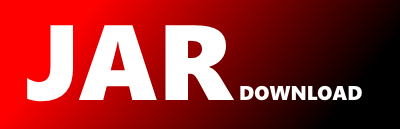
org.modelcc.language.syntax.InputSymbol Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelCC Show documentation
Show all versions of ModelCC Show documentation
ModelCC is a model-based parser generator (a.k.a. compiler compiler) that decouples language specification from language processing, avoiding some of the problems caused by grammar-driven parser generators. ModelCC receives a conceptual model as input, along with constraints that annotate it. It is then able to create a parser for the desired textual language and the generated parser fully automates the instantiation of the language conceptual model. ModelCC also includes a built-in reference resolution mechanism that results in abstract syntax graphs, rather than mere abstract syntax trees.
The newest version!
package org.modelcc.language.syntax;
/**
* Input symbol.
*
* @author Fernando Berzal ([email protected]))
*/
public abstract class InputSymbol
{
/**
* Type.
*/
private Object type;
/**
* Start index.
*/
private int startIndex;
/**
* Start index.
*/
private int endIndex;
/**
* User data.
*/
private Object userData;
// Constructor
public InputSymbol (Object type, int start, int end)
{
this.type = type;
this.startIndex = start;
this.endIndex = end;
}
// Getters
public Object getType()
{
return type;
}
public int getStartIndex()
{
return startIndex;
}
public int getEndIndex()
{
return endIndex;
}
// String
public abstract String getString ();
// User data
public Object getUserData()
{
return userData;
}
public void setUserData(Object userData)
{
this.userData = userData;
}
// Object methods
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final InputSymbol other = (InputSymbol) obj;
if (this.type != other.type && (this.type == null || !this.type.equals(other.type))) {
return false;
}
if (this.startIndex != other.startIndex) {
return false;
}
if (this.endIndex != other.endIndex) {
return false;
}
return true;
}
@Override
public int hashCode()
{
int hash = 7;
hash = 59 * hash + (this.type != null ? this.type.hashCode() : 0);
hash = 59 * hash + this.startIndex;
hash = 59 * hash + this.endIndex;
return hash;
}
@Override
public String toString()
{
return getType()+" ("+startIndex+"-"+endIndex+")";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy