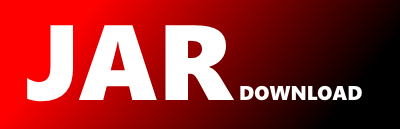
org.modelcc.parser.Parser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelCC Show documentation
Show all versions of ModelCC Show documentation
ModelCC is a model-based parser generator (a.k.a. compiler compiler) that decouples language specification from language processing, avoiding some of the problems caused by grammar-driven parser generators. ModelCC receives a conceptual model as input, along with constraints that annotate it. It is then able to create a parser for the desired textual language and the generated parser fully automates the instantiation of the language conceptual model. ModelCC also includes a built-in reference resolution mechanism that results in abstract syntax graphs, rather than mere abstract syntax trees.
The newest version!
/*
* ModelCC, distributed under ModelCC Shared Software License, www.modelcc.org
*/
package org.modelcc.parser;
import java.io.Reader;
import java.io.Serializable;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.modelcc.IModel;
/**
* ModelCC Parser
* @param the parser type
*
* @author Luis Quesada ([email protected]) & Fernando Berzal ([email protected])
*/
public abstract class Parser implements Serializable {
/**
* Parses an input string
* @param input the input string
* @return a parsed object
*/
public T parse(String input) throws ParserException {
return parse(new StringReader(input));
};
/**
* Parses an input reader
* @param input the input reader
* @return a parsed object
*/
public abstract T parse(Reader input) throws ParserException;
/**
* Parses an input string
* @param input the input string
* @return a collection of parsed objects
*/
public Collection parseAll(String input) throws ParserException {
return parseAll(new StringReader(input));
}
/**
* Parses an input reader
* @param input the input reader
* @return a collection of parsed objects
*/
public abstract Collection parseAll(Reader input) throws ParserException;
/**
* Parses an input string
* @param input the input string
* @return an iterator to the collection of parsed objects
*/
public Iterator parseIterator(String input) throws ParserException {
return parseIterator(new StringReader(input));
}
/**
* Parses an input reader
* @param input the input reader
* @return an iterator to the collection of parsed objects
*/
public abstract Iterator parseIterator(Reader input) throws ParserException;
/**
* Returns the parsing metadata for an object.
* @param object an object instantiated during the parsing.
* @return the parsing metadata.
*/
public abstract Map getParsingMetadata(Object object);
// Predefined references
// ---------------------
protected List predefined = new ArrayList();
/**
* Add predefined model element
*/
public final void add (IModel model)
{
predefined.add(model);
}
/**
* Remove predefined model element
*/
public final void remove (IModel model)
{
predefined.add(model);
}
/**
* Remove predefined model element
*/
public final void clear ()
{
predefined.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy