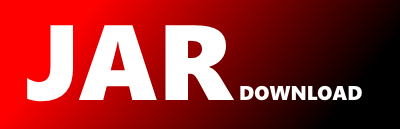
org.modeshape.jcr.query.validate.ImmutableKey Maven / Gradle / Ivy
/*
* ModeShape (http://www.modeshape.org)
* See the COPYRIGHT.txt file distributed with this work for information
* regarding copyright ownership. Some portions may be licensed
* to Red Hat, Inc. under one or more contributor license agreements.
* See the AUTHORS.txt file in the distribution for a full listing of
* individual contributors.
*
* ModeShape is free software. Unless otherwise indicated, all code in ModeShape
* is licensed to you under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* ModeShape is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.modeshape.jcr.query.validate;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import org.modeshape.jcr.query.validate.Schemata.Column;
import org.modeshape.jcr.query.validate.Schemata.Key;
/**
*
*/
public class ImmutableKey implements Key {
private final Set columns;
protected ImmutableKey( Iterable columns ) {
assert columns != null;
Set columnSet = new HashSet();
for (Column column : columns) {
if (column != null) columnSet.add(column);
}
assert !columnSet.isEmpty();
this.columns = Collections.unmodifiableSet(columnSet);
}
protected ImmutableKey( Column... columns ) {
assert columns != null;
assert columns.length > 0;
Set columnSet = new HashSet();
for (Column column : columns) {
if (column != null) columnSet.add(column);
}
assert !columnSet.isEmpty();
this.columns = Collections.unmodifiableSet(columnSet);
}
@Override
public Set getColumns() {
return columns;
}
@Override
public boolean hasColumns( Column... columns ) {
Set keyColumns = new HashSet(this.columns);
for (Column expected : columns) {
if (!keyColumns.remove(expected)) return false;
}
return keyColumns.isEmpty();
}
@Override
public boolean hasColumns( Iterable columns ) {
Set keyColumns = new HashSet(this.columns);
for (Column expected : columns) {
if (!keyColumns.remove(expected)) return false;
}
return keyColumns.isEmpty();
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
boolean first = true;
sb.append('[');
for (Column column : columns) {
if (first) first = false;
else sb.append(", ");
sb.append(column);
}
sb.append(']');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy