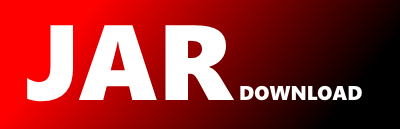
org.modelcc.lexer.LexerFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelCC Show documentation
Show all versions of ModelCC Show documentation
ModelCC is a model-based parser generator (a.k.a. compiler compiler) that decouples language specification from language processing, avoiding some of the problems caused by grammar-driven parser generators. ModelCC receives a conceptual model as input, along with constraints that annotate it. It is then able to create a parser for the desired textual language and the generated parser fully automates the instantiation of the language conceptual model. ModelCC also includes a built-in reference resolution mechanism that results in abstract syntax graphs, rather than mere abstract syntax trees.
The newest version!
/*
* ModelCC, distributed under ModelCC Shared Software License, www.modelcc.org
*/
package org.modelcc.lexer;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Set;
import org.modelcc.language.LanguageSpecification;
import org.modelcc.language.factory.LanguageSpecificationFactory;
import org.modelcc.language.lexis.LexicalSpecification;
import org.modelcc.language.lexis.TokenSpecification;
import org.modelcc.language.metamodel.SimpleLanguageElement;
import org.modelcc.language.metamodel.CompositeLanguageElement;
import org.modelcc.language.metamodel.LanguageModel;
import org.modelcc.language.metamodel.LanguageElement;
import org.modelcc.lexer.recognizer.PatternRecognizer;
/**
* ModelCC Parser Generator: Generic interface for lexers.
*
* @author Luis Quesada ([email protected]) & Fernando Berzal ([email protected])
*/
public abstract class LexerFactory implements Serializable
{
/**
* Creates a lexer
* @param lexis Lexical specification
* @return Lexer
* @throws LexerException
*/
public abstract Lexer createLexer (LexicalSpecification lexis) throws LexerException;
/**
* Creates a lexer
* @param m the model
* @return the created lexer
* @throws LexerException
*/
public final Lexer createLexer (LanguageModel m) throws LexerException
{
return createLexer(m, new HashSet());
}
/**
* Creates a lexer
* @param m the model
* @param skip the skip model.
* @return the created lexer
* @throws LexerException
*/
public final Lexer createLexer (LanguageModel m, LanguageModel skip) throws LexerException
{
Set skipSet = new HashSet();
if (skip != null)
fillSkipSet(skipSet,skip,skip.getStart());
return createLexer(m,skipSet);
}
/**
* Fills the skip set
* @param skipSet the skip set
* @param skip the skip model
* @param el the recursive model element
*/
private static void fillSkipSet (Set skipSet, LanguageModel skip, LanguageElement el) throws LexerException
{
if (el.getClass().equals(CompositeLanguageElement.class)) {
throw new LexerException("The skip model cannot contain composite elements such as "+el.getElementClass().getCanonicalName());
} else if (el.getClass().equals(SimpleLanguageElement.class)) {
skipSet.add(((SimpleLanguageElement)el).getPatternRecognizer());
} else {
for (LanguageElement element: skip.getSubelements().get(el))
fillSkipSet(skipSet,skip,element);
}
}
/**
* Creates a lexer
* @param m the model
* @param skip the skip set.
* @return the created lamb lexer
* @throws LexerException
*/
public final Lexer createLexer (LanguageModel m, Set skip) throws LexerException
{
try {
LanguageSpecificationFactory lsf = new LanguageSpecificationFactory();
LanguageSpecification language = lsf.create(m);
LexicalSpecification lexis = language.getLexicalSpecification();
if (skip != null)
for (PatternRecognizer recognizer: skip)
lexis.skipTokenSpecification(new TokenSpecification(null,recognizer));
return createLexer(lexis);
} catch (Exception e) {
throw new LexerException("Unable to create lexer", e);
}
}
/**
* Current lexer factory
*/
private static LexerFactory factory = new org.modelcc.lexer.lamb.LambLexerFactory();
// new org.modelcc.lexer.lamb.LambLexerFactory();
// new org.modelcc.lexer.flex.FlexLexerFactory();
public static LexerFactory getLexerFactory ()
{
return LexerFactory.factory;
}
public static void setLexerFactory (LexerFactory factory)
{
LexerFactory.factory = factory;
}
/**
* Creates a lexer (convenience method)
* @param m the model
* @return the created lexer
* @throws LexerException
*/
public static Lexer create(LanguageModel m) throws LexerException
{
return factory.createLexer(m);
}
/**
* Creates a lexer (convenience method)
* @param m the model
* @param skip the skip model.
* @return the created lexer
* @throws LexerException
*/
public static Lexer create (LanguageModel m, LanguageModel skip) throws LexerException
{
return factory.createLexer(m,skip);
}
/**
* Creates a lexer (convenience method)
* @param m the model
* @param skip the skip set.
* @return the created lexer
* @throws LexerException
*/
public static Lexer create (LanguageModel m, Set skip) throws LexerException
{
return factory.createLexer(m,skip);
}
/**
* Creates a lexer (convenience method)
* @param lexis the lexical specification
* @return the created lexer
* @throws LexerException
*/
public static Lexer create (LexicalSpecification lexis) throws LexerException
{
return factory.createLexer(lexis);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy