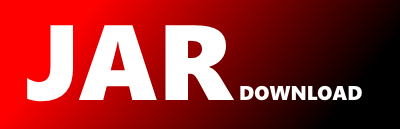
org.modelcc.parser.fence.FenceGrammarParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelCC Show documentation
Show all versions of ModelCC Show documentation
ModelCC is a model-based parser generator (a.k.a. compiler compiler) that decouples language specification from language processing, avoiding some of the problems caused by grammar-driven parser generators. ModelCC receives a conceptual model as input, along with constraints that annotate it. It is then able to create a parser for the desired textual language and the generated parser fully automates the instantiation of the language conceptual model. ModelCC also includes a built-in reference resolution mechanism that results in abstract syntax graphs, rather than mere abstract syntax trees.
The newest version!
/*
* ModelCC, distributed under ModelCC Shared Software License, www.modelcc.org
*/
package org.modelcc.parser.fence;
import java.io.Serializable;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.Stack;
import org.modelcc.language.syntax.Grammar;
import org.modelcc.language.syntax.Rule;
import org.modelcc.language.syntax.RuleSymbol;
import org.modelcc.language.syntax.InputSymbol;
import org.modelcc.lexer.LexicalGraph;
import org.modelcc.lexer.Token;
/**
* Fence Grammar Parser
*
* @author Luis Quesada ([email protected]), partially refactored by Fernando Berzal ([email protected])
*/
public final class FenceGrammarParser implements Serializable
{
/**
* The grammar.
*/
private Grammar g;
/**
* Lexical graph
*/
private LexicalGraph lg;
/**
* Parse graph
*/
private ParsedGraph pg;
// Handles & cores
/**
* Already generated handles.
*/
private Map> doneHandles;
/**
* Waiting handle pool.
*/
private Stack waitingHandlePool;
/**
* Cores.
*/
private Map>> cores;
/**
* Parse lexical graph
* @param g the grammar
* @param lg the lexical graph
* @return a parsed graph
*/
public ParsedGraph parse (Grammar g, LexicalGraph lg)
{
this.g = g;
this.lg = lg;
this.pg = new ParsedGraph(g,lg);
// Conversion to parse graph
// -------------------------
// Token -> ParsedSymbol correspondence map.
Map ttos = new HashMap();
// Generate correspondence map.
for (Token t: lg.getTokens()) {
ParsedSymbol s = new ParsedSymbol(t.getType(),t.getStartIndex(),t.getEndIndex(),t.getString());
s.setUserData(t.getUserData());
ttos.put(t,s);
pg.addSymbol(s);
}
// Complete preceding/following sets.
for (Token t: lg.getTokens()) {
ParsedSymbol s = ttos.get(t);
List prec = lg.getPreceding(t);
if (prec != null)
for (Token k: prec)
pg.addLink(ttos.get(k),s);
}
for (ParsedSymbol s: pg.getSymbols()) {
if (s.getType().equals(g.getStartType()))
if (s.getStartIndex()==lg.getInputStartIndex() && s.getEndIndex()==lg.getInputEndIndex())
pg.addStart(s);
// vs. LZ version: if (preceding.get(s) == null && following.get(s) == null)
}
// Parsing
// -------
WaitingHandle wh;
Object nextType;
int skip;
// Cores.
cores = new HashMap>>(256);
for (ParsedSymbol s: pg.getSymbols()) {
cores.put(s,new HashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy