefExternalReferenceIDTerminalRuleCall_4_0_1() { return cContextRefExternalReferenceIDTerminalRuleCall_4_0_1; }
}
public class DtoPropertyReferenceElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "DtoPropertyReference");
private final Assignment cNameAssignment = (Assignment)rule.eContents().get(1);
private final RuleCall cNameIDTerminalRuleCall_0 = (RuleCall)cNameAssignment.eContents().get(0);
//DtoPropertyReference:
// name=ID;
public ParserRule getRule() { return rule; }
//name=ID
public Assignment getNameAssignment() { return cNameAssignment; }
//ID
public RuleCall getNameIDTerminalRuleCall_0() { return cNameIDTerminalRuleCall_0; }
}
public class AssociationRoleReferenceElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "AssociationRoleReference");
private final Assignment cNameAssignment = (Assignment)rule.eContents().get(1);
private final RuleCall cNameIDTerminalRuleCall_0 = (RuleCall)cNameAssignment.eContents().get(0);
//AssociationRoleReference:
// name=ID;
public ParserRule getRule() { return rule; }
//name=ID
public Assignment getNameAssignment() { return cNameAssignment; }
//ID
public RuleCall getNameIDTerminalRuleCall_0() { return cNameIDTerminalRuleCall_0; }
}
public class UICallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "UICall");
private final RuleCall cUIModelElementCallParserRuleCall = (RuleCall)rule.eContents().get(1);
//UICall:
// UIModelElementCall;
public ParserRule getRule() { return rule; }
//UIModelElementCall
public RuleCall getUIModelElementCallParserRuleCall() { return cUIModelElementCallParserRuleCall; }
}
public class UIModelElementCallElements implements IParserRuleAccess {
private final ParserRule rule = (ParserRule) GrammarUtil.findRuleForName(getGrammar(), "UIModelElementCall");
private final Alternatives cAlternatives = (Alternatives)rule.eContents().get(1);
private final Group cGroup_0 = (Group)cAlternatives.eContents().get(0);
private final Keyword cDialogueKeyword_0_0 = (Keyword)cGroup_0.eContents().get(0);
private final RuleCall cDialogueCallParserRuleCall_0_1 = (RuleCall)cGroup_0.eContents().get(1);
private final Group cGroup_1 = (Group)cAlternatives.eContents().get(1);
private final Keyword cProcessKeyword_1_0 = (Keyword)cGroup_1.eContents().get(0);
private final RuleCall cProcessCallParserRuleCall_1_1 = (RuleCall)cGroup_1.eContents().get(1);
//UIModelElementCall:
// "dialogue" DialogueCall|"process" ProcessCall;
public ParserRule getRule() { return rule; }
//"dialogue" DialogueCall|"process" ProcessCall
public Alternatives getAlternatives() { return cAlternatives; }
//"dialogue" DialogueCall
public Group getGroup_0() { return cGroup_0; }
//"dialogue"
public Keyword getDialogueKeyword_0_0() { return cDialogueKeyword_0_0; }
//DialogueCall
public RuleCall getDialogueCallParserRuleCall_0_1() { return cDialogueCallParserRuleCall_0_1; }
//"process" ProcessCall
public Group getGroup_1() { return cGroup_1; }
//"process"
public Keyword getProcessKeyword_1_0() { return cProcessKeyword_1_0; }
//ProcessCall
public RuleCall getProcessCallParserRuleCall_1_1() { return cProcessCallParserRuleCall_1_1; }
}
private PresentationModelElements pPresentationModel;
private ExternalReferenceElements pExternalReference;
private ModelElementElements pModelElement;
private UIModelElementElements pUIModelElement;
private DialogueElements pDialogue;
private ContentFormElements pContentForm;
private CompoundDialogueElements pCompoundDialogue;
private CollectionDialogueElements pCollectionDialogue;
private MasterDetailElements pMasterDetail;
private ProcessElements pProcess;
private AutomatedProcessElements pAutomatedProcess;
private InteractiveProcessElements pInteractiveProcess;
private FormElementElements pFormElement;
private DialogueCallElements pDialogueCall;
private LinkedDialogueCallElements pLinkedDialogueCall;
private DirectDialogueCallElements pDirectDialogueCall;
private ProcessCallElements pProcessCall;
private LinkedProcessCallElements pLinkedProcessCall;
private DirectProcessCallElements pDirectProcessCall;
private LinkRefElements pLinkRef;
private LinkElements pLink;
private LinkStepElements pLinkStep;
private LinkPathElements pLinkPath;
private DtoPropertyReferenceElements pDtoPropertyReference;
private AssociationRoleReferenceElements pAssociationRoleReference;
private UICallElements pUICall;
private UIModelElementCallElements pUIModelElementCall;
private final GrammarProvider grammarProvider;
private TerminalsGrammarAccess gaTerminals;
@Inject
public PresentationGrammarAccess(GrammarProvider grammarProvider,
TerminalsGrammarAccess gaTerminals) {
this.grammarProvider = grammarProvider;
this.gaTerminals = gaTerminals;
}
public Grammar getGrammar() {
return grammarProvider.getGrammar(this);
}
public TerminalsGrammarAccess getTerminalsGrammarAccess() {
return gaTerminals;
}
//PresentationModel:
// description=STRING? "PresentationModel" name=ID externalReferences+=
// ExternalReference* elements+=ModelElement*;
//
////IMPORTANT: You should change the property 'overwrite.pluginresources=true' in the properties file to 'overwrite.pluginresources=false' AFTER first generation
public PresentationModelElements getPresentationModelAccess() {
return (pPresentationModel != null) ? pPresentationModel : (pPresentationModel = new PresentationModelElements());
}
public ParserRule getPresentationModelRule() {
return getPresentationModelAccess().getRule();
}
//ExternalReference:
// "from" modelName=ID "import" name=ID;
public ExternalReferenceElements getExternalReferenceAccess() {
return (pExternalReference != null) ? pExternalReference : (pExternalReference = new ExternalReferenceElements());
}
public ParserRule getExternalReferenceRule() {
return getExternalReferenceAccess().getRule();
}
//ModelElement:
// UIModelElement|Link;
public ModelElementElements getModelElementAccess() {
return (pModelElement != null) ? pModelElement : (pModelElement = new ModelElementElements());
}
public ParserRule getModelElementRule() {
return getModelElementAccess().getRule();
}
//UIModelElement:
// Dialogue|Process;
public UIModelElementElements getUIModelElementAccess() {
return (pUIModelElement != null) ? pUIModelElement : (pUIModelElement = new UIModelElementElements());
}
public ParserRule getUIModelElementRule() {
return getUIModelElementAccess().getRule();
}
//Dialogue:
// ContentForm|CompoundDialogue;
public DialogueElements getDialogueAccess() {
return (pDialogue != null) ? pDialogue : (pDialogue = new DialogueElements());
}
public ParserRule getDialogueRule() {
return getDialogueAccess().getRule();
}
//ContentForm:
// description=STRING? "ContentForm" name=ID "using" contextRef=[ExternalReference] (
// "readonly" (readonly?="true"|"false") ";")? formElements+=FormElement* ("processes"
// "[" processes+=ProcessCall* "]")?;
public ContentFormElements getContentFormAccess() {
return (pContentForm != null) ? pContentForm : (pContentForm = new ContentFormElements());
}
public ParserRule getContentFormRule() {
return getContentFormAccess().getRule();
}
//CompoundDialogue:
// description=STRING? "CompoundDialogue" name=ID "using" contextRef=[ExternalReference
// ] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "[" dialogues+=
// DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?|CollectionDialogue
// |MasterDetail;
public CompoundDialogueElements getCompoundDialogueAccess() {
return (pCompoundDialogue != null) ? pCompoundDialogue : (pCompoundDialogue = new CompoundDialogueElements());
}
public ParserRule getCompoundDialogueRule() {
return getCompoundDialogueAccess().getRule();
}
//CollectionDialogue:
// description=STRING? "CollectionDialogue" name=ID "using" contextRef=[
// ExternalReference] ("readonly" (readonly?="true"|"false") ";")? ("dialogues" "["
// dialogues+=DialogueCall* "]")? ("processes" "[" processes+=ProcessCall* "]")?;
public CollectionDialogueElements getCollectionDialogueAccess() {
return (pCollectionDialogue != null) ? pCollectionDialogue : (pCollectionDialogue = new CollectionDialogueElements());
}
public ParserRule getCollectionDialogueRule() {
return getCollectionDialogueAccess().getRule();
}
//MasterDetail:
// description=STRING? "MasterDetail" name=ID "using" contextRef=[ExternalReference] (
// "readonly" (readonly?="true"|"false") ";")? "master" master=DialogueCall "detail"
// detail=DialogueCall ("processes" "[" processes+=ProcessCall* "]")?;
public MasterDetailElements getMasterDetailAccess() {
return (pMasterDetail != null) ? pMasterDetail : (pMasterDetail = new MasterDetailElements());
}
public ParserRule getMasterDetailRule() {
return getMasterDetailAccess().getRule();
}
//Process:
// AutomatedProcess|InteractiveProcess;
public ProcessElements getProcessAccess() {
return (pProcess != null) ? pProcess : (pProcess = new ProcessElements());
}
public ParserRule getProcessRule() {
return getProcessAccess().getRule();
}
//AutomatedProcess:
// description=STRING? "AutomatedProcess" name=ID "using" contextRef=[ExternalReference
// ] ("steps" "[" processElements+=UICall* "]")?;
public AutomatedProcessElements getAutomatedProcessAccess() {
return (pAutomatedProcess != null) ? pAutomatedProcess : (pAutomatedProcess = new AutomatedProcessElements());
}
public ParserRule getAutomatedProcessRule() {
return getAutomatedProcessAccess().getRule();
}
//InteractiveProcess:
// description=STRING? "InteractiveProcess" name=ID "using" contextRef=[
// ExternalReference] ("steps" "[" processElements+=UICall* "]")?;
public InteractiveProcessElements getInteractiveProcessAccess() {
return (pInteractiveProcess != null) ? pInteractiveProcess : (pInteractiveProcess = new InteractiveProcessElements());
}
public ParserRule getInteractiveProcessRule() {
return getInteractiveProcessAccess().getRule();
}
//FormElement:
// "formElement" references=DtoPropertyReference "named" name=ID ("readonly" (readonly?=
// "true"|"false"))? ";";
//
//
//
//// ("navigate" LinkRef "to")?
public FormElementElements getFormElementAccess() {
return (pFormElement != null) ? pFormElement : (pFormElement = new FormElementElements());
}
public ParserRule getFormElementRule() {
return getFormElementAccess().getRule();
}
//DialogueCall:
// (DirectDialogueCall|LinkedDialogueCall) ("alias" alias=ID)? ";";
public DialogueCallElements getDialogueCallAccess() {
return (pDialogueCall != null) ? pDialogueCall : (pDialogueCall = new DialogueCallElements());
}
public ParserRule getDialogueCallRule() {
return getDialogueCallAccess().getRule();
}
//LinkedDialogueCall:
// "navigate" link=LinkRef "to" name=ID;
public LinkedDialogueCallElements getLinkedDialogueCallAccess() {
return (pLinkedDialogueCall != null) ? pLinkedDialogueCall : (pLinkedDialogueCall = new LinkedDialogueCallElements());
}
public ParserRule getLinkedDialogueCallRule() {
return getLinkedDialogueCallAccess().getRule();
}
//DirectDialogueCall:
// name=ID;
public DirectDialogueCallElements getDirectDialogueCallAccess() {
return (pDirectDialogueCall != null) ? pDirectDialogueCall : (pDirectDialogueCall = new DirectDialogueCallElements());
}
public ParserRule getDirectDialogueCallRule() {
return getDirectDialogueCallAccess().getRule();
}
//ProcessCall:
// (DirectProcessCall|LinkedProcessCall) ("alias" alias=ID)? ";";
public ProcessCallElements getProcessCallAccess() {
return (pProcessCall != null) ? pProcessCall : (pProcessCall = new ProcessCallElements());
}
public ParserRule getProcessCallRule() {
return getProcessCallAccess().getRule();
}
//LinkedProcessCall:
// "navigate" link=LinkRef "to" name=ID;
public LinkedProcessCallElements getLinkedProcessCallAccess() {
return (pLinkedProcessCall != null) ? pLinkedProcessCall : (pLinkedProcessCall = new LinkedProcessCallElements());
}
public ParserRule getLinkedProcessCallRule() {
return getLinkedProcessCallAccess().getRule();
}
//DirectProcessCall:
// name=ID;
public DirectProcessCallElements getDirectProcessCallAccess() {
return (pDirectProcessCall != null) ? pDirectProcessCall : (pDirectProcessCall = new DirectProcessCallElements());
}
public ParserRule getDirectProcessCallRule() {
return getDirectProcessCallAccess().getRule();
}
//LinkRef:
// name=ID;
public LinkRefElements getLinkRefAccess() {
return (pLinkRef != null) ? pLinkRef : (pLinkRef = new LinkRefElements());
}
public ParserRule getLinkRefRule() {
return getLinkRefAccess().getRule();
}
//Link:
// LinkStep|LinkPath;
public LinkElements getLinkAccess() {
return (pLink != null) ? pLink : (pLink = new LinkElements());
}
public ParserRule getLinkRule() {
return getLinkAccess().getRule();
}
//LinkStep:
// description=STRING? "LinkStep" name=ID "using" contextRef=[ExternalReference]
// "navigate" reference=AssociationRoleReference;
public LinkStepElements getLinkStepAccess() {
return (pLinkStep != null) ? pLinkStep : (pLinkStep = new LinkStepElements());
}
public ParserRule getLinkStepRule() {
return getLinkStepAccess().getRule();
}
//LinkPath:
// description=STRING? "LinkPath" name=ID "using" contextRef=[ExternalReference];
public LinkPathElements getLinkPathAccess() {
return (pLinkPath != null) ? pLinkPath : (pLinkPath = new LinkPathElements());
}
public ParserRule getLinkPathRule() {
return getLinkPathAccess().getRule();
}
//DtoPropertyReference:
// name=ID;
public DtoPropertyReferenceElements getDtoPropertyReferenceAccess() {
return (pDtoPropertyReference != null) ? pDtoPropertyReference : (pDtoPropertyReference = new DtoPropertyReferenceElements());
}
public ParserRule getDtoPropertyReferenceRule() {
return getDtoPropertyReferenceAccess().getRule();
}
//AssociationRoleReference:
// name=ID;
public AssociationRoleReferenceElements getAssociationRoleReferenceAccess() {
return (pAssociationRoleReference != null) ? pAssociationRoleReference : (pAssociationRoleReference = new AssociationRoleReferenceElements());
}
public ParserRule getAssociationRoleReferenceRule() {
return getAssociationRoleReferenceAccess().getRule();
}
//UICall:
// UIModelElementCall;
public UICallElements getUICallAccess() {
return (pUICall != null) ? pUICall : (pUICall = new UICallElements());
}
public ParserRule getUICallRule() {
return getUICallAccess().getRule();
}
//UIModelElementCall:
// "dialogue" DialogueCall|"process" ProcessCall;
public UIModelElementCallElements getUIModelElementCallAccess() {
return (pUIModelElementCall != null) ? pUIModelElementCall : (pUIModelElementCall = new UIModelElementCallElements());
}
public ParserRule getUIModelElementCallRule() {
return getUIModelElementCallAccess().getRule();
}
//terminal ID:
// "^"? ("a".."z" | "A".."Z" | "_") ("a".."z" | "A".."Z" | "_" | "0".."9")*;
public TerminalRule getIDRule() {
return gaTerminals.getIDRule();
}
//terminal INT returns ecore::EInt:
// "0".."9"+;
public TerminalRule getINTRule() {
return gaTerminals.getINTRule();
}
//terminal STRING:
// "\"" ("\\" ("b" | "t" | "n" | "f" | "r" | "\"" | "\'" | "\\") | !("\\" | "\""))* "\"" | "\'" ("\\" ("b" |
// "t" | "n" | "f" | "r" | "\"" | "\'" | "\\") | !("\\" | "\'"))* "\'";
public TerminalRule getSTRINGRule() {
return gaTerminals.getSTRINGRule();
}
//terminal ML_COMMENT:
// "/ *"->"* /";
public TerminalRule getML_COMMENTRule() {
return gaTerminals.getML_COMMENTRule();
}
//terminal SL_COMMENT:
// "//" !("\n" | "\r")* ("\r"? "\n")?;
public TerminalRule getSL_COMMENTRule() {
return gaTerminals.getSL_COMMENTRule();
}
//terminal WS:
// (" " | "\t" | "\r" | "\n")+;
public TerminalRule getWSRule() {
return gaTerminals.getWSRule();
}
//terminal ANY_OTHER:
// .;
public TerminalRule getANY_OTHERRule() {
return gaTerminals.getANY_OTHERRule();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy