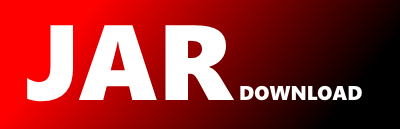
org.modeshape.jcr.index.elasticsearch.Operations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modeshape-elasticsearch-index-provider
Show all versions of modeshape-elasticsearch-index-provider
ModeShape index provider that uses Elasticsearch engine
The newest version!
/*
* ModeShape (http://www.modeshape.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.modeshape.jcr.index.elasticsearch;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Map;
import javax.jcr.query.qom.And;
import javax.jcr.query.qom.BindVariableValue;
import javax.jcr.query.qom.Constraint;
import javax.jcr.query.qom.DynamicOperand;
import javax.jcr.query.qom.LowerCase;
import javax.jcr.query.qom.NodeLocalName;
import javax.jcr.query.qom.NodeName;
import javax.jcr.query.qom.Not;
import javax.jcr.query.qom.PropertyExistence;
import javax.jcr.query.qom.PropertyValue;
import javax.jcr.query.qom.StaticOperand;
import javax.jcr.query.qom.UpperCase;
import org.modeshape.jcr.JcrLexicon;
import org.modeshape.jcr.ModeShapeLexicon;
import org.modeshape.jcr.api.query.qom.Between;
import org.modeshape.jcr.api.query.qom.SetCriteria;
import org.modeshape.jcr.index.elasticsearch.client.EsRequest;
import org.modeshape.jcr.index.elasticsearch.query.AndQuery;
import org.modeshape.jcr.index.elasticsearch.query.BoolQuery;
import org.modeshape.jcr.index.elasticsearch.query.ExistsQuery;
import org.modeshape.jcr.index.elasticsearch.query.MatchAllQuery;
import org.modeshape.jcr.index.elasticsearch.query.MatchQuery;
import org.modeshape.jcr.index.elasticsearch.query.NotQuery;
import org.modeshape.jcr.index.elasticsearch.query.OrQuery;
import org.modeshape.jcr.index.elasticsearch.query.Query;
import org.modeshape.jcr.index.elasticsearch.query.RangeQuery;
import org.modeshape.jcr.index.elasticsearch.query.StringQuery;
import org.modeshape.jcr.index.elasticsearch.query.TermsQuery;
import org.modeshape.jcr.index.elasticsearch.query.WildcardQuery;
import org.modeshape.jcr.query.model.Comparison;
import org.modeshape.jcr.query.model.FullTextSearch;
import org.modeshape.jcr.query.model.Length;
import org.modeshape.jcr.query.model.Literal;
import org.modeshape.jcr.query.model.NodeDepth;
import org.modeshape.jcr.query.model.NodePath;
import org.modeshape.jcr.query.model.Or;
import org.modeshape.jcr.value.ValueFactories;
/**
*
* @author kulikov
*/
@SuppressWarnings("unchecked")
public class Operations {
private final ValueFactories valueFactories;
private final EsIndexColumns columns;
/**
*
* @param columns
*/
public Operations(ValueFactories valueFactories, EsIndexColumns columns) {
this.valueFactories = valueFactories;
this.columns = columns;
}
public EsRequest createQuery(Collection constraints, Map variables) {
BoolQuery query = new BoolQuery();
if (constraints.isEmpty()) {
query.must(new MatchAllQuery());
}
for (Constraint c : constraints) {
query = query.must(build(c, variables));
}
EsRequest res = new EsRequest();
res.put("query", query.build());
return res;
}
public Query build(Constraint constraint, Map variables) {
if (constraint instanceof Between) {
Between between = (Between) constraint;
String field = (String)operand(between.getOperand()).apply(between.getOperand(), variables);
Object low = staticOperand(between.getLowerBound()).apply(field, between.getLowerBound(), variables);
Object high = staticOperand(between.getUpperBound()).apply(field, between.getUpperBound(), variables);
return new RangeQuery(field).from(low).to(high)
.includeLower(between.isLowerBoundIncluded())
.includeUpper(between.isUpperBoundIncluded());
}
if (constraint instanceof Or) {
Or or = (Or)constraint;
return new OrQuery(
build(or.getConstraint1(), variables),
build(or.getConstraint2(), variables));
}
if (constraint instanceof And) {
And and = (And)constraint;
return new AndQuery(
build(and.getConstraint1(), variables),
build(and.getConstraint2(), variables));
}
if (constraint instanceof Not) {
Not not = (Not)constraint;
return new NotQuery(build(not.getConstraint(), variables));
}
if (constraint instanceof Comparison) {
Comparison comp = (Comparison)constraint;
String field = (String) operand(comp.getOperand1()).apply(comp.getOperand1(), variables);
Object value = staticOperand(comp.getOperand2()).apply(field, comp.getOperand2(), variables);
switch (comp.operator()) {
case EQUAL_TO:
return new MatchQuery(field, value);
case GREATER_THAN:
return new RangeQuery(field).gt(value);
case GREATER_THAN_OR_EQUAL_TO:
return new RangeQuery(field).gte(value);
case LESS_THAN:
return new RangeQuery(field).lt(value);
case LESS_THAN_OR_EQUAL_TO:
return new RangeQuery(field).lte(value);
case NOT_EQUAL_TO:
return new NotQuery(new MatchQuery(field, value));
case LIKE:
String queryString = (String)value;
if (!queryString.contains("%")) {
return new StringQuery(queryString);
}
String[] terms = queryString.split(" ");
Query[] termFilters = new Query[terms.length];
for (int i = 0; i < termFilters.length; i++) {
termFilters[i] = terms[i].contains("%") ?
new WildcardQuery(field, terms[i].replaceAll("%", "*")) :
new StringQuery(terms[i]);
}
if (termFilters.length == 1) {
return termFilters[0];
}
Query builder = new AndQuery(termFilters[0],termFilters[1]);
for (int i = 2; i < termFilters.length; i++) {
builder = new AndQuery(builder, termFilters[i]);
}
return builder;
}
}
if (constraint instanceof SetCriteria) {
SetCriteria setCriteria = (SetCriteria)constraint;
String field = (String) operand(setCriteria.getOperand()).apply(setCriteria.getOperand(), variables);
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy