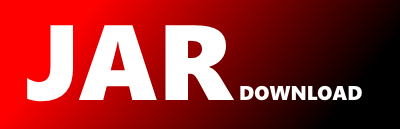
org.modeldriven.fuml.repository.model.UMLAssembler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuml Show documentation
Show all versions of fuml Show documentation
This open source software is a reference implementation, consisting of software and related files, for the OMG specification called the Semantics of a Foundational Subset for Executable UML Models (fUML). The reference implementation is intended to implement the execution semantics of UML activity models, accepting an XMI file from a conformant UML model as its input and providing an execution trace of the selected activity model(s) as its output. The core execution engine, which is directly generated from the normative syntactic and semantic models for fUML, may also be used as a library implementation of fUML in other software.
package org.modeldriven.fuml.repository.model;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import fuml.syntax.classification.Classifier;
import fuml.syntax.classification.Generalization;
import fuml.syntax.classification.Property;
import fuml.syntax.packages.Package;
import fuml.syntax.simpleclassifiers.Enumeration;
import fuml.syntax.simpleclassifiers.EnumerationLiteral;
import fuml.syntax.simpleclassifiers.PrimitiveType;
import fuml.syntax.structuredclassifiers.Association;
import fuml.syntax.structuredclassifiers.Class_;
import fuml.syntax.values.LiteralInteger;
import fuml.syntax.values.LiteralUnlimitedNatural;
import fuml.syntax.values.ValueSpecification;
import UMLPrimitiveTypes.UnlimitedNatural;
import org.modeldriven.fuml.repository.RepositoryArtifact;
import org.modeldriven.fuml.repository.RepositoryMapping;
import org.modeldriven.fuml.repository.Repository;
import org.modeldriven.fuml.repository.config.Artifact;
// this needs to read the artifact namespace info from the content
public class UMLAssembler extends ModelAssembler
implements RepositoryArtifact
{
private static Log log = LogFactory.getLog(UMLAssembler.class);
private ModelFactory factory;
public UMLAssembler(Artifact artifact, RepositoryMapping mapping, Repository model) {
super(artifact, mapping, model);
this.factory = new ModelFactory(mapping, model);
construct();
}
private void construct() {
log.info("initializing...");
constructPackages();
constructPrimitiveTypes();
constructEnumerations();
constructClasses();
constructProperties();
constructGeneralizations();
constructAssociations();
}
public String getURN() {
return this.artifact.getUrn();
}
public String getNamespaceURI() {
return this.artifact.getNamespaceURI();
}
private void constructPackages()
{
Package pkg = null;
// UML
pkg = factory.createPackage("UML", "UML", "_0", this); // root package
mapping.mapPackage(pkg, null, this);
// UML.Activities
pkg = factory.createPackage("Activities", "UML.Activities", "Activities", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.Values
pkg = factory.createPackage("Values", "UML.Values", "Values", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.UseCases
pkg = factory.createPackage("UseCases", "UML.UseCases", "UseCases", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.StructuredClassifiers
pkg = factory.createPackage("StructuredClassifiers", "UML.StructuredClassifiers", "StructuredClassifiers", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.StateMachines
pkg = factory.createPackage("StateMachines", "UML.StateMachines", "StateMachines", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.SimpleClassifiers
pkg = factory.createPackage("SimpleClassifiers", "UML.SimpleClassifiers", "SimpleClassifiers", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.Packages
pkg = factory.createPackage("Packages", "UML.Packages", "Packages", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.Interactions
pkg = factory.createPackage("Interactions", "UML.Interactions", "Interactions", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.InformationFlows
pkg = factory.createPackage("InformationFlows", "UML.InformationFlows", "InformationFlows", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.Deployments
pkg = factory.createPackage("Deployments", "UML.Deployments", "Deployments", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.CommonStructure
pkg = factory.createPackage("CommonStructure", "UML.CommonStructure", "CommonStructure", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.CommonBehavior
pkg = factory.createPackage("CommonBehavior", "UML.CommonBehavior", "CommonBehavior", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.Classification
pkg = factory.createPackage("Classification", "UML.Classification", "Classification", pkg, this);
mapping.mapPackage(pkg, "UML", this);
// UML.Actions
pkg = factory.createPackage("Actions", "UML.Actions", "Actions", pkg, this);
mapping.mapPackage(pkg, "UML", this);
}
private void constructPrimitiveTypes()
{
Package pkg = null;
String packageId = null;
PrimitiveType type = null;
}
private void constructClasses()
{
Package pkg = null;
String packageId = null;
Class_ clss = null;
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.Activity
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("Activity", "Activity", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ActivityEdge
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ActivityEdge", "ActivityEdge", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ActivityFinalNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ActivityFinalNode", "ActivityFinalNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ActivityGroup
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ActivityGroup", "ActivityGroup", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ActivityNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ActivityNode", "ActivityNode", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ActivityParameterNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ActivityParameterNode", "ActivityParameterNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ActivityPartition
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ActivityPartition", "ActivityPartition", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.CentralBufferNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("CentralBufferNode", "CentralBufferNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ControlFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ControlFlow", "ControlFlow", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ControlNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ControlNode", "ControlNode", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.DataStoreNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("DataStoreNode", "DataStoreNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.DecisionNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("DecisionNode", "DecisionNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ExceptionHandler
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ExceptionHandler", "ExceptionHandler", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ExecutableNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ExecutableNode", "ExecutableNode", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.FinalNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("FinalNode", "FinalNode", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.FlowFinalNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("FlowFinalNode", "FlowFinalNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ForkNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ForkNode", "ForkNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.InitialNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("InitialNode", "InitialNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.InterruptibleActivityRegion
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("InterruptibleActivityRegion", "InterruptibleActivityRegion", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.JoinNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("JoinNode", "JoinNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.MergeNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("MergeNode", "MergeNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ObjectFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ObjectFlow", "ObjectFlow", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.ObjectNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("ObjectNode", "ObjectNode", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.Variable
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Activities").getDelegate();
clss = factory.createClass("Variable", "Variable", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Activities", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.Duration
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("Duration", "Duration", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.DurationConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("DurationConstraint", "DurationConstraint", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.DurationInterval
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("DurationInterval", "DurationInterval", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.DurationObservation
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("DurationObservation", "DurationObservation", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.Expression
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("Expression", "Expression", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.Interval
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("Interval", "Interval", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.IntervalConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("IntervalConstraint", "IntervalConstraint", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralBoolean
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralBoolean", "LiteralBoolean", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralInteger
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralInteger", "LiteralInteger", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralNull
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralNull", "LiteralNull", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralReal
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralReal", "LiteralReal", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralSpecification", "LiteralSpecification", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralString
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralString", "LiteralString", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.LiteralUnlimitedNatural
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("LiteralUnlimitedNatural", "LiteralUnlimitedNatural", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.Observation
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("Observation", "Observation", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.OpaqueExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("OpaqueExpression", "OpaqueExpression", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.StringExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("StringExpression", "StringExpression", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.TimeConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("TimeConstraint", "TimeConstraint", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.TimeExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("TimeExpression", "TimeExpression", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.TimeInterval
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("TimeInterval", "TimeInterval", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.TimeObservation
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("TimeObservation", "TimeObservation", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.ValueSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Values").getDelegate();
clss = factory.createClass("ValueSpecification", "ValueSpecification", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Values", this);
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.Actor
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.UseCases").getDelegate();
clss = factory.createClass("Actor", "Actor", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.UseCases", this);
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.Extend
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.UseCases").getDelegate();
clss = factory.createClass("Extend", "Extend", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.UseCases", this);
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.ExtensionPoint
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.UseCases").getDelegate();
clss = factory.createClass("ExtensionPoint", "ExtensionPoint", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.UseCases", this);
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.Include
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.UseCases").getDelegate();
clss = factory.createClass("Include", "Include", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.UseCases", this);
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.UseCase
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.UseCases").getDelegate();
clss = factory.createClass("UseCase", "UseCase", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.UseCases", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.Association
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("Association", "Association", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.AssociationClass
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("AssociationClass", "AssociationClass", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.Class
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("Class", "Class", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.Collaboration
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("Collaboration", "Collaboration", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.CollaborationUse
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("CollaborationUse", "CollaborationUse", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.Component
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("Component", "Component", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.ComponentRealization
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("ComponentRealization", "ComponentRealization", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.ConnectableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("ConnectableElement", "ConnectableElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.ConnectableElementTemplateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("ConnectableElementTemplateParameter", "ConnectableElementTemplateParameter", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.Connector
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("Connector", "Connector", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.ConnectorEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("ConnectorEnd", "ConnectorEnd", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.EncapsulatedClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("EncapsulatedClassifier", "EncapsulatedClassifier", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.Port
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("Port", "Port", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.StructuredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StructuredClassifiers").getDelegate();
clss = factory.createClass("StructuredClassifier", "StructuredClassifier", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.StructuredClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.ConnectionPointReference
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("ConnectionPointReference", "ConnectionPointReference", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.FinalState
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("FinalState", "FinalState", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.ProtocolConformance
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("ProtocolConformance", "ProtocolConformance", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.ProtocolStateMachine
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("ProtocolStateMachine", "ProtocolStateMachine", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.ProtocolTransition
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("ProtocolTransition", "ProtocolTransition", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.Pseudostate
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("Pseudostate", "Pseudostate", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.Region
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("Region", "Region", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.State
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("State", "State", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.StateMachine
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("StateMachine", "StateMachine", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.Transition
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("Transition", "Transition", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.Vertex
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.StateMachines").getDelegate();
clss = factory.createClass("Vertex", "Vertex", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.StateMachines", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.BehavioredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("BehavioredClassifier", "BehavioredClassifier", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.DataType
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("DataType", "DataType", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.Enumeration
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("Enumeration", "Enumeration", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.EnumerationLiteral
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("EnumerationLiteral", "EnumerationLiteral", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.Interface
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("Interface", "Interface", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.InterfaceRealization
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("InterfaceRealization", "InterfaceRealization", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.PrimitiveType
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("PrimitiveType", "PrimitiveType", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.Reception
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("Reception", "Reception", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.Signal
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.SimpleClassifiers").getDelegate();
clss = factory.createClass("Signal", "Signal", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.SimpleClassifiers", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.Extension
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("Extension", "Extension", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.ExtensionEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("ExtensionEnd", "ExtensionEnd", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.Image
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("Image", "Image", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.Model
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("Model", "Model", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.Package
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("Package", "Package", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.PackageMerge
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("PackageMerge", "PackageMerge", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.Profile
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("Profile", "Profile", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.ProfileApplication
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("ProfileApplication", "ProfileApplication", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.Stereotype
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Packages").getDelegate();
clss = factory.createClass("Stereotype", "Stereotype", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Packages", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.ActionExecutionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("ActionExecutionSpecification", "ActionExecutionSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.BehaviorExecutionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("BehaviorExecutionSpecification", "BehaviorExecutionSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.CombinedFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("CombinedFragment", "CombinedFragment", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.ConsiderIgnoreFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("ConsiderIgnoreFragment", "ConsiderIgnoreFragment", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.Continuation
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("Continuation", "Continuation", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.DestructionOccurrenceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("DestructionOccurrenceSpecification", "DestructionOccurrenceSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.ExecutionOccurrenceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("ExecutionOccurrenceSpecification", "ExecutionOccurrenceSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.ExecutionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("ExecutionSpecification", "ExecutionSpecification", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.Gate
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("Gate", "Gate", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.GeneralOrdering
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("GeneralOrdering", "GeneralOrdering", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.Interaction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("Interaction", "Interaction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.InteractionConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("InteractionConstraint", "InteractionConstraint", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.InteractionFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("InteractionFragment", "InteractionFragment", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.InteractionOperand
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("InteractionOperand", "InteractionOperand", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.InteractionUse
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("InteractionUse", "InteractionUse", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.Lifeline
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("Lifeline", "Lifeline", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.Message
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("Message", "Message", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.MessageEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("MessageEnd", "MessageEnd", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.MessageOccurrenceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("MessageOccurrenceSpecification", "MessageOccurrenceSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.OccurrenceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("OccurrenceSpecification", "OccurrenceSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.PartDecomposition
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("PartDecomposition", "PartDecomposition", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.StateInvariant
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Interactions").getDelegate();
clss = factory.createClass("StateInvariant", "StateInvariant", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Interactions", this);
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.InformationFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.InformationFlows").getDelegate();
clss = factory.createClass("InformationFlow", "InformationFlow", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.InformationFlows", this);
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.InformationItem
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.InformationFlows").getDelegate();
clss = factory.createClass("InformationItem", "InformationItem", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.InformationFlows", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.Artifact
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("Artifact", "Artifact", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.CommunicationPath
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("CommunicationPath", "CommunicationPath", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.DeployedArtifact
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("DeployedArtifact", "DeployedArtifact", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.Deployment
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("Deployment", "Deployment", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.DeploymentSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("DeploymentSpecification", "DeploymentSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.DeploymentTarget
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("DeploymentTarget", "DeploymentTarget", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.Device
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("Device", "Device", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.ExecutionEnvironment
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("ExecutionEnvironment", "ExecutionEnvironment", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.Manifestation
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("Manifestation", "Manifestation", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.Node
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Deployments").getDelegate();
clss = factory.createClass("Node", "Node", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Deployments", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Abstraction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Abstraction", "Abstraction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Comment
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Comment", "Comment", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Constraint
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Constraint", "Constraint", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Dependency
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Dependency", "Dependency", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.DirectedRelationship
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("DirectedRelationship", "DirectedRelationship", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Element
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Element", "Element", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.ElementImport
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("ElementImport", "ElementImport", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.MultiplicityElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("MultiplicityElement", "MultiplicityElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.NamedElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("NamedElement", "NamedElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Namespace
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Namespace", "Namespace", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.PackageableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("PackageableElement", "PackageableElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.PackageImport
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("PackageImport", "PackageImport", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.ParameterableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("ParameterableElement", "ParameterableElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Realization
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Realization", "Realization", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Relationship
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Relationship", "Relationship", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.TemplateableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("TemplateableElement", "TemplateableElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.TemplateBinding
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("TemplateBinding", "TemplateBinding", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.TemplateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("TemplateParameter", "TemplateParameter", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.TemplateParameterSubstitution
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("TemplateParameterSubstitution", "TemplateParameterSubstitution", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.TemplateSignature
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("TemplateSignature", "TemplateSignature", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Type
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Type", "Type", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.TypedElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("TypedElement", "TypedElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.Usage
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonStructure").getDelegate();
clss = factory.createClass("Usage", "Usage", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonStructure", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.AnyReceiveEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("AnyReceiveEvent", "AnyReceiveEvent", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.Behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("Behavior", "Behavior", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.CallEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("CallEvent", "CallEvent", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.ChangeEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("ChangeEvent", "ChangeEvent", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.Event
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("Event", "Event", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.FunctionBehavior
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("FunctionBehavior", "FunctionBehavior", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.MessageEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("MessageEvent", "MessageEvent", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.OpaqueBehavior
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("OpaqueBehavior", "OpaqueBehavior", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.SignalEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("SignalEvent", "SignalEvent", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.TimeEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("TimeEvent", "TimeEvent", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.Trigger
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.CommonBehavior").getDelegate();
clss = factory.createClass("Trigger", "Trigger", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.CommonBehavior", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Substitution
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Substitution", "Substitution", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.BehavioralFeature
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("BehavioralFeature", "BehavioralFeature", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Classifier", "Classifier", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.ClassifierTemplateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("ClassifierTemplateParameter", "ClassifierTemplateParameter", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Feature
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Feature", "Feature", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Generalization
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Generalization", "Generalization", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.GeneralizationSet
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("GeneralizationSet", "GeneralizationSet", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.InstanceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("InstanceSpecification", "InstanceSpecification", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.InstanceValue
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("InstanceValue", "InstanceValue", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Operation
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Operation", "Operation", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.OperationTemplateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("OperationTemplateParameter", "OperationTemplateParameter", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Parameter
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Parameter", "Parameter", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.ParameterSet
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("ParameterSet", "ParameterSet", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Property
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Property", "Property", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.RedefinableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("RedefinableElement", "RedefinableElement", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.RedefinableTemplateSignature
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("RedefinableTemplateSignature", "RedefinableTemplateSignature", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.Slot
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("Slot", "Slot", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.StructuralFeature
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Classification").getDelegate();
clss = factory.createClass("StructuralFeature", "StructuralFeature", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Classification", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ValueSpecificationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ValueSpecificationAction", "ValueSpecificationAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.VariableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("VariableAction", "VariableAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.WriteLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("WriteLinkAction", "WriteLinkAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.WriteStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("WriteStructuralFeatureAction", "WriteStructuralFeatureAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.WriteVariableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("WriteVariableAction", "WriteVariableAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.AcceptCallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("AcceptCallAction", "AcceptCallAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.AcceptEventAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("AcceptEventAction", "AcceptEventAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.Action
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("Action", "Action", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ActionInputPin
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ActionInputPin", "ActionInputPin", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.AddStructuralFeatureValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("AddStructuralFeatureValueAction", "AddStructuralFeatureValueAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.AddVariableValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("AddVariableValueAction", "AddVariableValueAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.BroadcastSignalAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("BroadcastSignalAction", "BroadcastSignalAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.CallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("CallAction", "CallAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.CallBehaviorAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("CallBehaviorAction", "CallBehaviorAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.CallOperationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("CallOperationAction", "CallOperationAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.Clause
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("Clause", "Clause", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ClearAssociationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ClearAssociationAction", "ClearAssociationAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ClearStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ClearStructuralFeatureAction", "ClearStructuralFeatureAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ClearVariableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ClearVariableAction", "ClearVariableAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ConditionalNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ConditionalNode", "ConditionalNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.CreateLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("CreateLinkAction", "CreateLinkAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.CreateLinkObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("CreateLinkObjectAction", "CreateLinkObjectAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.CreateObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("CreateObjectAction", "CreateObjectAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.DestroyLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("DestroyLinkAction", "DestroyLinkAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.DestroyObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("DestroyObjectAction", "DestroyObjectAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ExpansionNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ExpansionNode", "ExpansionNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ExpansionRegion
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ExpansionRegion", "ExpansionRegion", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.InputPin
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("InputPin", "InputPin", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.InvocationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("InvocationAction", "InvocationAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.LinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("LinkAction", "LinkAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.LinkEndCreationData
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("LinkEndCreationData", "LinkEndCreationData", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.LinkEndData
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("LinkEndData", "LinkEndData", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.LinkEndDestructionData
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("LinkEndDestructionData", "LinkEndDestructionData", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.LoopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("LoopNode", "LoopNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.OpaqueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("OpaqueAction", "OpaqueAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.OutputPin
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("OutputPin", "OutputPin", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.Pin
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("Pin", "Pin", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.QualifierValue
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("QualifierValue", "QualifierValue", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.RaiseExceptionAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("RaiseExceptionAction", "RaiseExceptionAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadExtentAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadExtentAction", "ReadExtentAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadIsClassifiedObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadIsClassifiedObjectAction", "ReadIsClassifiedObjectAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadLinkAction", "ReadLinkAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadLinkObjectEndAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadLinkObjectEndAction", "ReadLinkObjectEndAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadLinkObjectEndQualifierAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadLinkObjectEndQualifierAction", "ReadLinkObjectEndQualifierAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadSelfAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadSelfAction", "ReadSelfAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadStructuralFeatureAction", "ReadStructuralFeatureAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReadVariableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReadVariableAction", "ReadVariableAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReclassifyObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReclassifyObjectAction", "ReclassifyObjectAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReduceAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReduceAction", "ReduceAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.RemoveStructuralFeatureValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("RemoveStructuralFeatureValueAction", "RemoveStructuralFeatureValueAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.RemoveVariableValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("RemoveVariableValueAction", "RemoveVariableValueAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ReplyAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ReplyAction", "ReplyAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.SendObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("SendObjectAction", "SendObjectAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.SendSignalAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("SendSignalAction", "SendSignalAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.SequenceNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("SequenceNode", "SequenceNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.StartClassifierBehaviorAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("StartClassifierBehaviorAction", "StartClassifierBehaviorAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.StartObjectBehaviorAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("StartObjectBehaviorAction", "StartObjectBehaviorAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.StructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("StructuralFeatureAction", "StructuralFeatureAction", pkg);
clss.isAbstract = true;
((Classifier)clss).isAbstract = true;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.StructuredActivityNode
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("StructuredActivityNode", "StructuredActivityNode", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.TestIdentityAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("TestIdentityAction", "TestIdentityAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.UnmarshallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("UnmarshallAction", "UnmarshallAction", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.ValuePin
pkg = (Package)model.getElementById(packageId).getDelegate();
//pkg = model.getPackageByQualifiedName("UML.Actions").getDelegate();
clss = factory.createClass("ValuePin", "ValuePin", pkg);
clss.isAbstract = false;
((Classifier)clss).isAbstract = false;
mapping.mapClass(clss, "UML.Actions", this);
}
private void constructEnumerations()
{
Enumeration enumeration = null;
EnumerationLiteral literal = null;
// ObjectNodeOrderingKind
enumeration = factory.createEnumeration("ObjectNodeOrderingKind", "ObjectNodeOrderingKind");
mapping.mapEnumeration(enumeration, "UML.Activities", this);
literal = factory.createEnumerationLiteral(enumeration,
"unordered",
"ObjectNodeOrderingKind-unordered");
mapping.mapEnumerationLiteral(literal, "UML.Activities", this);
literal = factory.createEnumerationLiteral(enumeration,
"ordered",
"ObjectNodeOrderingKind-ordered");
mapping.mapEnumerationLiteral(literal, "UML.Activities", this);
literal = factory.createEnumerationLiteral(enumeration,
"LIFO",
"ObjectNodeOrderingKind-LIFO");
mapping.mapEnumerationLiteral(literal, "UML.Activities", this);
literal = factory.createEnumerationLiteral(enumeration,
"FIFO",
"ObjectNodeOrderingKind-FIFO");
mapping.mapEnumerationLiteral(literal, "UML.Activities", this);
// ConnectorKind
enumeration = factory.createEnumeration("ConnectorKind", "ConnectorKind");
mapping.mapEnumeration(enumeration, "UML.StructuredClassifiers", this);
literal = factory.createEnumerationLiteral(enumeration,
"assembly",
"ConnectorKind-assembly");
mapping.mapEnumerationLiteral(literal, "UML.StructuredClassifiers", this);
literal = factory.createEnumerationLiteral(enumeration,
"delegation",
"ConnectorKind-delegation");
mapping.mapEnumerationLiteral(literal, "UML.StructuredClassifiers", this);
// PseudostateKind
enumeration = factory.createEnumeration("PseudostateKind", "PseudostateKind");
mapping.mapEnumeration(enumeration, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"initial",
"PseudostateKind-initial");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"deepHistory",
"PseudostateKind-deepHistory");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"shallowHistory",
"PseudostateKind-shallowHistory");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"join",
"PseudostateKind-join");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"fork",
"PseudostateKind-fork");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"junction",
"PseudostateKind-junction");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"choice",
"PseudostateKind-choice");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"entryPoint",
"PseudostateKind-entryPoint");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"exitPoint",
"PseudostateKind-exitPoint");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"terminate",
"PseudostateKind-terminate");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
// TransitionKind
enumeration = factory.createEnumeration("TransitionKind", "TransitionKind");
mapping.mapEnumeration(enumeration, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"internal",
"TransitionKind-internal");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"local",
"TransitionKind-local");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
literal = factory.createEnumerationLiteral(enumeration,
"external",
"TransitionKind-external");
mapping.mapEnumerationLiteral(literal, "UML.StateMachines", this);
// InteractionOperatorKind
enumeration = factory.createEnumeration("InteractionOperatorKind", "InteractionOperatorKind");
mapping.mapEnumeration(enumeration, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"seq",
"InteractionOperatorKind-seq");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"alt",
"InteractionOperatorKind-alt");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"opt",
"InteractionOperatorKind-opt");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"break",
"InteractionOperatorKind-break");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"par",
"InteractionOperatorKind-par");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"strict",
"InteractionOperatorKind-strict");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"loop",
"InteractionOperatorKind-loop");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"critical",
"InteractionOperatorKind-critical");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"neg",
"InteractionOperatorKind-neg");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"assert",
"InteractionOperatorKind-assert");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"ignore",
"InteractionOperatorKind-ignore");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"consider",
"InteractionOperatorKind-consider");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
// MessageKind
enumeration = factory.createEnumeration("MessageKind", "MessageKind");
mapping.mapEnumeration(enumeration, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"complete",
"MessageKind-complete");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"lost",
"MessageKind-lost");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"found",
"MessageKind-found");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"unknown",
"MessageKind-unknown");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
// MessageSort
enumeration = factory.createEnumeration("MessageSort", "MessageSort");
mapping.mapEnumeration(enumeration, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"synchCall",
"MessageSort-synchCall");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"asynchCall",
"MessageSort-asynchCall");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"asynchSignal",
"MessageSort-asynchSignal");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"createMessage",
"MessageSort-createMessage");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"deleteMessage",
"MessageSort-deleteMessage");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
literal = factory.createEnumerationLiteral(enumeration,
"reply",
"MessageSort-reply");
mapping.mapEnumerationLiteral(literal, "UML.Interactions", this);
// VisibilityKind
enumeration = factory.createEnumeration("VisibilityKind", "VisibilityKind");
mapping.mapEnumeration(enumeration, "UML.CommonStructure", this);
literal = factory.createEnumerationLiteral(enumeration,
"public",
"VisibilityKind-public");
mapping.mapEnumerationLiteral(literal, "UML.CommonStructure", this);
literal = factory.createEnumerationLiteral(enumeration,
"private",
"VisibilityKind-private");
mapping.mapEnumerationLiteral(literal, "UML.CommonStructure", this);
literal = factory.createEnumerationLiteral(enumeration,
"protected",
"VisibilityKind-protected");
mapping.mapEnumerationLiteral(literal, "UML.CommonStructure", this);
literal = factory.createEnumerationLiteral(enumeration,
"package",
"VisibilityKind-package");
mapping.mapEnumerationLiteral(literal, "UML.CommonStructure", this);
// AggregationKind
enumeration = factory.createEnumeration("AggregationKind", "AggregationKind");
mapping.mapEnumeration(enumeration, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"none",
"AggregationKind-none");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"shared",
"AggregationKind-shared");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"composite",
"AggregationKind-composite");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
// CallConcurrencyKind
enumeration = factory.createEnumeration("CallConcurrencyKind", "CallConcurrencyKind");
mapping.mapEnumeration(enumeration, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"sequential",
"CallConcurrencyKind-sequential");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"guarded",
"CallConcurrencyKind-guarded");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"concurrent",
"CallConcurrencyKind-concurrent");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
// ParameterDirectionKind
enumeration = factory.createEnumeration("ParameterDirectionKind", "ParameterDirectionKind");
mapping.mapEnumeration(enumeration, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"in",
"ParameterDirectionKind-in");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"inout",
"ParameterDirectionKind-inout");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"out",
"ParameterDirectionKind-out");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"return",
"ParameterDirectionKind-return");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
// ParameterEffectKind
enumeration = factory.createEnumeration("ParameterEffectKind", "ParameterEffectKind");
mapping.mapEnumeration(enumeration, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"create",
"ParameterEffectKind-create");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"read",
"ParameterEffectKind-read");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"update",
"ParameterEffectKind-update");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
literal = factory.createEnumerationLiteral(enumeration,
"delete",
"ParameterEffectKind-delete");
mapping.mapEnumerationLiteral(literal, "UML.Classification", this);
// ExpansionKind
enumeration = factory.createEnumeration("ExpansionKind", "ExpansionKind");
mapping.mapEnumeration(enumeration, "UML.Actions", this);
literal = factory.createEnumerationLiteral(enumeration,
"parallel",
"ExpansionKind-parallel");
mapping.mapEnumerationLiteral(literal, "UML.Actions", this);
literal = factory.createEnumerationLiteral(enumeration,
"iterative",
"ExpansionKind-iterative");
mapping.mapEnumerationLiteral(literal, "UML.Actions", this);
literal = factory.createEnumerationLiteral(enumeration,
"stream",
"ExpansionKind-stream");
mapping.mapEnumerationLiteral(literal, "UML.Actions", this);
}
private void constructProperties()
{
Class_ clss = null;
Property prop = null;
// Activity
clss = (Class_)model.getElementById("Activity").getDelegate();
prop = factory.createProperty(clss, "edge",
"Activity-edge",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "group",
"Activity-group",
"ActivityGroup",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isReadOnly",
"Activity-isReadOnly",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Activity-isReadOnly-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isSingleExecution",
"Activity-isSingleExecution",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Activity-isSingleExecution-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "node",
"Activity-node",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "partition",
"Activity-partition",
"ActivityPartition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "structuredNode",
"Activity-structuredNode",
"StructuredActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "variable",
"Activity-variable",
"Variable",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ActivityEdge
clss = (Class_)model.getElementById("ActivityEdge").getDelegate();
prop = factory.createProperty(clss, "activity",
"ActivityEdge-activity",
"Activity",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "guard",
"ActivityEdge-guard",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inGroup",
"ActivityEdge-inGroup",
"ActivityGroup",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inPartition",
"ActivityEdge-inPartition",
"ActivityPartition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inStructuredNode",
"ActivityEdge-inStructuredNode",
"StructuredActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interrupts",
"ActivityEdge-interrupts",
"InterruptibleActivityRegion",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedEdge",
"ActivityEdge-redefinedEdge",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "source",
"ActivityEdge-source",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "target",
"ActivityEdge-target",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "weight",
"ActivityEdge-weight",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ActivityFinalNode
clss = (Class_)model.getElementById("ActivityFinalNode").getDelegate();
// ActivityGroup
clss = (Class_)model.getElementById("ActivityGroup").getDelegate();
prop = factory.createProperty(clss, "containedEdge",
"ActivityGroup-containedEdge",
"ActivityEdge",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "containedNode",
"ActivityGroup-containedNode",
"ActivityNode",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inActivity",
"ActivityGroup-inActivity",
"Activity",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "subgroup",
"ActivityGroup-subgroup",
"ActivityGroup",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "superGroup",
"ActivityGroup-superGroup",
"ActivityGroup",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ActivityNode
clss = (Class_)model.getElementById("ActivityNode").getDelegate();
prop = factory.createProperty(clss, "activity",
"ActivityNode-activity",
"Activity",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inGroup",
"ActivityNode-inGroup",
"ActivityGroup",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inInterruptibleRegion",
"ActivityNode-inInterruptibleRegion",
"InterruptibleActivityRegion",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inPartition",
"ActivityNode-inPartition",
"ActivityPartition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inStructuredNode",
"ActivityNode-inStructuredNode",
"StructuredActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "incoming",
"ActivityNode-incoming",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "outgoing",
"ActivityNode-outgoing",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedNode",
"ActivityNode-redefinedNode",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ActivityParameterNode
clss = (Class_)model.getElementById("ActivityParameterNode").getDelegate();
prop = factory.createProperty(clss, "parameter",
"ActivityParameterNode-parameter",
"Parameter",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ActivityPartition
clss = (Class_)model.getElementById("ActivityPartition").getDelegate();
prop = factory.createProperty(clss, "edge",
"ActivityPartition-edge",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isDimension",
"ActivityPartition-isDimension",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ActivityPartition-isDimension-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isExternal",
"ActivityPartition-isExternal",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ActivityPartition-isExternal-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "node",
"ActivityPartition-node",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "represents",
"ActivityPartition-represents",
"Element",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "subpartition",
"ActivityPartition-subpartition",
"ActivityPartition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "superPartition",
"ActivityPartition-superPartition",
"ActivityPartition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// CentralBufferNode
clss = (Class_)model.getElementById("CentralBufferNode").getDelegate();
// ControlFlow
clss = (Class_)model.getElementById("ControlFlow").getDelegate();
// ControlNode
clss = (Class_)model.getElementById("ControlNode").getDelegate();
// DataStoreNode
clss = (Class_)model.getElementById("DataStoreNode").getDelegate();
// DecisionNode
clss = (Class_)model.getElementById("DecisionNode").getDelegate();
prop = factory.createProperty(clss, "decisionInput",
"DecisionNode-decisionInput",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "decisionInputFlow",
"DecisionNode-decisionInputFlow",
"ObjectFlow",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ExceptionHandler
clss = (Class_)model.getElementById("ExceptionHandler").getDelegate();
prop = factory.createProperty(clss, "exceptionInput",
"ExceptionHandler-exceptionInput",
"ObjectNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "exceptionType",
"ExceptionHandler-exceptionType",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "handlerBody",
"ExceptionHandler-handlerBody",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "protectedNode",
"ExceptionHandler-protectedNode",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ExecutableNode
clss = (Class_)model.getElementById("ExecutableNode").getDelegate();
prop = factory.createProperty(clss, "handler",
"ExecutableNode-handler",
"ExceptionHandler",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// FinalNode
clss = (Class_)model.getElementById("FinalNode").getDelegate();
// FlowFinalNode
clss = (Class_)model.getElementById("FlowFinalNode").getDelegate();
// ForkNode
clss = (Class_)model.getElementById("ForkNode").getDelegate();
// InitialNode
clss = (Class_)model.getElementById("InitialNode").getDelegate();
// InterruptibleActivityRegion
clss = (Class_)model.getElementById("InterruptibleActivityRegion").getDelegate();
prop = factory.createProperty(clss, "interruptingEdge",
"InterruptibleActivityRegion-interruptingEdge",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "node",
"InterruptibleActivityRegion-node",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// JoinNode
clss = (Class_)model.getElementById("JoinNode").getDelegate();
prop = factory.createProperty(clss, "isCombineDuplicate",
"JoinNode-isCombineDuplicate",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"JoinNode-isCombineDuplicate-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "joinSpec",
"JoinNode-joinSpec",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// MergeNode
clss = (Class_)model.getElementById("MergeNode").getDelegate();
// ObjectFlow
clss = (Class_)model.getElementById("ObjectFlow").getDelegate();
prop = factory.createProperty(clss, "isMulticast",
"ObjectFlow-isMulticast",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ObjectFlow-isMulticast-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isMultireceive",
"ObjectFlow-isMultireceive",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ObjectFlow-isMultireceive-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "selection",
"ObjectFlow-selection",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "transformation",
"ObjectFlow-transformation",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ObjectNode
clss = (Class_)model.getElementById("ObjectNode").getDelegate();
prop = factory.createProperty(clss, "inState",
"ObjectNode-inState",
"State",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isControlType",
"ObjectNode-isControlType",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ObjectNode-isControlType-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "ordering",
"ObjectNode-ordering",
"ObjectNodeOrderingKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"ObjectNodeOrderingKind-FIFO",
"ObjectNode-ordering-_defaultValue",
"uml:InstanceValue",
"ObjectNodeOrderingKind");
prop = factory.createProperty(clss, "selection",
"ObjectNode-selection",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "upperBound",
"ObjectNode-upperBound",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Variable
clss = (Class_)model.getElementById("Variable").getDelegate();
prop = factory.createProperty(clss, "activityScope",
"Variable-activityScope",
"Activity",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "scope",
"Variable-scope",
"StructuredActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Duration
clss = (Class_)model.getElementById("Duration").getDelegate();
prop = factory.createProperty(clss, "expr",
"Duration-expr",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "observation",
"Duration-observation",
"Observation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// DurationConstraint
clss = (Class_)model.getElementById("DurationConstraint").getDelegate();
prop = factory.createProperty(clss, "firstEvent",
"DurationConstraint-firstEvent",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "2");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "specification",
"DurationConstraint-specification",
"DurationInterval",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// DurationInterval
clss = (Class_)model.getElementById("DurationInterval").getDelegate();
prop = factory.createProperty(clss, "max",
"DurationInterval-max",
"Duration",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "min",
"DurationInterval-min",
"Duration",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// DurationObservation
clss = (Class_)model.getElementById("DurationObservation").getDelegate();
prop = factory.createProperty(clss, "event",
"DurationObservation-event",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "2");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "firstEvent",
"DurationObservation-firstEvent",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "2");
mapping.mapProperty(clss, prop, this);
// Expression
clss = (Class_)model.getElementById("Expression").getDelegate();
prop = factory.createProperty(clss, "operand",
"Expression-operand",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "symbol",
"Expression-symbol",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Interval
clss = (Class_)model.getElementById("Interval").getDelegate();
prop = factory.createProperty(clss, "max",
"Interval-max",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "min",
"Interval-min",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// IntervalConstraint
clss = (Class_)model.getElementById("IntervalConstraint").getDelegate();
prop = factory.createProperty(clss, "specification",
"IntervalConstraint-specification",
"Interval",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// LiteralBoolean
clss = (Class_)model.getElementById("LiteralBoolean").getDelegate();
prop = factory.createProperty(clss, "value",
"LiteralBoolean-value",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"LiteralBoolean-value-_defaultValue",
"uml:LiteralBoolean",
"");
// LiteralInteger
clss = (Class_)model.getElementById("LiteralInteger").getDelegate();
prop = factory.createProperty(clss, "value",
"LiteralInteger-value",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Integer",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Integer(0)
,
"",
"LiteralInteger-value-_defaultValue",
"uml:LiteralInteger",
"");
// LiteralNull
clss = (Class_)model.getElementById("LiteralNull").getDelegate();
// LiteralReal
clss = (Class_)model.getElementById("LiteralReal").getDelegate();
prop = factory.createProperty(clss, "value",
"LiteralReal-value",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Real",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// LiteralSpecification
clss = (Class_)model.getElementById("LiteralSpecification").getDelegate();
// LiteralString
clss = (Class_)model.getElementById("LiteralString").getDelegate();
prop = factory.createProperty(clss, "value",
"LiteralString-value",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// LiteralUnlimitedNatural
clss = (Class_)model.getElementById("LiteralUnlimitedNatural").getDelegate();
prop = factory.createProperty(clss, "value",
"LiteralUnlimitedNatural-value",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#UnlimitedNatural",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"",
"LiteralUnlimitedNatural-value-_defaultValue",
"uml:LiteralUnlimitedNatural",
"");
// Observation
clss = (Class_)model.getElementById("Observation").getDelegate();
// OpaqueExpression
clss = (Class_)model.getElementById("OpaqueExpression").getDelegate();
prop = factory.createProperty(clss, "behavior",
"OpaqueExpression-behavior",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "body",
"OpaqueExpression-body",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "language",
"OpaqueExpression-language",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"OpaqueExpression-result",
"Parameter",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// StringExpression
clss = (Class_)model.getElementById("StringExpression").getDelegate();
prop = factory.createProperty(clss, "owningExpression",
"StringExpression-owningExpression",
"StringExpression",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "subExpression",
"StringExpression-subExpression",
"StringExpression",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// TimeConstraint
clss = (Class_)model.getElementById("TimeConstraint").getDelegate();
prop = factory.createProperty(clss, "firstEvent",
"TimeConstraint-firstEvent",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"TimeConstraint-firstEvent-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "specification",
"TimeConstraint-specification",
"TimeInterval",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TimeExpression
clss = (Class_)model.getElementById("TimeExpression").getDelegate();
prop = factory.createProperty(clss, "expr",
"TimeExpression-expr",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "observation",
"TimeExpression-observation",
"Observation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// TimeInterval
clss = (Class_)model.getElementById("TimeInterval").getDelegate();
prop = factory.createProperty(clss, "max",
"TimeInterval-max",
"TimeExpression",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "min",
"TimeInterval-min",
"TimeExpression",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TimeObservation
clss = (Class_)model.getElementById("TimeObservation").getDelegate();
prop = factory.createProperty(clss, "event",
"TimeObservation-event",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "firstEvent",
"TimeObservation-firstEvent",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"TimeObservation-firstEvent-_defaultValue",
"uml:LiteralBoolean",
"");
// ValueSpecification
clss = (Class_)model.getElementById("ValueSpecification").getDelegate();
// Actor
clss = (Class_)model.getElementById("Actor").getDelegate();
// Extend
clss = (Class_)model.getElementById("Extend").getDelegate();
prop = factory.createProperty(clss, "condition",
"Extend-condition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "extendedCase",
"Extend-extendedCase",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "extension",
"Extend-extension",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "extensionLocation",
"Extend-extensionLocation",
"ExtensionPoint",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ExtensionPoint
clss = (Class_)model.getElementById("ExtensionPoint").getDelegate();
prop = factory.createProperty(clss, "useCase",
"ExtensionPoint-useCase",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Include
clss = (Class_)model.getElementById("Include").getDelegate();
prop = factory.createProperty(clss, "addition",
"Include-addition",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "includingCase",
"Include-includingCase",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// UseCase
clss = (Class_)model.getElementById("UseCase").getDelegate();
prop = factory.createProperty(clss, "extend",
"UseCase-extend",
"Extend",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "extensionPoint",
"UseCase-extensionPoint",
"ExtensionPoint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "include",
"UseCase-include",
"Include",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "subject",
"UseCase-subject",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Association
clss = (Class_)model.getElementById("Association").getDelegate();
prop = factory.createProperty(clss, "endType",
"Association-endType",
"Type",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isDerived",
"Association-isDerived",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Association-isDerived-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "memberEnd",
"Association-memberEnd",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "2");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "navigableOwnedEnd",
"Association-navigableOwnedEnd",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedEnd",
"Association-ownedEnd",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// AssociationClass
clss = (Class_)model.getElementById("AssociationClass").getDelegate();
// Class
clss = (Class_)model.getElementById("Class").getDelegate();
prop = factory.createProperty(clss, "extension",
"Class-extension",
"Extension",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isAbstract",
"Class-isAbstract",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Class-isAbstract-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isActive",
"Class-isActive",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Class-isActive-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "nestedClassifier",
"Class-nestedClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedAttribute",
"Class-ownedAttribute",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedOperation",
"Class-ownedOperation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedReception",
"Class-ownedReception",
"Reception",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "superClass",
"Class-superClass",
"Class",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Collaboration
clss = (Class_)model.getElementById("Collaboration").getDelegate();
prop = factory.createProperty(clss, "collaborationRole",
"Collaboration-collaborationRole",
"ConnectableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// CollaborationUse
clss = (Class_)model.getElementById("CollaborationUse").getDelegate();
prop = factory.createProperty(clss, "roleBinding",
"CollaborationUse-roleBinding",
"Dependency",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "type",
"CollaborationUse-type",
"Collaboration",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Component
clss = (Class_)model.getElementById("Component").getDelegate();
prop = factory.createProperty(clss, "isIndirectlyInstantiated",
"Component-isIndirectlyInstantiated",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"Component-isIndirectlyInstantiated-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "packagedElement",
"Component-packagedElement",
"PackageableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "provided",
"Component-provided",
"Interface",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "realization",
"Component-realization",
"ComponentRealization",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "required",
"Component-required",
"Interface",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ComponentRealization
clss = (Class_)model.getElementById("ComponentRealization").getDelegate();
prop = factory.createProperty(clss, "abstraction",
"ComponentRealization-abstraction",
"Component",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "realizingClassifier",
"ComponentRealization-realizingClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ConnectableElement
clss = (Class_)model.getElementById("ConnectableElement").getDelegate();
prop = factory.createProperty(clss, "end",
"ConnectableElement-end",
"ConnectorEnd",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "templateParameter",
"ConnectableElement-templateParameter",
"ConnectableElementTemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ConnectableElementTemplateParameter
clss = (Class_)model.getElementById("ConnectableElementTemplateParameter").getDelegate();
prop = factory.createProperty(clss, "parameteredElement",
"ConnectableElementTemplateParameter-parameteredElement",
"ConnectableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Connector
clss = (Class_)model.getElementById("Connector").getDelegate();
prop = factory.createProperty(clss, "contract",
"Connector-contract",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "end",
"Connector-end",
"ConnectorEnd",
"",
false, false, false);
factory.createLowerValue(prop, true, "2");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "kind",
"Connector-kind",
"ConnectorKind",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedConnector",
"Connector-redefinedConnector",
"Connector",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "type",
"Connector-type",
"Association",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ConnectorEnd
clss = (Class_)model.getElementById("ConnectorEnd").getDelegate();
prop = factory.createProperty(clss, "definingEnd",
"ConnectorEnd-definingEnd",
"Property",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "partWithPort",
"ConnectorEnd-partWithPort",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "role",
"ConnectorEnd-role",
"ConnectableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// EncapsulatedClassifier
clss = (Class_)model.getElementById("EncapsulatedClassifier").getDelegate();
prop = factory.createProperty(clss, "ownedPort",
"EncapsulatedClassifier-ownedPort",
"Port",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Port
clss = (Class_)model.getElementById("Port").getDelegate();
prop = factory.createProperty(clss, "isBehavior",
"Port-isBehavior",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Port-isBehavior-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isConjugated",
"Port-isConjugated",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Port-isConjugated-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isService",
"Port-isService",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"Port-isService-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "protocol",
"Port-protocol",
"ProtocolStateMachine",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "provided",
"Port-provided",
"Interface",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedPort",
"Port-redefinedPort",
"Port",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "required",
"Port-required",
"Interface",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// StructuredClassifier
clss = (Class_)model.getElementById("StructuredClassifier").getDelegate();
prop = factory.createProperty(clss, "ownedAttribute",
"StructuredClassifier-ownedAttribute",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedConnector",
"StructuredClassifier-ownedConnector",
"Connector",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "part",
"StructuredClassifier-part",
"Property",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "role",
"StructuredClassifier-role",
"ConnectableElement",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ConnectionPointReference
clss = (Class_)model.getElementById("ConnectionPointReference").getDelegate();
prop = factory.createProperty(clss, "entry",
"ConnectionPointReference-entry",
"Pseudostate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "exit",
"ConnectionPointReference-exit",
"Pseudostate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "state",
"ConnectionPointReference-state",
"State",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// FinalState
clss = (Class_)model.getElementById("FinalState").getDelegate();
// ProtocolConformance
clss = (Class_)model.getElementById("ProtocolConformance").getDelegate();
prop = factory.createProperty(clss, "generalMachine",
"ProtocolConformance-generalMachine",
"ProtocolStateMachine",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "specificMachine",
"ProtocolConformance-specificMachine",
"ProtocolStateMachine",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ProtocolStateMachine
clss = (Class_)model.getElementById("ProtocolStateMachine").getDelegate();
prop = factory.createProperty(clss, "conformance",
"ProtocolStateMachine-conformance",
"ProtocolConformance",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ProtocolTransition
clss = (Class_)model.getElementById("ProtocolTransition").getDelegate();
prop = factory.createProperty(clss, "postCondition",
"ProtocolTransition-postCondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "preCondition",
"ProtocolTransition-preCondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "referred",
"ProtocolTransition-referred",
"Operation",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Pseudostate
clss = (Class_)model.getElementById("Pseudostate").getDelegate();
prop = factory.createProperty(clss, "kind",
"Pseudostate-kind",
"PseudostateKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"PseudostateKind-initial",
"Pseudostate-kind-_defaultValue",
"uml:InstanceValue",
"PseudostateKind");
prop = factory.createProperty(clss, "state",
"Pseudostate-state",
"State",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "stateMachine",
"Pseudostate-stateMachine",
"StateMachine",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Region
clss = (Class_)model.getElementById("Region").getDelegate();
prop = factory.createProperty(clss, "extendedRegion",
"Region-extendedRegion",
"Region",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinitionContext",
"Region-redefinitionContext",
"Classifier",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "state",
"Region-state",
"State",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "stateMachine",
"Region-stateMachine",
"StateMachine",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "subvertex",
"Region-subvertex",
"Vertex",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "transition",
"Region-transition",
"Transition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// State
clss = (Class_)model.getElementById("State").getDelegate();
prop = factory.createProperty(clss, "connection",
"State-connection",
"ConnectionPointReference",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "connectionPoint",
"State-connectionPoint",
"Pseudostate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "deferrableTrigger",
"State-deferrableTrigger",
"Trigger",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "doActivity",
"State-doActivity",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "entry",
"State-entry",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "exit",
"State-exit",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isComposite",
"State-isComposite",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isOrthogonal",
"State-isOrthogonal",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isSimple",
"State-isSimple",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isSubmachineState",
"State-isSubmachineState",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "region",
"State-region",
"Region",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "stateInvariant",
"State-stateInvariant",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "submachine",
"State-submachine",
"StateMachine",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// StateMachine
clss = (Class_)model.getElementById("StateMachine").getDelegate();
prop = factory.createProperty(clss, "connectionPoint",
"StateMachine-connectionPoint",
"Pseudostate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "extendedStateMachine",
"StateMachine-extendedStateMachine",
"StateMachine",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "region",
"StateMachine-region",
"Region",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "submachineState",
"StateMachine-submachineState",
"State",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Transition
clss = (Class_)model.getElementById("Transition").getDelegate();
prop = factory.createProperty(clss, "container",
"Transition-container",
"Region",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "effect",
"Transition-effect",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "guard",
"Transition-guard",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "kind",
"Transition-kind",
"TransitionKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"TransitionKind-external",
"Transition-kind-_defaultValue",
"uml:InstanceValue",
"TransitionKind");
prop = factory.createProperty(clss, "redefinedTransition",
"Transition-redefinedTransition",
"Transition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinitionContext",
"Transition-redefinitionContext",
"Classifier",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "source",
"Transition-source",
"Vertex",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "target",
"Transition-target",
"Vertex",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "trigger",
"Transition-trigger",
"Trigger",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Vertex
clss = (Class_)model.getElementById("Vertex").getDelegate();
prop = factory.createProperty(clss, "container",
"Vertex-container",
"Region",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "incoming",
"Vertex-incoming",
"Transition",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "outgoing",
"Vertex-outgoing",
"Transition",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinitionContext",
"State-redefinitionContext",
"Classifier",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedVertex",
"State-redefinedState",
"Vertex",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// BehavioredClassifier
clss = (Class_)model.getElementById("BehavioredClassifier").getDelegate();
prop = factory.createProperty(clss, "classifierBehavior",
"BehavioredClassifier-classifierBehavior",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interfaceRealization",
"BehavioredClassifier-interfaceRealization",
"InterfaceRealization",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedBehavior",
"BehavioredClassifier-ownedBehavior",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// DataType
clss = (Class_)model.getElementById("DataType").getDelegate();
prop = factory.createProperty(clss, "ownedAttribute",
"DataType-ownedAttribute",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedOperation",
"DataType-ownedOperation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Enumeration
clss = (Class_)model.getElementById("Enumeration").getDelegate();
prop = factory.createProperty(clss, "ownedLiteral",
"Enumeration-ownedLiteral",
"EnumerationLiteral",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// EnumerationLiteral
clss = (Class_)model.getElementById("EnumerationLiteral").getDelegate();
prop = factory.createProperty(clss, "classifier",
"EnumerationLiteral-classifier",
"Enumeration",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "enumeration",
"EnumerationLiteral-enumeration",
"Enumeration",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Interface
clss = (Class_)model.getElementById("Interface").getDelegate();
prop = factory.createProperty(clss, "nestedClassifier",
"Interface-nestedClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedAttribute",
"Interface-ownedAttribute",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedOperation",
"Interface-ownedOperation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedReception",
"Interface-ownedReception",
"Reception",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "protocol",
"Interface-protocol",
"ProtocolStateMachine",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedInterface",
"Interface-redefinedInterface",
"Interface",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// InterfaceRealization
clss = (Class_)model.getElementById("InterfaceRealization").getDelegate();
prop = factory.createProperty(clss, "contract",
"InterfaceRealization-contract",
"Interface",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "implementingClassifier",
"InterfaceRealization-implementingClassifier",
"BehavioredClassifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// PrimitiveType
clss = (Class_)model.getElementById("PrimitiveType").getDelegate();
// Reception
clss = (Class_)model.getElementById("Reception").getDelegate();
prop = factory.createProperty(clss, "signal",
"Reception-signal",
"Signal",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Signal
clss = (Class_)model.getElementById("Signal").getDelegate();
prop = factory.createProperty(clss, "ownedAttribute",
"Signal-ownedAttribute",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Extension
clss = (Class_)model.getElementById("Extension").getDelegate();
prop = factory.createProperty(clss, "isRequired",
"Extension-isRequired",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "metaclass",
"Extension-metaclass",
"Class",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedEnd",
"Extension-ownedEnd",
"ExtensionEnd",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ExtensionEnd
clss = (Class_)model.getElementById("ExtensionEnd").getDelegate();
prop = factory.createProperty(clss, "lower",
"ExtensionEnd-lower",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Integer",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "type",
"ExtensionEnd-type",
"Stereotype",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Image
clss = (Class_)model.getElementById("Image").getDelegate();
prop = factory.createProperty(clss, "content",
"Image-content",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "format",
"Image-format",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "location",
"Image-location",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Model
clss = (Class_)model.getElementById("Model").getDelegate();
prop = factory.createProperty(clss, "viewpoint",
"Model-viewpoint",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Package
clss = (Class_)model.getElementById("Package").getDelegate();
prop = factory.createProperty(clss, "URI",
"Package-URI",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "nestedPackage",
"Package-nestedPackage",
"Package",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "nestingPackage",
"Package-nestingPackage",
"Package",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedStereotype",
"Package-ownedStereotype",
"Stereotype",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedType",
"Package-ownedType",
"Type",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "packageMerge",
"Package-packageMerge",
"PackageMerge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "packagedElement",
"Package-packagedElement",
"PackageableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "profileApplication",
"Package-profileApplication",
"ProfileApplication",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// PackageMerge
clss = (Class_)model.getElementById("PackageMerge").getDelegate();
prop = factory.createProperty(clss, "mergedPackage",
"PackageMerge-mergedPackage",
"Package",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "receivingPackage",
"PackageMerge-receivingPackage",
"Package",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Profile
clss = (Class_)model.getElementById("Profile").getDelegate();
prop = factory.createProperty(clss, "metaclassReference",
"Profile-metaclassReference",
"ElementImport",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "metamodelReference",
"Profile-metamodelReference",
"PackageImport",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ProfileApplication
clss = (Class_)model.getElementById("ProfileApplication").getDelegate();
prop = factory.createProperty(clss, "appliedProfile",
"ProfileApplication-appliedProfile",
"Profile",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "applyingPackage",
"ProfileApplication-applyingPackage",
"Package",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isStrict",
"ProfileApplication-isStrict",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ProfileApplication-isStrict-_defaultValue",
"uml:LiteralBoolean",
"");
// Stereotype
clss = (Class_)model.getElementById("Stereotype").getDelegate();
prop = factory.createProperty(clss, "icon",
"Stereotype-icon",
"Image",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "profile",
"Stereotype-profile",
"Profile",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ActionExecutionSpecification
clss = (Class_)model.getElementById("ActionExecutionSpecification").getDelegate();
prop = factory.createProperty(clss, "action",
"ActionExecutionSpecification-action",
"Action",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// BehaviorExecutionSpecification
clss = (Class_)model.getElementById("BehaviorExecutionSpecification").getDelegate();
prop = factory.createProperty(clss, "behavior",
"BehaviorExecutionSpecification-behavior",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// CombinedFragment
clss = (Class_)model.getElementById("CombinedFragment").getDelegate();
prop = factory.createProperty(clss, "cfragmentGate",
"CombinedFragment-cfragmentGate",
"Gate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interactionOperator",
"CombinedFragment-interactionOperator",
"InteractionOperatorKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"InteractionOperatorKind-seq",
"CombinedFragment-interactionOperator-_defaultValue",
"uml:InstanceValue",
"InteractionOperatorKind");
prop = factory.createProperty(clss, "operand",
"CombinedFragment-operand",
"InteractionOperand",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ConsiderIgnoreFragment
clss = (Class_)model.getElementById("ConsiderIgnoreFragment").getDelegate();
prop = factory.createProperty(clss, "message",
"ConsiderIgnoreFragment-message",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Continuation
clss = (Class_)model.getElementById("Continuation").getDelegate();
prop = factory.createProperty(clss, "setting",
"Continuation-setting",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"Continuation-setting-_defaultValue",
"uml:LiteralBoolean",
"");
// DestructionOccurrenceSpecification
clss = (Class_)model.getElementById("DestructionOccurrenceSpecification").getDelegate();
// ExecutionOccurrenceSpecification
clss = (Class_)model.getElementById("ExecutionOccurrenceSpecification").getDelegate();
prop = factory.createProperty(clss, "execution",
"ExecutionOccurrenceSpecification-execution",
"ExecutionSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ExecutionSpecification
clss = (Class_)model.getElementById("ExecutionSpecification").getDelegate();
prop = factory.createProperty(clss, "finish",
"ExecutionSpecification-finish",
"OccurrenceSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "start",
"ExecutionSpecification-start",
"OccurrenceSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Gate
clss = (Class_)model.getElementById("Gate").getDelegate();
// GeneralOrdering
clss = (Class_)model.getElementById("GeneralOrdering").getDelegate();
prop = factory.createProperty(clss, "after",
"GeneralOrdering-after",
"OccurrenceSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "before",
"GeneralOrdering-before",
"OccurrenceSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Interaction
clss = (Class_)model.getElementById("Interaction").getDelegate();
prop = factory.createProperty(clss, "action",
"Interaction-action",
"Action",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "formalGate",
"Interaction-formalGate",
"Gate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "fragment",
"Interaction-fragment",
"InteractionFragment",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "lifeline",
"Interaction-lifeline",
"Lifeline",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "message",
"Interaction-message",
"Message",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// InteractionConstraint
clss = (Class_)model.getElementById("InteractionConstraint").getDelegate();
prop = factory.createProperty(clss, "maxint",
"InteractionConstraint-maxint",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "minint",
"InteractionConstraint-minint",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// InteractionFragment
clss = (Class_)model.getElementById("InteractionFragment").getDelegate();
prop = factory.createProperty(clss, "covered",
"InteractionFragment-covered",
"Lifeline",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "enclosingInteraction",
"InteractionFragment-enclosingInteraction",
"Interaction",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "enclosingOperand",
"InteractionFragment-enclosingOperand",
"InteractionOperand",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "generalOrdering",
"InteractionFragment-generalOrdering",
"GeneralOrdering",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// InteractionOperand
clss = (Class_)model.getElementById("InteractionOperand").getDelegate();
prop = factory.createProperty(clss, "fragment",
"InteractionOperand-fragment",
"InteractionFragment",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "guard",
"InteractionOperand-guard",
"InteractionConstraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// InteractionUse
clss = (Class_)model.getElementById("InteractionUse").getDelegate();
prop = factory.createProperty(clss, "actualGate",
"InteractionUse-actualGate",
"Gate",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "argument",
"InteractionUse-argument",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "refersTo",
"InteractionUse-refersTo",
"Interaction",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "returnValue",
"InteractionUse-returnValue",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "returnValueRecipient",
"InteractionUse-returnValueRecipient",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Lifeline
clss = (Class_)model.getElementById("Lifeline").getDelegate();
prop = factory.createProperty(clss, "coveredBy",
"Lifeline-coveredBy",
"InteractionFragment",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "decomposedAs",
"Lifeline-decomposedAs",
"PartDecomposition",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interaction",
"Lifeline-interaction",
"Interaction",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "represents",
"Lifeline-represents",
"ConnectableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "selector",
"Lifeline-selector",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Message
clss = (Class_)model.getElementById("Message").getDelegate();
prop = factory.createProperty(clss, "argument",
"Message-argument",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "connector",
"Message-connector",
"Connector",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interaction",
"Message-interaction",
"Interaction",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "messageKind",
"Message-messageKind",
"MessageKind",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "messageSort",
"Message-messageSort",
"MessageSort",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"MessageSort-synchCall",
"Message-messageSort-_defaultValue",
"uml:InstanceValue",
"MessageSort");
prop = factory.createProperty(clss, "receiveEvent",
"Message-receiveEvent",
"MessageEnd",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "sendEvent",
"Message-sendEvent",
"MessageEnd",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "signature",
"Message-signature",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// MessageEnd
clss = (Class_)model.getElementById("MessageEnd").getDelegate();
prop = factory.createProperty(clss, "message",
"MessageEnd-message",
"Message",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// MessageOccurrenceSpecification
clss = (Class_)model.getElementById("MessageOccurrenceSpecification").getDelegate();
// OccurrenceSpecification
clss = (Class_)model.getElementById("OccurrenceSpecification").getDelegate();
prop = factory.createProperty(clss, "covered",
"OccurrenceSpecification-covered",
"Lifeline",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "toAfter",
"OccurrenceSpecification-toAfter",
"GeneralOrdering",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "toBefore",
"OccurrenceSpecification-toBefore",
"GeneralOrdering",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// PartDecomposition
clss = (Class_)model.getElementById("PartDecomposition").getDelegate();
// StateInvariant
clss = (Class_)model.getElementById("StateInvariant").getDelegate();
prop = factory.createProperty(clss, "covered",
"StateInvariant-covered",
"Lifeline",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "invariant",
"StateInvariant-invariant",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// InformationFlow
clss = (Class_)model.getElementById("InformationFlow").getDelegate();
prop = factory.createProperty(clss, "conveyed",
"InformationFlow-conveyed",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "informationSource",
"InformationFlow-informationSource",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "informationTarget",
"InformationFlow-informationTarget",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "realization",
"InformationFlow-realization",
"Relationship",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "realizingActivityEdge",
"InformationFlow-realizingActivityEdge",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "realizingConnector",
"InformationFlow-realizingConnector",
"Connector",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "realizingMessage",
"InformationFlow-realizingMessage",
"Message",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// InformationItem
clss = (Class_)model.getElementById("InformationItem").getDelegate();
prop = factory.createProperty(clss, "represented",
"InformationItem-represented",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Artifact
clss = (Class_)model.getElementById("Artifact").getDelegate();
prop = factory.createProperty(clss, "fileName",
"Artifact-fileName",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "manifestation",
"Artifact-manifestation",
"Manifestation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "nestedArtifact",
"Artifact-nestedArtifact",
"Artifact",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedAttribute",
"Artifact-ownedAttribute",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedOperation",
"Artifact-ownedOperation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// CommunicationPath
clss = (Class_)model.getElementById("CommunicationPath").getDelegate();
// DeployedArtifact
clss = (Class_)model.getElementById("DeployedArtifact").getDelegate();
// Deployment
clss = (Class_)model.getElementById("Deployment").getDelegate();
prop = factory.createProperty(clss, "configuration",
"Deployment-configuration",
"DeploymentSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "deployedArtifact",
"Deployment-deployedArtifact",
"DeployedArtifact",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "location",
"Deployment-location",
"DeploymentTarget",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// DeploymentSpecification
clss = (Class_)model.getElementById("DeploymentSpecification").getDelegate();
prop = factory.createProperty(clss, "deployment",
"DeploymentSpecification-deployment",
"Deployment",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "deploymentLocation",
"DeploymentSpecification-deploymentLocation",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "executionLocation",
"DeploymentSpecification-executionLocation",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// DeploymentTarget
clss = (Class_)model.getElementById("DeploymentTarget").getDelegate();
prop = factory.createProperty(clss, "deployedElement",
"DeploymentTarget-deployedElement",
"PackageableElement",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "deployment",
"DeploymentTarget-deployment",
"Deployment",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Device
clss = (Class_)model.getElementById("Device").getDelegate();
// ExecutionEnvironment
clss = (Class_)model.getElementById("ExecutionEnvironment").getDelegate();
// Manifestation
clss = (Class_)model.getElementById("Manifestation").getDelegate();
prop = factory.createProperty(clss, "utilizedElement",
"Manifestation-utilizedElement",
"PackageableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Node
clss = (Class_)model.getElementById("Node").getDelegate();
prop = factory.createProperty(clss, "nestedNode",
"Node-nestedNode",
"Node",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Abstraction
clss = (Class_)model.getElementById("Abstraction").getDelegate();
prop = factory.createProperty(clss, "mapping",
"Abstraction-mapping",
"OpaqueExpression",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Comment
clss = (Class_)model.getElementById("Comment").getDelegate();
prop = factory.createProperty(clss, "annotatedElement",
"Comment-annotatedElement",
"Element",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "body",
"Comment-body",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Constraint
clss = (Class_)model.getElementById("Constraint").getDelegate();
prop = factory.createProperty(clss, "constrainedElement",
"Constraint-constrainedElement",
"Element",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "context",
"Constraint-context",
"Namespace",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "specification",
"Constraint-specification",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Dependency
clss = (Class_)model.getElementById("Dependency").getDelegate();
prop = factory.createProperty(clss, "client",
"Dependency-client",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "supplier",
"Dependency-supplier",
"NamedElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// DirectedRelationship
clss = (Class_)model.getElementById("DirectedRelationship").getDelegate();
prop = factory.createProperty(clss, "source",
"DirectedRelationship-source",
"Element",
"",
true, true, true);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "target",
"DirectedRelationship-target",
"Element",
"",
true, true, true);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Element
clss = (Class_)model.getElementById("Element").getDelegate();
prop = factory.createProperty(clss, "ownedComment",
"Element-ownedComment",
"Comment",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedElement",
"Element-ownedElement",
"Element",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "owner",
"Element-owner",
"Element",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ElementImport
clss = (Class_)model.getElementById("ElementImport").getDelegate();
prop = factory.createProperty(clss, "alias",
"ElementImport-alias",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "importedElement",
"ElementImport-importedElement",
"PackageableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "importingNamespace",
"ElementImport-importingNamespace",
"Namespace",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "visibility",
"ElementImport-visibility",
"VisibilityKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"VisibilityKind-public",
"ElementImport-visibility-_defaultValue",
"uml:InstanceValue",
"VisibilityKind");
// MultiplicityElement
clss = (Class_)model.getElementById("MultiplicityElement").getDelegate();
prop = factory.createProperty(clss, "isOrdered",
"MultiplicityElement-isOrdered",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"MultiplicityElement-isOrdered-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isUnique",
"MultiplicityElement-isUnique",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"MultiplicityElement-isUnique-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "lower",
"MultiplicityElement-lower",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Integer",
"",
false, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "lowerValue",
"MultiplicityElement-lowerValue",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "upper",
"MultiplicityElement-upper",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#UnlimitedNatural",
"",
false, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "upperValue",
"MultiplicityElement-upperValue",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// NamedElement
clss = (Class_)model.getElementById("NamedElement").getDelegate();
prop = factory.createProperty(clss, "clientDependency",
"NamedElement-clientDependency",
"Dependency",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "name",
"NamedElement-name",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "nameExpression",
"NamedElement-nameExpression",
"StringExpression",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "namespace",
"NamedElement-namespace",
"Namespace",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "qualifiedName",
"NamedElement-qualifiedName",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "visibility",
"NamedElement-visibility",
"VisibilityKind",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Namespace
clss = (Class_)model.getElementById("Namespace").getDelegate();
prop = factory.createProperty(clss, "elementImport",
"Namespace-elementImport",
"ElementImport",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "importedMember",
"Namespace-importedMember",
"PackageableElement",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "member",
"Namespace-member",
"NamedElement",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedMember",
"Namespace-ownedMember",
"NamedElement",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedRule",
"Namespace-ownedRule",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "packageImport",
"Namespace-packageImport",
"PackageImport",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// PackageableElement
clss = (Class_)model.getElementById("PackageableElement").getDelegate();
prop = factory.createProperty(clss, "visibility",
"PackageableElement-visibility",
"VisibilityKind",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"VisibilityKind-public",
"PackageableElement-visibility-_defaultValue",
"uml:InstanceValue",
"VisibilityKind");
// PackageImport
clss = (Class_)model.getElementById("PackageImport").getDelegate();
prop = factory.createProperty(clss, "importedPackage",
"PackageImport-importedPackage",
"Package",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "importingNamespace",
"PackageImport-importingNamespace",
"Namespace",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "visibility",
"PackageImport-visibility",
"VisibilityKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"VisibilityKind-public",
"PackageImport-visibility-_defaultValue",
"uml:InstanceValue",
"VisibilityKind");
// ParameterableElement
clss = (Class_)model.getElementById("ParameterableElement").getDelegate();
prop = factory.createProperty(clss, "owningTemplateParameter",
"ParameterableElement-owningTemplateParameter",
"TemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "templateParameter",
"ParameterableElement-templateParameter",
"TemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Realization
clss = (Class_)model.getElementById("Realization").getDelegate();
// Relationship
clss = (Class_)model.getElementById("Relationship").getDelegate();
prop = factory.createProperty(clss, "relatedElement",
"Relationship-relatedElement",
"Element",
"",
true, true, true);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// TemplateableElement
clss = (Class_)model.getElementById("TemplateableElement").getDelegate();
prop = factory.createProperty(clss, "ownedTemplateSignature",
"TemplateableElement-ownedTemplateSignature",
"TemplateSignature",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "templateBinding",
"TemplateableElement-templateBinding",
"TemplateBinding",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// TemplateBinding
clss = (Class_)model.getElementById("TemplateBinding").getDelegate();
prop = factory.createProperty(clss, "boundElement",
"TemplateBinding-boundElement",
"TemplateableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "parameterSubstitution",
"TemplateBinding-parameterSubstitution",
"TemplateParameterSubstitution",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "signature",
"TemplateBinding-signature",
"TemplateSignature",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TemplateParameter
clss = (Class_)model.getElementById("TemplateParameter").getDelegate();
prop = factory.createProperty(clss, "default",
"TemplateParameter-default",
"ParameterableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedDefault",
"TemplateParameter-ownedDefault",
"ParameterableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedParameteredElement",
"TemplateParameter-ownedParameteredElement",
"ParameterableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "parameteredElement",
"TemplateParameter-parameteredElement",
"ParameterableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "signature",
"TemplateParameter-signature",
"TemplateSignature",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TemplateParameterSubstitution
clss = (Class_)model.getElementById("TemplateParameterSubstitution").getDelegate();
prop = factory.createProperty(clss, "actual",
"TemplateParameterSubstitution-actual",
"ParameterableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "formal",
"TemplateParameterSubstitution-formal",
"TemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedActual",
"TemplateParameterSubstitution-ownedActual",
"ParameterableElement",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "templateBinding",
"TemplateParameterSubstitution-templateBinding",
"TemplateBinding",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TemplateSignature
clss = (Class_)model.getElementById("TemplateSignature").getDelegate();
prop = factory.createProperty(clss, "ownedParameter",
"TemplateSignature-ownedParameter",
"TemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "parameter",
"TemplateSignature-parameter",
"TemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "template",
"TemplateSignature-template",
"TemplateableElement",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Type
clss = (Class_)model.getElementById("Type").getDelegate();
prop = factory.createProperty(clss, "package",
"Type-package",
"Package",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TypedElement
clss = (Class_)model.getElementById("TypedElement").getDelegate();
prop = factory.createProperty(clss, "type",
"TypedElement-type",
"Type",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Usage
clss = (Class_)model.getElementById("Usage").getDelegate();
// AnyReceiveEvent
clss = (Class_)model.getElementById("AnyReceiveEvent").getDelegate();
// Behavior
clss = (Class_)model.getElementById("Behavior").getDelegate();
prop = factory.createProperty(clss, "context",
"Behavior-context",
"BehavioredClassifier",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isReentrant",
"Behavior-isReentrant",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"Behavior-isReentrant-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "ownedParameter",
"Behavior-ownedParameter",
"Parameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedParameterSet",
"Behavior-ownedParameterSet",
"ParameterSet",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "postcondition",
"Behavior-postcondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "precondition",
"Behavior-precondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "specification",
"Behavior-specification",
"BehavioralFeature",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedBehavior",
"Behavior-redefinedBehavior",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// CallEvent
clss = (Class_)model.getElementById("CallEvent").getDelegate();
prop = factory.createProperty(clss, "operation",
"CallEvent-operation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ChangeEvent
clss = (Class_)model.getElementById("ChangeEvent").getDelegate();
prop = factory.createProperty(clss, "changeExpression",
"ChangeEvent-changeExpression",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Event
clss = (Class_)model.getElementById("Event").getDelegate();
// FunctionBehavior
clss = (Class_)model.getElementById("FunctionBehavior").getDelegate();
// MessageEvent
clss = (Class_)model.getElementById("MessageEvent").getDelegate();
// OpaqueBehavior
clss = (Class_)model.getElementById("OpaqueBehavior").getDelegate();
prop = factory.createProperty(clss, "body",
"OpaqueBehavior-body",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "language",
"OpaqueBehavior-language",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// SignalEvent
clss = (Class_)model.getElementById("SignalEvent").getDelegate();
prop = factory.createProperty(clss, "signal",
"SignalEvent-signal",
"Signal",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// TimeEvent
clss = (Class_)model.getElementById("TimeEvent").getDelegate();
prop = factory.createProperty(clss, "isRelative",
"TimeEvent-isRelative",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"TimeEvent-isRelative-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "when",
"TimeEvent-when",
"TimeExpression",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Trigger
clss = (Class_)model.getElementById("Trigger").getDelegate();
prop = factory.createProperty(clss, "event",
"Trigger-event",
"Event",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "port",
"Trigger-port",
"Port",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Substitution
clss = (Class_)model.getElementById("Substitution").getDelegate();
prop = factory.createProperty(clss, "contract",
"Substitution-contract",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "substitutingClassifier",
"Substitution-substitutingClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// BehavioralFeature
clss = (Class_)model.getElementById("BehavioralFeature").getDelegate();
prop = factory.createProperty(clss, "concurrency",
"BehavioralFeature-concurrency",
"CallConcurrencyKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"CallConcurrencyKind-sequential",
"BehavioralFeature-concurrency-_defaultValue",
"uml:InstanceValue",
"CallConcurrencyKind");
prop = factory.createProperty(clss, "isAbstract",
"BehavioralFeature-isAbstract",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"BehavioralFeature-isAbstract-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "method",
"BehavioralFeature-method",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedParameter",
"BehavioralFeature-ownedParameter",
"Parameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedParameterSet",
"BehavioralFeature-ownedParameterSet",
"ParameterSet",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "raisedException",
"BehavioralFeature-raisedException",
"Type",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Classifier
clss = (Class_)model.getElementById("Classifier").getDelegate();
prop = factory.createProperty(clss, "attribute",
"Classifier-attribute",
"Property",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "collaborationUse",
"Classifier-collaborationUse",
"CollaborationUse",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "feature",
"Classifier-feature",
"Feature",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "general",
"Classifier-general",
"Classifier",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "generalization",
"Classifier-generalization",
"Generalization",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inheritedMember",
"Classifier-inheritedMember",
"NamedElement",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isAbstract",
"Classifier-isAbstract",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Classifier-isAbstract-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isFinalSpecialization",
"Classifier-isFinalSpecialization",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Classifier-isFinalSpecialization-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "ownedTemplateSignature",
"Classifier-ownedTemplateSignature",
"RedefinableTemplateSignature",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedUseCase",
"Classifier-ownedUseCase",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "powertypeExtent",
"Classifier-powertypeExtent",
"GeneralizationSet",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedClassifier",
"Classifier-redefinedClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "representation",
"Classifier-representation",
"CollaborationUse",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "substitution",
"Classifier-substitution",
"Substitution",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "templateParameter",
"Classifier-templateParameter",
"ClassifierTemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "useCase",
"Classifier-useCase",
"UseCase",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ClassifierTemplateParameter
clss = (Class_)model.getElementById("ClassifierTemplateParameter").getDelegate();
prop = factory.createProperty(clss, "allowSubstitutable",
"ClassifierTemplateParameter-allowSubstitutable",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"ClassifierTemplateParameter-allowSubstitutable-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "constrainingClassifier",
"ClassifierTemplateParameter-constrainingClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "parameteredElement",
"ClassifierTemplateParameter-parameteredElement",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Feature
clss = (Class_)model.getElementById("Feature").getDelegate();
prop = factory.createProperty(clss, "featuringClassifier",
"Feature-featuringClassifier",
"Classifier",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isStatic",
"Feature-isStatic",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Feature-isStatic-_defaultValue",
"uml:LiteralBoolean",
"");
// Generalization
clss = (Class_)model.getElementById("Generalization").getDelegate();
prop = factory.createProperty(clss, "general",
"Generalization-general",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "generalizationSet",
"Generalization-generalizationSet",
"GeneralizationSet",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isSubstitutable",
"Generalization-isSubstitutable",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"Generalization-isSubstitutable-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "specific",
"Generalization-specific",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// GeneralizationSet
clss = (Class_)model.getElementById("GeneralizationSet").getDelegate();
prop = factory.createProperty(clss, "generalization",
"GeneralizationSet-generalization",
"Generalization",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isCovering",
"GeneralizationSet-isCovering",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"GeneralizationSet-isCovering-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isDisjoint",
"GeneralizationSet-isDisjoint",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"GeneralizationSet-isDisjoint-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "powertype",
"GeneralizationSet-powertype",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// InstanceSpecification
clss = (Class_)model.getElementById("InstanceSpecification").getDelegate();
prop = factory.createProperty(clss, "classifier",
"InstanceSpecification-classifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "slot",
"InstanceSpecification-slot",
"Slot",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "specification",
"InstanceSpecification-specification",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// InstanceValue
clss = (Class_)model.getElementById("InstanceValue").getDelegate();
prop = factory.createProperty(clss, "instance",
"InstanceValue-instance",
"InstanceSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Operation
clss = (Class_)model.getElementById("Operation").getDelegate();
prop = factory.createProperty(clss, "bodyCondition",
"Operation-bodyCondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "class",
"Operation-class",
"Class",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "datatype",
"Operation-datatype",
"DataType",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interface",
"Operation-interface",
"Interface",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isOrdered",
"Operation-isOrdered",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isQuery",
"Operation-isQuery",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Operation-isQuery-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isUnique",
"Operation-isUnique",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
true, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "lower",
"Operation-lower",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Integer",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "ownedParameter",
"Operation-ownedParameter",
"Parameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "postcondition",
"Operation-postcondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "precondition",
"Operation-precondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "raisedException",
"Operation-raisedException",
"Type",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedOperation",
"Operation-redefinedOperation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "templateParameter",
"Operation-templateParameter",
"OperationTemplateParameter",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "type",
"Operation-type",
"Type",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "upper",
"Operation-upper",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#UnlimitedNatural",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// OperationTemplateParameter
clss = (Class_)model.getElementById("OperationTemplateParameter").getDelegate();
prop = factory.createProperty(clss, "parameteredElement",
"OperationTemplateParameter-parameteredElement",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Parameter
clss = (Class_)model.getElementById("Parameter").getDelegate();
prop = factory.createProperty(clss, "default",
"Parameter-default",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "defaultValue",
"Parameter-defaultValue",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "direction",
"Parameter-direction",
"ParameterDirectionKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"ParameterDirectionKind-in",
"Parameter-direction-_defaultValue",
"uml:InstanceValue",
"ParameterDirectionKind");
prop = factory.createProperty(clss, "effect",
"Parameter-effect",
"ParameterEffectKind",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isException",
"Parameter-isException",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Parameter-isException-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isStream",
"Parameter-isStream",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Parameter-isStream-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "operation",
"Parameter-operation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "parameterSet",
"Parameter-parameterSet",
"ParameterSet",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ParameterSet
clss = (Class_)model.getElementById("ParameterSet").getDelegate();
prop = factory.createProperty(clss, "condition",
"ParameterSet-condition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "parameter",
"ParameterSet-parameter",
"Parameter",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Property
clss = (Class_)model.getElementById("Property").getDelegate();
prop = factory.createProperty(clss, "aggregation",
"Property-aggregation",
"AggregationKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"AggregationKind-none",
"Property-aggregation-_defaultValue",
"uml:InstanceValue",
"AggregationKind");
prop = factory.createProperty(clss, "association",
"Property-association",
"Association",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "associationEnd",
"Property-associationEnd",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "class",
"Property-class",
"Class",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "datatype",
"Property-datatype",
"DataType",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "defaultValue",
"Property-defaultValue",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "interface",
"Property-interface",
"Interface",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isComposite",
"Property-isComposite",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, true, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Property-isComposite-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isDerived",
"Property-isDerived",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Property-isDerived-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isDerivedUnion",
"Property-isDerivedUnion",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Property-isDerivedUnion-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isID",
"Property-isID",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Property-isID-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "opposite",
"Property-opposite",
"Property",
"",
false, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "owningAssociation",
"Property-owningAssociation",
"Association",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "qualifier",
"Property-qualifier",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinedProperty",
"Property-redefinedProperty",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "subsettedProperty",
"Property-subsettedProperty",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// RedefinableElement
clss = (Class_)model.getElementById("RedefinableElement").getDelegate();
prop = factory.createProperty(clss, "isLeaf",
"RedefinableElement-isLeaf",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"RedefinableElement-isLeaf-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "redefinedElement",
"RedefinableElement-redefinedElement",
"RedefinableElement",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "redefinitionContext",
"RedefinableElement-redefinitionContext",
"Classifier",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// RedefinableTemplateSignature
clss = (Class_)model.getElementById("RedefinableTemplateSignature").getDelegate();
prop = factory.createProperty(clss, "classifier",
"RedefinableTemplateSignature-classifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "extendedSignature",
"RedefinableTemplateSignature-extendedSignature",
"RedefinableTemplateSignature",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inheritedParameter",
"RedefinableTemplateSignature-inheritedParameter",
"TemplateParameter",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Slot
clss = (Class_)model.getElementById("Slot").getDelegate();
prop = factory.createProperty(clss, "definingFeature",
"Slot-definingFeature",
"StructuralFeature",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "owningInstance",
"Slot-owningInstance",
"InstanceSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "value",
"Slot-value",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// StructuralFeature
clss = (Class_)model.getElementById("StructuralFeature").getDelegate();
prop = factory.createProperty(clss, "isReadOnly",
"StructuralFeature-isReadOnly",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"StructuralFeature-isReadOnly-_defaultValue",
"uml:LiteralBoolean",
"");
// ValueSpecificationAction
clss = (Class_)model.getElementById("ValueSpecificationAction").getDelegate();
prop = factory.createProperty(clss, "result",
"ValueSpecificationAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "value",
"ValueSpecificationAction-value",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// VariableAction
clss = (Class_)model.getElementById("VariableAction").getDelegate();
prop = factory.createProperty(clss, "variable",
"VariableAction-variable",
"Variable",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// WriteLinkAction
clss = (Class_)model.getElementById("WriteLinkAction").getDelegate();
// WriteStructuralFeatureAction
clss = (Class_)model.getElementById("WriteStructuralFeatureAction").getDelegate();
prop = factory.createProperty(clss, "result",
"WriteStructuralFeatureAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "value",
"WriteStructuralFeatureAction-value",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// WriteVariableAction
clss = (Class_)model.getElementById("WriteVariableAction").getDelegate();
prop = factory.createProperty(clss, "value",
"WriteVariableAction-value",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// AcceptCallAction
clss = (Class_)model.getElementById("AcceptCallAction").getDelegate();
prop = factory.createProperty(clss, "returnInformation",
"AcceptCallAction-returnInformation",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// AcceptEventAction
clss = (Class_)model.getElementById("AcceptEventAction").getDelegate();
prop = factory.createProperty(clss, "isUnmarshall",
"AcceptEventAction-isUnmarshall",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"AcceptEventAction-isUnmarshall-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "result",
"AcceptEventAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "trigger",
"AcceptEventAction-trigger",
"Trigger",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// Action
clss = (Class_)model.getElementById("Action").getDelegate();
prop = factory.createProperty(clss, "context",
"Action-context",
"Classifier",
"",
true, true, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "input",
"Action-input",
"InputPin",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isLocallyReentrant",
"Action-isLocallyReentrant",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Action-isLocallyReentrant-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "localPostcondition",
"Action-localPostcondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "localPrecondition",
"Action-localPrecondition",
"Constraint",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "output",
"Action-output",
"OutputPin",
"",
true, true, true);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ActionInputPin
clss = (Class_)model.getElementById("ActionInputPin").getDelegate();
prop = factory.createProperty(clss, "fromAction",
"ActionInputPin-fromAction",
"Action",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// AddStructuralFeatureValueAction
clss = (Class_)model.getElementById("AddStructuralFeatureValueAction").getDelegate();
prop = factory.createProperty(clss, "insertAt",
"AddStructuralFeatureValueAction-insertAt",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isReplaceAll",
"AddStructuralFeatureValueAction-isReplaceAll",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"AddStructuralFeatureValueAction-isReplaceAll-_defaultValue",
"uml:LiteralBoolean",
"");
// AddVariableValueAction
clss = (Class_)model.getElementById("AddVariableValueAction").getDelegate();
prop = factory.createProperty(clss, "insertAt",
"AddVariableValueAction-insertAt",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isReplaceAll",
"AddVariableValueAction-isReplaceAll",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"AddVariableValueAction-isReplaceAll-_defaultValue",
"uml:LiteralBoolean",
"");
// BroadcastSignalAction
clss = (Class_)model.getElementById("BroadcastSignalAction").getDelegate();
prop = factory.createProperty(clss, "signal",
"BroadcastSignalAction-signal",
"Signal",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// CallAction
clss = (Class_)model.getElementById("CallAction").getDelegate();
prop = factory.createProperty(clss, "isSynchronous",
"CallAction-isSynchronous",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(true),
"",
"CallAction-isSynchronous-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "result",
"CallAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// CallBehaviorAction
clss = (Class_)model.getElementById("CallBehaviorAction").getDelegate();
prop = factory.createProperty(clss, "behavior",
"CallBehaviorAction-behavior",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// CallOperationAction
clss = (Class_)model.getElementById("CallOperationAction").getDelegate();
prop = factory.createProperty(clss, "operation",
"CallOperationAction-operation",
"Operation",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "target",
"CallOperationAction-target",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// Clause
clss = (Class_)model.getElementById("Clause").getDelegate();
prop = factory.createProperty(clss, "body",
"Clause-body",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "bodyOutput",
"Clause-bodyOutput",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "decider",
"Clause-decider",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "predecessorClause",
"Clause-predecessorClause",
"Clause",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "successorClause",
"Clause-successorClause",
"Clause",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "test",
"Clause-test",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ClearAssociationAction
clss = (Class_)model.getElementById("ClearAssociationAction").getDelegate();
prop = factory.createProperty(clss, "association",
"ClearAssociationAction-association",
"Association",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "object",
"ClearAssociationAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ClearStructuralFeatureAction
clss = (Class_)model.getElementById("ClearStructuralFeatureAction").getDelegate();
prop = factory.createProperty(clss, "result",
"ClearStructuralFeatureAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ClearVariableAction
clss = (Class_)model.getElementById("ClearVariableAction").getDelegate();
// ConditionalNode
clss = (Class_)model.getElementById("ConditionalNode").getDelegate();
prop = factory.createProperty(clss, "clause",
"ConditionalNode-clause",
"Clause",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isAssured",
"ConditionalNode-isAssured",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ConditionalNode-isAssured-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isDeterminate",
"ConditionalNode-isDeterminate",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ConditionalNode-isDeterminate-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "result",
"ConditionalNode-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// CreateLinkAction
clss = (Class_)model.getElementById("CreateLinkAction").getDelegate();
prop = factory.createProperty(clss, "endData",
"CreateLinkAction-endData",
"LinkEndCreationData",
"",
false, false, false);
factory.createLowerValue(prop, true, "2");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// CreateLinkObjectAction
clss = (Class_)model.getElementById("CreateLinkObjectAction").getDelegate();
prop = factory.createProperty(clss, "result",
"CreateLinkObjectAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// CreateObjectAction
clss = (Class_)model.getElementById("CreateObjectAction").getDelegate();
prop = factory.createProperty(clss, "classifier",
"CreateObjectAction-classifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"CreateObjectAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// DestroyLinkAction
clss = (Class_)model.getElementById("DestroyLinkAction").getDelegate();
prop = factory.createProperty(clss, "endData",
"DestroyLinkAction-endData",
"LinkEndDestructionData",
"",
false, false, false);
factory.createLowerValue(prop, true, "2");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// DestroyObjectAction
clss = (Class_)model.getElementById("DestroyObjectAction").getDelegate();
prop = factory.createProperty(clss, "isDestroyLinks",
"DestroyObjectAction-isDestroyLinks",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"DestroyObjectAction-isDestroyLinks-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "isDestroyOwnedObjects",
"DestroyObjectAction-isDestroyOwnedObjects",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"DestroyObjectAction-isDestroyOwnedObjects-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "target",
"DestroyObjectAction-target",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ExpansionNode
clss = (Class_)model.getElementById("ExpansionNode").getDelegate();
prop = factory.createProperty(clss, "regionAsInput",
"ExpansionNode-regionAsInput",
"ExpansionRegion",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "regionAsOutput",
"ExpansionNode-regionAsOutput",
"ExpansionRegion",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ExpansionRegion
clss = (Class_)model.getElementById("ExpansionRegion").getDelegate();
prop = factory.createProperty(clss, "inputElement",
"ExpansionRegion-inputElement",
"ExpansionNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "mode",
"ExpansionRegion-mode",
"ExpansionKind",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new String(""),
"ExpansionKind-iterative",
"ExpansionRegion-mode-_defaultValue",
"uml:InstanceValue",
"ExpansionKind");
prop = factory.createProperty(clss, "outputElement",
"ExpansionRegion-outputElement",
"ExpansionNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// InputPin
clss = (Class_)model.getElementById("InputPin").getDelegate();
// InvocationAction
clss = (Class_)model.getElementById("InvocationAction").getDelegate();
prop = factory.createProperty(clss, "argument",
"InvocationAction-argument",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "onPort",
"InvocationAction-onPort",
"Port",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// LinkAction
clss = (Class_)model.getElementById("LinkAction").getDelegate();
prop = factory.createProperty(clss, "endData",
"LinkAction-endData",
"LinkEndData",
"",
false, false, false);
factory.createLowerValue(prop, true, "2");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inputValue",
"LinkAction-inputValue",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// LinkEndCreationData
clss = (Class_)model.getElementById("LinkEndCreationData").getDelegate();
prop = factory.createProperty(clss, "insertAt",
"LinkEndCreationData-insertAt",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isReplaceAll",
"LinkEndCreationData-isReplaceAll",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"LinkEndCreationData-isReplaceAll-_defaultValue",
"uml:LiteralBoolean",
"");
// LinkEndData
clss = (Class_)model.getElementById("LinkEndData").getDelegate();
prop = factory.createProperty(clss, "end",
"LinkEndData-end",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "qualifier",
"LinkEndData-qualifier",
"QualifierValue",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "value",
"LinkEndData-value",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// LinkEndDestructionData
clss = (Class_)model.getElementById("LinkEndDestructionData").getDelegate();
prop = factory.createProperty(clss, "destroyAt",
"LinkEndDestructionData-destroyAt",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isDestroyDuplicates",
"LinkEndDestructionData-isDestroyDuplicates",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"LinkEndDestructionData-isDestroyDuplicates-_defaultValue",
"uml:LiteralBoolean",
"");
// LoopNode
clss = (Class_)model.getElementById("LoopNode").getDelegate();
prop = factory.createProperty(clss, "bodyOutput",
"LoopNode-bodyOutput",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "bodyPart",
"LoopNode-bodyPart",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "decider",
"LoopNode-decider",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isTestedFirst",
"LoopNode-isTestedFirst",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"LoopNode-isTestedFirst-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "loopVariable",
"LoopNode-loopVariable",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "loopVariableInput",
"LoopNode-loopVariableInput",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"LoopNode-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "setupPart",
"LoopNode-setupPart",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "test",
"LoopNode-test",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// OpaqueAction
clss = (Class_)model.getElementById("OpaqueAction").getDelegate();
prop = factory.createProperty(clss, "body",
"OpaqueAction-body",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "inputValue",
"OpaqueAction-inputValue",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "language",
"OpaqueAction-language",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#String",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "outputValue",
"OpaqueAction-outputValue",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// OutputPin
clss = (Class_)model.getElementById("OutputPin").getDelegate();
// Pin
clss = (Class_)model.getElementById("Pin").getDelegate();
prop = factory.createProperty(clss, "isControl",
"Pin-isControl",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"Pin-isControl-_defaultValue",
"uml:LiteralBoolean",
"");
// QualifierValue
clss = (Class_)model.getElementById("QualifierValue").getDelegate();
prop = factory.createProperty(clss, "qualifier",
"QualifierValue-qualifier",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "value",
"QualifierValue-value",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// RaiseExceptionAction
clss = (Class_)model.getElementById("RaiseExceptionAction").getDelegate();
prop = factory.createProperty(clss, "exception",
"RaiseExceptionAction-exception",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadExtentAction
clss = (Class_)model.getElementById("ReadExtentAction").getDelegate();
prop = factory.createProperty(clss, "classifier",
"ReadExtentAction-classifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"ReadExtentAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadIsClassifiedObjectAction
clss = (Class_)model.getElementById("ReadIsClassifiedObjectAction").getDelegate();
prop = factory.createProperty(clss, "classifier",
"ReadIsClassifiedObjectAction-classifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isDirect",
"ReadIsClassifiedObjectAction-isDirect",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ReadIsClassifiedObjectAction-isDirect-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "object",
"ReadIsClassifiedObjectAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"ReadIsClassifiedObjectAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadLinkAction
clss = (Class_)model.getElementById("ReadLinkAction").getDelegate();
prop = factory.createProperty(clss, "result",
"ReadLinkAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadLinkObjectEndAction
clss = (Class_)model.getElementById("ReadLinkObjectEndAction").getDelegate();
prop = factory.createProperty(clss, "end",
"ReadLinkObjectEndAction-end",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "object",
"ReadLinkObjectEndAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"ReadLinkObjectEndAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadLinkObjectEndQualifierAction
clss = (Class_)model.getElementById("ReadLinkObjectEndQualifierAction").getDelegate();
prop = factory.createProperty(clss, "object",
"ReadLinkObjectEndQualifierAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "qualifier",
"ReadLinkObjectEndQualifierAction-qualifier",
"Property",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"ReadLinkObjectEndQualifierAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadSelfAction
clss = (Class_)model.getElementById("ReadSelfAction").getDelegate();
prop = factory.createProperty(clss, "result",
"ReadSelfAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadStructuralFeatureAction
clss = (Class_)model.getElementById("ReadStructuralFeatureAction").getDelegate();
prop = factory.createProperty(clss, "result",
"ReadStructuralFeatureAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReadVariableAction
clss = (Class_)model.getElementById("ReadVariableAction").getDelegate();
prop = factory.createProperty(clss, "result",
"ReadVariableAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReclassifyObjectAction
clss = (Class_)model.getElementById("ReclassifyObjectAction").getDelegate();
prop = factory.createProperty(clss, "isReplaceAll",
"ReclassifyObjectAction-isReplaceAll",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ReclassifyObjectAction-isReplaceAll-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "newClassifier",
"ReclassifyObjectAction-newClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "object",
"ReclassifyObjectAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "oldClassifier",
"ReclassifyObjectAction-oldClassifier",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// ReduceAction
clss = (Class_)model.getElementById("ReduceAction").getDelegate();
prop = factory.createProperty(clss, "collection",
"ReduceAction-collection",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "isOrdered",
"ReduceAction-isOrdered",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"ReduceAction-isOrdered-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "reducer",
"ReduceAction-reducer",
"Behavior",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"ReduceAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// RemoveStructuralFeatureValueAction
clss = (Class_)model.getElementById("RemoveStructuralFeatureValueAction").getDelegate();
prop = factory.createProperty(clss, "isRemoveDuplicates",
"RemoveStructuralFeatureValueAction-isRemoveDuplicates",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"RemoveStructuralFeatureValueAction-isRemoveDuplicates-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "removeAt",
"RemoveStructuralFeatureValueAction-removeAt",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// RemoveVariableValueAction
clss = (Class_)model.getElementById("RemoveVariableValueAction").getDelegate();
prop = factory.createProperty(clss, "isRemoveDuplicates",
"RemoveVariableValueAction-isRemoveDuplicates",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"RemoveVariableValueAction-isRemoveDuplicates-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "removeAt",
"RemoveVariableValueAction-removeAt",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ReplyAction
clss = (Class_)model.getElementById("ReplyAction").getDelegate();
prop = factory.createProperty(clss, "replyToCall",
"ReplyAction-replyToCall",
"Trigger",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "replyValue",
"ReplyAction-replyValue",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "returnInformation",
"ReplyAction-returnInformation",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// SendObjectAction
clss = (Class_)model.getElementById("SendObjectAction").getDelegate();
prop = factory.createProperty(clss, "request",
"SendObjectAction-request",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "target",
"SendObjectAction-target",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// SendSignalAction
clss = (Class_)model.getElementById("SendSignalAction").getDelegate();
prop = factory.createProperty(clss, "signal",
"SendSignalAction-signal",
"Signal",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "target",
"SendSignalAction-target",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// SequenceNode
clss = (Class_)model.getElementById("SequenceNode").getDelegate();
prop = factory.createProperty(clss, "executableNode",
"SequenceNode-executableNode",
"ExecutableNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// StartClassifierBehaviorAction
clss = (Class_)model.getElementById("StartClassifierBehaviorAction").getDelegate();
prop = factory.createProperty(clss, "object",
"StartClassifierBehaviorAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// StartObjectBehaviorAction
clss = (Class_)model.getElementById("StartObjectBehaviorAction").getDelegate();
prop = factory.createProperty(clss, "object",
"StartObjectBehaviorAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// StructuralFeatureAction
clss = (Class_)model.getElementById("StructuralFeatureAction").getDelegate();
prop = factory.createProperty(clss, "object",
"StructuralFeatureAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "structuralFeature",
"StructuralFeatureAction-structuralFeature",
"StructuralFeature",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// StructuredActivityNode
clss = (Class_)model.getElementById("StructuredActivityNode").getDelegate();
prop = factory.createProperty(clss, "activity",
"StructuredActivityNode-activity",
"Activity",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "edge",
"StructuredActivityNode-edge",
"ActivityEdge",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "mustIsolate",
"StructuredActivityNode-mustIsolate",
"http://www.omg.org/spec/UML/20131001/PrimitiveTypes.xmi#Boolean",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
factory.createDefault(prop,
new Boolean(false),
"",
"StructuredActivityNode-mustIsolate-_defaultValue",
"uml:LiteralBoolean",
"");
prop = factory.createProperty(clss, "node",
"StructuredActivityNode-node",
"ActivityNode",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "structuredNodeInput",
"StructuredActivityNode-structuredNodeInput",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "structuredNodeOutput",
"StructuredActivityNode-structuredNodeOutput",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "variable",
"StructuredActivityNode-variable",
"Variable",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
// TestIdentityAction
clss = (Class_)model.getElementById("TestIdentityAction").getDelegate();
prop = factory.createProperty(clss, "first",
"TestIdentityAction-first",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"TestIdentityAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "second",
"TestIdentityAction-second",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// UnmarshallAction
clss = (Class_)model.getElementById("UnmarshallAction").getDelegate();
prop = factory.createProperty(clss, "object",
"UnmarshallAction-object",
"InputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "result",
"UnmarshallAction-result",
"OutputPin",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(clss, prop, this);
prop = factory.createProperty(clss, "unmarshallType",
"UnmarshallAction-unmarshallType",
"Classifier",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
// ValuePin
clss = (Class_)model.getElementById("ValuePin").getDelegate();
prop = factory.createProperty(clss, "value",
"ValuePin-value",
"ValueSpecification",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(clss, prop, this);
}
private void constructGeneralizations()
{
Class_ clss = null;
// Activity
clss = (Class_)model.getElementById("Activity").getDelegate();
factory.createGeneralization(clss, "Behavior");
// ActivityEdge
clss = (Class_)model.getElementById("ActivityEdge").getDelegate();
factory.createGeneralization(clss, "RedefinableElement");
// ActivityFinalNode
clss = (Class_)model.getElementById("ActivityFinalNode").getDelegate();
factory.createGeneralization(clss, "FinalNode");
// ActivityGroup
clss = (Class_)model.getElementById("ActivityGroup").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// ActivityNode
clss = (Class_)model.getElementById("ActivityNode").getDelegate();
factory.createGeneralization(clss, "RedefinableElement");
// ActivityParameterNode
clss = (Class_)model.getElementById("ActivityParameterNode").getDelegate();
factory.createGeneralization(clss, "ObjectNode");
// ActivityPartition
clss = (Class_)model.getElementById("ActivityPartition").getDelegate();
factory.createGeneralization(clss, "ActivityGroup");
// CentralBufferNode
clss = (Class_)model.getElementById("CentralBufferNode").getDelegate();
factory.createGeneralization(clss, "ObjectNode");
// ControlFlow
clss = (Class_)model.getElementById("ControlFlow").getDelegate();
factory.createGeneralization(clss, "ActivityEdge");
// ControlNode
clss = (Class_)model.getElementById("ControlNode").getDelegate();
factory.createGeneralization(clss, "ActivityNode");
// DataStoreNode
clss = (Class_)model.getElementById("DataStoreNode").getDelegate();
factory.createGeneralization(clss, "CentralBufferNode");
// DecisionNode
clss = (Class_)model.getElementById("DecisionNode").getDelegate();
factory.createGeneralization(clss, "ControlNode");
// ExceptionHandler
clss = (Class_)model.getElementById("ExceptionHandler").getDelegate();
factory.createGeneralization(clss, "Element");
// ExecutableNode
clss = (Class_)model.getElementById("ExecutableNode").getDelegate();
factory.createGeneralization(clss, "ActivityNode");
// FinalNode
clss = (Class_)model.getElementById("FinalNode").getDelegate();
factory.createGeneralization(clss, "ControlNode");
// FlowFinalNode
clss = (Class_)model.getElementById("FlowFinalNode").getDelegate();
factory.createGeneralization(clss, "FinalNode");
// ForkNode
clss = (Class_)model.getElementById("ForkNode").getDelegate();
factory.createGeneralization(clss, "ControlNode");
// InitialNode
clss = (Class_)model.getElementById("InitialNode").getDelegate();
factory.createGeneralization(clss, "ControlNode");
// InterruptibleActivityRegion
clss = (Class_)model.getElementById("InterruptibleActivityRegion").getDelegate();
factory.createGeneralization(clss, "ActivityGroup");
// JoinNode
clss = (Class_)model.getElementById("JoinNode").getDelegate();
factory.createGeneralization(clss, "ControlNode");
// MergeNode
clss = (Class_)model.getElementById("MergeNode").getDelegate();
factory.createGeneralization(clss, "ControlNode");
// ObjectFlow
clss = (Class_)model.getElementById("ObjectFlow").getDelegate();
factory.createGeneralization(clss, "ActivityEdge");
// ObjectNode
clss = (Class_)model.getElementById("ObjectNode").getDelegate();
factory.createGeneralization(clss, "TypedElement");
factory.createGeneralization(clss, "ActivityNode");
// Variable
clss = (Class_)model.getElementById("Variable").getDelegate();
factory.createGeneralization(clss, "ConnectableElement");
factory.createGeneralization(clss, "MultiplicityElement");
// Duration
clss = (Class_)model.getElementById("Duration").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// DurationConstraint
clss = (Class_)model.getElementById("DurationConstraint").getDelegate();
factory.createGeneralization(clss, "IntervalConstraint");
// DurationInterval
clss = (Class_)model.getElementById("DurationInterval").getDelegate();
factory.createGeneralization(clss, "Interval");
// DurationObservation
clss = (Class_)model.getElementById("DurationObservation").getDelegate();
factory.createGeneralization(clss, "Observation");
// Expression
clss = (Class_)model.getElementById("Expression").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// Interval
clss = (Class_)model.getElementById("Interval").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// IntervalConstraint
clss = (Class_)model.getElementById("IntervalConstraint").getDelegate();
factory.createGeneralization(clss, "Constraint");
// LiteralBoolean
clss = (Class_)model.getElementById("LiteralBoolean").getDelegate();
factory.createGeneralization(clss, "LiteralSpecification");
// LiteralInteger
clss = (Class_)model.getElementById("LiteralInteger").getDelegate();
factory.createGeneralization(clss, "LiteralSpecification");
// LiteralNull
clss = (Class_)model.getElementById("LiteralNull").getDelegate();
factory.createGeneralization(clss, "LiteralSpecification");
// LiteralReal
clss = (Class_)model.getElementById("LiteralReal").getDelegate();
factory.createGeneralization(clss, "LiteralSpecification");
// LiteralSpecification
clss = (Class_)model.getElementById("LiteralSpecification").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// LiteralString
clss = (Class_)model.getElementById("LiteralString").getDelegate();
factory.createGeneralization(clss, "LiteralSpecification");
// LiteralUnlimitedNatural
clss = (Class_)model.getElementById("LiteralUnlimitedNatural").getDelegate();
factory.createGeneralization(clss, "LiteralSpecification");
// Observation
clss = (Class_)model.getElementById("Observation").getDelegate();
factory.createGeneralization(clss, "PackageableElement");
// OpaqueExpression
clss = (Class_)model.getElementById("OpaqueExpression").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// StringExpression
clss = (Class_)model.getElementById("StringExpression").getDelegate();
factory.createGeneralization(clss, "TemplateableElement");
factory.createGeneralization(clss, "Expression");
// TimeConstraint
clss = (Class_)model.getElementById("TimeConstraint").getDelegate();
factory.createGeneralization(clss, "IntervalConstraint");
// TimeExpression
clss = (Class_)model.getElementById("TimeExpression").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// TimeInterval
clss = (Class_)model.getElementById("TimeInterval").getDelegate();
factory.createGeneralization(clss, "Interval");
// TimeObservation
clss = (Class_)model.getElementById("TimeObservation").getDelegate();
factory.createGeneralization(clss, "Observation");
// ValueSpecification
clss = (Class_)model.getElementById("ValueSpecification").getDelegate();
factory.createGeneralization(clss, "TypedElement");
factory.createGeneralization(clss, "PackageableElement");
// Actor
clss = (Class_)model.getElementById("Actor").getDelegate();
factory.createGeneralization(clss, "BehavioredClassifier");
// Extend
clss = (Class_)model.getElementById("Extend").getDelegate();
factory.createGeneralization(clss, "NamedElement");
factory.createGeneralization(clss, "DirectedRelationship");
// ExtensionPoint
clss = (Class_)model.getElementById("ExtensionPoint").getDelegate();
factory.createGeneralization(clss, "RedefinableElement");
// Include
clss = (Class_)model.getElementById("Include").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
factory.createGeneralization(clss, "NamedElement");
// UseCase
clss = (Class_)model.getElementById("UseCase").getDelegate();
factory.createGeneralization(clss, "BehavioredClassifier");
// Association
clss = (Class_)model.getElementById("Association").getDelegate();
factory.createGeneralization(clss, "Relationship");
factory.createGeneralization(clss, "Classifier");
// AssociationClass
clss = (Class_)model.getElementById("AssociationClass").getDelegate();
factory.createGeneralization(clss, "Class");
factory.createGeneralization(clss, "Association");
// Class
clss = (Class_)model.getElementById("Class").getDelegate();
factory.createGeneralization(clss, "BehavioredClassifier");
factory.createGeneralization(clss, "EncapsulatedClassifier");
// Collaboration
clss = (Class_)model.getElementById("Collaboration").getDelegate();
factory.createGeneralization(clss, "StructuredClassifier");
factory.createGeneralization(clss, "BehavioredClassifier");
// CollaborationUse
clss = (Class_)model.getElementById("CollaborationUse").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Component
clss = (Class_)model.getElementById("Component").getDelegate();
factory.createGeneralization(clss, "Class");
// ComponentRealization
clss = (Class_)model.getElementById("ComponentRealization").getDelegate();
factory.createGeneralization(clss, "Realization");
// ConnectableElement
clss = (Class_)model.getElementById("ConnectableElement").getDelegate();
factory.createGeneralization(clss, "TypedElement");
factory.createGeneralization(clss, "ParameterableElement");
// ConnectableElementTemplateParameter
clss = (Class_)model.getElementById("ConnectableElementTemplateParameter").getDelegate();
factory.createGeneralization(clss, "TemplateParameter");
// Connector
clss = (Class_)model.getElementById("Connector").getDelegate();
factory.createGeneralization(clss, "Feature");
// ConnectorEnd
clss = (Class_)model.getElementById("ConnectorEnd").getDelegate();
factory.createGeneralization(clss, "MultiplicityElement");
// EncapsulatedClassifier
clss = (Class_)model.getElementById("EncapsulatedClassifier").getDelegate();
factory.createGeneralization(clss, "StructuredClassifier");
// Port
clss = (Class_)model.getElementById("Port").getDelegate();
factory.createGeneralization(clss, "Property");
// StructuredClassifier
clss = (Class_)model.getElementById("StructuredClassifier").getDelegate();
factory.createGeneralization(clss, "Classifier");
// ConnectionPointReference
clss = (Class_)model.getElementById("ConnectionPointReference").getDelegate();
factory.createGeneralization(clss, "Vertex");
// FinalState
clss = (Class_)model.getElementById("FinalState").getDelegate();
factory.createGeneralization(clss, "State");
// ProtocolConformance
clss = (Class_)model.getElementById("ProtocolConformance").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// ProtocolStateMachine
clss = (Class_)model.getElementById("ProtocolStateMachine").getDelegate();
factory.createGeneralization(clss, "StateMachine");
// ProtocolTransition
clss = (Class_)model.getElementById("ProtocolTransition").getDelegate();
factory.createGeneralization(clss, "Transition");
// Pseudostate
clss = (Class_)model.getElementById("Pseudostate").getDelegate();
factory.createGeneralization(clss, "Vertex");
// Region
clss = (Class_)model.getElementById("Region").getDelegate();
factory.createGeneralization(clss, "Namespace");
factory.createGeneralization(clss, "RedefinableElement");
// State
clss = (Class_)model.getElementById("State").getDelegate();
factory.createGeneralization(clss, "Namespace");
factory.createGeneralization(clss, "Vertex");
// StateMachine
clss = (Class_)model.getElementById("StateMachine").getDelegate();
factory.createGeneralization(clss, "Behavior");
// Transition
clss = (Class_)model.getElementById("Transition").getDelegate();
factory.createGeneralization(clss, "Namespace");
factory.createGeneralization(clss, "RedefinableElement");
// Vertex
clss = (Class_)model.getElementById("Vertex").getDelegate();
factory.createGeneralization(clss, "NamedElement");
factory.createGeneralization(clss, "RedefinableElement");
// BehavioredClassifier
clss = (Class_)model.getElementById("BehavioredClassifier").getDelegate();
factory.createGeneralization(clss, "Classifier");
// DataType
clss = (Class_)model.getElementById("DataType").getDelegate();
factory.createGeneralization(clss, "Classifier");
// Enumeration
clss = (Class_)model.getElementById("Enumeration").getDelegate();
factory.createGeneralization(clss, "DataType");
// EnumerationLiteral
clss = (Class_)model.getElementById("EnumerationLiteral").getDelegate();
factory.createGeneralization(clss, "InstanceSpecification");
// Interface
clss = (Class_)model.getElementById("Interface").getDelegate();
factory.createGeneralization(clss, "Classifier");
// InterfaceRealization
clss = (Class_)model.getElementById("InterfaceRealization").getDelegate();
factory.createGeneralization(clss, "Realization");
// PrimitiveType
clss = (Class_)model.getElementById("PrimitiveType").getDelegate();
factory.createGeneralization(clss, "DataType");
// Reception
clss = (Class_)model.getElementById("Reception").getDelegate();
factory.createGeneralization(clss, "BehavioralFeature");
// Signal
clss = (Class_)model.getElementById("Signal").getDelegate();
factory.createGeneralization(clss, "Classifier");
// Extension
clss = (Class_)model.getElementById("Extension").getDelegate();
factory.createGeneralization(clss, "Association");
// ExtensionEnd
clss = (Class_)model.getElementById("ExtensionEnd").getDelegate();
factory.createGeneralization(clss, "Property");
// Image
clss = (Class_)model.getElementById("Image").getDelegate();
factory.createGeneralization(clss, "Element");
// Model
clss = (Class_)model.getElementById("Model").getDelegate();
factory.createGeneralization(clss, "Package");
// Package
clss = (Class_)model.getElementById("Package").getDelegate();
factory.createGeneralization(clss, "PackageableElement");
factory.createGeneralization(clss, "TemplateableElement");
factory.createGeneralization(clss, "Namespace");
// PackageMerge
clss = (Class_)model.getElementById("PackageMerge").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// Profile
clss = (Class_)model.getElementById("Profile").getDelegate();
factory.createGeneralization(clss, "Package");
// ProfileApplication
clss = (Class_)model.getElementById("ProfileApplication").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// Stereotype
clss = (Class_)model.getElementById("Stereotype").getDelegate();
factory.createGeneralization(clss, "Class");
// ActionExecutionSpecification
clss = (Class_)model.getElementById("ActionExecutionSpecification").getDelegate();
factory.createGeneralization(clss, "ExecutionSpecification");
// BehaviorExecutionSpecification
clss = (Class_)model.getElementById("BehaviorExecutionSpecification").getDelegate();
factory.createGeneralization(clss, "ExecutionSpecification");
// CombinedFragment
clss = (Class_)model.getElementById("CombinedFragment").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
// ConsiderIgnoreFragment
clss = (Class_)model.getElementById("ConsiderIgnoreFragment").getDelegate();
factory.createGeneralization(clss, "CombinedFragment");
// Continuation
clss = (Class_)model.getElementById("Continuation").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
// DestructionOccurrenceSpecification
clss = (Class_)model.getElementById("DestructionOccurrenceSpecification").getDelegate();
factory.createGeneralization(clss, "MessageOccurrenceSpecification");
// ExecutionOccurrenceSpecification
clss = (Class_)model.getElementById("ExecutionOccurrenceSpecification").getDelegate();
factory.createGeneralization(clss, "OccurrenceSpecification");
// ExecutionSpecification
clss = (Class_)model.getElementById("ExecutionSpecification").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
// Gate
clss = (Class_)model.getElementById("Gate").getDelegate();
factory.createGeneralization(clss, "MessageEnd");
// GeneralOrdering
clss = (Class_)model.getElementById("GeneralOrdering").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Interaction
clss = (Class_)model.getElementById("Interaction").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
factory.createGeneralization(clss, "Behavior");
// InteractionConstraint
clss = (Class_)model.getElementById("InteractionConstraint").getDelegate();
factory.createGeneralization(clss, "Constraint");
// InteractionFragment
clss = (Class_)model.getElementById("InteractionFragment").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// InteractionOperand
clss = (Class_)model.getElementById("InteractionOperand").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
factory.createGeneralization(clss, "Namespace");
// InteractionUse
clss = (Class_)model.getElementById("InteractionUse").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
// Lifeline
clss = (Class_)model.getElementById("Lifeline").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Message
clss = (Class_)model.getElementById("Message").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// MessageEnd
clss = (Class_)model.getElementById("MessageEnd").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// MessageOccurrenceSpecification
clss = (Class_)model.getElementById("MessageOccurrenceSpecification").getDelegate();
factory.createGeneralization(clss, "MessageEnd");
factory.createGeneralization(clss, "OccurrenceSpecification");
// OccurrenceSpecification
clss = (Class_)model.getElementById("OccurrenceSpecification").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
// PartDecomposition
clss = (Class_)model.getElementById("PartDecomposition").getDelegate();
factory.createGeneralization(clss, "InteractionUse");
// StateInvariant
clss = (Class_)model.getElementById("StateInvariant").getDelegate();
factory.createGeneralization(clss, "InteractionFragment");
// InformationFlow
clss = (Class_)model.getElementById("InformationFlow").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
factory.createGeneralization(clss, "PackageableElement");
// InformationItem
clss = (Class_)model.getElementById("InformationItem").getDelegate();
factory.createGeneralization(clss, "Classifier");
// Artifact
clss = (Class_)model.getElementById("Artifact").getDelegate();
factory.createGeneralization(clss, "Classifier");
factory.createGeneralization(clss, "DeployedArtifact");
// CommunicationPath
clss = (Class_)model.getElementById("CommunicationPath").getDelegate();
factory.createGeneralization(clss, "Association");
// DeployedArtifact
clss = (Class_)model.getElementById("DeployedArtifact").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Deployment
clss = (Class_)model.getElementById("Deployment").getDelegate();
factory.createGeneralization(clss, "Dependency");
// DeploymentSpecification
clss = (Class_)model.getElementById("DeploymentSpecification").getDelegate();
factory.createGeneralization(clss, "Artifact");
// DeploymentTarget
clss = (Class_)model.getElementById("DeploymentTarget").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Device
clss = (Class_)model.getElementById("Device").getDelegate();
factory.createGeneralization(clss, "Node");
// ExecutionEnvironment
clss = (Class_)model.getElementById("ExecutionEnvironment").getDelegate();
factory.createGeneralization(clss, "Node");
// Manifestation
clss = (Class_)model.getElementById("Manifestation").getDelegate();
factory.createGeneralization(clss, "Abstraction");
// Node
clss = (Class_)model.getElementById("Node").getDelegate();
factory.createGeneralization(clss, "Class");
factory.createGeneralization(clss, "DeploymentTarget");
// Abstraction
clss = (Class_)model.getElementById("Abstraction").getDelegate();
factory.createGeneralization(clss, "Dependency");
// Comment
clss = (Class_)model.getElementById("Comment").getDelegate();
factory.createGeneralization(clss, "Element");
// Constraint
clss = (Class_)model.getElementById("Constraint").getDelegate();
factory.createGeneralization(clss, "PackageableElement");
// Dependency
clss = (Class_)model.getElementById("Dependency").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
factory.createGeneralization(clss, "PackageableElement");
// DirectedRelationship
clss = (Class_)model.getElementById("DirectedRelationship").getDelegate();
factory.createGeneralization(clss, "Relationship");
// Element
clss = (Class_)model.getElementById("Element").getDelegate();
// ElementImport
clss = (Class_)model.getElementById("ElementImport").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// MultiplicityElement
clss = (Class_)model.getElementById("MultiplicityElement").getDelegate();
factory.createGeneralization(clss, "Element");
// NamedElement
clss = (Class_)model.getElementById("NamedElement").getDelegate();
factory.createGeneralization(clss, "Element");
// Namespace
clss = (Class_)model.getElementById("Namespace").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// PackageableElement
clss = (Class_)model.getElementById("PackageableElement").getDelegate();
factory.createGeneralization(clss, "ParameterableElement");
factory.createGeneralization(clss, "NamedElement");
// PackageImport
clss = (Class_)model.getElementById("PackageImport").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// ParameterableElement
clss = (Class_)model.getElementById("ParameterableElement").getDelegate();
factory.createGeneralization(clss, "Element");
// Realization
clss = (Class_)model.getElementById("Realization").getDelegate();
factory.createGeneralization(clss, "Abstraction");
// Relationship
clss = (Class_)model.getElementById("Relationship").getDelegate();
factory.createGeneralization(clss, "Element");
// TemplateableElement
clss = (Class_)model.getElementById("TemplateableElement").getDelegate();
factory.createGeneralization(clss, "Element");
// TemplateBinding
clss = (Class_)model.getElementById("TemplateBinding").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// TemplateParameter
clss = (Class_)model.getElementById("TemplateParameter").getDelegate();
factory.createGeneralization(clss, "Element");
// TemplateParameterSubstitution
clss = (Class_)model.getElementById("TemplateParameterSubstitution").getDelegate();
factory.createGeneralization(clss, "Element");
// TemplateSignature
clss = (Class_)model.getElementById("TemplateSignature").getDelegate();
factory.createGeneralization(clss, "Element");
// Type
clss = (Class_)model.getElementById("Type").getDelegate();
factory.createGeneralization(clss, "PackageableElement");
// TypedElement
clss = (Class_)model.getElementById("TypedElement").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Usage
clss = (Class_)model.getElementById("Usage").getDelegate();
factory.createGeneralization(clss, "Dependency");
// AnyReceiveEvent
clss = (Class_)model.getElementById("AnyReceiveEvent").getDelegate();
factory.createGeneralization(clss, "MessageEvent");
// Behavior
clss = (Class_)model.getElementById("Behavior").getDelegate();
factory.createGeneralization(clss, "Class");
// CallEvent
clss = (Class_)model.getElementById("CallEvent").getDelegate();
factory.createGeneralization(clss, "MessageEvent");
// ChangeEvent
clss = (Class_)model.getElementById("ChangeEvent").getDelegate();
factory.createGeneralization(clss, "Event");
// Event
clss = (Class_)model.getElementById("Event").getDelegate();
factory.createGeneralization(clss, "PackageableElement");
// FunctionBehavior
clss = (Class_)model.getElementById("FunctionBehavior").getDelegate();
factory.createGeneralization(clss, "OpaqueBehavior");
// MessageEvent
clss = (Class_)model.getElementById("MessageEvent").getDelegate();
factory.createGeneralization(clss, "Event");
// OpaqueBehavior
clss = (Class_)model.getElementById("OpaqueBehavior").getDelegate();
factory.createGeneralization(clss, "Behavior");
// SignalEvent
clss = (Class_)model.getElementById("SignalEvent").getDelegate();
factory.createGeneralization(clss, "MessageEvent");
// TimeEvent
clss = (Class_)model.getElementById("TimeEvent").getDelegate();
factory.createGeneralization(clss, "Event");
// Trigger
clss = (Class_)model.getElementById("Trigger").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Substitution
clss = (Class_)model.getElementById("Substitution").getDelegate();
factory.createGeneralization(clss, "Realization");
// BehavioralFeature
clss = (Class_)model.getElementById("BehavioralFeature").getDelegate();
factory.createGeneralization(clss, "Feature");
factory.createGeneralization(clss, "Namespace");
// Classifier
clss = (Class_)model.getElementById("Classifier").getDelegate();
factory.createGeneralization(clss, "Namespace");
factory.createGeneralization(clss, "Type");
factory.createGeneralization(clss, "TemplateableElement");
factory.createGeneralization(clss, "RedefinableElement");
// ClassifierTemplateParameter
clss = (Class_)model.getElementById("ClassifierTemplateParameter").getDelegate();
factory.createGeneralization(clss, "TemplateParameter");
// Feature
clss = (Class_)model.getElementById("Feature").getDelegate();
factory.createGeneralization(clss, "RedefinableElement");
// Generalization
clss = (Class_)model.getElementById("Generalization").getDelegate();
factory.createGeneralization(clss, "DirectedRelationship");
// GeneralizationSet
clss = (Class_)model.getElementById("GeneralizationSet").getDelegate();
factory.createGeneralization(clss, "PackageableElement");
// InstanceSpecification
clss = (Class_)model.getElementById("InstanceSpecification").getDelegate();
factory.createGeneralization(clss, "DeploymentTarget");
factory.createGeneralization(clss, "PackageableElement");
factory.createGeneralization(clss, "DeployedArtifact");
// InstanceValue
clss = (Class_)model.getElementById("InstanceValue").getDelegate();
factory.createGeneralization(clss, "ValueSpecification");
// Operation
clss = (Class_)model.getElementById("Operation").getDelegate();
factory.createGeneralization(clss, "TemplateableElement");
factory.createGeneralization(clss, "ParameterableElement");
factory.createGeneralization(clss, "BehavioralFeature");
// OperationTemplateParameter
clss = (Class_)model.getElementById("OperationTemplateParameter").getDelegate();
factory.createGeneralization(clss, "TemplateParameter");
// Parameter
clss = (Class_)model.getElementById("Parameter").getDelegate();
factory.createGeneralization(clss, "MultiplicityElement");
factory.createGeneralization(clss, "ConnectableElement");
// ParameterSet
clss = (Class_)model.getElementById("ParameterSet").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// Property
clss = (Class_)model.getElementById("Property").getDelegate();
factory.createGeneralization(clss, "ConnectableElement");
factory.createGeneralization(clss, "DeploymentTarget");
factory.createGeneralization(clss, "StructuralFeature");
// RedefinableElement
clss = (Class_)model.getElementById("RedefinableElement").getDelegate();
factory.createGeneralization(clss, "NamedElement");
// RedefinableTemplateSignature
clss = (Class_)model.getElementById("RedefinableTemplateSignature").getDelegate();
factory.createGeneralization(clss, "RedefinableElement");
factory.createGeneralization(clss, "TemplateSignature");
// Slot
clss = (Class_)model.getElementById("Slot").getDelegate();
factory.createGeneralization(clss, "Element");
// StructuralFeature
clss = (Class_)model.getElementById("StructuralFeature").getDelegate();
factory.createGeneralization(clss, "MultiplicityElement");
factory.createGeneralization(clss, "TypedElement");
factory.createGeneralization(clss, "Feature");
// ValueSpecificationAction
clss = (Class_)model.getElementById("ValueSpecificationAction").getDelegate();
factory.createGeneralization(clss, "Action");
// VariableAction
clss = (Class_)model.getElementById("VariableAction").getDelegate();
factory.createGeneralization(clss, "Action");
// WriteLinkAction
clss = (Class_)model.getElementById("WriteLinkAction").getDelegate();
factory.createGeneralization(clss, "LinkAction");
// WriteStructuralFeatureAction
clss = (Class_)model.getElementById("WriteStructuralFeatureAction").getDelegate();
factory.createGeneralization(clss, "StructuralFeatureAction");
// WriteVariableAction
clss = (Class_)model.getElementById("WriteVariableAction").getDelegate();
factory.createGeneralization(clss, "VariableAction");
// AcceptCallAction
clss = (Class_)model.getElementById("AcceptCallAction").getDelegate();
factory.createGeneralization(clss, "AcceptEventAction");
// AcceptEventAction
clss = (Class_)model.getElementById("AcceptEventAction").getDelegate();
factory.createGeneralization(clss, "Action");
// Action
clss = (Class_)model.getElementById("Action").getDelegate();
factory.createGeneralization(clss, "ExecutableNode");
// ActionInputPin
clss = (Class_)model.getElementById("ActionInputPin").getDelegate();
factory.createGeneralization(clss, "InputPin");
// AddStructuralFeatureValueAction
clss = (Class_)model.getElementById("AddStructuralFeatureValueAction").getDelegate();
factory.createGeneralization(clss, "WriteStructuralFeatureAction");
// AddVariableValueAction
clss = (Class_)model.getElementById("AddVariableValueAction").getDelegate();
factory.createGeneralization(clss, "WriteVariableAction");
// BroadcastSignalAction
clss = (Class_)model.getElementById("BroadcastSignalAction").getDelegate();
factory.createGeneralization(clss, "InvocationAction");
// CallAction
clss = (Class_)model.getElementById("CallAction").getDelegate();
factory.createGeneralization(clss, "InvocationAction");
// CallBehaviorAction
clss = (Class_)model.getElementById("CallBehaviorAction").getDelegate();
factory.createGeneralization(clss, "CallAction");
// CallOperationAction
clss = (Class_)model.getElementById("CallOperationAction").getDelegate();
factory.createGeneralization(clss, "CallAction");
// Clause
clss = (Class_)model.getElementById("Clause").getDelegate();
factory.createGeneralization(clss, "Element");
// ClearAssociationAction
clss = (Class_)model.getElementById("ClearAssociationAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ClearStructuralFeatureAction
clss = (Class_)model.getElementById("ClearStructuralFeatureAction").getDelegate();
factory.createGeneralization(clss, "StructuralFeatureAction");
// ClearVariableAction
clss = (Class_)model.getElementById("ClearVariableAction").getDelegate();
factory.createGeneralization(clss, "VariableAction");
// ConditionalNode
clss = (Class_)model.getElementById("ConditionalNode").getDelegate();
factory.createGeneralization(clss, "StructuredActivityNode");
// CreateLinkAction
clss = (Class_)model.getElementById("CreateLinkAction").getDelegate();
factory.createGeneralization(clss, "WriteLinkAction");
// CreateLinkObjectAction
clss = (Class_)model.getElementById("CreateLinkObjectAction").getDelegate();
factory.createGeneralization(clss, "CreateLinkAction");
// CreateObjectAction
clss = (Class_)model.getElementById("CreateObjectAction").getDelegate();
factory.createGeneralization(clss, "Action");
// DestroyLinkAction
clss = (Class_)model.getElementById("DestroyLinkAction").getDelegate();
factory.createGeneralization(clss, "WriteLinkAction");
// DestroyObjectAction
clss = (Class_)model.getElementById("DestroyObjectAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ExpansionNode
clss = (Class_)model.getElementById("ExpansionNode").getDelegate();
factory.createGeneralization(clss, "ObjectNode");
// ExpansionRegion
clss = (Class_)model.getElementById("ExpansionRegion").getDelegate();
factory.createGeneralization(clss, "StructuredActivityNode");
// InputPin
clss = (Class_)model.getElementById("InputPin").getDelegate();
factory.createGeneralization(clss, "Pin");
// InvocationAction
clss = (Class_)model.getElementById("InvocationAction").getDelegate();
factory.createGeneralization(clss, "Action");
// LinkAction
clss = (Class_)model.getElementById("LinkAction").getDelegate();
factory.createGeneralization(clss, "Action");
// LinkEndCreationData
clss = (Class_)model.getElementById("LinkEndCreationData").getDelegate();
factory.createGeneralization(clss, "LinkEndData");
// LinkEndData
clss = (Class_)model.getElementById("LinkEndData").getDelegate();
factory.createGeneralization(clss, "Element");
// LinkEndDestructionData
clss = (Class_)model.getElementById("LinkEndDestructionData").getDelegate();
factory.createGeneralization(clss, "LinkEndData");
// LoopNode
clss = (Class_)model.getElementById("LoopNode").getDelegate();
factory.createGeneralization(clss, "StructuredActivityNode");
// OpaqueAction
clss = (Class_)model.getElementById("OpaqueAction").getDelegate();
factory.createGeneralization(clss, "Action");
// OutputPin
clss = (Class_)model.getElementById("OutputPin").getDelegate();
factory.createGeneralization(clss, "Pin");
// Pin
clss = (Class_)model.getElementById("Pin").getDelegate();
factory.createGeneralization(clss, "ObjectNode");
factory.createGeneralization(clss, "MultiplicityElement");
// QualifierValue
clss = (Class_)model.getElementById("QualifierValue").getDelegate();
factory.createGeneralization(clss, "Element");
// RaiseExceptionAction
clss = (Class_)model.getElementById("RaiseExceptionAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReadExtentAction
clss = (Class_)model.getElementById("ReadExtentAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReadIsClassifiedObjectAction
clss = (Class_)model.getElementById("ReadIsClassifiedObjectAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReadLinkAction
clss = (Class_)model.getElementById("ReadLinkAction").getDelegate();
factory.createGeneralization(clss, "LinkAction");
// ReadLinkObjectEndAction
clss = (Class_)model.getElementById("ReadLinkObjectEndAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReadLinkObjectEndQualifierAction
clss = (Class_)model.getElementById("ReadLinkObjectEndQualifierAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReadSelfAction
clss = (Class_)model.getElementById("ReadSelfAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReadStructuralFeatureAction
clss = (Class_)model.getElementById("ReadStructuralFeatureAction").getDelegate();
factory.createGeneralization(clss, "StructuralFeatureAction");
// ReadVariableAction
clss = (Class_)model.getElementById("ReadVariableAction").getDelegate();
factory.createGeneralization(clss, "VariableAction");
// ReclassifyObjectAction
clss = (Class_)model.getElementById("ReclassifyObjectAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ReduceAction
clss = (Class_)model.getElementById("ReduceAction").getDelegate();
factory.createGeneralization(clss, "Action");
// RemoveStructuralFeatureValueAction
clss = (Class_)model.getElementById("RemoveStructuralFeatureValueAction").getDelegate();
factory.createGeneralization(clss, "WriteStructuralFeatureAction");
// RemoveVariableValueAction
clss = (Class_)model.getElementById("RemoveVariableValueAction").getDelegate();
factory.createGeneralization(clss, "WriteVariableAction");
// ReplyAction
clss = (Class_)model.getElementById("ReplyAction").getDelegate();
factory.createGeneralization(clss, "Action");
// SendObjectAction
clss = (Class_)model.getElementById("SendObjectAction").getDelegate();
factory.createGeneralization(clss, "InvocationAction");
// SendSignalAction
clss = (Class_)model.getElementById("SendSignalAction").getDelegate();
factory.createGeneralization(clss, "InvocationAction");
// SequenceNode
clss = (Class_)model.getElementById("SequenceNode").getDelegate();
factory.createGeneralization(clss, "StructuredActivityNode");
// StartClassifierBehaviorAction
clss = (Class_)model.getElementById("StartClassifierBehaviorAction").getDelegate();
factory.createGeneralization(clss, "Action");
// StartObjectBehaviorAction
clss = (Class_)model.getElementById("StartObjectBehaviorAction").getDelegate();
factory.createGeneralization(clss, "CallAction");
// StructuralFeatureAction
clss = (Class_)model.getElementById("StructuralFeatureAction").getDelegate();
factory.createGeneralization(clss, "Action");
// StructuredActivityNode
clss = (Class_)model.getElementById("StructuredActivityNode").getDelegate();
factory.createGeneralization(clss, "Namespace");
factory.createGeneralization(clss, "ActivityGroup");
factory.createGeneralization(clss, "Action");
// TestIdentityAction
clss = (Class_)model.getElementById("TestIdentityAction").getDelegate();
factory.createGeneralization(clss, "Action");
// UnmarshallAction
clss = (Class_)model.getElementById("UnmarshallAction").getDelegate();
factory.createGeneralization(clss, "Action");
// ValuePin
clss = (Class_)model.getElementById("ValuePin").getDelegate();
factory.createGeneralization(clss, "InputPin");
}
private void constructAssociations()
{
Package pkg = null;
String packageId = null;
Association assoc = null;
Property prop = null;
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_selection_objectNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_selection_objectNode", "A_selection_objectNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "objectNode",
"A_selection_objectNode-objectNode",
"ObjectNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ObjectNode-selection A_selection_objectNode-objectNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_inState_objectNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inState_objectNode", "A_inState_objectNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "objectNode",
"A_inState_objectNode-objectNode",
"ObjectNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ObjectNode-inState A_inState_objectNode-objectNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_upperBound_objectNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_upperBound_objectNode", "A_upperBound_objectNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "objectNode",
"A_upperBound_objectNode-objectNode",
"ObjectNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ObjectNode-upperBound A_upperBound_objectNode-objectNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_transformation_objectFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_transformation_objectFlow", "A_transformation_objectFlow");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "objectFlow",
"A_transformation_objectFlow-objectFlow",
"ObjectFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ObjectFlow-transformation A_transformation_objectFlow-objectFlow ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_selection_objectFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_selection_objectFlow", "A_selection_objectFlow");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "objectFlow",
"A_selection_objectFlow-objectFlow",
"ObjectFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ObjectFlow-selection A_selection_objectFlow-objectFlow ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_joinSpec_joinNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_joinSpec_joinNode", "A_joinSpec_joinNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "joinNode",
"A_joinSpec_joinNode-joinNode",
"JoinNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "JoinNode-joinSpec A_joinSpec_joinNode-joinNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_interruptingEdge_interrupts
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_interruptingEdge_interrupts", "A_interruptingEdge_interrupts");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "InterruptibleActivityRegion-interruptingEdge ActivityEdge-interrupts ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_handler_protectedNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_handler_protectedNode", "A_handler_protectedNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ExecutableNode-handler ExceptionHandler-protectedNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_handlerBody_exceptionHandler
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_handlerBody_exceptionHandler", "A_handlerBody_exceptionHandler");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "exceptionHandler",
"A_handlerBody_exceptionHandler-exceptionHandler",
"ExceptionHandler",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExceptionHandler-handlerBody A_handlerBody_exceptionHandler-exceptionHandler ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_exceptionType_exceptionHandler
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_exceptionType_exceptionHandler", "A_exceptionType_exceptionHandler");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "exceptionHandler",
"A_exceptionType_exceptionHandler-exceptionHandler",
"ExceptionHandler",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExceptionHandler-exceptionType A_exceptionType_exceptionHandler-exceptionHandler ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_exceptionInput_exceptionHandler
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_exceptionInput_exceptionHandler", "A_exceptionInput_exceptionHandler");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "exceptionHandler",
"A_exceptionInput_exceptionHandler-exceptionHandler",
"ExceptionHandler",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExceptionHandler-exceptionInput A_exceptionInput_exceptionHandler-exceptionHandler ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_decisionInput_decisionNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_decisionInput_decisionNode", "A_decisionInput_decisionNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "decisionNode",
"A_decisionInput_decisionNode-decisionNode",
"DecisionNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DecisionNode-decisionInput A_decisionInput_decisionNode-decisionNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_decisionInputFlow_decisionNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_decisionInputFlow_decisionNode", "A_decisionInputFlow_decisionNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "decisionNode",
"A_decisionInputFlow_decisionNode-decisionNode",
"DecisionNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DecisionNode-decisionInputFlow A_decisionInputFlow_decisionNode-decisionNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_represents_activityPartition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_represents_activityPartition", "A_represents_activityPartition");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activityPartition",
"A_represents_activityPartition-activityPartition",
"ActivityPartition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActivityPartition-represents A_represents_activityPartition-activityPartition ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_subpartition_superPartition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_subpartition_superPartition", "A_subpartition_superPartition");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityPartition-subpartition ActivityPartition-superPartition ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_edge_inPartition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_edge_inPartition", "A_edge_inPartition");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityPartition-edge ActivityEdge-inPartition ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_parameter_activityParameterNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_parameter_activityParameterNode", "A_parameter_activityParameterNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activityParameterNode",
"A_parameter_activityParameterNode-activityParameterNode",
"ActivityParameterNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActivityParameterNode-parameter A_parameter_activityParameterNode-activityParameterNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_inInterruptibleRegion_node
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inInterruptibleRegion_node", "A_inInterruptibleRegion_node");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityNode-inInterruptibleRegion InterruptibleActivityRegion-node ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_inPartition_node
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inPartition_node", "A_inPartition_node");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityNode-inPartition ActivityPartition-node ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_incoming_target_node
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_incoming_target_node", "A_incoming_target_node");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityNode-incoming ActivityEdge-target ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_outgoing_source_node
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_outgoing_source_node", "A_outgoing_source_node");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityNode-outgoing ActivityEdge-source ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_redefinedNode_activityNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedNode_activityNode", "A_redefinedNode_activityNode");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activityNode",
"A_redefinedNode_activityNode-activityNode",
"ActivityNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActivityNode-redefinedNode A_redefinedNode_activityNode-activityNode ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_containedEdge_inGroup
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_containedEdge_inGroup", "A_containedEdge_inGroup");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityGroup-containedEdge ActivityEdge-inGroup ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_containedNode_inGroup
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_containedNode_inGroup", "A_containedNode_inGroup");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityGroup-containedNode ActivityNode-inGroup ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_subgroup_superGroup
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_subgroup_superGroup", "A_subgroup_superGroup");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "ActivityGroup-subgroup ActivityGroup-superGroup ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_redefinedEdge_activityEdge
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedEdge_activityEdge", "A_redefinedEdge_activityEdge");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activityEdge",
"A_redefinedEdge_activityEdge-activityEdge",
"ActivityEdge",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActivityEdge-redefinedEdge A_redefinedEdge_activityEdge-activityEdge ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_weight_activityEdge
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_weight_activityEdge", "A_weight_activityEdge");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activityEdge",
"A_weight_activityEdge-activityEdge",
"ActivityEdge",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActivityEdge-weight A_weight_activityEdge-activityEdge ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_guard_activityEdge
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_guard_activityEdge", "A_guard_activityEdge");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activityEdge",
"A_guard_activityEdge-activityEdge",
"ActivityEdge",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActivityEdge-guard A_guard_activityEdge-activityEdge ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_structuredNode_activity
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_structuredNode_activity", "A_structuredNode_activity");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "Activity-structuredNode StructuredActivityNode-activity ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_group_inActivity
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_group_inActivity", "A_group_inActivity");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "Activity-group ActivityGroup-inActivity ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_node_activity
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_node_activity", "A_node_activity");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "Activity-node ActivityNode-activity ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_variable_activityScope
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_variable_activityScope", "A_variable_activityScope");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "Activity-variable Variable-activityScope ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_edge_activity
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_edge_activity", "A_edge_activity");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
factory.createAssociationEnds(assoc, "Activity-edge ActivityEdge-activity ");
packageId = this.artifact.getUrn() + "#" + "Activities";
// UML.Activities.A_partition_activity
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_partition_activity", "A_partition_activity");
mapping.mapAssociation(assoc, "UML.Activities", this);
// create owned ends
prop = factory.createProperty(assoc, "activity",
"A_partition_activity-activity",
"Activity",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Activity-partition A_partition_activity-activity ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_event_timeObservation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_event_timeObservation", "A_event_timeObservation");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "timeObservation",
"A_event_timeObservation-timeObservation",
"TimeObservation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeObservation-event A_event_timeObservation-timeObservation ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_max_timeInterval
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_max_timeInterval", "A_max_timeInterval");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "timeInterval",
"A_max_timeInterval-timeInterval",
"TimeInterval",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeInterval-max A_max_timeInterval-timeInterval ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_min_timeInterval
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_min_timeInterval", "A_min_timeInterval");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "timeInterval",
"A_min_timeInterval-timeInterval",
"TimeInterval",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeInterval-min A_min_timeInterval-timeInterval ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_expr_timeExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_expr_timeExpression", "A_expr_timeExpression");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "timeExpression",
"A_expr_timeExpression-timeExpression",
"TimeExpression",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeExpression-expr A_expr_timeExpression-timeExpression ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_observation_timeExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_observation_timeExpression", "A_observation_timeExpression");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "timeExpression",
"A_observation_timeExpression-timeExpression",
"TimeExpression",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeExpression-observation A_observation_timeExpression-timeExpression ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_specification_timeConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_specification_timeConstraint", "A_specification_timeConstraint");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "timeConstraint",
"A_specification_timeConstraint-timeConstraint",
"TimeConstraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeConstraint-specification A_specification_timeConstraint-timeConstraint ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_subExpression_owningExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_subExpression_owningExpression", "A_subExpression_owningExpression");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
factory.createAssociationEnds(assoc, "StringExpression-subExpression StringExpression-owningExpression ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_result_opaqueExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_opaqueExpression", "A_result_opaqueExpression");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "opaqueExpression",
"A_result_opaqueExpression-opaqueExpression",
"OpaqueExpression",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "OpaqueExpression-result A_result_opaqueExpression-opaqueExpression ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_behavior_opaqueExpression
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_behavior_opaqueExpression", "A_behavior_opaqueExpression");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "opaqueExpression",
"A_behavior_opaqueExpression-opaqueExpression",
"OpaqueExpression",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "OpaqueExpression-behavior A_behavior_opaqueExpression-opaqueExpression ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_specification_intervalConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_specification_intervalConstraint", "A_specification_intervalConstraint");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "intervalConstraint",
"A_specification_intervalConstraint-intervalConstraint",
"IntervalConstraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "IntervalConstraint-specification A_specification_intervalConstraint-intervalConstraint ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_max_interval
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_max_interval", "A_max_interval");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "interval",
"A_max_interval-interval",
"Interval",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interval-max A_max_interval-interval ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_min_interval
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_min_interval", "A_min_interval");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "interval",
"A_min_interval-interval",
"Interval",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interval-min A_min_interval-interval ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_operand_expression
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_operand_expression", "A_operand_expression");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "expression",
"A_operand_expression-expression",
"Expression",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Expression-operand A_operand_expression-expression ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_event_durationObservation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_event_durationObservation", "A_event_durationObservation");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "durationObservation",
"A_event_durationObservation-durationObservation",
"DurationObservation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DurationObservation-event A_event_durationObservation-durationObservation ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_max_durationInterval
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_max_durationInterval", "A_max_durationInterval");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "durationInterval",
"A_max_durationInterval-durationInterval",
"DurationInterval",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DurationInterval-max A_max_durationInterval-durationInterval ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_min_durationInterval
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_min_durationInterval", "A_min_durationInterval");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "durationInterval",
"A_min_durationInterval-durationInterval",
"DurationInterval",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DurationInterval-min A_min_durationInterval-durationInterval ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_specification_durationConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_specification_durationConstraint", "A_specification_durationConstraint");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "durationConstraint",
"A_specification_durationConstraint-durationConstraint",
"DurationConstraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DurationConstraint-specification A_specification_durationConstraint-durationConstraint ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_expr_duration
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_expr_duration", "A_expr_duration");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "duration",
"A_expr_duration-duration",
"Duration",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Duration-expr A_expr_duration-duration ");
packageId = this.artifact.getUrn() + "#" + "Values";
// UML.Values.A_observation_duration
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_observation_duration", "A_observation_duration");
mapping.mapAssociation(assoc, "UML.Values", this);
// create owned ends
prop = factory.createProperty(assoc, "duration",
"A_observation_duration-duration",
"Duration",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Duration-observation A_observation_duration-duration ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_extensionPoint_useCase
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extensionPoint_useCase", "A_extensionPoint_useCase");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
factory.createAssociationEnds(assoc, "UseCase-extensionPoint ExtensionPoint-useCase ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_include_includingCase
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_include_includingCase", "A_include_includingCase");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
factory.createAssociationEnds(assoc, "UseCase-include Include-includingCase ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_subject_useCase
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_subject_useCase", "A_subject_useCase");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
factory.createAssociationEnds(assoc, "UseCase-subject Classifier-useCase ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_extend_extension
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extend_extension", "A_extend_extension");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
factory.createAssociationEnds(assoc, "UseCase-extend Extend-extension ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_addition_include
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_addition_include", "A_addition_include");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
prop = factory.createProperty(assoc, "include",
"A_addition_include-include",
"Include",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Include-addition A_addition_include-include ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_extensionLocation_extension
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extensionLocation_extension", "A_extensionLocation_extension");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
prop = factory.createProperty(assoc, "extension",
"A_extensionLocation_extension-extension",
"Extend",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Extend-extensionLocation A_extensionLocation_extension-extension ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_condition_extend
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_condition_extend", "A_condition_extend");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
prop = factory.createProperty(assoc, "extend",
"A_condition_extend-extend",
"Extend",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Extend-condition A_condition_extend-extend ");
packageId = this.artifact.getUrn() + "#" + "UseCases";
// UML.UseCases.A_extendedCase_extend
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extendedCase_extend", "A_extendedCase_extend");
mapping.mapAssociation(assoc, "UML.UseCases", this);
// create owned ends
prop = factory.createProperty(assoc, "extend",
"A_extendedCase_extend-extend",
"Extend",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Extend-extendedCase A_extendedCase_extend-extend ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_part_structuredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_part_structuredClassifier", "A_part_structuredClassifier");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "structuredClassifier",
"A_part_structuredClassifier-structuredClassifier",
"StructuredClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuredClassifier-part A_part_structuredClassifier-structuredClassifier ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedConnector_structuredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedConnector_structuredClassifier", "A_ownedConnector_structuredClassifier");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "structuredClassifier",
"A_ownedConnector_structuredClassifier-structuredClassifier",
"StructuredClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuredClassifier-ownedConnector A_ownedConnector_structuredClassifier-structuredClassifier ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedAttribute_structuredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedAttribute_structuredClassifier", "A_ownedAttribute_structuredClassifier");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "structuredClassifier",
"A_ownedAttribute_structuredClassifier-structuredClassifier",
"StructuredClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuredClassifier-ownedAttribute A_ownedAttribute_structuredClassifier-structuredClassifier ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_role_structuredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_role_structuredClassifier", "A_role_structuredClassifier");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "structuredClassifier",
"A_role_structuredClassifier-structuredClassifier",
"StructuredClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuredClassifier-role A_role_structuredClassifier-structuredClassifier ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_protocol_port
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_protocol_port", "A_protocol_port");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "port",
"A_protocol_port-port",
"Port",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Port-protocol A_protocol_port-port ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_required_port
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_required_port", "A_required_port");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "port",
"A_required_port-port",
"Port",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Port-required A_required_port-port ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_redefinedPort_port
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedPort_port", "A_redefinedPort_port");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "port",
"A_redefinedPort_port-port",
"Port",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Port-redefinedPort A_redefinedPort_port-port ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_provided_port
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_provided_port", "A_provided_port");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "port",
"A_provided_port-port",
"Port",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Port-provided A_provided_port-port ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedPort_encapsulatedClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedPort_encapsulatedClassifier", "A_ownedPort_encapsulatedClassifier");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "encapsulatedClassifier",
"A_ownedPort_encapsulatedClassifier-encapsulatedClassifier",
"EncapsulatedClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "EncapsulatedClassifier-ownedPort A_ownedPort_encapsulatedClassifier-encapsulatedClassifier ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_partWithPort_connectorEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_partWithPort_connectorEnd", "A_partWithPort_connectorEnd");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "connectorEnd",
"A_partWithPort_connectorEnd-connectorEnd",
"ConnectorEnd",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConnectorEnd-partWithPort A_partWithPort_connectorEnd-connectorEnd ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_definingEnd_connectorEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_definingEnd_connectorEnd", "A_definingEnd_connectorEnd");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "connectorEnd",
"A_definingEnd_connectorEnd-connectorEnd",
"ConnectorEnd",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConnectorEnd-definingEnd A_definingEnd_connectorEnd-connectorEnd ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_end_connector
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_end_connector", "A_end_connector");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "connector",
"A_end_connector-connector",
"Connector",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Connector-end A_end_connector-connector ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_redefinedConnector_connector
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedConnector_connector", "A_redefinedConnector_connector");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "connector",
"A_redefinedConnector_connector-connector",
"Connector",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Connector-redefinedConnector A_redefinedConnector_connector-connector ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_contract_connector
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_contract_connector", "A_contract_connector");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "connector",
"A_contract_connector-connector",
"Connector",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Connector-contract A_contract_connector-connector ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_type_connector
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_type_connector", "A_type_connector");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "connector",
"A_type_connector-connector",
"Connector",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Connector-type A_type_connector-connector ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_connectableElement_templateParameter_parameteredElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_connectableElement_templateParameter_parameteredElement", "A_connectableElement_templateParameter_parameteredElement");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "ConnectableElement-templateParameter ConnectableElementTemplateParameter-parameteredElement ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_end_role
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_end_role", "A_end_role");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "ConnectableElement-end ConnectorEnd-role ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_realizingClassifier_componentRealization
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_realizingClassifier_componentRealization", "A_realizingClassifier_componentRealization");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "componentRealization",
"A_realizingClassifier_componentRealization-componentRealization",
"ComponentRealization",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ComponentRealization-realizingClassifier A_realizingClassifier_componentRealization-componentRealization ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_required_component
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_required_component", "A_required_component");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "component",
"A_required_component-component",
"Component",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Component-required A_required_component-component ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_packagedElement_component
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_packagedElement_component", "A_packagedElement_component");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "component",
"A_packagedElement_component-component",
"Component",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Component-packagedElement A_packagedElement_component-component ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_realization_abstraction_component
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_realization_abstraction_component", "A_realization_abstraction_component");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Component-realization ComponentRealization-abstraction ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_provided_component
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_provided_component", "A_provided_component");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "component",
"A_provided_component-component",
"Component",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Component-provided A_provided_component-component ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_type_collaborationUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_type_collaborationUse", "A_type_collaborationUse");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "collaborationUse",
"A_type_collaborationUse-collaborationUse",
"CollaborationUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CollaborationUse-type A_type_collaborationUse-collaborationUse ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_roleBinding_collaborationUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_roleBinding_collaborationUse", "A_roleBinding_collaborationUse");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "collaborationUse",
"A_roleBinding_collaborationUse-collaborationUse",
"CollaborationUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CollaborationUse-roleBinding A_roleBinding_collaborationUse-collaborationUse ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_collaborationRole_collaboration
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_collaborationRole_collaboration", "A_collaborationRole_collaboration");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "collaboration",
"A_collaborationRole_collaboration-collaboration",
"Collaboration",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Collaboration-collaborationRole A_collaborationRole_collaboration-collaboration ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedOperation_class
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedOperation_class", "A_ownedOperation_class");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Class-ownedOperation Operation-class ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_superClass_class
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_superClass_class", "A_superClass_class");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "class",
"A_superClass_class-class",
"Class",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Class-superClass A_superClass_class-class ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_extension_metaclass
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extension_metaclass", "A_extension_metaclass");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Class-extension Extension-metaclass ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedAttribute_class
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedAttribute_class", "A_ownedAttribute_class");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Class-ownedAttribute Property-class ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_nestedClassifier_nestingClass
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_nestedClassifier_nestingClass", "A_nestedClassifier_nestingClass");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "nestingClass",
"A_nestedClassifier_nestingClass-nestingClass",
"Class",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Class-nestedClassifier A_nestedClassifier_nestingClass-nestingClass ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedReception_class
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedReception_class", "A_ownedReception_class");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "class",
"A_ownedReception_class-class",
"Class",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Class-ownedReception A_ownedReception_class-class ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_memberEnd_association
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_memberEnd_association", "A_memberEnd_association");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Association-memberEnd Property-association ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_endType_association
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_endType_association", "A_endType_association");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "association",
"A_endType_association-association",
"Association",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Association-endType A_endType_association-association ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_ownedEnd_owningAssociation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedEnd_owningAssociation", "A_ownedEnd_owningAssociation");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Association-ownedEnd Property-owningAssociation ");
packageId = this.artifact.getUrn() + "#" + "StructuredClassifiers";
// UML.StructuredClassifiers.A_navigableOwnedEnd_association
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_navigableOwnedEnd_association", "A_navigableOwnedEnd_association");
mapping.mapAssociation(assoc, "UML.StructuredClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "association",
"A_navigableOwnedEnd_association-association",
"Association",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Association-navigableOwnedEnd A_navigableOwnedEnd_association-association ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_incoming_target_vertex
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_incoming_target_vertex", "A_incoming_target_vertex");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "Vertex-incoming Transition-target ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_outgoing_source_vertex
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_outgoing_source_vertex", "A_outgoing_source_vertex");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "Vertex-outgoing Transition-source ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_trigger_transition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_trigger_transition", "A_trigger_transition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "transition",
"A_trigger_transition-transition",
"Transition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Transition-trigger A_trigger_transition-transition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_guard_transition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_guard_transition", "A_guard_transition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "transition",
"A_guard_transition-transition",
"Transition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Transition-guard A_guard_transition-transition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_redefinedTransition_transition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedTransition_transition", "A_redefinedTransition_transition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "transition",
"A_redefinedTransition_transition-transition",
"Transition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Transition-redefinedTransition A_redefinedTransition_transition-transition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_redefinitionContext_transition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinitionContext_transition", "A_redefinitionContext_transition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "transition",
"A_redefinitionContext_transition-transition",
"Transition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Transition-redefinitionContext A_redefinitionContext_transition-transition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_effect_transition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_effect_transition", "A_effect_transition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "transition",
"A_effect_transition-transition",
"Transition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Transition-effect A_effect_transition-transition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_submachineState_submachine
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_submachineState_submachine", "A_submachineState_submachine");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "StateMachine-submachineState State-submachine ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_connectionPoint_stateMachine
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_connectionPoint_stateMachine", "A_connectionPoint_stateMachine");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "StateMachine-connectionPoint Pseudostate-stateMachine ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_region_stateMachine
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_region_stateMachine", "A_region_stateMachine");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "StateMachine-region Region-stateMachine ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_extendedStateMachine_stateMachine
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extendedStateMachine_stateMachine", "A_extendedStateMachine_stateMachine");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "stateMachine",
"A_extendedStateMachine_stateMachine-stateMachine",
"StateMachine",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StateMachine-extendedStateMachine A_extendedStateMachine_stateMachine-stateMachine ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_redefinitionContext_vertex
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinitionContext_vertex", "A_redefinitionContext_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "vertex",
"A_redefinitionContext_state-state",
"Vertex",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-redefinitionContext A_redefinitionContext_state-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_region_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_region_state", "A_region_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "State-region Region-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_stateInvariant_owningState
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_stateInvariant_owningState", "A_stateInvariant_owningState");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "owningState",
"A_stateInvariant_owningState-owningState",
"State",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-stateInvariant A_stateInvariant_owningState-owningState ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_redefinedVertex_vertex
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedVertex_vertex", "A_redefinedState_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "vertex",
"A_redefinedState_state-state",
"Vertex",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-redefinedState A_redefinedState_state-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_deferrableTrigger_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_deferrableTrigger_state", "A_deferrableTrigger_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "state",
"A_deferrableTrigger_state-state",
"State",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-deferrableTrigger A_deferrableTrigger_state-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_connection_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_connection_state", "A_connection_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "State-connection ConnectionPointReference-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_entry_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_entry_state", "A_entry_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "state",
"A_entry_state-state",
"State",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-entry A_entry_state-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_connectionPoint_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_connectionPoint_state", "A_connectionPoint_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "State-connectionPoint Pseudostate-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_doActivity_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_doActivity_state", "A_doActivity_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "state",
"A_doActivity_state-state",
"State",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-doActivity A_doActivity_state-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_exit_state
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_exit_state", "A_exit_state");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "state",
"A_exit_state-state",
"State",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "State-exit A_exit_state-state ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_extendedRegion_region
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extendedRegion_region", "A_extendedRegion_region");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "region",
"A_extendedRegion_region-region",
"Region",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Region-extendedRegion A_extendedRegion_region-region ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_subvertex_container
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_subvertex_container", "A_subvertex_container");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "Region-subvertex Vertex-container ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_redefinitionContext_region
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinitionContext_region", "A_redefinitionContext_region");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "region",
"A_redefinitionContext_region-region",
"Region",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Region-redefinitionContext A_redefinitionContext_region-region ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_transition_container
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_transition_container", "A_transition_container");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "Region-transition Transition-container ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_referred_protocolTransition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_referred_protocolTransition", "A_referred_protocolTransition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "protocolTransition",
"A_referred_protocolTransition-protocolTransition",
"ProtocolTransition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ProtocolTransition-referred A_referred_protocolTransition-protocolTransition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_preCondition_protocolTransition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_preCondition_protocolTransition", "A_preCondition_protocolTransition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "protocolTransition",
"A_preCondition_protocolTransition-protocolTransition",
"ProtocolTransition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ProtocolTransition-preCondition A_preCondition_protocolTransition-protocolTransition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_postCondition_owningTransition
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_postCondition_owningTransition", "A_postCondition_owningTransition");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "owningTransition",
"A_postCondition_owningTransition-owningTransition",
"ProtocolTransition",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ProtocolTransition-postCondition A_postCondition_owningTransition-owningTransition ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_conformance_specificMachine
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_conformance_specificMachine", "A_conformance_specificMachine");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
factory.createAssociationEnds(assoc, "ProtocolStateMachine-conformance ProtocolConformance-specificMachine ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_generalMachine_protocolConformance
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_generalMachine_protocolConformance", "A_generalMachine_protocolConformance");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "protocolConformance",
"A_generalMachine_protocolConformance-protocolConformance",
"ProtocolConformance",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ProtocolConformance-generalMachine A_generalMachine_protocolConformance-protocolConformance ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_entry_connectionPointReference
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_entry_connectionPointReference", "A_entry_connectionPointReference");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "connectionPointReference",
"A_entry_connectionPointReference-connectionPointReference",
"ConnectionPointReference",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConnectionPointReference-entry A_entry_connectionPointReference-connectionPointReference ");
packageId = this.artifact.getUrn() + "#" + "StateMachines";
// UML.StateMachines.A_exit_connectionPointReference
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_exit_connectionPointReference", "A_exit_connectionPointReference");
mapping.mapAssociation(assoc, "UML.StateMachines", this);
// create owned ends
prop = factory.createProperty(assoc, "connectionPointReference",
"A_exit_connectionPointReference-connectionPointReference",
"ConnectionPointReference",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConnectionPointReference-exit A_exit_connectionPointReference-connectionPointReference ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedAttribute_owningSignal
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedAttribute_owningSignal", "A_ownedAttribute_owningSignal");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "owningSignal",
"A_ownedAttribute_owningSignal-owningSignal",
"Signal",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Signal-ownedAttribute A_ownedAttribute_owningSignal-owningSignal ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_signal_reception
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_signal_reception", "A_signal_reception");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "reception",
"A_signal_reception-reception",
"Reception",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Reception-signal A_signal_reception-reception ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_contract_interfaceRealization
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_contract_interfaceRealization", "A_contract_interfaceRealization");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "interfaceRealization",
"A_contract_interfaceRealization-interfaceRealization",
"InterfaceRealization",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InterfaceRealization-contract A_contract_interfaceRealization-interfaceRealization ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_protocol_interface
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_protocol_interface", "A_protocol_interface");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "interface",
"A_protocol_interface-interface",
"Interface",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interface-protocol A_protocol_interface-interface ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedReception_interface
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedReception_interface", "A_ownedReception_interface");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "interface",
"A_ownedReception_interface-interface",
"Interface",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interface-ownedReception A_ownedReception_interface-interface ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_redefinedInterface_interface
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedInterface_interface", "A_redefinedInterface_interface");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "interface",
"A_redefinedInterface_interface-interface",
"Interface",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interface-redefinedInterface A_redefinedInterface_interface-interface ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_nestedClassifier_interface
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_nestedClassifier_interface", "A_nestedClassifier_interface");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "interface",
"A_nestedClassifier_interface-interface",
"Interface",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interface-nestedClassifier A_nestedClassifier_interface-interface ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedOperation_interface
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedOperation_interface", "A_ownedOperation_interface");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Interface-ownedOperation Operation-interface ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedAttribute_interface
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedAttribute_interface", "A_ownedAttribute_interface");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Interface-ownedAttribute Property-interface ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_classifier_enumerationLiteral
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifier_enumerationLiteral", "A_classifier_enumerationLiteral");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "enumerationLiteral",
"A_classifier_enumerationLiteral-enumerationLiteral",
"EnumerationLiteral",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "EnumerationLiteral-classifier A_classifier_enumerationLiteral-enumerationLiteral ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedLiteral_enumeration
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedLiteral_enumeration", "A_ownedLiteral_enumeration");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "Enumeration-ownedLiteral EnumerationLiteral-enumeration ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedAttribute_datatype
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedAttribute_datatype", "A_ownedAttribute_datatype");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "DataType-ownedAttribute Property-datatype ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedOperation_datatype
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedOperation_datatype", "A_ownedOperation_datatype");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "DataType-ownedOperation Operation-datatype ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_ownedBehavior_behavioredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedBehavior_behavioredClassifier", "A_ownedBehavior_behavioredClassifier");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "behavioredClassifier",
"A_ownedBehavior_behavioredClassifier-behavioredClassifier",
"BehavioredClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BehavioredClassifier-ownedBehavior A_ownedBehavior_behavioredClassifier-behavioredClassifier ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_classifierBehavior_behavioredClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifierBehavior_behavioredClassifier", "A_classifierBehavior_behavioredClassifier");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
prop = factory.createProperty(assoc, "behavioredClassifier",
"A_classifierBehavior_behavioredClassifier-behavioredClassifier",
"BehavioredClassifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BehavioredClassifier-classifierBehavior A_classifierBehavior_behavioredClassifier-behavioredClassifier ");
packageId = this.artifact.getUrn() + "#" + "SimpleClassifiers";
// UML.SimpleClassifiers.A_interfaceRealization_implementingClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_interfaceRealization_implementingClassifier", "A_interfaceRealization_implementingClassifier");
mapping.mapAssociation(assoc, "UML.SimpleClassifiers", this);
// create owned ends
factory.createAssociationEnds(assoc, "BehavioredClassifier-interfaceRealization InterfaceRealization-implementingClassifier ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_profile_stereotype
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_profile_stereotype", "A_profile_stereotype");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "stereotype",
"A_profile_stereotype-stereotype",
"Stereotype",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Stereotype-profile A_profile_stereotype-stereotype ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_icon_stereotype
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_icon_stereotype", "A_icon_stereotype");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "stereotype",
"A_icon_stereotype-stereotype",
"Stereotype",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Stereotype-icon A_icon_stereotype-stereotype ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_appliedProfile_profileApplication
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_appliedProfile_profileApplication", "A_appliedProfile_profileApplication");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "profileApplication",
"A_appliedProfile_profileApplication-profileApplication",
"ProfileApplication",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ProfileApplication-appliedProfile A_appliedProfile_profileApplication-profileApplication ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_metaclassReference_profile
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_metaclassReference_profile", "A_metaclassReference_profile");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "profile",
"A_metaclassReference_profile-profile",
"Profile",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Profile-metaclassReference A_metaclassReference_profile-profile ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_metamodelReference_profile
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_metamodelReference_profile", "A_metamodelReference_profile");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "profile",
"A_metamodelReference_profile-profile",
"Profile",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Profile-metamodelReference A_metamodelReference_profile-profile ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_mergedPackage_packageMerge
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_mergedPackage_packageMerge", "A_mergedPackage_packageMerge");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "packageMerge",
"A_mergedPackage_packageMerge-packageMerge",
"PackageMerge",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "PackageMerge-mergedPackage A_mergedPackage_packageMerge-packageMerge ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_packagedElement_owningPackage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_packagedElement_owningPackage", "A_packagedElement_owningPackage");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "owningPackage",
"A_packagedElement_owningPackage-owningPackage",
"Package",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Package-packagedElement A_packagedElement_owningPackage-owningPackage ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_packageMerge_receivingPackage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_packageMerge_receivingPackage", "A_packageMerge_receivingPackage");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
factory.createAssociationEnds(assoc, "Package-packageMerge PackageMerge-receivingPackage ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_nestedPackage_nestingPackage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_nestedPackage_nestingPackage", "A_nestedPackage_nestingPackage");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
factory.createAssociationEnds(assoc, "Package-nestedPackage Package-nestingPackage ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_profileApplication_applyingPackage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_profileApplication_applyingPackage", "A_profileApplication_applyingPackage");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
factory.createAssociationEnds(assoc, "Package-profileApplication ProfileApplication-applyingPackage ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_ownedType_package
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedType_package", "A_ownedType_package");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
factory.createAssociationEnds(assoc, "Package-ownedType Type-package ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_ownedStereotype_owningPackage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedStereotype_owningPackage", "A_ownedStereotype_owningPackage");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "owningPackage",
"A_ownedStereotype_owningPackage-owningPackage",
"Package",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Package-ownedStereotype A_ownedStereotype_owningPackage-owningPackage ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_type_extensionEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_type_extensionEnd", "A_type_extensionEnd");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "extensionEnd",
"A_type_extensionEnd-extensionEnd",
"ExtensionEnd",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExtensionEnd-type A_type_extensionEnd-extensionEnd ");
packageId = this.artifact.getUrn() + "#" + "Packages";
// UML.Packages.A_ownedEnd_extension
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedEnd_extension", "A_ownedEnd_extension");
mapping.mapAssociation(assoc, "UML.Packages", this);
// create owned ends
prop = factory.createProperty(assoc, "extension",
"A_ownedEnd_extension-extension",
"Extension",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Extension-ownedEnd A_ownedEnd_extension-extension ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_invariant_stateInvariant
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_invariant_stateInvariant", "A_invariant_stateInvariant");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "stateInvariant",
"A_invariant_stateInvariant-stateInvariant",
"StateInvariant",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StateInvariant-invariant A_invariant_stateInvariant-stateInvariant ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_covered_stateInvariant
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_covered_stateInvariant", "A_covered_stateInvariant");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "stateInvariant",
"A_covered_stateInvariant-stateInvariant",
"StateInvariant",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StateInvariant-covered A_covered_stateInvariant-stateInvariant ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_covered_events
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_covered_events", "A_covered_events");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "events",
"A_covered_events-events",
"OccurrenceSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "OccurrenceSpecification-covered A_covered_events-events ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_toBefore_after
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_toBefore_after", "A_toBefore_after");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "OccurrenceSpecification-toBefore GeneralOrdering-after ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_message_messageEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_message_messageEnd", "A_message_messageEnd");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "messageEnd",
"A_message_messageEnd-messageEnd",
"MessageEnd",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "2");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "MessageEnd-message A_message_messageEnd-messageEnd ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_signature_message
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_signature_message", "A_signature_message");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "message",
"A_signature_message-message",
"Message",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Message-signature A_signature_message-message ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_connector_message
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_connector_message", "A_connector_message");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "message",
"A_connector_message-message",
"Message",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Message-connector A_connector_message-message ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_receiveEvent_endMessage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_receiveEvent_endMessage", "A_receiveEvent_endMessage");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "endMessage",
"A_receiveEvent_endMessage-endMessage",
"Message",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Message-receiveEvent A_receiveEvent_endMessage-endMessage ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_sendEvent_endMessage
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_sendEvent_endMessage", "A_sendEvent_endMessage");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "endMessage",
"A_sendEvent_endMessage-endMessage",
"Message",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Message-sendEvent A_sendEvent_endMessage-endMessage ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_argument_message
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_argument_message", "A_argument_message");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "message",
"A_argument_message-message",
"Message",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Message-argument A_argument_message-message ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_selector_lifeline
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_selector_lifeline", "A_selector_lifeline");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "lifeline",
"A_selector_lifeline-lifeline",
"Lifeline",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Lifeline-selector A_selector_lifeline-lifeline ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_decomposedAs_lifeline
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_decomposedAs_lifeline", "A_decomposedAs_lifeline");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "lifeline",
"A_decomposedAs_lifeline-lifeline",
"Lifeline",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Lifeline-decomposedAs A_decomposedAs_lifeline-lifeline ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_represents_lifeline
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_represents_lifeline", "A_represents_lifeline");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "lifeline",
"A_represents_lifeline-lifeline",
"Lifeline",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Lifeline-represents A_represents_lifeline-lifeline ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_returnValueRecipient_interactionUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_returnValueRecipient_interactionUse", "A_returnValueRecipient_interactionUse");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionUse",
"A_returnValueRecipient_interactionUse-interactionUse",
"InteractionUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionUse-returnValueRecipient A_returnValueRecipient_interactionUse-interactionUse ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_refersTo_interactionUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_refersTo_interactionUse", "A_refersTo_interactionUse");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionUse",
"A_refersTo_interactionUse-interactionUse",
"InteractionUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionUse-refersTo A_refersTo_interactionUse-interactionUse ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_returnValue_interactionUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_returnValue_interactionUse", "A_returnValue_interactionUse");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionUse",
"A_returnValue_interactionUse-interactionUse",
"InteractionUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionUse-returnValue A_returnValue_interactionUse-interactionUse ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_argument_interactionUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_argument_interactionUse", "A_argument_interactionUse");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionUse",
"A_argument_interactionUse-interactionUse",
"InteractionUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionUse-argument A_argument_interactionUse-interactionUse ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_actualGate_interactionUse
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_actualGate_interactionUse", "A_actualGate_interactionUse");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionUse",
"A_actualGate_interactionUse-interactionUse",
"InteractionUse",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionUse-actualGate A_actualGate_interactionUse-interactionUse ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_fragment_enclosingOperand
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_fragment_enclosingOperand", "A_fragment_enclosingOperand");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "InteractionOperand-fragment InteractionFragment-enclosingOperand ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_guard_interactionOperand
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_guard_interactionOperand", "A_guard_interactionOperand");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionOperand",
"A_guard_interactionOperand-interactionOperand",
"InteractionOperand",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionOperand-guard A_guard_interactionOperand-interactionOperand ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_generalOrdering_interactionFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_generalOrdering_interactionFragment", "A_generalOrdering_interactionFragment");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionFragment",
"A_generalOrdering_interactionFragment-interactionFragment",
"InteractionFragment",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionFragment-generalOrdering A_generalOrdering_interactionFragment-interactionFragment ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_covered_coveredBy
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_covered_coveredBy", "A_covered_coveredBy");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "InteractionFragment-covered Lifeline-coveredBy ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_minint_interactionConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_minint_interactionConstraint", "A_minint_interactionConstraint");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionConstraint",
"A_minint_interactionConstraint-interactionConstraint",
"InteractionConstraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionConstraint-minint A_minint_interactionConstraint-interactionConstraint ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_maxint_interactionConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_maxint_interactionConstraint", "A_maxint_interactionConstraint");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interactionConstraint",
"A_maxint_interactionConstraint-interactionConstraint",
"InteractionConstraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InteractionConstraint-maxint A_maxint_interactionConstraint-interactionConstraint ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_action_interaction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_action_interaction", "A_action_interaction");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interaction",
"A_action_interaction-interaction",
"Interaction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interaction-action A_action_interaction-interaction ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_formalGate_interaction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_formalGate_interaction", "A_formalGate_interaction");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "interaction",
"A_formalGate_interaction-interaction",
"Interaction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Interaction-formalGate A_formalGate_interaction-interaction ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_lifeline_interaction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_lifeline_interaction", "A_lifeline_interaction");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "Interaction-lifeline Lifeline-interaction ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_fragment_enclosingInteraction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_fragment_enclosingInteraction", "A_fragment_enclosingInteraction");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "Interaction-fragment InteractionFragment-enclosingInteraction ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_message_interaction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_message_interaction", "A_message_interaction");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "Interaction-message Message-interaction ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_before_toAfter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_before_toAfter", "A_before_toAfter");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
factory.createAssociationEnds(assoc, "GeneralOrdering-before OccurrenceSpecification-toAfter ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_finish_executionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_finish_executionSpecification", "A_finish_executionSpecification");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "executionSpecification",
"A_finish_executionSpecification-executionSpecification",
"ExecutionSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExecutionSpecification-finish A_finish_executionSpecification-executionSpecification ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_start_executionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_start_executionSpecification", "A_start_executionSpecification");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "executionSpecification",
"A_start_executionSpecification-executionSpecification",
"ExecutionSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExecutionSpecification-start A_start_executionSpecification-executionSpecification ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_execution_executionOccurrenceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_execution_executionOccurrenceSpecification", "A_execution_executionOccurrenceSpecification");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "executionOccurrenceSpecification",
"A_execution_executionOccurrenceSpecification-executionOccurrenceSpecification",
"ExecutionOccurrenceSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "2");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ExecutionOccurrenceSpecification-execution A_execution_executionOccurrenceSpecification-executionOccurrenceSpecification ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_message_considerIgnoreFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_message_considerIgnoreFragment", "A_message_considerIgnoreFragment");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "considerIgnoreFragment",
"A_message_considerIgnoreFragment-considerIgnoreFragment",
"ConsiderIgnoreFragment",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConsiderIgnoreFragment-message A_message_considerIgnoreFragment-considerIgnoreFragment ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_cfragmentGate_combinedFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_cfragmentGate_combinedFragment", "A_cfragmentGate_combinedFragment");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "combinedFragment",
"A_cfragmentGate_combinedFragment-combinedFragment",
"CombinedFragment",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CombinedFragment-cfragmentGate A_cfragmentGate_combinedFragment-combinedFragment ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_operand_combinedFragment
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_operand_combinedFragment", "A_operand_combinedFragment");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "combinedFragment",
"A_operand_combinedFragment-combinedFragment",
"CombinedFragment",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CombinedFragment-operand A_operand_combinedFragment-combinedFragment ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_behavior_behaviorExecutionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_behavior_behaviorExecutionSpecification", "A_behavior_behaviorExecutionSpecification");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "behaviorExecutionSpecification",
"A_behavior_behaviorExecutionSpecification-behaviorExecutionSpecification",
"BehaviorExecutionSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BehaviorExecutionSpecification-behavior A_behavior_behaviorExecutionSpecification-behaviorExecutionSpecification ");
packageId = this.artifact.getUrn() + "#" + "Interactions";
// UML.Interactions.A_action_actionExecutionSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_action_actionExecutionSpecification", "A_action_actionExecutionSpecification");
mapping.mapAssociation(assoc, "UML.Interactions", this);
// create owned ends
prop = factory.createProperty(assoc, "actionExecutionSpecification",
"A_action_actionExecutionSpecification-actionExecutionSpecification",
"ActionExecutionSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActionExecutionSpecification-action A_action_actionExecutionSpecification-actionExecutionSpecification ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_represented_representation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_represented_representation", "A_represented_representation");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "representation",
"A_represented_representation-representation",
"InformationItem",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationItem-represented A_represented_representation-representation ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_conveyed_conveyingFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_conveyed_conveyingFlow", "A_conveyed_conveyingFlow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "conveyingFlow",
"A_conveyed_conveyingFlow-conveyingFlow",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-conveyed A_conveyed_conveyingFlow-conveyingFlow ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_realizingActivityEdge_informationFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_realizingActivityEdge_informationFlow", "A_realizingActivityEdge_informationFlow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "informationFlow",
"A_realizingActivityEdge_informationFlow-informationFlow",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-realizingActivityEdge A_realizingActivityEdge_informationFlow-informationFlow ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_realizingMessage_informationFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_realizingMessage_informationFlow", "A_realizingMessage_informationFlow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "informationFlow",
"A_realizingMessage_informationFlow-informationFlow",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-realizingMessage A_realizingMessage_informationFlow-informationFlow ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_informationSource_informationFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_informationSource_informationFlow", "A_informationSource_informationFlow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "informationFlow",
"A_informationSource_informationFlow-informationFlow",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-informationSource A_informationSource_informationFlow-informationFlow ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_realization_abstraction_flow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_realization_abstraction_flow", "A_realization_abstraction_flow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "abstraction",
"A_realization_abstraction_flow-abstraction",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-realization A_realization_abstraction_flow-abstraction ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_realizingConnector_informationFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_realizingConnector_informationFlow", "A_realizingConnector_informationFlow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "informationFlow",
"A_realizingConnector_informationFlow-informationFlow",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-realizingConnector A_realizingConnector_informationFlow-informationFlow ");
packageId = this.artifact.getUrn() + "#" + "InformationFlows";
// UML.InformationFlows.A_informationTarget_informationFlow
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_informationTarget_informationFlow", "A_informationTarget_informationFlow");
mapping.mapAssociation(assoc, "UML.InformationFlows", this);
// create owned ends
prop = factory.createProperty(assoc, "informationFlow",
"A_informationTarget_informationFlow-informationFlow",
"InformationFlow",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InformationFlow-informationTarget A_informationTarget_informationFlow-informationFlow ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_nestedNode_node
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_nestedNode_node", "A_nestedNode_node");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "node",
"A_nestedNode_node-node",
"Node",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Node-nestedNode A_nestedNode_node-node ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_utilizedElement_manifestation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_utilizedElement_manifestation", "A_utilizedElement_manifestation");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "manifestation",
"A_utilizedElement_manifestation-manifestation",
"Manifestation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Manifestation-utilizedElement A_utilizedElement_manifestation-manifestation ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_deployedElement_deploymentTarget
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_deployedElement_deploymentTarget", "A_deployedElement_deploymentTarget");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "deploymentTarget",
"A_deployedElement_deploymentTarget-deploymentTarget",
"DeploymentTarget",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DeploymentTarget-deployedElement A_deployedElement_deploymentTarget-deploymentTarget ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_deployment_location
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_deployment_location", "A_deployment_location");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
factory.createAssociationEnds(assoc, "DeploymentTarget-deployment Deployment-location ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_deployedArtifact_deploymentForArtifact
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_deployedArtifact_deploymentForArtifact", "A_deployedArtifact_deploymentForArtifact");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "deploymentForArtifact",
"A_deployedArtifact_deploymentForArtifact-deploymentForArtifact",
"Deployment",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Deployment-deployedArtifact A_deployedArtifact_deploymentForArtifact-deploymentForArtifact ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_configuration_deployment
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_configuration_deployment", "A_configuration_deployment");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
factory.createAssociationEnds(assoc, "Deployment-configuration DeploymentSpecification-deployment ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_ownedAttribute_artifact
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedAttribute_artifact", "A_ownedAttribute_artifact");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "artifact",
"A_ownedAttribute_artifact-artifact",
"Artifact",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Artifact-ownedAttribute A_ownedAttribute_artifact-artifact ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_ownedOperation_artifact
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedOperation_artifact", "A_ownedOperation_artifact");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "artifact",
"A_ownedOperation_artifact-artifact",
"Artifact",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Artifact-ownedOperation A_ownedOperation_artifact-artifact ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_nestedArtifact_artifact
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_nestedArtifact_artifact", "A_nestedArtifact_artifact");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "artifact",
"A_nestedArtifact_artifact-artifact",
"Artifact",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Artifact-nestedArtifact A_nestedArtifact_artifact-artifact ");
packageId = this.artifact.getUrn() + "#" + "Deployments";
// UML.Deployments.A_manifestation_artifact
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_manifestation_artifact", "A_manifestation_artifact");
mapping.mapAssociation(assoc, "UML.Deployments", this);
// create owned ends
prop = factory.createProperty(assoc, "artifact",
"A_manifestation_artifact-artifact",
"Artifact",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Artifact-manifestation A_manifestation_artifact-artifact ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_type_typedElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_type_typedElement", "A_type_typedElement");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "typedElement",
"A_type_typedElement-typedElement",
"TypedElement",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TypedElement-type A_type_typedElement-typedElement ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedParameter_signature
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameter_signature", "A_ownedParameter_signature");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "TemplateSignature-ownedParameter TemplateParameter-signature ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_parameter_templateSignature
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_parameter_templateSignature", "A_parameter_templateSignature");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "templateSignature",
"A_parameter_templateSignature-templateSignature",
"TemplateSignature",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateSignature-parameter A_parameter_templateSignature-templateSignature ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_actual_templateParameterSubstitution
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_actual_templateParameterSubstitution", "A_actual_templateParameterSubstitution");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "templateParameterSubstitution",
"A_actual_templateParameterSubstitution-templateParameterSubstitution",
"TemplateParameterSubstitution",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateParameterSubstitution-actual A_actual_templateParameterSubstitution-templateParameterSubstitution ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedActual_owningTemplateParameterSubstitution
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedActual_owningTemplateParameterSubstitution", "A_ownedActual_owningTemplateParameterSubstitution");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "owningTemplateParameterSubstitution",
"A_ownedActual_owningTemplateParameterSubstitution-owningTemplateParameterSubstitution",
"TemplateParameterSubstitution",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateParameterSubstitution-ownedActual A_ownedActual_owningTemplateParameterSubstitution-owningTemplateParameterSubstitution ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_formal_templateParameterSubstitution
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_formal_templateParameterSubstitution", "A_formal_templateParameterSubstitution");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "templateParameterSubstitution",
"A_formal_templateParameterSubstitution-templateParameterSubstitution",
"TemplateParameterSubstitution",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateParameterSubstitution-formal A_formal_templateParameterSubstitution-templateParameterSubstitution ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedDefault_templateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedDefault_templateParameter", "A_ownedDefault_templateParameter");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "templateParameter",
"A_ownedDefault_templateParameter-templateParameter",
"TemplateParameter",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateParameter-ownedDefault A_ownedDefault_templateParameter-templateParameter ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedParameteredElement_owningTemplateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameteredElement_owningTemplateParameter", "A_ownedParameteredElement_owningTemplateParameter");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "TemplateParameter-ownedParameteredElement ParameterableElement-owningTemplateParameter ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_default_templateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_default_templateParameter", "A_default_templateParameter");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "templateParameter",
"A_default_templateParameter-templateParameter",
"TemplateParameter",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateParameter-default A_default_templateParameter-templateParameter ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_parameteredElement_templateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_parameteredElement_templateParameter", "A_parameteredElement_templateParameter");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "TemplateParameter-parameteredElement ParameterableElement-templateParameter ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_signature_templateBinding
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_signature_templateBinding", "A_signature_templateBinding");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "templateBinding",
"A_signature_templateBinding-templateBinding",
"TemplateBinding",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TemplateBinding-signature A_signature_templateBinding-templateBinding ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_parameterSubstitution_templateBinding
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_parameterSubstitution_templateBinding", "A_parameterSubstitution_templateBinding");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "TemplateBinding-parameterSubstitution TemplateParameterSubstitution-templateBinding ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_templateBinding_boundElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_templateBinding_boundElement", "A_templateBinding_boundElement");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "TemplateableElement-templateBinding TemplateBinding-boundElement ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedTemplateSignature_template
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedTemplateSignature_template", "A_ownedTemplateSignature_template");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "TemplateableElement-ownedTemplateSignature TemplateSignature-template ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_relatedElement_relationship
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_relatedElement_relationship", "A_relatedElement_relationship");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "relationship",
"A_relatedElement_relationship-relationship",
"Relationship",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Relationship-relatedElement A_relatedElement_relationship-relationship ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_importedPackage_packageImport
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_importedPackage_packageImport", "A_importedPackage_packageImport");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "packageImport",
"A_importedPackage_packageImport-packageImport",
"PackageImport",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "PackageImport-importedPackage A_importedPackage_packageImport-packageImport ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_elementImport_importingNamespace
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_elementImport_importingNamespace", "A_elementImport_importingNamespace");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "Namespace-elementImport ElementImport-importingNamespace ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedMember_namespace
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedMember_namespace", "A_ownedMember_namespace");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "Namespace-ownedMember NamedElement-namespace ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedRule_context
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedRule_context", "A_ownedRule_context");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "Namespace-ownedRule Constraint-context ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_packageImport_importingNamespace
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_packageImport_importingNamespace", "A_packageImport_importingNamespace");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "Namespace-packageImport PackageImport-importingNamespace ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_member_memberNamespace
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_member_memberNamespace", "A_member_memberNamespace");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "memberNamespace",
"A_member_memberNamespace-memberNamespace",
"Namespace",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Namespace-member A_member_memberNamespace-memberNamespace ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_importedMember_namespace
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_importedMember_namespace", "A_importedMember_namespace");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "namespace",
"A_importedMember_namespace-namespace",
"Namespace",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Namespace-importedMember A_importedMember_namespace-namespace ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_nameExpression_namedElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_nameExpression_namedElement", "A_nameExpression_namedElement");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "namedElement",
"A_nameExpression_namedElement-namedElement",
"NamedElement",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "NamedElement-nameExpression A_nameExpression_namedElement-namedElement ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_clientDependency_client
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_clientDependency_client", "A_clientDependency_client");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "NamedElement-clientDependency Dependency-client ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_lowerValue_owningLower
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_lowerValue_owningLower", "A_lowerValue_owningLower");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "owningLower",
"A_lowerValue_owningLower-owningLower",
"MultiplicityElement",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "MultiplicityElement-lowerValue A_lowerValue_owningLower-owningLower ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_upperValue_owningUpper
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_upperValue_owningUpper", "A_upperValue_owningUpper");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "owningUpper",
"A_upperValue_owningUpper-owningUpper",
"MultiplicityElement",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "MultiplicityElement-upperValue A_upperValue_owningUpper-owningUpper ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_importedElement_import
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_importedElement_import", "A_importedElement_import");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "import",
"A_importedElement_import-import",
"ElementImport",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ElementImport-importedElement A_importedElement_import-import ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedElement_owner
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedElement_owner", "A_ownedElement_owner");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
factory.createAssociationEnds(assoc, "Element-ownedElement Element-owner ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_ownedComment_owningElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedComment_owningElement", "A_ownedComment_owningElement");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "owningElement",
"A_ownedComment_owningElement-owningElement",
"Element",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Element-ownedComment A_ownedComment_owningElement-owningElement ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_source_directedRelationship
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_source_directedRelationship", "A_source_directedRelationship");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "directedRelationship",
"A_source_directedRelationship-directedRelationship",
"DirectedRelationship",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DirectedRelationship-source A_source_directedRelationship-directedRelationship ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_target_directedRelationship
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_target_directedRelationship", "A_target_directedRelationship");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "directedRelationship",
"A_target_directedRelationship-directedRelationship",
"DirectedRelationship",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DirectedRelationship-target A_target_directedRelationship-directedRelationship ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_supplier_supplierDependency
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_supplier_supplierDependency", "A_supplier_supplierDependency");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "supplierDependency",
"A_supplier_supplierDependency-supplierDependency",
"Dependency",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Dependency-supplier A_supplier_supplierDependency-supplierDependency ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_constrainedElement_constraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_constrainedElement_constraint", "A_constrainedElement_constraint");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "constraint",
"A_constrainedElement_constraint-constraint",
"Constraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Constraint-constrainedElement A_constrainedElement_constraint-constraint ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_specification_owningConstraint
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_specification_owningConstraint", "A_specification_owningConstraint");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "owningConstraint",
"A_specification_owningConstraint-owningConstraint",
"Constraint",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Constraint-specification A_specification_owningConstraint-owningConstraint ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_annotatedElement_comment
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_annotatedElement_comment", "A_annotatedElement_comment");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "comment",
"A_annotatedElement_comment-comment",
"Comment",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Comment-annotatedElement A_annotatedElement_comment-comment ");
packageId = this.artifact.getUrn() + "#" + "CommonStructure";
// UML.CommonStructure.A_mapping_abstraction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_mapping_abstraction", "A_mapping_abstraction");
mapping.mapAssociation(assoc, "UML.CommonStructure", this);
// create owned ends
prop = factory.createProperty(assoc, "abstraction",
"A_mapping_abstraction-abstraction",
"Abstraction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Abstraction-mapping A_mapping_abstraction-abstraction ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_event_trigger
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_event_trigger", "A_event_trigger");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "trigger",
"A_event_trigger-trigger",
"Trigger",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Trigger-event A_event_trigger-trigger ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_port_trigger
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_port_trigger", "A_port_trigger");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "trigger",
"A_port_trigger-trigger",
"Trigger",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Trigger-port A_port_trigger-trigger ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_when_timeEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_when_timeEvent", "A_when_timeEvent");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "timeEvent",
"A_when_timeEvent-timeEvent",
"TimeEvent",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TimeEvent-when A_when_timeEvent-timeEvent ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_signal_signalEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_signal_signalEvent", "A_signal_signalEvent");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "signalEvent",
"A_signal_signalEvent-signalEvent",
"SignalEvent",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "SignalEvent-signal A_signal_signalEvent-signalEvent ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_changeExpression_changeEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_changeExpression_changeEvent", "A_changeExpression_changeEvent");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "changeEvent",
"A_changeExpression_changeEvent-changeEvent",
"ChangeEvent",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ChangeEvent-changeExpression A_changeExpression_changeEvent-changeEvent ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_operation_callEvent
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_operation_callEvent", "A_operation_callEvent");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "callEvent",
"A_operation_callEvent-callEvent",
"CallEvent",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CallEvent-operation A_operation_callEvent-callEvent ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_ownedParameterSet_behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameterSet_behavior", "A_ownedParameterSet_behavior");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "behavior",
"A_ownedParameterSet_behavior-behavior",
"Behavior",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Behavior-ownedParameterSet A_ownedParameterSet_behavior-behavior ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_context_behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_context_behavior", "A_context_behavior");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "behavior",
"A_context_behavior-behavior",
"Behavior",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Behavior-context A_context_behavior-behavior ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_precondition_behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_precondition_behavior", "A_precondition_behavior");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "behavior",
"A_precondition_behavior-behavior",
"Behavior",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Behavior-precondition A_precondition_behavior-behavior ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_postcondition_behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_postcondition_behavior", "A_postcondition_behavior");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "behavior",
"A_postcondition_behavior-behavior",
"Behavior",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Behavior-postcondition A_postcondition_behavior-behavior ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_redefinedBehavior_behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedBehavior_behavior", "A_redefinedBehavior_behavior");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "behavior",
"A_redefinedBehavior_behavior-behavior",
"Behavior",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Behavior-redefinedBehavior A_redefinedBehavior_behavior-behavior ");
packageId = this.artifact.getUrn() + "#" + "CommonBehavior";
// UML.CommonBehavior.A_ownedParameter_behavior
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameter_behavior", "A_ownedParameter_behavior");
mapping.mapAssociation(assoc, "UML.CommonBehavior", this);
// create owned ends
prop = factory.createProperty(assoc, "behavior",
"A_ownedParameter_behavior-behavior",
"Behavior",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Behavior-ownedParameter A_ownedParameter_behavior-behavior ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_definingFeature_slot
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_definingFeature_slot", "A_definingFeature_slot");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "slot",
"A_definingFeature_slot-slot",
"Slot",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Slot-definingFeature A_definingFeature_slot-slot ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_value_owningSlot
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_owningSlot", "A_value_owningSlot");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "owningSlot",
"A_value_owningSlot-owningSlot",
"Slot",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Slot-value A_value_owningSlot-owningSlot ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_extendedSignature_redefinableTemplateSignature
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_extendedSignature_redefinableTemplateSignature", "A_extendedSignature_redefinableTemplateSignature");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "redefinableTemplateSignature",
"A_extendedSignature_redefinableTemplateSignature-redefinableTemplateSignature",
"RedefinableTemplateSignature",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RedefinableTemplateSignature-extendedSignature A_extendedSignature_redefinableTemplateSignature-redefinableTemplateSignature ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_inheritedParameter_redefinableTemplateSignature
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inheritedParameter_redefinableTemplateSignature", "A_inheritedParameter_redefinableTemplateSignature");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "redefinableTemplateSignature",
"A_inheritedParameter_redefinableTemplateSignature-redefinableTemplateSignature",
"RedefinableTemplateSignature",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RedefinableTemplateSignature-inheritedParameter A_inheritedParameter_redefinableTemplateSignature-redefinableTemplateSignature ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_redefinitionContext_redefinableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinitionContext_redefinableElement", "A_redefinitionContext_redefinableElement");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "redefinableElement",
"A_redefinitionContext_redefinableElement-redefinableElement",
"RedefinableElement",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RedefinableElement-redefinitionContext A_redefinitionContext_redefinableElement-redefinableElement ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_redefinedElement_redefinableElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedElement_redefinableElement", "A_redefinedElement_redefinableElement");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "redefinableElement",
"A_redefinedElement_redefinableElement-redefinableElement",
"RedefinableElement",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RedefinableElement-redefinedElement A_redefinedElement_redefinableElement-redefinableElement ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_qualifier_associationEnd
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_qualifier_associationEnd", "A_qualifier_associationEnd");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Property-qualifier Property-associationEnd ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_redefinedProperty_property
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedProperty_property", "A_redefinedProperty_property");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "property",
"A_redefinedProperty_property-property",
"Property",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Property-redefinedProperty A_redefinedProperty_property-property ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_defaultValue_owningProperty
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_defaultValue_owningProperty", "A_defaultValue_owningProperty");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "owningProperty",
"A_defaultValue_owningProperty-owningProperty",
"Property",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Property-defaultValue A_defaultValue_owningProperty-owningProperty ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_opposite_property
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_opposite_property", "A_opposite_property");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "property",
"A_opposite_property-property",
"Property",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Property-opposite A_opposite_property-property ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_subsettedProperty_property
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_subsettedProperty_property", "A_subsettedProperty_property");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "property",
"A_subsettedProperty_property-property",
"Property",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Property-subsettedProperty A_subsettedProperty_property-property ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_condition_parameterSet
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_condition_parameterSet", "A_condition_parameterSet");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "parameterSet",
"A_condition_parameterSet-parameterSet",
"ParameterSet",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ParameterSet-condition A_condition_parameterSet-parameterSet ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_parameterSet_parameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_parameterSet_parameter", "A_parameterSet_parameter");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Parameter-parameterSet ParameterSet-parameter ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_defaultValue_owningParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_defaultValue_owningParameter", "A_defaultValue_owningParameter");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "owningParameter",
"A_defaultValue_owningParameter-owningParameter",
"Parameter",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Parameter-defaultValue A_defaultValue_owningParameter-owningParameter ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_type_operation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_type_operation", "A_type_operation");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "operation",
"A_type_operation-operation",
"Operation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Operation-type A_type_operation-operation ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_operation_templateParameter_parameteredElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_operation_templateParameter_parameteredElement", "A_operation_templateParameter_parameteredElement");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Operation-templateParameter OperationTemplateParameter-parameteredElement ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_bodyCondition_bodyContext
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_bodyCondition_bodyContext", "A_bodyCondition_bodyContext");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "bodyContext",
"A_bodyCondition_bodyContext-bodyContext",
"Operation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Operation-bodyCondition A_bodyCondition_bodyContext-bodyContext ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_postcondition_postContext
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_postcondition_postContext", "A_postcondition_postContext");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "postContext",
"A_postcondition_postContext-postContext",
"Operation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Operation-postcondition A_postcondition_postContext-postContext ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_redefinedOperation_operation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedOperation_operation", "A_redefinedOperation_operation");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "operation",
"A_redefinedOperation_operation-operation",
"Operation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Operation-redefinedOperation A_redefinedOperation_operation-operation ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_raisedException_operation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_raisedException_operation", "A_raisedException_operation");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "operation",
"A_raisedException_operation-operation",
"Operation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Operation-raisedException A_raisedException_operation-operation ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_precondition_preContext
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_precondition_preContext", "A_precondition_preContext");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "preContext",
"A_precondition_preContext-preContext",
"Operation",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Operation-precondition A_precondition_preContext-preContext ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_ownedParameter_operation
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameter_operation", "A_ownedParameter_operation");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Operation-ownedParameter Parameter-operation ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_instance_instanceValue
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_instance_instanceValue", "A_instance_instanceValue");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "instanceValue",
"A_instance_instanceValue-instanceValue",
"InstanceValue",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InstanceValue-instance A_instance_instanceValue-instanceValue ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_classifier_instanceSpecification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifier_instanceSpecification", "A_classifier_instanceSpecification");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "instanceSpecification",
"A_classifier_instanceSpecification-instanceSpecification",
"InstanceSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InstanceSpecification-classifier A_classifier_instanceSpecification-instanceSpecification ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_slot_owningInstance
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_slot_owningInstance", "A_slot_owningInstance");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "InstanceSpecification-slot Slot-owningInstance ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_specification_owningInstanceSpec
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_specification_owningInstanceSpec", "A_specification_owningInstanceSpec");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "owningInstanceSpec",
"A_specification_owningInstanceSpec-owningInstanceSpec",
"InstanceSpecification",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InstanceSpecification-specification A_specification_owningInstanceSpec-owningInstanceSpec ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_generalizationSet_generalization
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_generalizationSet_generalization", "A_generalizationSet_generalization");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Generalization-generalizationSet GeneralizationSet-generalization ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_general_generalization
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_general_generalization", "A_general_generalization");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "generalization",
"A_general_generalization-generalization",
"Generalization",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Generalization-general A_general_generalization-generalization ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_constrainingClassifier_classifierTemplateParameter
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_constrainingClassifier_classifierTemplateParameter", "A_constrainingClassifier_classifierTemplateParameter");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifierTemplateParameter",
"A_constrainingClassifier_classifierTemplateParameter-classifierTemplateParameter",
"ClassifierTemplateParameter",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ClassifierTemplateParameter-constrainingClassifier A_constrainingClassifier_classifierTemplateParameter-classifierTemplateParameter ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_classifier_templateParameter_parameteredElement
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifier_templateParameter_parameteredElement", "A_classifier_templateParameter_parameteredElement");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Classifier-templateParameter ClassifierTemplateParameter-parameteredElement ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_powertypeExtent_powertype
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_powertypeExtent_powertype", "A_powertypeExtent_powertype");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Classifier-powertypeExtent GeneralizationSet-powertype ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_generalization_specific
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_generalization_specific", "A_generalization_specific");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Classifier-generalization Generalization-specific ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_general_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_general_classifier", "A_general_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifier",
"A_general_classifier-classifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-general A_general_classifier-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_feature_featuringClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_feature_featuringClassifier", "A_feature_featuringClassifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Classifier-feature Feature-featuringClassifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_substitution_substitutingClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_substitution_substitutingClassifier", "A_substitution_substitutingClassifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Classifier-substitution Substitution-substitutingClassifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_representation_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_representation_classifier", "A_representation_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifier",
"A_representation_classifier-classifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-representation A_representation_classifier-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_ownedTemplateSignature_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedTemplateSignature_classifier", "A_ownedTemplateSignature_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "Classifier-ownedTemplateSignature RedefinableTemplateSignature-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_inheritedMember_inheritingClassifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inheritedMember_inheritingClassifier", "A_inheritedMember_inheritingClassifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "inheritingClassifier",
"A_inheritedMember_inheritingClassifier-inheritingClassifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-inheritedMember A_inheritedMember_inheritingClassifier-inheritingClassifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_redefinedClassifier_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_redefinedClassifier_classifier", "A_redefinedClassifier_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifier",
"A_redefinedClassifier_classifier-classifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-redefinedClassifier A_redefinedClassifier_classifier-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_collaborationUse_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_collaborationUse_classifier", "A_collaborationUse_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifier",
"A_collaborationUse_classifier-classifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-collaborationUse A_collaborationUse_classifier-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_attribute_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_attribute_classifier", "A_attribute_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifier",
"A_attribute_classifier-classifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-attribute A_attribute_classifier-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_ownedUseCase_classifier
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedUseCase_classifier", "A_ownedUseCase_classifier");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "classifier",
"A_ownedUseCase_classifier-classifier",
"Classifier",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Classifier-ownedUseCase A_ownedUseCase_classifier-classifier ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_ownedParameter_ownerFormalParam
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameter_ownerFormalParam", "A_ownedParameter_ownerFormalParam");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "ownerFormalParam",
"A_ownedParameter_ownerFormalParam-ownerFormalParam",
"BehavioralFeature",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BehavioralFeature-ownedParameter A_ownedParameter_ownerFormalParam-ownerFormalParam ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_raisedException_behavioralFeature
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_raisedException_behavioralFeature", "A_raisedException_behavioralFeature");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "behavioralFeature",
"A_raisedException_behavioralFeature-behavioralFeature",
"BehavioralFeature",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BehavioralFeature-raisedException A_raisedException_behavioralFeature-behavioralFeature ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_ownedParameterSet_behavioralFeature
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_ownedParameterSet_behavioralFeature", "A_ownedParameterSet_behavioralFeature");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "behavioralFeature",
"A_ownedParameterSet_behavioralFeature-behavioralFeature",
"BehavioralFeature",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BehavioralFeature-ownedParameterSet A_ownedParameterSet_behavioralFeature-behavioralFeature ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_method_specification
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_method_specification", "A_method_specification");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
factory.createAssociationEnds(assoc, "BehavioralFeature-method Behavior-specification ");
packageId = this.artifact.getUrn() + "#" + "Classification";
// UML.Classification.A_contract_substitution
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_contract_substitution", "A_contract_substitution");
mapping.mapAssociation(assoc, "UML.Classification", this);
// create owned ends
prop = factory.createProperty(assoc, "substitution",
"A_contract_substitution-substitution",
"Substitution",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Substitution-contract A_contract_substitution-substitution ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_value_valuePin
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_valuePin", "A_value_valuePin");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "valuePin",
"A_value_valuePin-valuePin",
"ValuePin",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ValuePin-value A_value_valuePin-valuePin ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_unmarshallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_unmarshallAction", "A_result_unmarshallAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "unmarshallAction",
"A_result_unmarshallAction-unmarshallAction",
"UnmarshallAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "UnmarshallAction-result A_result_unmarshallAction-unmarshallAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_unmarshallType_unmarshallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_unmarshallType_unmarshallAction", "A_unmarshallType_unmarshallAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "unmarshallAction",
"A_unmarshallType_unmarshallAction-unmarshallAction",
"UnmarshallAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "UnmarshallAction-unmarshallType A_unmarshallType_unmarshallAction-unmarshallAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_unmarshallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_unmarshallAction", "A_object_unmarshallAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "unmarshallAction",
"A_object_unmarshallAction-unmarshallAction",
"UnmarshallAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "UnmarshallAction-object A_object_unmarshallAction-unmarshallAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_first_testIdentityAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_first_testIdentityAction", "A_first_testIdentityAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "testIdentityAction",
"A_first_testIdentityAction-testIdentityAction",
"TestIdentityAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TestIdentityAction-first A_first_testIdentityAction-testIdentityAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_second_testIdentityAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_second_testIdentityAction", "A_second_testIdentityAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "testIdentityAction",
"A_second_testIdentityAction-testIdentityAction",
"TestIdentityAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TestIdentityAction-second A_second_testIdentityAction-testIdentityAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_testIdentityAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_testIdentityAction", "A_result_testIdentityAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "testIdentityAction",
"A_result_testIdentityAction-testIdentityAction",
"TestIdentityAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "TestIdentityAction-result A_result_testIdentityAction-testIdentityAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_structuredNodeOutput_structuredActivityNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_structuredNodeOutput_structuredActivityNode", "A_structuredNodeOutput_structuredActivityNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "structuredActivityNode",
"A_structuredNodeOutput_structuredActivityNode-structuredActivityNode",
"StructuredActivityNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuredActivityNode-structuredNodeOutput A_structuredNodeOutput_structuredActivityNode-structuredActivityNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_structuredNodeInput_structuredActivityNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_structuredNodeInput_structuredActivityNode", "A_structuredNodeInput_structuredActivityNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "structuredActivityNode",
"A_structuredNodeInput_structuredActivityNode-structuredActivityNode",
"StructuredActivityNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuredActivityNode-structuredNodeInput A_structuredNodeInput_structuredActivityNode-structuredActivityNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_edge_inStructuredNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_edge_inStructuredNode", "A_edge_inStructuredNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
factory.createAssociationEnds(assoc, "StructuredActivityNode-edge ActivityEdge-inStructuredNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_node_inStructuredNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_node_inStructuredNode", "A_node_inStructuredNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
factory.createAssociationEnds(assoc, "StructuredActivityNode-node ActivityNode-inStructuredNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_variable_scope
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_variable_scope", "A_variable_scope");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
factory.createAssociationEnds(assoc, "StructuredActivityNode-variable Variable-scope ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_structuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_structuralFeatureAction", "A_object_structuralFeatureAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "structuralFeatureAction",
"A_object_structuralFeatureAction-structuralFeatureAction",
"StructuralFeatureAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuralFeatureAction-object A_object_structuralFeatureAction-structuralFeatureAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_structuralFeature_structuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_structuralFeature_structuralFeatureAction", "A_structuralFeature_structuralFeatureAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "structuralFeatureAction",
"A_structuralFeature_structuralFeatureAction-structuralFeatureAction",
"StructuralFeatureAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StructuralFeatureAction-structuralFeature A_structuralFeature_structuralFeatureAction-structuralFeatureAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_startObjectBehaviorAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_startObjectBehaviorAction", "A_object_startObjectBehaviorAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "startObjectBehaviorAction",
"A_object_startObjectBehaviorAction-startObjectBehaviorAction",
"StartObjectBehaviorAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StartObjectBehaviorAction-object A_object_startObjectBehaviorAction-startObjectBehaviorAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_startClassifierBehaviorAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_startClassifierBehaviorAction", "A_object_startClassifierBehaviorAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "startClassifierBehaviorAction",
"A_object_startClassifierBehaviorAction-startClassifierBehaviorAction",
"StartClassifierBehaviorAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "StartClassifierBehaviorAction-object A_object_startClassifierBehaviorAction-startClassifierBehaviorAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_executableNode_sequenceNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_executableNode_sequenceNode", "A_executableNode_sequenceNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "sequenceNode",
"A_executableNode_sequenceNode-sequenceNode",
"SequenceNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "SequenceNode-executableNode A_executableNode_sequenceNode-sequenceNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_signal_sendSignalAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_signal_sendSignalAction", "A_signal_sendSignalAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "sendSignalAction",
"A_signal_sendSignalAction-sendSignalAction",
"SendSignalAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "SendSignalAction-signal A_signal_sendSignalAction-sendSignalAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_target_sendSignalAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_target_sendSignalAction", "A_target_sendSignalAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "sendSignalAction",
"A_target_sendSignalAction-sendSignalAction",
"SendSignalAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "SendSignalAction-target A_target_sendSignalAction-sendSignalAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_request_sendObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_request_sendObjectAction", "A_request_sendObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "sendObjectAction",
"A_request_sendObjectAction-sendObjectAction",
"SendObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "SendObjectAction-request A_request_sendObjectAction-sendObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_target_sendObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_target_sendObjectAction", "A_target_sendObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "sendObjectAction",
"A_target_sendObjectAction-sendObjectAction",
"SendObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "SendObjectAction-target A_target_sendObjectAction-sendObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_returnInformation_replyAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_returnInformation_replyAction", "A_returnInformation_replyAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "replyAction",
"A_returnInformation_replyAction-replyAction",
"ReplyAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReplyAction-returnInformation A_returnInformation_replyAction-replyAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_replyToCall_replyAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_replyToCall_replyAction", "A_replyToCall_replyAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "replyAction",
"A_replyToCall_replyAction-replyAction",
"ReplyAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReplyAction-replyToCall A_replyToCall_replyAction-replyAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_replyValue_replyAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_replyValue_replyAction", "A_replyValue_replyAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "replyAction",
"A_replyValue_replyAction-replyAction",
"ReplyAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReplyAction-replyValue A_replyValue_replyAction-replyAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_removeAt_removeVariableValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_removeAt_removeVariableValueAction", "A_removeAt_removeVariableValueAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "removeVariableValueAction",
"A_removeAt_removeVariableValueAction-removeVariableValueAction",
"RemoveVariableValueAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RemoveVariableValueAction-removeAt A_removeAt_removeVariableValueAction-removeVariableValueAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_removeAt_removeStructuralFeatureValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_removeAt_removeStructuralFeatureValueAction", "A_removeAt_removeStructuralFeatureValueAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "removeStructuralFeatureValueAction",
"A_removeAt_removeStructuralFeatureValueAction-removeStructuralFeatureValueAction",
"RemoveStructuralFeatureValueAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RemoveStructuralFeatureValueAction-removeAt A_removeAt_removeStructuralFeatureValueAction-removeStructuralFeatureValueAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_collection_reduceAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_collection_reduceAction", "A_collection_reduceAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "reduceAction",
"A_collection_reduceAction-reduceAction",
"ReduceAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReduceAction-collection A_collection_reduceAction-reduceAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_reducer_reduceAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_reducer_reduceAction", "A_reducer_reduceAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "reduceAction",
"A_reducer_reduceAction-reduceAction",
"ReduceAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReduceAction-reducer A_reducer_reduceAction-reduceAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_reduceAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_reduceAction", "A_result_reduceAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "reduceAction",
"A_result_reduceAction-reduceAction",
"ReduceAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReduceAction-result A_result_reduceAction-reduceAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_reclassifyObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_reclassifyObjectAction", "A_object_reclassifyObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "reclassifyObjectAction",
"A_object_reclassifyObjectAction-reclassifyObjectAction",
"ReclassifyObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReclassifyObjectAction-object A_object_reclassifyObjectAction-reclassifyObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_newClassifier_reclassifyObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_newClassifier_reclassifyObjectAction", "A_newClassifier_reclassifyObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "reclassifyObjectAction",
"A_newClassifier_reclassifyObjectAction-reclassifyObjectAction",
"ReclassifyObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReclassifyObjectAction-newClassifier A_newClassifier_reclassifyObjectAction-reclassifyObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_oldClassifier_reclassifyObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_oldClassifier_reclassifyObjectAction", "A_oldClassifier_reclassifyObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "reclassifyObjectAction",
"A_oldClassifier_reclassifyObjectAction-reclassifyObjectAction",
"ReclassifyObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReclassifyObjectAction-oldClassifier A_oldClassifier_reclassifyObjectAction-reclassifyObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readVariableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readVariableAction", "A_result_readVariableAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readVariableAction",
"A_result_readVariableAction-readVariableAction",
"ReadVariableAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadVariableAction-result A_result_readVariableAction-readVariableAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readStructuralFeatureAction", "A_result_readStructuralFeatureAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readStructuralFeatureAction",
"A_result_readStructuralFeatureAction-readStructuralFeatureAction",
"ReadStructuralFeatureAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadStructuralFeatureAction-result A_result_readStructuralFeatureAction-readStructuralFeatureAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readSelfAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readSelfAction", "A_result_readSelfAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readSelfAction",
"A_result_readSelfAction-readSelfAction",
"ReadSelfAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadSelfAction-result A_result_readSelfAction-readSelfAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_qualifier_readLinkObjectEndQualifierAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_qualifier_readLinkObjectEndQualifierAction", "A_qualifier_readLinkObjectEndQualifierAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkObjectEndQualifierAction",
"A_qualifier_readLinkObjectEndQualifierAction-readLinkObjectEndQualifierAction",
"ReadLinkObjectEndQualifierAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkObjectEndQualifierAction-qualifier A_qualifier_readLinkObjectEndQualifierAction-readLinkObjectEndQualifierAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_readLinkObjectEndQualifierAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_readLinkObjectEndQualifierAction", "A_object_readLinkObjectEndQualifierAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkObjectEndQualifierAction",
"A_object_readLinkObjectEndQualifierAction-readLinkObjectEndQualifierAction",
"ReadLinkObjectEndQualifierAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkObjectEndQualifierAction-object A_object_readLinkObjectEndQualifierAction-readLinkObjectEndQualifierAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readLinkObjectEndQualifierAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readLinkObjectEndQualifierAction", "A_result_readLinkObjectEndQualifierAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkObjectEndQualifierAction",
"A_result_readLinkObjectEndQualifierAction-readLinkObjectEndQualifierAction",
"ReadLinkObjectEndQualifierAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkObjectEndQualifierAction-result A_result_readLinkObjectEndQualifierAction-readLinkObjectEndQualifierAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_readLinkObjectEndAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_readLinkObjectEndAction", "A_object_readLinkObjectEndAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkObjectEndAction",
"A_object_readLinkObjectEndAction-readLinkObjectEndAction",
"ReadLinkObjectEndAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkObjectEndAction-object A_object_readLinkObjectEndAction-readLinkObjectEndAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_end_readLinkObjectEndAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_end_readLinkObjectEndAction", "A_end_readLinkObjectEndAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkObjectEndAction",
"A_end_readLinkObjectEndAction-readLinkObjectEndAction",
"ReadLinkObjectEndAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkObjectEndAction-end A_end_readLinkObjectEndAction-readLinkObjectEndAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readLinkObjectEndAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readLinkObjectEndAction", "A_result_readLinkObjectEndAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkObjectEndAction",
"A_result_readLinkObjectEndAction-readLinkObjectEndAction",
"ReadLinkObjectEndAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkObjectEndAction-result A_result_readLinkObjectEndAction-readLinkObjectEndAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readLinkAction", "A_result_readLinkAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readLinkAction",
"A_result_readLinkAction-readLinkAction",
"ReadLinkAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadLinkAction-result A_result_readLinkAction-readLinkAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_classifier_readIsClassifiedObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifier_readIsClassifiedObjectAction", "A_classifier_readIsClassifiedObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readIsClassifiedObjectAction",
"A_classifier_readIsClassifiedObjectAction-readIsClassifiedObjectAction",
"ReadIsClassifiedObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadIsClassifiedObjectAction-classifier A_classifier_readIsClassifiedObjectAction-readIsClassifiedObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readIsClassifiedObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readIsClassifiedObjectAction", "A_result_readIsClassifiedObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readIsClassifiedObjectAction",
"A_result_readIsClassifiedObjectAction-readIsClassifiedObjectAction",
"ReadIsClassifiedObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadIsClassifiedObjectAction-result A_result_readIsClassifiedObjectAction-readIsClassifiedObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_readIsClassifiedObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_readIsClassifiedObjectAction", "A_object_readIsClassifiedObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readIsClassifiedObjectAction",
"A_object_readIsClassifiedObjectAction-readIsClassifiedObjectAction",
"ReadIsClassifiedObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadIsClassifiedObjectAction-object A_object_readIsClassifiedObjectAction-readIsClassifiedObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_readExtentAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_readExtentAction", "A_result_readExtentAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readExtentAction",
"A_result_readExtentAction-readExtentAction",
"ReadExtentAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadExtentAction-result A_result_readExtentAction-readExtentAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_classifier_readExtentAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifier_readExtentAction", "A_classifier_readExtentAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "readExtentAction",
"A_classifier_readExtentAction-readExtentAction",
"ReadExtentAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ReadExtentAction-classifier A_classifier_readExtentAction-readExtentAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_exception_raiseExceptionAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_exception_raiseExceptionAction", "A_exception_raiseExceptionAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "raiseExceptionAction",
"A_exception_raiseExceptionAction-raiseExceptionAction",
"RaiseExceptionAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "RaiseExceptionAction-exception A_exception_raiseExceptionAction-raiseExceptionAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_qualifier_qualifierValue
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_qualifier_qualifierValue", "A_qualifier_qualifierValue");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "qualifierValue",
"A_qualifier_qualifierValue-qualifierValue",
"QualifierValue",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "QualifierValue-qualifier A_qualifier_qualifierValue-qualifierValue ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_value_qualifierValue
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_qualifierValue", "A_value_qualifierValue");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "qualifierValue",
"A_value_qualifierValue-qualifierValue",
"QualifierValue",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "QualifierValue-value A_value_qualifierValue-qualifierValue ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_outputValue_opaqueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_outputValue_opaqueAction", "A_outputValue_opaqueAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "opaqueAction",
"A_outputValue_opaqueAction-opaqueAction",
"OpaqueAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "OpaqueAction-outputValue A_outputValue_opaqueAction-opaqueAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_inputValue_opaqueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inputValue_opaqueAction", "A_inputValue_opaqueAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "opaqueAction",
"A_inputValue_opaqueAction-opaqueAction",
"OpaqueAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "OpaqueAction-inputValue A_inputValue_opaqueAction-opaqueAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_setupPart_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_setupPart_loopNode", "A_setupPart_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_setupPart_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-setupPart A_setupPart_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_bodyPart_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_bodyPart_loopNode", "A_bodyPart_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_bodyPart_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-bodyPart A_bodyPart_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_loopVariable_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_loopVariable_loopNode", "A_loopVariable_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_loopVariable_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-loopVariable A_loopVariable_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_loopNode", "A_result_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_result_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-result A_result_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_bodyOutput_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_bodyOutput_loopNode", "A_bodyOutput_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_bodyOutput_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-bodyOutput A_bodyOutput_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_loopVariableInput_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_loopVariableInput_loopNode", "A_loopVariableInput_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_loopVariableInput_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-loopVariableInput A_loopVariableInput_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_test_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_test_loopNode", "A_test_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_test_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-test A_test_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_decider_loopNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_decider_loopNode", "A_decider_loopNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "loopNode",
"A_decider_loopNode-loopNode",
"LoopNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LoopNode-decider A_decider_loopNode-loopNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_destroyAt_linkEndDestructionData
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_destroyAt_linkEndDestructionData", "A_destroyAt_linkEndDestructionData");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkEndDestructionData",
"A_destroyAt_linkEndDestructionData-linkEndDestructionData",
"LinkEndDestructionData",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkEndDestructionData-destroyAt A_destroyAt_linkEndDestructionData-linkEndDestructionData ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_value_linkEndData
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_linkEndData", "A_value_linkEndData");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkEndData",
"A_value_linkEndData-linkEndData",
"LinkEndData",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkEndData-value A_value_linkEndData-linkEndData ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_end_linkEndData
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_end_linkEndData", "A_end_linkEndData");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkEndData",
"A_end_linkEndData-linkEndData",
"LinkEndData",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkEndData-end A_end_linkEndData-linkEndData ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_qualifier_linkEndData
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_qualifier_linkEndData", "A_qualifier_linkEndData");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkEndData",
"A_qualifier_linkEndData-linkEndData",
"LinkEndData",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkEndData-qualifier A_qualifier_linkEndData-linkEndData ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_insertAt_linkEndCreationData
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_insertAt_linkEndCreationData", "A_insertAt_linkEndCreationData");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkEndCreationData",
"A_insertAt_linkEndCreationData-linkEndCreationData",
"LinkEndCreationData",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkEndCreationData-insertAt A_insertAt_linkEndCreationData-linkEndCreationData ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_inputValue_linkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inputValue_linkAction", "A_inputValue_linkAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkAction",
"A_inputValue_linkAction-linkAction",
"LinkAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkAction-inputValue A_inputValue_linkAction-linkAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_endData_linkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_endData_linkAction", "A_endData_linkAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "linkAction",
"A_endData_linkAction-linkAction",
"LinkAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "LinkAction-endData A_endData_linkAction-linkAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_argument_invocationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_argument_invocationAction", "A_argument_invocationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "invocationAction",
"A_argument_invocationAction-invocationAction",
"InvocationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InvocationAction-argument A_argument_invocationAction-invocationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_onPort_invocationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_onPort_invocationAction", "A_onPort_invocationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "invocationAction",
"A_onPort_invocationAction-invocationAction",
"InvocationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "InvocationAction-onPort A_onPort_invocationAction-invocationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_inputElement_regionAsInput
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_inputElement_regionAsInput", "A_inputElement_regionAsInput");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
factory.createAssociationEnds(assoc, "ExpansionRegion-inputElement ExpansionNode-regionAsInput ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_outputElement_regionAsOutput
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_outputElement_regionAsOutput", "A_outputElement_regionAsOutput");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
factory.createAssociationEnds(assoc, "ExpansionRegion-outputElement ExpansionNode-regionAsOutput ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_target_destroyObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_target_destroyObjectAction", "A_target_destroyObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "destroyObjectAction",
"A_target_destroyObjectAction-destroyObjectAction",
"DestroyObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DestroyObjectAction-target A_target_destroyObjectAction-destroyObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_endData_destroyLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_endData_destroyLinkAction", "A_endData_destroyLinkAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "destroyLinkAction",
"A_endData_destroyLinkAction-destroyLinkAction",
"DestroyLinkAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "DestroyLinkAction-endData A_endData_destroyLinkAction-destroyLinkAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_classifier_createObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_classifier_createObjectAction", "A_classifier_createObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "createObjectAction",
"A_classifier_createObjectAction-createObjectAction",
"CreateObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CreateObjectAction-classifier A_classifier_createObjectAction-createObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_createObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_createObjectAction", "A_result_createObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "createObjectAction",
"A_result_createObjectAction-createObjectAction",
"CreateObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CreateObjectAction-result A_result_createObjectAction-createObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_createLinkObjectAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_createLinkObjectAction", "A_result_createLinkObjectAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "createLinkObjectAction",
"A_result_createLinkObjectAction-createLinkObjectAction",
"CreateLinkObjectAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CreateLinkObjectAction-result A_result_createLinkObjectAction-createLinkObjectAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_endData_createLinkAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_endData_createLinkAction", "A_endData_createLinkAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "createLinkAction",
"A_endData_createLinkAction-createLinkAction",
"CreateLinkAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CreateLinkAction-endData A_endData_createLinkAction-createLinkAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_clause_conditionalNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_clause_conditionalNode", "A_clause_conditionalNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "conditionalNode",
"A_clause_conditionalNode-conditionalNode",
"ConditionalNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, false, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConditionalNode-clause A_clause_conditionalNode-conditionalNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_conditionalNode
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_conditionalNode", "A_result_conditionalNode");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "conditionalNode",
"A_result_conditionalNode-conditionalNode",
"ConditionalNode",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ConditionalNode-result A_result_conditionalNode-conditionalNode ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_clearStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_clearStructuralFeatureAction", "A_result_clearStructuralFeatureAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clearStructuralFeatureAction",
"A_result_clearStructuralFeatureAction-clearStructuralFeatureAction",
"ClearStructuralFeatureAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ClearStructuralFeatureAction-result A_result_clearStructuralFeatureAction-clearStructuralFeatureAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_object_clearAssociationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_object_clearAssociationAction", "A_object_clearAssociationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clearAssociationAction",
"A_object_clearAssociationAction-clearAssociationAction",
"ClearAssociationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ClearAssociationAction-object A_object_clearAssociationAction-clearAssociationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_association_clearAssociationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_association_clearAssociationAction", "A_association_clearAssociationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clearAssociationAction",
"A_association_clearAssociationAction-clearAssociationAction",
"ClearAssociationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ClearAssociationAction-association A_association_clearAssociationAction-clearAssociationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_decider_clause
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_decider_clause", "A_decider_clause");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clause",
"A_decider_clause-clause",
"Clause",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Clause-decider A_decider_clause-clause ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_bodyOutput_clause
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_bodyOutput_clause", "A_bodyOutput_clause");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clause",
"A_bodyOutput_clause-clause",
"Clause",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Clause-bodyOutput A_bodyOutput_clause-clause ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_test_clause
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_test_clause", "A_test_clause");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clause",
"A_test_clause-clause",
"Clause",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Clause-test A_test_clause-clause ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_body_clause
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_body_clause", "A_body_clause");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "clause",
"A_body_clause-clause",
"Clause",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Clause-body A_body_clause-clause ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_predecessorClause_successorClause
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_predecessorClause_successorClause", "A_predecessorClause_successorClause");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
factory.createAssociationEnds(assoc, "Clause-predecessorClause Clause-successorClause ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_target_callOperationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_target_callOperationAction", "A_target_callOperationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "callOperationAction",
"A_target_callOperationAction-callOperationAction",
"CallOperationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CallOperationAction-target A_target_callOperationAction-callOperationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_operation_callOperationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_operation_callOperationAction", "A_operation_callOperationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "callOperationAction",
"A_operation_callOperationAction-callOperationAction",
"CallOperationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CallOperationAction-operation A_operation_callOperationAction-callOperationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_behavior_callBehaviorAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_behavior_callBehaviorAction", "A_behavior_callBehaviorAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "callBehaviorAction",
"A_behavior_callBehaviorAction-callBehaviorAction",
"CallBehaviorAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CallBehaviorAction-behavior A_behavior_callBehaviorAction-callBehaviorAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_callAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_callAction", "A_result_callAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "callAction",
"A_result_callAction-callAction",
"CallAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "CallAction-result A_result_callAction-callAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_signal_broadcastSignalAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_signal_broadcastSignalAction", "A_signal_broadcastSignalAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "broadcastSignalAction",
"A_signal_broadcastSignalAction-broadcastSignalAction",
"BroadcastSignalAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "BroadcastSignalAction-signal A_signal_broadcastSignalAction-broadcastSignalAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_insertAt_addVariableValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_insertAt_addVariableValueAction", "A_insertAt_addVariableValueAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "addVariableValueAction",
"A_insertAt_addVariableValueAction-addVariableValueAction",
"AddVariableValueAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "AddVariableValueAction-insertAt A_insertAt_addVariableValueAction-addVariableValueAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_insertAt_addStructuralFeatureValueAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_insertAt_addStructuralFeatureValueAction", "A_insertAt_addStructuralFeatureValueAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "addStructuralFeatureValueAction",
"A_insertAt_addStructuralFeatureValueAction-addStructuralFeatureValueAction",
"AddStructuralFeatureValueAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "AddStructuralFeatureValueAction-insertAt A_insertAt_addStructuralFeatureValueAction-addStructuralFeatureValueAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_fromAction_actionInputPin
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_fromAction_actionInputPin", "A_fromAction_actionInputPin");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "actionInputPin",
"A_fromAction_actionInputPin-actionInputPin",
"ActionInputPin",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ActionInputPin-fromAction A_fromAction_actionInputPin-actionInputPin ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_output_action
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_output_action", "A_output_action");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "action",
"A_output_action-action",
"Action",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Action-output A_output_action-action ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_context_action
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_context_action", "A_context_action");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "action",
"A_context_action-action",
"Action",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Action-context A_context_action-action ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_input_action
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_input_action", "A_input_action");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "action",
"A_input_action-action",
"Action",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Action-input A_input_action-action ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_localPrecondition_action
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_localPrecondition_action", "A_localPrecondition_action");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "action",
"A_localPrecondition_action-action",
"Action",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Action-localPrecondition A_localPrecondition_action-action ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_localPostcondition_action
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_localPostcondition_action", "A_localPostcondition_action");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "action",
"A_localPostcondition_action-action",
"Action",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "Action-localPostcondition A_localPostcondition_action-action ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_trigger_acceptEventAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_trigger_acceptEventAction", "A_trigger_acceptEventAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "acceptEventAction",
"A_trigger_acceptEventAction-acceptEventAction",
"AcceptEventAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "AcceptEventAction-trigger A_trigger_acceptEventAction-acceptEventAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_acceptEventAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_acceptEventAction", "A_result_acceptEventAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "acceptEventAction",
"A_result_acceptEventAction-acceptEventAction",
"AcceptEventAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "AcceptEventAction-result A_result_acceptEventAction-acceptEventAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_returnInformation_acceptCallAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_returnInformation_acceptCallAction", "A_returnInformation_acceptCallAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "acceptCallAction",
"A_returnInformation_acceptCallAction-acceptCallAction",
"AcceptCallAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "AcceptCallAction-returnInformation A_returnInformation_acceptCallAction-acceptCallAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_value_writeVariableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_writeVariableAction", "A_value_writeVariableAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "writeVariableAction",
"A_value_writeVariableAction-writeVariableAction",
"WriteVariableAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "WriteVariableAction-value A_value_writeVariableAction-writeVariableAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_writeStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_writeStructuralFeatureAction", "A_result_writeStructuralFeatureAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "writeStructuralFeatureAction",
"A_result_writeStructuralFeatureAction-writeStructuralFeatureAction",
"WriteStructuralFeatureAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "WriteStructuralFeatureAction-result A_result_writeStructuralFeatureAction-writeStructuralFeatureAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_value_writeStructuralFeatureAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_writeStructuralFeatureAction", "A_value_writeStructuralFeatureAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "writeStructuralFeatureAction",
"A_value_writeStructuralFeatureAction-writeStructuralFeatureAction",
"WriteStructuralFeatureAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "WriteStructuralFeatureAction-value A_value_writeStructuralFeatureAction-writeStructuralFeatureAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_variable_variableAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_variable_variableAction", "A_variable_variableAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "variableAction",
"A_variable_variableAction-variableAction",
"VariableAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, true, "*");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "VariableAction-variable A_variable_variableAction-variableAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_result_valueSpecificationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_result_valueSpecificationAction", "A_result_valueSpecificationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "valueSpecificationAction",
"A_result_valueSpecificationAction-valueSpecificationAction",
"ValueSpecificationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ValueSpecificationAction-result A_result_valueSpecificationAction-valueSpecificationAction ");
packageId = this.artifact.getUrn() + "#" + "Actions";
// UML.Actions.A_value_valueSpecificationAction
pkg = (Package)model.getElementById(packageId).getDelegate();
assoc = factory.createAssociation("A_value_valueSpecificationAction", "A_value_valueSpecificationAction");
mapping.mapAssociation(assoc, "UML.Actions", this);
// create owned ends
prop = factory.createProperty(assoc, "valueSpecificationAction",
"A_value_valueSpecificationAction-valueSpecificationAction",
"ValueSpecificationAction",
"",
"",
false, false, false);
factory.createLowerValue(prop, true, "");
factory.createUpperValue(prop, false, "");
mapping.mapProperty(assoc, prop, this);
factory.createAssociationEnds(assoc, "ValueSpecificationAction-value A_value_valueSpecificationAction-valueSpecificationAction ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy