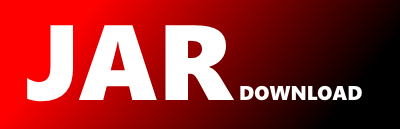
org.modelcc.language.syntax.SyntaxConstraints Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelCC Show documentation
Show all versions of ModelCC Show documentation
ModelCC is a model-based parser generator (a.k.a. compiler compiler) that decouples language specification from language processing, avoiding some of the problems caused by grammar-driven parser generators. ModelCC receives a conceptual model as input, along with constraints that annotate it. It is then able to create a parser for the desired textual language and the generated parser fully automates the instantiation of the language conceptual model. ModelCC also includes a built-in reference resolution mechanism that results in abstract syntax graphs, rather than mere abstract syntax trees.
The newest version!
/*
* ModelCC, distributed under ModelCC Shared Software License, www.modelcc.org
*/
package org.modelcc.language.syntax;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.modelcc.AssociativityType;
import org.modelcc.language.metamodel.LanguageModel;
/**
* Syntactic constraints.
*
* @author Luis Quesada ([email protected])
*/
public final class SyntaxConstraints implements Serializable
{
/**
* Language model
*/
private LanguageModel model;
/**
* Map of associativities.
*/
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy