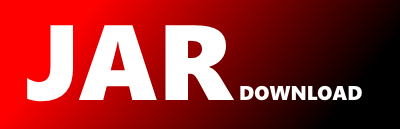
fUML.Semantics.Actions.BasicActions.ActionActivation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuml Show documentation
Show all versions of fuml Show documentation
This open source software is a reference implementation, consisting of software and related files, for the OMG specification called the Semantics of a Foundational Subset for Executable UML Models (fUML). The reference implementation is intended to implement the execution semantics of UML activity models, accepting an XMI file from a conformant UML model as its input and providing an execution trace of the selected activity model(s) as its output. The core execution engine, which is directly generated from the normative syntactic and semantic models for fUML, may also be used as a library implementation of fUML in other software.
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy