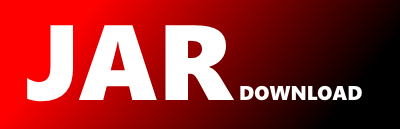
org.mod4j.runtime.validation.MinValueValidator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mod4j-runtime-common Show documentation
Show all versions of mod4j-runtime-common Show documentation
This module contains a small number of Java classes and Spring configuration files used in applications generated by
mod4j.
package org.mod4j.runtime.validation;
import org.springframework.util.Assert;
import org.springframework.validation.Errors;
import org.springframework.validation.Validator;
/**
* @author Eric Jan Malotaux
*
*/
public class MinValueValidator implements Validator {
private String field;
private long min;
private Class clazz;
/**
* @param field
* @param min
*/
public MinValueValidator(Class clazz, String field, long min) {
this.clazz = clazz;
this.field = field;
this.min = min;
}
@SuppressWarnings("unchecked")
public boolean supports(Class clazz) {
return this.clazz.isAssignableFrom(clazz);
}
public void validate(Object target, Errors errors) {
Long value = null;
if (errors.getFieldValue(field) instanceof Integer) {
value = ((Integer) errors.getFieldValue(field)).longValue();
} else if (errors.getFieldValue(field) instanceof Long) {
value = ((Long) errors.getFieldValue(field));
}
if (value != null) {
Assert.notNull(value);
if (value < min) {
errors.rejectValue(field, "field.value.min", new Long[] { new Long(min), new Long(value) }, field
+ " should be at least " + min + ", but was " + value);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy