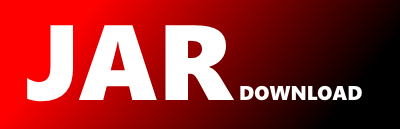
org.modelcc.lexer.recognizer.regexp.RegExps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelCC Show documentation
Show all versions of ModelCC Show documentation
ModelCC is a model-based parser generator (a.k.a. compiler compiler) that decouples language specification from language processing, avoiding some of the problems caused by grammar-driven parser generators. ModelCC receives a conceptual model as input, along with constraints that annotate it. It is then able to create a parser for the desired textual language and the generated parser fully automates the instantiation of the language conceptual model. ModelCC also includes a built-in reference resolution mechanism that results in abstract syntax graphs, rather than mere abstract syntax trees.
The newest version!
/*
* ModelCC, distributed under ModelCC Shared Software License, www.modelcc.org
*/
package org.modelcc.lexer.recognizer.regexp;
/**
* Common regular expressions.
*
* @author Luis Quesada ([email protected]) & Fernando Berzal ([email protected])
*/
public abstract class RegExps
{
/**
* Regular expression for double-precision floating-point numbers (in scientific notation), i.e. double.
*/
public static final String DOUBLE="(-|\\+)?[0-9]+(\\.[0-9]*)?((e|E)(|-|\\+)?[0-9]+)?";
/**
* Regular expression for simple-precision floating-point numbers (in scientific notation), i.e. float.
*/
public static final String FLOAT="(-|\\+)?[0-9]+(\\.[0-9]*)?((e|E)(|-|\\+)?[0-9]+)?";
/**
* Regular expression for long integers, i.e. long.
*/
public static final String LONG="(-|\\+)?[0-9]+";
/**
* Regular expression for integers, i.e. int.
*/
public static final String INT="(-|\\+)?[0-9]+";
/**
* Regular expression for short integers, i.e. short.
*/
public static final String SHORT="(-|\\+)?[0-9]+";
/**
* Regular expression for Boolean values.
*/
public static final String BOOLEAN="true|false";
/**
* Regular expression for Java comments.
*/
public static final String COMMENT="/\\*(?:.|[\\n\\r])*?\\*/";
/**
* Regular expression for single-line comments.
*/
public static final String SINGLE_LINE_COMMENT="(//.*)";
/**
* Tab regular expression.
*/
public static final String TAB="\\t";
/**
* Space regular expression.
*/
public static final String SPACE=" ";
/**
* New-line regular expression.
*/
public static final String NEW_LINE="\\n|\\r";
/**
* Whitespace regular expression.
*/
public static final String WHITESPACE="("+TAB+"|"+SPACE+"|"+NEW_LINE+")+";
/**
* Gets the regular expression of the specified Java primitive type.
* @param c Java primitive type.
* @return Regular expression of the corresponding Java primitive type.
*/
public static final String getRegularExpression (Class c)
{
if (c.equals(double.class)) return DOUBLE;
if (c.equals(Double.class)) return DOUBLE;
if (c.equals(float.class)) return FLOAT;
if (c.equals(Float.class)) return FLOAT;
if (c.equals(long.class)) return LONG;
if (c.equals(Long.class)) return LONG;
if (c.equals(int.class)) return INT;
if (c.equals(Integer.class)) return INT;
if (c.equals(short.class)) return SHORT;
if (c.equals(Short.class)) return SHORT;
if (c.equals(boolean.class)) return BOOLEAN;
if (c.equals(Boolean.class)) return BOOLEAN;
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy